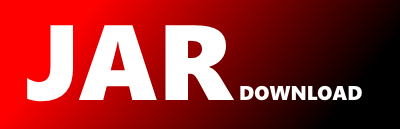
com.github.valdr.ConstraintParser Maven / Gradle / Ivy
package com.github.valdr;
import com.fasterxml.jackson.databind.ObjectMapper;
import com.fasterxml.jackson.databind.ObjectWriter;
import com.fasterxml.jackson.databind.module.SimpleModule;
import com.github.valdr.serializer.MinimalMapSerializer;
import com.google.common.base.Function;
import com.google.common.collect.Iterables;
import lombok.SneakyThrows;
import org.reflections.ReflectionUtils;
import org.slf4j.Logger;
import org.slf4j.LoggerFactory;
import java.io.IOException;
import java.lang.annotation.Annotation;
import java.util.HashMap;
import java.util.Map;
/**
* Parses classes in defined packages for supported Bean Validation (JSR 303)
* annotations ({@code javax.validation.*}) and configured custom annotations. The parsing result is a
* JSON string that complies with the document specified by valdr.
*
* @see BuiltInConstraint
* @see Options
*/
public class ConstraintParser {
private final Logger logger = LoggerFactory.getLogger(ConstraintParser.class);
private final ClasspathScanner classpathScanner;
private final Iterable> allRelevantAnnotationClasses;
private final Options options;
/**
* Constructor.
*
* @param options the only relevant input for the parser is this configuration
*/
public ConstraintParser(Options options) {
this.options = options;
this.classpathScanner = new ClasspathScanner(options);
allRelevantAnnotationClasses = Iterables.concat(BuiltInConstraint.getAllBeanValidationAnnotations(),
getConfiguredCustomAnnotations());
}
/**
* Based on the configuration passed to the constructor model classes are parsed for constraints.
*
* @return JSON string for valdr
*/
public String parse() {
Map classNameToValidationRulesMap = new HashMap<>();
for (Class clazz : classpathScanner.findClassesToParse()) {
if (clazz != null) {
ClassConstraints classValidationRules = new AnnotatedClass(clazz, options.getExcludedFields(),
allRelevantAnnotationClasses).extractValidationRules();
if (classValidationRules.size() > 0) {
String name = options.getOutputFullTypeName() ? clazz.getName() : clazz.getSimpleName();
classNameToValidationRulesMap.put(name, classValidationRules);
}
}
}
return toJson(classNameToValidationRulesMap);
}
@SneakyThrows(IOException.class)
private String toJson(Map classNameToValidationRulesMap) {
ObjectMapper objectMapper = new ObjectMapper();
SimpleModule module = new SimpleModule();
module.addSerializer(MinimalMap.class, new MinimalMapSerializer());
objectMapper.registerModule(module);
ObjectWriter ow = objectMapper.writer().withDefaultPrettyPrinter();
return ow.writeValueAsString(classNameToValidationRulesMap);
}
private Iterable extends Class extends Annotation>> getConfiguredCustomAnnotations() {
return Iterables.transform(options.getCustomAnnotationClasses(), new Function>() {
@Override
@SuppressWarnings("unchecked")
public Class extends Annotation> apply(String className) {
Class> validatorClass = ReflectionUtils.forName(className);
if (validatorClass.isAnnotation()) {
return (Class extends Annotation>) validatorClass;
} else {
logger.warn("The configured custom annotation class '{}' is not an annotation. It will be ignored.",
validatorClass);
return null;
}
}
});
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy