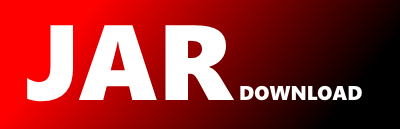
com.activeandroid.Cache Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of activeandroidrx Show documentation
Show all versions of activeandroidrx Show documentation
ActiveAndroid fork with reactive extensions.
package com.activeandroid;
/*
* Copyright (C) 2010 Michael Pardo
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
import android.content.Context;
import android.support.v4.util.LruCache;
import com.activeandroid.serializer.TypeSerializer;
import com.activeandroid.sqlbrite.BriteDatabase;
import com.activeandroid.sqlbrite.SqlBrite;
import com.activeandroid.util.Log;
import java.util.Collection;
public final class Cache {
//////////////////////////////////////////////////////////////////////////////////////
// PUBLIC CONSTANTS
//////////////////////////////////////////////////////////////////////////////////////
public static final int DEFAULT_CACHE_SIZE = 1024;
//////////////////////////////////////////////////////////////////////////////////////
// PRIVATE MEMBERS
//////////////////////////////////////////////////////////////////////////////////////
private static Context sContext;
private static ModelInfo sModelInfo;
private static DatabaseHelper sDatabaseHelper;
private static LruCache sEntities;
private static SqlBrite sSqlBrite;
private static BriteDatabase sDatabase;
private static boolean sIsInitialized = false;
//////////////////////////////////////////////////////////////////////////////////////
// CONSTRUCTORS
//////////////////////////////////////////////////////////////////////////////////////
private Cache() {
}
//////////////////////////////////////////////////////////////////////////////////////
// PUBLIC METHODS
//////////////////////////////////////////////////////////////////////////////////////
public static synchronized void initialize(Configuration configuration) {
if (sIsInitialized) {
Log.v("ActiveAndroid already initialized.");
return;
}
sContext = configuration.getContext();
sModelInfo = new ModelInfo(configuration);
sDatabaseHelper = new DatabaseHelper(configuration);
sSqlBrite = SqlBrite.create();
sDatabase = sSqlBrite.wrapDatabaseHelper(sDatabaseHelper);
// TODO: It would be nice to override sizeOf here and calculate the memory
// actually used, however at this point it seems like the reflection
// required would be too costly to be of any benefit. We'll just set a max
// object size instead.
sEntities = new LruCache(configuration.getCacheSize());
openDatabase();
sIsInitialized = true;
Log.v("ActiveAndroid initialized successfully.");
}
public static synchronized void clear() {
sEntities.evictAll();
Log.v("Cache cleared.");
}
public static synchronized void dispose() {
closeDatabase();
sEntities = null;
sModelInfo = null;
sDatabaseHelper = null;
sIsInitialized = false;
Log.v("ActiveAndroid disposed. Call initialize to use library.");
}
// Database access
public static boolean isInitialized() {
return sIsInitialized;
}
public static synchronized BriteDatabase openDatabase() {
return sDatabase;
}
public static synchronized void closeDatabase() {
sDatabaseHelper.close();
}
// Context access
public static Context getContext() {
return sContext;
}
// Entity cache
public static String getIdentifier(Class extends Model> type, Long id) {
return getTableName(type) + "@" + id;
}
public static String getIdentifier(Model entity) {
return getIdentifier(entity.getClass(), entity.getId());
}
public static synchronized void addEntity(Model entity) {
sEntities.put(getIdentifier(entity), entity);
}
public static synchronized Model getEntity(Class extends Model> type, long id) {
return sEntities.get(getIdentifier(type, id));
}
public static synchronized void removeEntity(Model entity) {
sEntities.remove(getIdentifier(entity));
}
// Model cache
public static synchronized Collection getTableInfos() {
return sModelInfo.getTableInfos();
}
public static synchronized TableInfo getTableInfo(Class extends Model> type) {
return sModelInfo.getTableInfo(type);
}
public static synchronized TypeSerializer getParserForType(Class> type) {
return sModelInfo.getTypeSerializer(type);
}
public static synchronized String getTableName(Class extends Model> type) {
return sModelInfo.getTableInfo(type).getTableName();
}
public static DatabaseHelper getHelper(){
return sDatabaseHelper;
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy