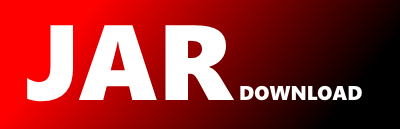
com.carrotsearch.hppcrt.FloatContainer Maven / Gradle / Ivy
package com.carrotsearch.hppcrt;
import java.util.Iterator;
import com.carrotsearch.hppcrt.cursors.FloatCursor;
import com.carrotsearch.hppcrt.predicates.FloatPredicate;
import com.carrotsearch.hppcrt.procedures.FloatProcedure;
/**
* A generic container holding float
s. An overview of interface relationships
* is given in the figure below:
*
* 
*/
@javax.annotation.Generated(
date = "2016-01-30T23:09:29+0100",
value = "KTypeContainer.java")
public interface FloatContainer extends Iterable
{
/**
* Returns an iterator to a cursor traversing the collection. The order of traversal
* is not defined. More than one cursor may be active at a time. The behavior of
* iterators is undefined if structural changes are made to the underlying collection.
*
* The iterator is implemented as a
* cursor and it returns the same cursor instance on every call to
* {@link Iterator#next()} (to avoid boxing of primitive types). To read the current
* list's value (or index in the list) use the cursor's public fields. An example is
* shown below.
*
*
* for (FloatCursor<float> c : container) {
* System.out.println("index=" + c.index + " value=" + c.value);
* }
*
*/
@Override
Iterator iterator();
/**
* Lookup a given element in the container. This operation has no speed
* guarantees (may be linear with respect to the size of this container).
*
* @return Returns true
if this container has an element
* equal to e
.
*/
boolean contains(float e);
/**
* Return the current number of elements in this container. The time for calculating
* the container's size may take O(n)
time, although implementing classes
* should try to maintain the current size and return in constant time.
*/
int size();
/**
* Return the maximum number of elements this container is guaranteed to hold without reallocating.
* The time for calculating the container's capacity may take O(n)
time.
*/
int capacity();
/**
* True if there is no elements in the container,
* equivalent to size() == 0
*/
boolean isEmpty();
/**
* Copies all elements from this container to an array.
* The returned array is sized to match exactly
* the number of elements of the container.
* If you need an array of the type identical with this container's generic type, use {@link #toArray(Class)}.
*
* @see #toArray(Class)
*/
public float [] toArray();
/**
* Copies all elements of this container to an existing array of the same type.
* @param target The target array must be large enough to hold all elements, i.e >= {@link #size()}.
* @return Returns the target argument for chaining.
*/
float[] toArray(float[] target);
/**
* Applies a procedure
to all container elements. Returns the argument (any
* subclass of {@link FloatProcedure}. This lets the caller to call methods of the argument
* by chaining the call (even if the argument is an anonymous type) to retrieve computed values,
* for example (IntContainer):
*
* int count = container.forEach(new IntProcedure() {
* int count; // this is a field declaration in an anonymous class.
* public void apply(int value) { count++; }}).count;
*
*/
T forEach(T procedure);
/**
* Applies a predicate
to container elements, as long as the predicate
* returns true
. The iteration is interrupted otherwise.
*/
T forEach(T predicate);
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy