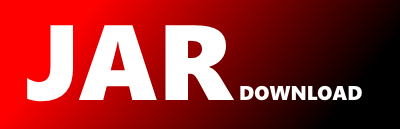
com.carrotsearch.hppcrt.CharDeque Maven / Gradle / Ivy
package com.carrotsearch.hppcrt;
import java.util.Iterator;
import java.util.List;
import com.carrotsearch.hppcrt.cursors.*;
import com.carrotsearch.hppcrt.predicates.*;
import com.carrotsearch.hppcrt.procedures.*;
/**
* A double-sided queue of char
s.
*/
@javax.annotation.Generated(
date = "2016-01-30T23:09:29+0100",
value = "KTypeDeque.java")
public interface CharDeque extends CharCollection
{
/**
* Removes the first element that equals e1
, returning its
* deleted position or -1
if the element was not found.
*/
public int removeFirst(char e1);
/**
* Removes the last element that equals e1
, returning its
* deleted position or -1
if the element was not found.
*/
public int removeLast(char e1);
/**
* Inserts the specified element at the front of this deque.
*
* @param e1 the element to add
*/
public void addFirst(char e1);
/**
* Inserts the specified element at the end of this deque.
*
* @param e1 the element to add
*/
public void addLast(char e1);
/**
* Retrieves and removes the first element of this deque.
* Precondition : the deque is not empty !
* @return the head element of this deque.
* @throws AssertionError if this deque is empty and assertions are enabled.
*/
public char removeFirst();
/**
* Retrieves and removes the last element of this deque.
* Precondition : the deque is not empty !
* @return the tail of this deque.
* @throws AssertionError if this deque is empty and assertions are enabled.
*/
public char removeLast();
/**
* Retrieves, but does not remove, the first element of this deque.
* Precondition : the deque is not empty !
* @return the head of this deque.
* @throws AssertionError if this deque is empty and assertions are enabled.
*/
public char getFirst();
/**
* Retrieves, but does not remove, the last element of this deque.
* Precondition : the deque is not empty !
* @return the tail of this deque.
* @throws AssertionError if this deque is empty and assertions are enabled.
*/
public char getLast();
/**
* @return An iterator over elements in this deque in tail-to-head order.
*/
public Iterator descendingIterator();
/**
* Applies a procedure
to all container elements.
*/
public T descendingForEach(T procedure);
/**
* Applies a predicate
to container elements as long, as the predicate
* returns true
. The iteration is interrupted otherwise.
*/
public T descendingForEach(T predicate);
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy