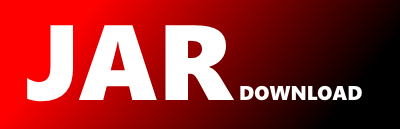
com.github.webdriverextensions.InstallDriversMojo Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of webdriverextensions-maven-plugin Show documentation
Show all versions of webdriverextensions-maven-plugin Show documentation
Use this plugin to manage, download and install WebDriver drivers directly from your pom.
package com.github.webdriverextensions;
import org.apache.commons.io.FileUtils;
import org.apache.maven.plugin.AbstractMojo;
import org.apache.maven.plugin.MojoExecutionException;
import org.apache.maven.plugins.annotations.Component;
import org.apache.maven.plugins.annotations.LifecyclePhase;
import org.apache.maven.plugins.annotations.Mojo;
import org.apache.maven.plugins.annotations.Parameter;
import org.apache.maven.project.MavenProject;
import org.apache.maven.settings.Settings;
import java.io.File;
import java.io.IOException;
import java.net.URL;
import java.nio.file.Path;
import java.nio.file.Paths;
import java.util.ArrayList;
import java.util.List;
import static com.github.webdriverextensions.ProxyUtils.getProxyFromSettings;
import static com.github.webdriverextensions.Utils.quote;
@Mojo(name = "install-drivers", defaultPhase = LifecyclePhase.GENERATE_SOURCES)
public class InstallDriversMojo extends AbstractMojo {
@Component
MavenProject project;
@Parameter(defaultValue = "${settings}", readonly = true)
Settings settings;
/**
* URL to where the repository file is located. The repository file is a
* json file containing information of available drivers and their
* locations, checksums, etc.
*/
@Parameter(defaultValue = "https://raw.githubusercontent.com/webdriverextensions/webdriverextensions-maven-plugin-repository/master/repository-3.0.json")
URL repositoryUrl;
/**
* The path to the directory where the drivers are going to be installed.
*/
@Parameter(defaultValue = "${basedir}/drivers")
File installationDirectory;
/**
* The id of the proxy to use if it is configured in settings.xml. If not provided the first
* active proxy in settings.xml will be used.
*/
@Parameter
String proxyId;
/**
* List of drivers to install. Each driver has a name, platform, bit,
* version, URL and checksum that can be provided.
*
* If no drivers are provided the latest drivers will be installed for the
* running platform and the bit version will be chosen as if it has not
* been provided (see rule below).
*
* If no platform is provided for a driver the platform will automatically
* be set to the running platform.
*
* If no bit version is provided for a driver the bit will automatically
* be set to 32 if running the plugin on a windows or mac platform. However
* if running the plugin from a linux platform the bit will be determined
* from the OS bit version.
*
* If the driver is not available in the repository the plugin does not know
* from which URL to download the driver. In that case the URL should be
* provided for the driver together with a checksum (to retrieve the
* checksum run the plugin without providing a checksum once, the plugin
* will then calculate and print the checksum for you). The default
* repository with all available drivers can be found here.
*
* Some Examples
* Installing all latest drivers
*
* <drivers>
* <driver>
* <name>chromedriver</name>
* <platform>windows</platform>
* <bit>32</bit>
* </driver>
* <driver>
* <name>chromedriver</name>
* <platform>mac</platform>
* <bit>32</bit>
* </driver>
* <driver>
* <name>chromedriver</name>
* <platform>linux</platform>
* <bit>32</bit>
* </driver>
* <driver>
* <name>chromedriver</name>
* <platform>linux</platform>
* <bit>64</bit>
* </driver>
* <driver>
* <name>internetexplorerdriver</name>
* <platform>windows</platform>
* <bit>32</bit>
* </driver>
* <driver>
* <name>internetexplorerdriver</name>
* <platform>windows</platform>
* <bit>64</bit>
* </driver>
* </drivers>
*
*
*
* Installing a driver not available in the repository, e.g. PhantomJS
*
* <driver>
* <name>phanthomjs</name>
* <platform>mac</platform>
* <bit>32</bit>
* <version>1.9.7</version>
* <url>http://bitbucket.org/ariya/phantomjs/downloads/phantomjs-1.9.7-macosx.zip</url>
* <checksum>0f4a64db9327d19a387446d43bbf5186</checksum>
* </driver>
*
*/
@Parameter
List drivers = new ArrayList<>();
/**
* Skips installation of drivers.
*/
@Parameter(defaultValue = "false")
boolean skip;
/**
* Keep downloaded files as local cache
*/
@Parameter(defaultValue = "false")
boolean keepDownloadedWebdrivers;
Path pluginWorkingDirectory = Paths.get(System.getProperty("java.io.tmpdir")).resolve("webdriverextensions-maven-plugin");
Path downloadDirectory = pluginWorkingDirectory.resolve("downloads");
Path tempDirectory = pluginWorkingDirectory.resolve("temp");
Repository repository;
public void execute() throws MojoExecutionException {
if (skip) {
getLog().info("Skipping install-drivers goal execution");
} else {
repository = Repository.load(repositoryUrl, getProxyFromSettings(this));
getLog().info("Installation directory " + quote(installationDirectory.toPath()));
if (drivers.isEmpty()) {
getLog().info("Installing latest drivers for current platform");
drivers = repository.getLatestDrivers();
} else {
getLog().info("Installing drivers from configuration");
}
DriverDownloader driverDownloader = new DriverDownloader(this);
DriverExtractor driverExtractor = new DriverExtractor(this);
DriverInstaller driverInstaller = new DriverInstaller(this);
cleanupTempDirectory();
for (Driver _driver : drivers) {
Driver driver = repository.enrichDriver(_driver);
if (driver == null) {
continue;
}
getLog().info(driver.getId() + " version " + driver.getVersion());
if (driverInstaller.needInstallation(driver)) {
Path downloadPath = downloadDirectory.resolve(driver.getDriverDownloadDirectoryName());
Path downloadLocation = driverDownloader.downloadFile(driver, downloadPath);
Path extractLocation = driverExtractor.extractDriver(driver, downloadLocation);
driverInstaller.install(driver, extractLocation);
if (!keepDownloadedWebdrivers) {
cleanupDownloadsDirectory();
}
cleanupTempDirectory();
} else {
getLog().info(" Already installed");
}
}
}
}
private void cleanupDownloadsDirectory() throws MojoExecutionException {
try {
FileUtils.deleteDirectory(downloadDirectory.toFile());
} catch (IOException e) {
throw new InstallDriversMojoExecutionException("Failed to delete downloads directory:" + System.lineSeparator()
+ Utils.directoryToString(downloadDirectory), e);
}
}
private void cleanupTempDirectory() throws MojoExecutionException {
try {
FileUtils.deleteDirectory(tempDirectory.toFile());
} catch (IOException e) {
throw new InstallDriversMojoExecutionException("Failed to delete temp directory:" + System.lineSeparator()
+ Utils.directoryToString(tempDirectory), e);
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy