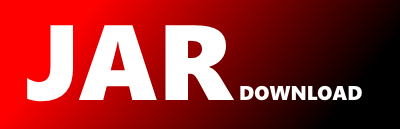
com.github.fluent.hibernate.request.HibernateObjectQuery Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of fluent-hibernate-core Show documentation
Show all versions of fluent-hibernate-core Show documentation
A library to work with Hibernate by fluent API. This library hasn't dependencies except Hibernate dependencies. It requires Java 1.6 and above.
The newest version!
package com.github.fluent.hibernate.request;
import org.hibernate.Session;
import com.github.fluent.hibernate.IRequest;
import com.github.fluent.hibernate.cfg.HibernateSessionFactory;
/**
* @author DoubleF1re
* @author V.Ladynev
*/
public class HibernateObjectQuery {
public static T save(final T entity) {
return HibernateSessionFactory.doInTransaction(new IRequest() {
@Override
public T doInTransaction(Session session) {
session.save(entity);
return entity;
}
});
}
public static T saveOrUpdate(final T entity) {
return HibernateSessionFactory.doInTransaction(new IRequest() {
@Override
public T doInTransaction(Session session) {
session.saveOrUpdate(entity);
return entity;
}
});
}
public static Iterable saveAll(final Iterable entities) {
return HibernateSessionFactory.doInTransaction(new IRequest>() {
@Override
public Iterable doInTransaction(Session session) {
for (T entity : entities) {
session.save(entity);
}
return entities;
}
});
}
public static Iterable saveOrUpdateAll(final Iterable entities) {
return HibernateSessionFactory.doInTransaction(new IRequest>() {
@Override
public Iterable doInTransaction(Session session) {
// TODO need batch update
for (T entity : entities) {
session.saveOrUpdate(entity);
}
return entities;
}
});
}
public static void delete(final T entity) {
HibernateSessionFactory.doInTransaction(new IRequest() {
@Override
public Void doInTransaction(Session session) {
session.delete(entity);
return null;
}
});
}
public static void deleteAll(final Iterable entities) {
HibernateSessionFactory.doInTransaction(new IRequest() {
@Override
public Void doInTransaction(Session session) {
for (T entity : entities) {
session.delete(entity);
}
return null;
}
});
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy