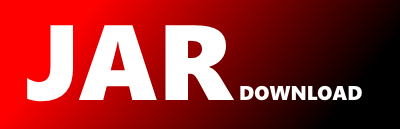
com.activeandroid.sqlbrite.QueryToOneOperator Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of activeandroidrx Show documentation
Show all versions of activeandroidrx Show documentation
ActiveAndroid fork with reactive extensions.
package com.activeandroid.sqlbrite;
import android.database.Cursor;
import rx.Observable;
import rx.Subscriber;
import rx.exceptions.Exceptions;
import rx.exceptions.OnErrorThrowable;
import rx.functions.Func1;
final class QueryToOneOperator implements Observable.Operator {
private final Func1 mapper;
private boolean emitDefault;
private T defaultValue;
QueryToOneOperator(Func1 mapper, boolean emitDefault, T defaultValue) {
this.mapper = mapper;
this.emitDefault = emitDefault;
this.defaultValue = defaultValue;
}
@Override public Subscriber super SqlBrite.Query> call(final Subscriber super T> subscriber) {
return new Subscriber(subscriber) {
@Override public void onNext(SqlBrite.Query query) {
try {
T item = null;
Cursor cursor = query.run();
try {
if (cursor.moveToNext()) {
item = mapper.call(cursor);
if (item == null) {
throw new NullPointerException("Mapper returned null for row 1");
}
if (cursor.moveToNext()) {
throw new IllegalStateException("Cursor returned more than 1 row");
}
}
} finally {
cursor.close();
}
if (!subscriber.isUnsubscribed()) {
if (item != null) {
subscriber.onNext(item);
} else if (emitDefault) {
subscriber.onNext(defaultValue);
}
}
} catch (Throwable e) {
Exceptions.throwIfFatal(e);
onError(OnErrorThrowable.addValueAsLastCause(e, query.toString()));
}
}
@Override public void onCompleted() {
subscriber.onCompleted();
}
@Override public void onError(Throwable e) {
subscriber.onError(e);
}
};
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy