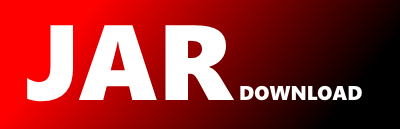
com.carrotsearch.hppcrt.CharIndexedContainer Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of hppcrt Show documentation
Show all versions of hppcrt Show documentation
High Performance Primitive Collections Realtime
(fork of HPPC of Carrotsearch)
Fundamental data structures (maps, sets, lists, stacks, queues, heaps, sorts) generated for
combinations of object and primitive types to conserve JVM memory and speed
up execution. The Realtime fork intend of extending collections while tweaking and optimizations to remove any dynamic allocations at runtime,
and low variance execution times.
package com.carrotsearch.hppcrt;
import java.util.List;
import java.util.RandomAccess;
/**
* An indexed container provides random access to elements based on an
* index
. Indexes are zero-based.
*/
@javax.annotation.Generated(date = "2015-02-27T19:21:18+0100", value = "HPPC-RT generated from: CharIndexedContainer.java")
public interface CharIndexedContainer extends CharCollection, RandomAccess
{
/**
* Removes the first element that equals e1
, returning its
* deleted position or -1
if the element was not found.
*/
public int removeFirstOccurrence(char e1);
/**
* Removes the last element that equals e1
, returning its
* deleted position or -1
if the element was not found.
*/
public int removeLastOccurrence(char e1);
/**
* Returns the index of the first occurrence of the specified element in this list,
* or -1 if this list does not contain the element.
*/
public int indexOf(char e1);
/**
* Returns the index of the last occurrence of the specified element in this list,
* or -1 if this list does not contain the element.
*/
public int lastIndexOf(char e1);
/**
* Adds an element to the end of this container (the last index is incremented by one).
*/
public void add(char e1);
/**
* Inserts the specified element at the specified position in this list.
*
* @param index The index at which the element should be inserted, shifting
* any existing and subsequent elements to the right.
* Precondition : index must be valid !
*/
public void insert(int index, char e1);
/**
* Replaces the element at the specified position in this list
* with the specified element.
* Precondition : index must be valid !
* @return Returns the previous value in the list.
*/
public char set(int index, char e1);
/**
* @return Returns the element at index index
from the list.
* Precondition : index must be valid !
*/
public char get(int index);
/**
* Removes the element at the specified position in this list and returns it.
* Precondition : index must be valid !
* Careful. Do not confuse this method with the overridden signature in
* Java Collections ({@link List#remove(Object)}). Use: {@link #removeAll},
* {@link #removeFirstOccurrence} or {@link #removeLastOccurrence} depending
* on the actual need.
*/
public char remove(int index);
/**
* Removes from this list all of the elements whose index is between
* fromIndex
, inclusive, and toIndex
, exclusive.
* Precondition : both indices must be valid !
*/
public void removeRange(int fromIndex, int toIndex);
/**
* Compares the specified object with this container for equality. Returns
* true if and only if the specified object is also a
* {@link CharIndexedContainer}, both have the same size, and all corresponding
* pairs of elements at the same index are equal. In other words, two indexed
* containers are defined to be equal if they contain the same elements in the same
* order.
*
* Note that, unlike in {@link List}, containers may be of different types and still
* return true
from {@link #equals}. This may be dangerous if you use
* different hash functions in two containers, but don't override the default
* implementation of {@link #equals}. It is the programmer's responsibility to
* enforcing these contracts properly.
*
*/
public boolean equals(Object obj);
/**
* @return A hash code of elements stored in the container. The hash code
* is defined identically to {@link List#hashCode()} (should be implemented
* with the same algorithm).
*/
public int hashCode();
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy