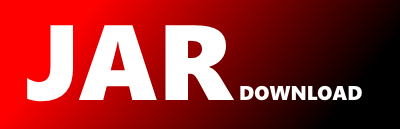
com.github.vatbub.randomusers.result.RandomUser Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of randomusers Show documentation
Show all versions of randomusers Show documentation
API for generating random user data. Like Lorem Ipsum, but for people.
package com.github.vatbub.randomusers.result;
/*-
* #%L
* Random Users
* %%
* Copyright (C) 2017 Frederik Kammel
* %%
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
* #L%
*/
import com.github.vatbub.randomusers.Generator;
import com.github.vatbub.randomusers.Generator.PasswordSpec;
import java.util.Calendar;
import java.util.Date;
import java.util.List;
/**
* Represents a user generated by {@link Generator#generateRandomUser(RandomUserSpec)}
*/
public class RandomUser {
private Gender gender;
private Name name;
private Location location;
private String email;
private Login login;
private Date dateOfBirth;
private Date registrationDate;
private String phone;
private String cell;
private AvatarPicture picture;
private Nationality nationality;
public RandomUser(Gender gender, Name name, Location location, String email, Login login, Date dateOfBirth, Date registrationDate, String phone, String cell, AvatarPicture picture, Nationality nationality) {
setGender(gender);
setName(name);
setLocation(location);
setEmail(email);
setLogin(login);
setDateOfBirth(dateOfBirth);
setRegistrationDate(registrationDate);
setPhone(phone);
setCell(cell);
setPicture(picture);
setNationality(nationality);
}
@Override
public boolean equals(Object obj) {
if (!(obj instanceof RandomUser)) {
return false;
} else {
RandomUser cast = (RandomUser) obj;
boolean tempRes = cast.getGender().equals(getGender()) &&
cast.getName().equals(getName()) &&
cast.getLocation().equals(getLocation()) &&
cast.getEmail().equals(getEmail()) &&
cast.getLogin().equals(getLogin()) &&
cast.getPhone().equals(getPhone()) &&
cast.getCell().equals(getCell()) &&
// TODO cast.getPicture().equals(getPicture()) &&
cast.getNationality().equals(getNationality());
Calendar birthdayCalendar1 = Calendar.getInstance();
birthdayCalendar1.setTime(getDateOfBirth());
Calendar registrationCalendar1 = Calendar.getInstance();
registrationCalendar1.setTime(getRegistrationDate());
Calendar birthdayCalendar2 = Calendar.getInstance();
birthdayCalendar2.setTime(cast.getDateOfBirth());
Calendar registrationCalendar2 = Calendar.getInstance();
registrationCalendar2.setTime(cast.getRegistrationDate());
boolean dateRes = birthdayCalendar1.get(Calendar.YEAR) == birthdayCalendar2.get(Calendar.YEAR) &&
birthdayCalendar1.get(Calendar.MONTH) == birthdayCalendar2.get(Calendar.MONTH) &&
birthdayCalendar1.get(Calendar.DATE) == birthdayCalendar2.get(Calendar.DATE) &&
registrationCalendar1.get(Calendar.YEAR) == registrationCalendar2.get(Calendar.YEAR) &&
registrationCalendar1.get(Calendar.MONTH) == registrationCalendar2.get(Calendar.MONTH) &&
registrationCalendar1.get(Calendar.DATE) == registrationCalendar2.get(Calendar.DATE);
return tempRes && dateRes;
}
}
@Override
public String toString() {
return getName().toString() + ", " + getGender().toString();
}
public Gender getGender() {
return gender;
}
public void setGender(Gender gender) {
this.gender = gender;
}
public Name getName() {
return name;
}
public void setName(Name name) {
this.name = name;
}
public Location getLocation() {
return location;
}
public void setLocation(Location location) {
this.location = location;
}
public String getEmail() {
return email;
}
public void setEmail(String email) {
this.email = email;
}
public Login getLogin() {
return login;
}
public void setLogin(Login login) {
this.login = login;
}
public Date getDateOfBirth() {
return dateOfBirth;
}
public void setDateOfBirth(Date dateOfBirth) {
this.dateOfBirth = dateOfBirth;
}
public Date getRegistrationDate() {
return registrationDate;
}
public void setRegistrationDate(Date registrationDate) {
this.registrationDate = registrationDate;
}
public String getPhone() {
return phone;
}
public void setPhone(String phone) {
this.phone = phone;
}
public String getCell() {
return cell;
}
public void setCell(String cell) {
this.cell = cell;
}
public AvatarPicture getPicture() {
return picture;
}
public void setPicture(AvatarPicture picture) {
this.picture = picture;
}
public Nationality getNationality() {
return nationality;
}
public void setNationality(Nationality nationality) {
this.nationality = nationality;
}
/**
* Specs used to control what types of {@link RandomUser} s are generated using the {@link Generator}.
*
* If any of the fields ({@link Gender}, {@link Nationality}, {@link PasswordSpec} or {@link #setSeed(long) seed}) is not specified using the corresponding setter, a suitable random value will be picked by the {@link Generator}.
* If you add more than one entry to the lists for the gender or nationality, the {@link Generator} will pick a random one among the genders/nationalities you specified in the corresponding list.
*
* @see Generator
* @see Generator#generateRandomUser(RandomUserSpec)
* @see Generator#generateRandomUsers(RandomUserSpec, int)
*/
public static class RandomUserSpec {
private List genders;
private List nationalities;
private PasswordSpec passwordSpec;
private long seed;
public RandomUserSpec() {
this(null, null, null, Long.MIN_VALUE);
}
@SuppressWarnings("SameParameterValue")
public RandomUserSpec(List genders, List nationalities, PasswordSpec passwordSpec, long seed) {
setGenders(genders);
setNationalities(nationalities);
setPasswordSpec(passwordSpec);
setSeed(seed);
}
public List getGenders() {
return genders;
}
public void setGenders(List genders) {
this.genders = genders;
}
public List getNationalities() {
return nationalities;
}
public void setNationalities(List nationalities) {
this.nationalities = nationalities;
}
public PasswordSpec getPasswordSpec() {
return passwordSpec;
}
public void setPasswordSpec(PasswordSpec passwordSpec) {
this.passwordSpec = passwordSpec;
}
public long getSeed() {
return seed;
}
public void setSeed(long seed) {
this.seed = seed;
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy