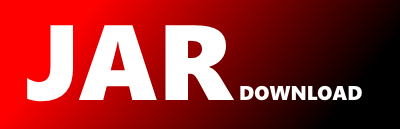
com.carrotsearch.hppcrt.sets.ShortOpenHashSet Maven / Gradle / Ivy
Show all versions of hppcrt Show documentation
package com.carrotsearch.hppcrt.sets;
import java.util.*;
import com.carrotsearch.hppcrt.*;
import com.carrotsearch.hppcrt.cursors.*;
import com.carrotsearch.hppcrt.predicates.*;
import com.carrotsearch.hppcrt.procedures.*;
import com.carrotsearch.hppcrt.hash.*;
// If RH is defined, RobinHood Hashing is in effect
/**
* A hash set of short
s, implemented using using open
* addressing with linear probing for collision resolution.
*
*
* The internal buffers of this implementation ({@link #keys}, etc...)
* are always allocated to the nearest size that is a power of two. When
* the capacity exceeds the given load factor, the buffer size is doubled.
*
* See {@link ObjectOpenHashSet} class for API similarities and differences against Java
* Collections.
*
*
* @author This code is inspired by the collaboration and implementation in the fastutil project.
*
*
*/
@javax.annotation.Generated(date = "2015-02-27T19:21:17+0100", value = "HPPC-RT generated from: ShortOpenHashSet.java")
public class ShortOpenHashSet
extends AbstractShortCollection
implements ShortLookupContainer, ShortSet, Cloneable
{
/**
* Minimum capacity for the map.
*/
public final static int MIN_CAPACITY = HashContainerUtils.MIN_CAPACITY;
/**
* Default capacity.
*/
public final static int DEFAULT_CAPACITY = HashContainerUtils.DEFAULT_CAPACITY;
/**
* Default load factor.
*/
public final static float DEFAULT_LOAD_FACTOR = HashContainerUtils.DEFAULT_LOAD_FACTOR;
/**
* Hash-indexed array holding all set entries.
*
* Direct set iteration: iterate {keys[i]} for i in [0; keys.length[ where keys[i] != 0/null, then also
* {0/null} is in the set if {@link #allocatedDefaultKey} = true.
*
*
* Direct iteration warning:
* If the iteration goal is to fill another hash container, please iterate {@link #keys} in reverse to prevent performance losses.
* @see #keys
*/
public short[] keys;
/**
*True if key = 0/null is in the map.
*/
public boolean allocatedDefaultKey = false;
/**
* Cached number of assigned slots in {@link #keys}.
*/
protected int assigned;
/**
* The load factor for this map (fraction of allocated slots
* before the buffers must be rehashed or reallocated).
*/
protected float loadFactor;
/**
* Resize buffers when {@link #keys} hits this value.
*/
protected int resizeAt;
/**
* The most recent slot accessed in {@link #contains}.
*
* @see #contains
* @see #lkey
*/
protected int lastSlot;
/**
* Creates a hash set with the default capacity of {@value #DEFAULT_CAPACITY},
* load factor of {@value #DEFAULT_LOAD_FACTOR}.
` */
public ShortOpenHashSet()
{
this(ShortOpenHashSet.DEFAULT_CAPACITY, ShortOpenHashSet.DEFAULT_LOAD_FACTOR);
}
/**
* Creates a hash set with the given capacity,
* load factor of {@value #DEFAULT_LOAD_FACTOR}.
*/
public ShortOpenHashSet(final int initialCapacity)
{
this(initialCapacity, ShortOpenHashSet.DEFAULT_LOAD_FACTOR);
}
/**
* Creates a hash set with the given capacity and load factor.
*/
public ShortOpenHashSet(final int initialCapacity, final float loadFactor)
{
assert loadFactor > 0 && loadFactor <= 1 : "Load factor must be between (0, 1].";
this.loadFactor = loadFactor;
//take into account of the load factor to garantee no reallocations before reaching initialCapacity.
int internalCapacity = (int) (initialCapacity / loadFactor) + ShortOpenHashSet.MIN_CAPACITY;
//align on next power of two
internalCapacity = HashContainerUtils.roundCapacity(internalCapacity);
this.keys = (new short[internalCapacity]);
//Take advantage of the rounding so that the resize occur a bit later than expected.
//allocate so that there is at least one slot that remains allocated = false
//this is compulsory to guarantee proper stop in searching loops
this.resizeAt = Math.max(3, (int) (internalCapacity * loadFactor)) - 2;
}
/**
* Creates a hash set from elements of another container. Default load factor is used.
*/
public ShortOpenHashSet(final ShortContainer container)
{
this(container.size());
addAll(container);
}
/**
* {@inheritDoc}
*/
@Override
public boolean add(short e)
{
if (e == ((short)0)) {
if (this.allocatedDefaultKey) {
return false;
}
this.allocatedDefaultKey = true;
return true;
}
final int mask = this.keys.length - 1;
final short[] keys = this.keys;
//copied straight from fastutil "fast-path"
int slot;
short curr;
//1.1 The rehashed key slot is occupied...
if ((curr = keys[slot = (PhiMix.hash(e)) & mask]) != ((short)0)) {
//1.2 the occupied place is indeed key, return false
if ((curr == e)) {
return false;
}
//1.3 key is colliding, manage below :
}
else if (this.assigned < this.resizeAt) {
//1.4 key is not colliding, without resize, so insert, return true.
keys[slot] = e;
this.assigned++;
return true;
}
while ((keys[slot] != ((short)0)))
{
if ((e == keys[slot]))
{
return false;
}
slot = (slot + 1) & mask;
}
// Check if we need to grow. If so, reallocate new data,
// fill in the last element and rehash.
if (this.assigned == this.resizeAt) {
expandAndAdd(e, slot);
}
else {
this.assigned++;
keys[slot] = e;
}
return true;
}
/**
* Adds two elements to the set.
*/
public int add(final short e1, final short e2)
{
int count = 0;
if (add(e1)) {
count++;
}
if (add(e2)) {
count++;
}
return count;
}
/**
* Vararg-signature method for adding elements to this set.
*
This method is handy, but costly if used in tight loops (anonymous
* array passing)
*
* @return Returns the number of elements that were added to the set
* (were not present in the set).
*/
public int add(final short... elements)
{
int count = 0;
for (final short e : elements) {
if (add(e)) {
count++;
}
}
return count;
}
/**
* Adds all elements from a given container to this set.
*
* @return Returns the number of elements actually added as a result of this
* call (not previously present in the set).
*/
public int addAll(final ShortContainer container)
{
return addAll((Iterable extends ShortCursor>) container);
}
/**
* Adds all elements from a given iterable to this set.
*
* @return Returns the number of elements actually added as a result of this
* call (not previously present in the set).
*/
public int addAll(final Iterable extends ShortCursor> iterable)
{
int count = 0;
for (final ShortCursor cursor : iterable)
{
if (add(cursor.value)) {
count++;
}
}
return count;
}
/**
* Expand the internal storage buffers (capacity) or rehash current
* keys and values if there are a lot of deleted slots.
*/
private void expandAndAdd(final short pendingKey, final int freeSlot)
{
assert this.assigned == this.resizeAt;
//default sentinel value is never in the keys[] array, so never trigger reallocs
assert (pendingKey != ((short)0));
// Try to allocate new buffers first. If we OOM, it'll be now without
// leaving the data structure in an inconsistent state.
final short[] oldKeys = this.keys;
allocateBuffers(HashContainerUtils.nextCapacity(this.keys.length));
// We have succeeded at allocating new data so insert the pending key/value at
// the free slot in the old arrays before rehashing.
this.lastSlot = -1;
this.assigned++;
oldKeys[freeSlot] = pendingKey;
//Variables for adding
final int mask = this.keys.length - 1;
short e = ((short)0);
//adding phase
int slot = -1;
final short[] keys = this.keys;
//iterate all the old arrays to add in the newly allocated buffers
//It is important to iterate backwards to minimize the conflict chain length !
for (int i = oldKeys.length; --i >= 0;)
{
if ((oldKeys[i] != ((short)0)))
{
e = oldKeys[i];
slot = (PhiMix.hash(e)) & mask;
while ((keys[slot] != ((short)0)))
{
slot = (slot + 1) & mask;
} //end while
//place it at that position
keys[slot] = e;
}
}
}
/**
* Allocate internal buffers for a given capacity.
*
* @param capacity New capacity (must be a power of two).
*/
private void allocateBuffers(final int capacity)
{
final short[] keys = (new short[capacity]);
this.keys = keys;
//allocate so that there is at least one slot that remains allocated = false
//this is compulsory to guarantee proper stop in searching loops
this.resizeAt = Math.max(3, (int) (capacity * this.loadFactor)) - 2;
}
/**
* {@inheritDoc}
*/
@Override
public int removeAllOccurrences(final short key)
{
return remove(key) ? 1 : 0;
}
/**
* An alias for the (preferred) {@link #removeAllOccurrences}.
*/
public boolean remove(final short key)
{
if (key == ((short)0)) {
if (this.allocatedDefaultKey) {
this.allocatedDefaultKey = false;
return true;
}
return false;
}
final int mask = this.keys.length - 1;
final short[] keys = this.keys;
//copied straight from fastutil "fast-path"
int slot;
short curr;
//1.1 The rehashed slot is free, nothing to remove, return false
if ((curr = keys[slot = (PhiMix.hash(key)) & mask]) == ((short)0)) {
return false;
}
//1.2) The rehashed entry is occupied by the key, remove it, return true
if ((curr == key)) {
this.assigned--;
shiftConflictingKeys(slot);
return true;
}
//2. Hash collision, search for the key along the path
slot = (slot + 1) & mask;
while ((keys[slot] != ((short)0))
)
{
if ((key == keys[slot]))
{
this.assigned--;
shiftConflictingKeys(slot);
return true;
}
slot = (slot + 1) & mask;
} //end while true
return false;
}
/**
* Shift all the slot-conflicting keys allocated to (and including) slot
.
*/
protected void shiftConflictingKeys(int slotCurr)
{
// Copied nearly verbatim from fastutil's impl.
final int mask = this.keys.length - 1;
int slotPrev, slotOther;
final short[] keys = this.keys;
while (true)
{
slotCurr = ((slotPrev = slotCurr) + 1) & mask;
while ((keys[slotCurr] != ((short)0)))
{
slotOther = (PhiMix.hash(keys[slotCurr])) & mask;
if (slotPrev <= slotCurr)
{
// We are on the right of the original slot.
if (slotPrev >= slotOther || slotOther > slotCurr) {
break;
}
}
else
{
// We have wrapped around.
if (slotPrev >= slotOther && slotOther > slotCurr) {
break;
}
}
slotCurr = (slotCurr + 1) & mask;
}
if (!(keys[slotCurr] != ((short)0)))
{
break;
}
// Shift key/allocated pair.
keys[slotPrev] = keys[slotCurr];
}
//means not allocated and for GC
keys[slotPrev] = ((short)0);
}
/**
* Returns the last key saved in a call to {@link #contains} if it returned true
.
* Precondition : {@link #contains} must have been called previously !
* @see #contains
*/
public short lkey()
{
if (this.lastSlot == -2) {
return ((short)0);
}
assert this.lastSlot >= 0 : "Call containsKey() first.";
assert (this.keys[this.lastSlot] != ((short)0)) : "Last call to exists did not have any associated value.";
return this.keys[this.lastSlot];
}
/**
* @return Returns the slot of the last key looked up in a call to {@link #contains} if
* it returned true
.
* or else -2 if {@link #contains} were succesfull on key = 0/null
* @see #contains
*/
public int lslot()
{
assert this.lastSlot >= 0 || this.lastSlot == -2 : "Call contains() first.";
return this.lastSlot;
}
/**
* {@inheritDoc}
*
* */
@Override
public boolean contains(final short key)
{
if (key == ((short)0)) {
if (this.allocatedDefaultKey) {
this.lastSlot = -2;
}
else {
this.lastSlot = -1;
}
return this.allocatedDefaultKey;
}
final int mask = this.keys.length - 1;
final short[] keys = this.keys;
//copied straight from fastutil "fast-path"
int slot;
short curr;
//1.1 The rehashed slot is free, return false
if ((curr = keys[slot = (PhiMix.hash(key)) & mask]) == ((short)0)) {
this.lastSlot = -1;
return false;
}
//1.2) The rehashed entry is occupied by the key, return true
if ((curr == key)) {
this.lastSlot = slot;
return true;
}
//2. Hash collision, search for the key along the path
slot = (slot + 1) & mask;
while ((keys[slot] != ((short)0))
)
{
if ((key == keys[slot]))
{
this.lastSlot = slot;
return true;
}
slot = (slot + 1) & mask;
} //end while true
//unsuccessful search
this.lastSlot = -1;
return false;
}
/**
* {@inheritDoc}
*
* Does not release internal buffers.
*/
@Override
public void clear()
{
this.assigned = 0;
this.lastSlot = -1;
// States are always cleared.
this.allocatedDefaultKey = false;
//Faster than Arrays.fill(keys, null); // Help the GC.
ShortArrays.blankArray(this.keys, 0, this.keys.length);
}
/**
* {@inheritDoc}
*/
@Override
public int size()
{
return this.assigned + (this.allocatedDefaultKey ? 1 : 0);
}
/**
* {@inheritDoc}
*/
@Override
public int capacity() {
return this.resizeAt - 1;
}
/**
* {@inheritDoc}
*/
@Override
public int hashCode()
{
int h = 0;
if (this.allocatedDefaultKey) {
h += 0;
}
final short[] keys = this.keys;
for (int i = keys.length; --i >= 0;)
{
if ((keys[i] != ((short)0)))
{
h += (PhiMix.hash(keys[i]));
}
}
return h;
}
/**
* {@inheritDoc}
*/
@Override
public boolean equals(final Object obj)
{
if (obj != null)
{
if (obj == this) {
return true;
}
if (!(obj instanceof ShortOpenHashSet)) {
return false;
}
@SuppressWarnings("unchecked")
final ShortSet other = (ShortSet) obj;
if (other.size() == this.size())
{
final EntryIterator it = this.iterator();
while (it.hasNext())
{
if (!other.contains(it.next().value))
{
//recycle
it.release();
return false;
}
}
return true;
}
}
return false;
}
/**
* An iterator implementation for {@link #iterator}.
*/
public final class EntryIterator extends AbstractIterator
{
public final ShortCursor cursor;
public EntryIterator()
{
this.cursor = new ShortCursor();
this.cursor.index = -2;
}
/**
* Iterate backwards w.r.t the buffer, to
* minimize collision chains when filling another hash container (ex. with putAll())
*/
@Override
protected ShortCursor fetch()
{
if (this.cursor.index == ShortOpenHashSet.this.keys.length + 1) {
if (ShortOpenHashSet.this.allocatedDefaultKey) {
this.cursor.index = ShortOpenHashSet.this.keys.length;
this.cursor.value = ((short)0);
return this.cursor;
}
//no value associated with the default key, continue iteration...
this.cursor.index = ShortOpenHashSet.this.keys.length;
}
int i = this.cursor.index - 1;
while (i >= 0 &&
!(ShortOpenHashSet.this.keys[i] != ((short)0)))
{
i--;
}
if (i == -1) {
return done();
}
this.cursor.index = i;
this.cursor.value = ShortOpenHashSet.this.keys[i];
return this.cursor;
}
}
/**
* internal pool of EntryIterator
*/
protected final IteratorPool entryIteratorPool = new IteratorPool(
new ObjectFactory() {
@Override
public EntryIterator create() {
return new EntryIterator();
}
@Override
public void initialize(final EntryIterator obj) {
obj.cursor.index = ShortOpenHashSet.this.keys.length + 1;
}
@Override
public void reset(final EntryIterator obj) {
// nothing
}
});
/**
* {@inheritDoc}
* @return
*/
@Override
public EntryIterator iterator()
{
//return new EntryIterator();
return this.entryIteratorPool.borrow();
}
/**
* {@inheritDoc}
*/
@Override
public T forEach(final T procedure)
{
if (this.allocatedDefaultKey) {
procedure.apply(((short)0));
}
final short[] keys = this.keys;
//Iterate in reverse for side-stepping the longest conflict chain
//in another hash, in case apply() is actually used to fill another hash container.
for (int i = keys.length - 1; i >= 0; i--)
{
if ((keys[i] != ((short)0))) {
procedure.apply(keys[i]);
}
}
return procedure;
}
/**
* {@inheritDoc}
*/
@Override
public short[] toArray(final short[] target)
{
int count = 0;
if (this.allocatedDefaultKey) {
target[count++] = ((short)0);
}
final short[] keys = this.keys;
for (int i = 0; i < keys.length; i++)
{
if ((keys[i] != ((short)0)))
{
target[count++] = keys[i];
}
}
assert count == this.size();
return target;
}
/**
* Clone this object.
* */
@Override
public ShortOpenHashSet clone()
{
final ShortOpenHashSet cloned = new ShortOpenHashSet(this.size(), this.loadFactor);
cloned.addAll(this);
cloned.allocatedDefaultKey = this.allocatedDefaultKey;
cloned.defaultValue = this.defaultValue;
return cloned;
}
/**
* {@inheritDoc}
*/
@Override
public T forEach(final T predicate)
{
if (this.allocatedDefaultKey) {
if (!predicate.apply(((short)0))) {
return predicate;
}
}
final short[] keys = this.keys;
//Iterate in reverse for side-stepping the longest conflict chain
//in another hash, in case apply() is actually used to fill another hash container.
for (int i = keys.length - 1; i >= 0; i--)
{
if ((keys[i] != ((short)0)))
{
if (!predicate.apply(keys[i])) {
break;
}
}
}
return predicate;
}
/**
* {@inheritDoc}
* Important!
* If the predicate actually injects the removed keys in another hash container, you may experience performance losses.
*/
@Override
public int removeAll(final ShortPredicate predicate)
{
final int before = this.size();
if (this.allocatedDefaultKey) {
if (predicate.apply(((short)0)))
{
this.allocatedDefaultKey = false;
}
}
final short[] keys = this.keys;
for (int i = 0; i < keys.length;)
{
if ((keys[i] != ((short)0)))
{
if (predicate.apply(keys[i]))
{
this.assigned--;
shiftConflictingKeys(i);
// Repeat the check for the same i.
continue;
}
}
i++;
}
return before - this.size();
}
/**
* Create a set from a variable number of arguments or an array of short
.
*/
public static ShortOpenHashSet from(final short... elements)
{
final ShortOpenHashSet set = new ShortOpenHashSet(elements.length);
set.add(elements);
return set;
}
/**
* Create a set from elements of another container.
*/
public static ShortOpenHashSet from(final ShortContainer container)
{
return new ShortOpenHashSet(container);
}
/**
* Create a new hash set with default parameters (shortcut
* instead of using a constructor).
*/
public static ShortOpenHashSet newInstance()
{
return new ShortOpenHashSet();
}
/**
* Returns a new object of this class with no need to declare generic type (shortcut
* instead of using a constructor).
*/
public static ShortOpenHashSet newInstanceWithCapacity(final int initialCapacity, final float loadFactor)
{
return new ShortOpenHashSet(initialCapacity, loadFactor);
}
//Test for existence in template
}