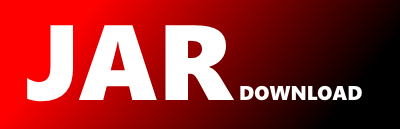
cl.core.util.Sets Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of cl-core Show documentation
Show all versions of cl-core Show documentation
Tools for the busy Java developer
The newest version!
package cl.core.util;
import java.util.Collection;
import java.util.HashSet;
import java.util.Set;
import java.util.function.Supplier;
/**
* Simple set operations
*/
public final class Sets {
private Sets() {}
/**
* Return a union of two collections as a set. The original collections will
* not be modified.
*
* @param a Collection a
* @param b Collection b
* @param setFactory Set constructor
* @return the union of two collections
*/
public static Set union(Collection a, Collection b, Supplier> setFactory) {
Set result = createSet(a, setFactory);
result.addAll(b);
return result;
}
/**
* Return a union of two collections as a set. The original collections will
* not be modified.
*
* @param a Collection a
* @param b Collection b
* @return the union of two collections
*/
public static Set union(Collection a, Collection b) {
return union(a, b, defaultSetFactory());
}
/**
* Return an intersection of two collections as a set. The original collections will
* not be modified.
*
* @param a Collection a
* @param b Collection b
* @param setFactory Set constructor
* @return the intersection of two collections
*/
public static Set intersection(Collection a, Collection b, Supplier> setFactory) {
Set result = createSet(a, setFactory);
result.retainAll(b);
return result;
}
/**
* Return an intersection of two collections as a set. The original collections will
* not be modified.
*
* @param a Collection a
* @param b Collection b
* @return the intersection of two collections
*/
public static Set intersection(Collection a, Collection b) {
return intersection(a, b, defaultSetFactory());
}
/**
* Return a difference of two collections as a set. The original collections will
* not be modified.
*
* @param a Collection a
* @param b Collection b
* @param setFactory Set constructor
* @return the difference of two collections
*/
public static Set difference(Collection a, Collection b, Supplier> setFactory) {
Set result = createSet(a, setFactory);
result.removeAll(b);
return result;
}
/**
* Return a difference of two collections as a set. The original collections will
* not be modified.
*
* @param a Collection a
* @param b Collection b
* @return the difference of two collections
*/
public static Set difference(Collection a, Collection b) {
return difference(a, b, defaultSetFactory());
}
/**
* Return a complement of two collections as a set. The original collections will
* not be modified.
*
* @param a Collection a
* @param b Collection b
* @param setFactory Set constructor
* @return the complement of two collections
*/
public static Set complement(Collection a, Collection b, Supplier> setFactory) {
return difference(union(a, b, setFactory), intersection(a, b, setFactory), setFactory);
}
/**
* Return a complement of two collections as a set. The original collections will
* not be modified.
*
* @param a Collection a
* @param b Collection b
* @return the complement of two collections
*/
public static Set complement(Collection a, Collection b) {
return complement(a, b, defaultSetFactory());
}
private static Set createSet(Collection col, Supplier> setFactory) {
Set result = setFactory.get();
result.addAll(col);
return result;
}
private static Supplier> defaultSetFactory() {
return HashSet::new;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy