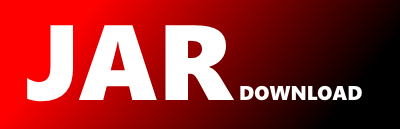
com.github.victools.jsonschema.module.jackson.CustomEnumJsonValueDefinitionProvider Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of jsonschema-module-jackson Show documentation
Show all versions of jsonschema-module-jackson Show documentation
Module for the jsonschema-generator – deriving JSON Schema attributes from jackson annotations
/*
* Copyright 2020 VicTools.
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package com.github.victools.jsonschema.module.jackson;
import com.fasterxml.classmate.ResolvedType;
import com.fasterxml.classmate.members.ResolvedMethod;
import com.fasterxml.jackson.annotation.JsonValue;
import com.fasterxml.jackson.databind.node.ObjectNode;
import com.github.victools.jsonschema.generator.CustomDefinition;
import com.github.victools.jsonschema.generator.CustomDefinitionProviderV2;
import com.github.victools.jsonschema.generator.SchemaGenerationContext;
import com.github.victools.jsonschema.generator.SchemaKeyword;
import com.github.victools.jsonschema.generator.impl.AttributeCollector;
import java.lang.reflect.InvocationTargetException;
import java.util.ArrayList;
import java.util.List;
import java.util.Optional;
import java.util.Set;
import java.util.stream.Collectors;
import java.util.stream.Stream;
/**
* Implementation of the {@link CustomDefinitionProviderV2} interface for treating enum types as plain strings based on a {@link JsonValue} annotation
* being present with {@code value = true} on exactly one argument-free method. If no such annotation exists, no custom definition will be returned;
* thereby falling back on whatever is defined in a following custom definition (e.g. from one of the standard generator {@code Option}s).
*/
public class CustomEnumJsonValueDefinitionProvider implements CustomDefinitionProviderV2 {
@Override
public CustomDefinition provideCustomSchemaDefinition(ResolvedType javaType, SchemaGenerationContext context) {
Object[] enumConstants = javaType.getErasedType().getEnumConstants();
if (enumConstants == null || enumConstants.length == 0) {
return null;
}
ResolvedMethod jsonValueAnnotatedEnumMethod = this.getJsonValueAnnotatedMethod(javaType, context);
if (jsonValueAnnotatedEnumMethod == null) {
return null;
}
List
© 2015 - 2025 Weber Informatics LLC | Privacy Policy