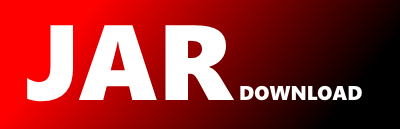
com.smartbear.soapui.spring.boot.SoapuiAutoConfiguration Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of spring-boot-starter-soapui Show documentation
Show all versions of spring-boot-starter-soapui Show documentation
Spring Boot Starter For Soapui
package com.smartbear.soapui.spring.boot;
import java.util.Iterator;
import java.util.Properties;
import javax.annotation.PostConstruct;
import org.slf4j.Logger;
import org.slf4j.LoggerFactory;
import org.springframework.boot.autoconfigure.AutoConfigureAfter;
import org.springframework.boot.autoconfigure.condition.ConditionalOnClass;
import org.springframework.boot.autoconfigure.web.servlet.WebMvcAutoConfiguration;
import org.springframework.boot.context.properties.EnableConfigurationProperties;
import org.springframework.context.annotation.Bean;
import org.springframework.context.annotation.Configuration;
import org.springframework.util.StringUtils;
import com.eviware.soapui.SoapUI;
import com.eviware.soapui.impl.wsdl.WsdlProject;
import com.eviware.soapui.model.environment.DefaultEnvironment;
import com.eviware.soapui.model.environment.Environment;
import com.eviware.soapui.settings.HttpSettings;
import com.eviware.soapui.support.scripting.groovy.SoapUIGroovyScriptEngine;
import com.eviware.soapui.support.scripting.js.JsScriptEngine;
import com.smartbear.soapui.spring.boot.property.EnvironmentProperty;
@Configuration
@ConditionalOnClass(WsdlProject.class)
@AutoConfigureAfter(WebMvcAutoConfiguration.class)
@EnableConfigurationProperties(SoapuiProperties.class)
public class SoapuiAutoConfiguration {
private static final Logger logger = LoggerFactory.getLogger(SoapuiAutoConfiguration.class);
@Bean
public JsScriptEngine jsScriptEngine() throws Exception {
// 创建Javascript脚本解析引擎
JsScriptEngine engine = new JsScriptEngine(getClass().getClassLoader());
return engine;
}
@Bean
public SoapUIGroovyScriptEngine groovyScriptEngine() throws Exception {
// 创建Groovy脚本解析引擎
SoapUIGroovyScriptEngine engine = new SoapUIGroovyScriptEngine(getClass().getClassLoader());
return engine;
}
@Bean
public Environment soapEnv(SoapuiProperties properties) throws Exception {
Environment env = DefaultEnvironment.getInstance();
EnvironmentProperty envProperties = properties.getEnv();
env.setName(envProperties.getName());
Properties pro = envProperties.getSettings();
Iterator
© 2015 - 2025 Weber Informatics LLC | Privacy Policy