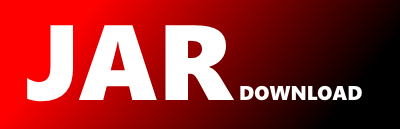
com.github.valfirst.slf4jtest.TestMDCAdapter Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of slf4j-test Show documentation
Show all versions of slf4j-test Show documentation
An in-memory SLF4J implementation focused on aiding unit testing.
package com.github.valfirst.slf4jtest;
import java.util.Collections;
import java.util.HashMap;
import java.util.Map;
import java.util.TreeMap;
import org.slf4j.MDC;
import org.slf4j.helpers.BasicMDCAdapter;
public class TestMDCAdapter extends BasicMDCAdapter {
private final ThreadLocal
© 2015 - 2025 Weber Informatics LLC | Privacy Policy