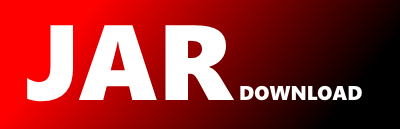
com.datastax.data.exploration.biz.datatable.DataRowCollection Maven / Gradle / Ivy
The newest version!
package com.datastax.data.exploration.biz.datatable;
import java.util.ArrayList;
import java.util.HashMap;
import java.util.List;
import java.util.Map;
/**
* 添加一行数据、根据行号获取行的DataRow
*/
public class DataRowCollection extends ArrayList {
private DataTable table;
DataRowCollection(DataTable table) {
this.table = table;
}
public DataRow add(String[] values) throws Exception {
int count = table.getColumns().size();
if (count < values.length) {
throw new Exception("输入数组长度大于此表中的列数。");
}
for (int i = 0; i < values.length; i++) {
if (values[i] != null) {
this.table.getColumns().getColumn(i).setData(this.size(), values[i]);
} else {
this.table.getColumns().getColumn(i).setData(this.size(), null);
}
}
for (int j = values.length; j < count; j++) {
this.table.getColumns().getColumn(j).setData(this.size(), null);
}
DataRow row = new DataRow(table, this.size());
super.add(row);
return row;
}
public DataRow getRow(int i) throws Exception {
DataRow datarow;
try {
datarow = super.get(i);
} catch (Exception x) {
throw new Exception("此索引处不存在列");
}
return datarow;
}
public Map
© 2015 - 2025 Weber Informatics LLC | Privacy Policy