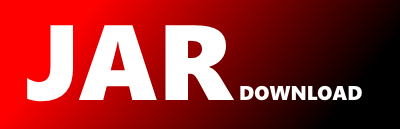
com.github.fluent.hibernate.request.HibernateQuery Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of fluent-hibernate-core Show documentation
Show all versions of fluent-hibernate-core Show documentation
A library to work with Hibernate by fluent API. This library hasn't dependencies except Hibernate dependencies. It requires Java 1.6 and above.
The newest version!
package com.github.fluent.hibernate.request;
import java.util.Arrays;
import java.util.List;
import org.hibernate.Query;
import org.hibernate.Session;
import org.hibernate.transform.ResultTransformer;
import com.github.fluent.hibernate.IRequest;
import com.github.fluent.hibernate.cfg.HibernateSessionFactory;
import com.github.fluent.hibernate.internal.util.InternalUtils;
import com.github.fluent.hibernate.transformer.FluentHibernateResultTransformer;
/**
* @param
* type of return value.
*
* @author V.Ladynev
*/
class HibernateQuery {
private final HibernateQueryParameters params = new HibernateQueryParameters();
private int maxResults;
private ResultTransformer transformer;
private IQueryFactory queryFactory;
public HibernateQuery(IQueryFactory queryFactory) {
this.queryFactory = queryFactory;
}
/**
* Add a named query parameter.
*
* @param name
* name of parameter
* @param val
* parameter value
*/
public HibernateQuery p(String name, Object val) {
params.add(name, val);
return this;
}
public HibernateQuery p(String name, Object... vals) {
params.add(name, Arrays.asList(vals));
return this;
}
public HibernateQuery maxResults(int maxResults) {
this.maxResults = maxResults;
return this;
}
// TODO transformer not works for pid a nested fields
public HibernateQuery transform(Class> clazz) {
transformer = new FluentHibernateResultTransformer(clazz);
return this;
}
public HibernateQuery useTransformer(ResultTransformer transformer) {
this.transformer = transformer;
return this;
}
public T first() {
return InternalUtils.CollectionUtils.first(list());
}
public List list() {
return HibernateSessionFactory.doInTransaction(new IRequest>() {
@Override
public List doInTransaction(Session session) {
return tuneForSelect(createQuery(session)).list();
}
});
}
private Query tuneForSelect(Query hibernateQuery) {
if (maxResults != 0) {
hibernateQuery.setMaxResults(maxResults);
}
if (transformer != null) {
hibernateQuery.setResultTransformer(transformer);
}
return hibernateQuery;
}
// TODO may be return long?
public int count() {
Number result = HibernateSessionFactory.doInTransaction(new IRequest() {
@Override
public Number doInTransaction(Session session) {
return (Number) createQuery(session).uniqueResult();
}
});
return result == null ? 0 : result.intValue();
}
private Query createQuery(Session session) {
Query result = queryFactory.create(session);
params.setParametersToQuery(result);
return result;
}
public interface IQueryFactory {
Query create(Session session);
};
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy