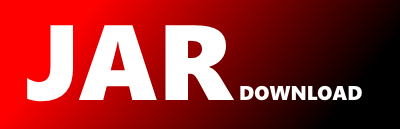
com.github.valdr.MinimalMap Maven / Gradle / Ivy
package com.github.valdr;
import java.util.Map;
import java.util.Set;
/**
* Max-reduced map interface to allow for POJOs that delegate to an internal {@link Map} rather than extend one. The
* map key must a {@link String}.
*
* @param map value type
*/
public interface MinimalMap {
/**
* Returns a {@link Set} view of the mappings contained in this map. The set is backed by the map,
* so changes to the map are reflected in the set, and vice-versa. If the map is modified while an
* iteration over the set is in progress (except through the iterator's own remove operation,
* or through the setValue operation on a map entry returned by the iterator) the results of
* the iteration are undefined. The set supports element removal, which removes the corresponding
* mapping from the map, via the Iterator.remove, Set.remove, removeAll,
* retainAll and clear operations. It does not support the add or
* addAll operations.
*
* @return a set view of the mappings contained in this map
*/
Set> entrySet();
/**
* Associates the specified value with the specified key in this map. If the map previously contained a mapping for
* the key, the old value is replaced by the specified value.
*
* @param key key with which the specified value is to be associated
* @param value value to be associated with the specified key
* @return the previous value associated with key, or null if there was no mapping for
* key. (A null return can also indicate that the map previously associated null
* with
* key, if the implementation supports null values.)
* @throws UnsupportedOperationException if the put operation
* is not supported by this map
* @throws ClassCastException if the class of the specified key or value
* prevents it from being stored in this map
* @throws NullPointerException if the specified key or value is null
* and this map does not permit null keys or values
* @throws IllegalArgumentException if some property of the specified key
* or value prevents it from being stored in this map
*/
V put(String key, V value);
/**
* Returns the number of key-value mappings in this map. If the map contains more than Integer.MAX_VALUE
* elements, returns Integer.MAX_VALUE.
*
* @return the number of key-value mappings in this map
*/
int size();
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy