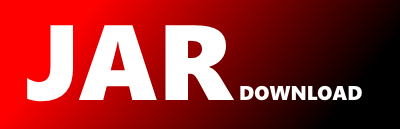
br.com.vtex.VtexSDK Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of vtex-android-sdk Show documentation
Show all versions of vtex-android-sdk Show documentation
Uma biblioteca para ajudar as agências a criar suas lojas Android.
package br.com.vtex;
import br.com.vtex.Models.OrderForm;
import com.google.gson.Gson;
import com.mashape.unirest.http.HttpResponse;
import com.mashape.unirest.http.Unirest;
import com.mashape.unirest.http.async.Callback;
import com.mashape.unirest.http.exceptions.UnirestException;
import com.mashape.unirest.request.HttpRequestWithBody;
import java.util.HashMap;
import java.util.Map;
import java.util.concurrent.Future;
/**
* Created by felipe-moraais on 02/02/15.
*/
public class VtexSDK {
private static VtexSDK instance = null;
String cookies = "";
String accountName = "";
private VtexSDK(String accountName) {
this.accountName = accountName;
}
//Singleton
public static synchronized VtexSDK getInstance(String accountName) {
if (instance == null) {
instance = new VtexSDK(accountName);
}
return instance;
}
/* TODO Array Section ExpectedFormSections as parameters */
// TODO getOrderForm() with orderFormId and accountName
// TODO addItemsToOrderForm() with orderId and accountName + array
public OrderForm getOrderForm() throws UnirestException {
Map headersMap = new HashMap();
if (!this.cookies.isEmpty()) {
headersMap.put("Cookie", this.cookies);
}
return getOrderFormBase(headersMap);
}
// TODO controle de exceção
public OrderForm getOrderForm(String email) throws UnirestException {
Map headersMap = new HashMap();
headersMap.put("data", String.format("{email: '%s'}", email));
return getOrderFormBase(headersMap);
}
private OrderForm getOrderFormBase(Map headersMap) throws UnirestException {
headersMap.put("contentType", "application/json");
headersMap.put("dataType", "json");
String url = String.format("http://%s.vtexcommercestable.com.br/api/checkout/pub/orderForm", this.accountName);
HttpResponse response;
response = Unirest.post(url)
.headers(headersMap)
.asString();
if (this.cookies.isEmpty()) {
for (String cookie : response.getHeaders().get("set-cookie")) {
if (cookie != null) {
this.cookies += cookie.split(";")[0] + ';';
}
}
}
Gson gson = new Gson();
OrderForm orderForm = gson.fromJson(response.getBody(), OrderForm.class);
return orderForm;
}
// O retorno deste método é uma future
public Future> addToCart(Integer sku, Integer quantity, Integer seller, Integer salesChannel, final Callback callback) {
final VtexSDK sdk = this;
Future> future;
Map headersMap = new HashMap();
String url = String.format("http://%s.vtexcommercestable.com.br/checkout/cart/add?sku=%s&qty=%s&seller=%s&sc=%s&redirect=false", this.accountName, sku, quantity, seller, salesChannel);
HttpRequestWithBody request = Unirest.post(url);
if (!this.cookies.isEmpty()) {
headersMap.put("Cookie", cookies);
request.headers(headersMap);
}
// Dica para encapsular isso aqui em baixo
// return request.asStringAsync(new SDKCallback(sdk, callback));
return request.asStringAsync(new Callback() {
@Override
public void completed(HttpResponse response) {
for (String cookie : response.getHeaders().get("set-cookie")) {
if (cookie != null) {
sdk.cookies += cookie.split(";")[0] + ';';
}
}
callback.completed(response);
}
@Override
public void failed(UnirestException e) {
e.printStackTrace();
callback.failed(e);
}
@Override
public void cancelled() {
System.out.println("O request foi cancelado.");
callback.cancelled();
}
});
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy