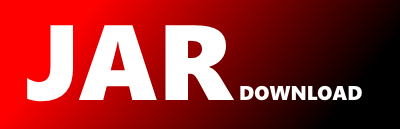
com.github.vindell.websocket.session.handler.chain.def.DefaultHandlerChainManager Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of spring-boot-starter-websocket Show documentation
Show all versions of spring-boot-starter-websocket Show documentation
Spring Boot Starter For WebSocket
The newest version!
package com.github.vindell.websocket.session.handler.chain.def;
import java.util.Collections;
import java.util.LinkedHashMap;
import java.util.Map;
import java.util.Set;
import org.slf4j.Logger;
import org.slf4j.LoggerFactory;
import org.springframework.util.CollectionUtils;
import com.github.vindell.websocket.event.WebSocketMessageEvent;
import com.github.vindell.websocket.session.handler.Nameable;
import com.github.vindell.websocket.session.handler.NamedHandlerList;
import com.github.vindell.websocket.session.handler.WebSocketMessageHandler;
import com.github.vindell.websocket.session.handler.chain.HandlerChain;
import com.github.vindell.websocket.session.handler.chain.HandlerChainManager;
import com.github.vindell.websocket.utils.StringUtils;
public class DefaultHandlerChainManager implements HandlerChainManager {
private static transient final Logger log = LoggerFactory.getLogger(DefaultHandlerChainManager.class);
private Map> handlers;
private Map> handlerChains;
private final static String DEFAULT_CHAIN_DEFINATION_DELIMITER_CHAR = ",";
public DefaultHandlerChainManager() {
this.handlers = new LinkedHashMap>();
this.handlerChains = new LinkedHashMap>();
}
@Override
public Map> getHandlers() {
return handlers;
}
public void setHandlers(Map> handlers) {
this.handlers = handlers;
}
public Map> getHandlerChains() {
return handlerChains;
}
public void setHandlerChains(Map> handlerChains) {
this.handlerChains = handlerChains;
}
public WebSocketMessageHandler getHandler(String name) {
return this.handlers.get(name);
}
@Override
public void addHandler(String name, WebSocketMessageHandler handler) {
addHandler(name, handler, true);
}
protected void addHandler(String name, WebSocketMessageHandler handler, boolean overwrite) {
WebSocketMessageHandler existing = getHandler(name);
if (existing == null || overwrite) {
if (handler instanceof Nameable) {
((Nameable) handler).setName(name);
}
this.handlers.put(name, handler);
}
}
@Override
public void createChain(String chainName, String chainDefinition) {
if (StringUtils.isBlank(chainName)) {
throw new NullPointerException("chainName cannot be null or empty.");
}
if (StringUtils.isBlank(chainDefinition)) {
throw new NullPointerException("chainDefinition cannot be null or empty.");
}
if (log.isDebugEnabled()) {
log.debug("Creating chain [" + chainName + "] from String definition [" + chainDefinition + "]");
}
String[] handlerTokens = splitChainDefinition(chainDefinition);
for (String token : handlerTokens) {
addToChain(chainName, token);
}
}
/*
* Splits the comma-delimited handler chain definition line into individual handler definition tokens.
*/
protected String[] splitChainDefinition(String chainDefinition) {
String trimToNull = StringUtils.trimToNull(chainDefinition);
if(trimToNull == null){
return null;
}
String[] split = StringUtils.splits(trimToNull, DEFAULT_CHAIN_DEFINATION_DELIMITER_CHAR);
for (int i = 0; i < split.length; i++) {
split[i] = StringUtils.trimToNull(split[i]);
}
return split;
}
public static void main(String[] args) {
}
@Override
public void addToChain(String chainName, String handlerName) {
if (!StringUtils.hasText(chainName)) {
throw new IllegalArgumentException("chainName cannot be null or empty.");
}
WebSocketMessageHandler handler = getHandler(handlerName);
if (handler == null) {
throw new IllegalArgumentException("There is no handler with name '" + handlerName +
"' to apply to chain [" + chainName + "] in the pool of available Handlers. Ensure a " +
"handler with that name/path has first been registered with the addHandler method(s).");
}
NamedHandlerList chain = ensureChain(chainName);
chain.add(handler);
}
protected NamedHandlerList ensureChain(String chainName) {
NamedHandlerList chain = getChain(chainName);
if (chain == null) {
chain = new DefaultNamedHandlerList(chainName);
this.handlerChains.put(chainName, chain);
}
return chain;
}
@Override
public NamedHandlerList getChain(String chainName) {
return this.handlerChains.get(chainName);
}
@Override
public boolean hasChains() {
return !CollectionUtils.isEmpty(this.handlerChains);
}
@SuppressWarnings("unchecked")
@Override
public Set getChainNames() {
return this.handlerChains != null ? this.handlerChains.keySet() : Collections.EMPTY_SET;
}
@Override
public HandlerChain proxy(HandlerChain original, String chainName) {
NamedHandlerList configured = getChain(chainName);
if (configured == null) {
String msg = "There is no configured chain under the name/key [" + chainName + "].";
throw new IllegalArgumentException(msg);
}
return configured.proxy(original);
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy