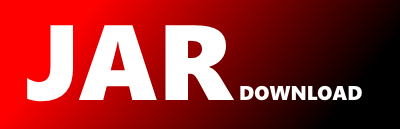
com.github.vskrahul.request.HttpRequest Maven / Gradle / Ivy
package com.github.vskrahul.request;
import java.util.ArrayList;
import java.util.List;
import java.util.stream.Collectors;
import com.github.vskrahul.method.HttpMethod;
public class HttpRequest {
private String body;
private List param;
private List queryParam;
private HttpHeader header;
private HttpMethod method;
private String url;
private boolean trace;
public HttpRequest(HttpMethod method, String url) {
this.method = method;
this.url = url;
this.header = new HttpHeader();
this.param = new ArrayList<>();
this.queryParam = new ArrayList<>();
}
public String getBody() {
body = param.stream()
.map(Parameter::toString)
.collect(Collectors.joining("&"));
return body;
}
public void setBody(String body) {
this.body = body;
}
public HttpHeader getHeader() {
return header;
}
public HttpMethod getMethod() {
return method;
}
public void param(String key, String value) {
param.add(new Parameter(key, value));
}
public void queryParam(String key, String value) {
queryParam.add(new QueryParameter(key, value));
}
public List getParam() {
return param;
}
public List getQueryParam() {
return queryParam;
}
public boolean traceFlag() {
return trace;
}
public void trace() {
trace = true;
}
public String url() {
if(!queryParam.isEmpty())
this.url = this.url + queryParam.stream().map(QueryParameter::toString).collect(Collectors.joining("&", "?", ""));
return this.url;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy