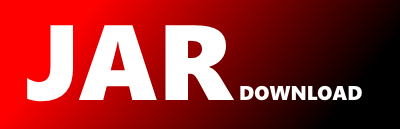
com.cc.common.mybatis.Pageable Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of common Show documentation
Show all versions of common Show documentation
A concise description of my library
package com.cc.common.mybatis;
import com.cc.common.exception.BadUserInputException;
import com.cc.common.exception.BasedException;
import java.util.HashMap;
import java.util.Map;
public class Pageable {
private static final int LIMIT = 150;
private int limit;
private int offset;
public Pageable() {
}
public Pageable(int limit, int offset) throws BasedException {
Map errors = new HashMap<>();
if (offset < 0) {
errors.put("offset", "invalid user input");
}
if (limit > LIMIT) {
errors.put("limit", String.format("invalid user input limit > %d", LIMIT));
}
if (!errors.isEmpty()) {
throw new BadUserInputException(errors);
}
this.limit = limit;
this.offset = offset;
}
public int getLimit() {
return limit;
}
public void setLimit(int limit) throws BasedException {
if (limit > LIMIT) {
Map error = Map.of("limit", String.format("invalid user input limit > %d", LIMIT));
throw new BadUserInputException(error);
}
this.limit = limit;
}
public int getOffset() {
return offset;
}
public void setOffset(int offset) throws BasedException {
if (offset < 0) {
throw new BadUserInputException(Map.of("offset", "invalid user input"));
}
this.offset = offset;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy