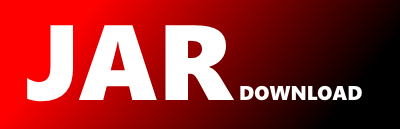
com.github.easydoc.EasydocLexer Maven / Gradle / Ivy
// $ANTLR 3.4 com/github/easydoc/Easydoc.g 2012-06-04 19:34:28
package com.github.easydoc;
import org.antlr.runtime.*;
import java.util.Stack;
import java.util.List;
import java.util.ArrayList;
@SuppressWarnings({"all", "warnings", "unchecked"})
public class EasydocLexer extends Lexer {
public static final int EOF=-1;
public static final int T__11=11;
public static final int T__12=12;
public static final int T__13=13;
public static final int CHAR=4;
public static final int COMMA=5;
public static final int DOUBLEAT=6;
public static final int ED_END=7;
public static final int ED_START=8;
public static final int EQ=9;
public static final int WS=10;
// delegates
// delegators
public Lexer[] getDelegates() {
return new Lexer[] {};
}
public EasydocLexer() {}
public EasydocLexer(CharStream input) {
this(input, new RecognizerSharedState());
}
public EasydocLexer(CharStream input, RecognizerSharedState state) {
super(input,state);
}
public String getGrammarFileName() { return "com/github/easydoc/Easydoc.g"; }
// $ANTLR start "T__11"
public final void mT__11() throws RecognitionException {
try {
int _type = T__11;
int _channel = DEFAULT_TOKEN_CHANNEL;
// com/github/easydoc/Easydoc.g:11:7: ( '\\\\,' )
// com/github/easydoc/Easydoc.g:11:9: '\\\\,'
{
match("\\,");
}
state.type = _type;
state.channel = _channel;
}
finally {
// do for sure before leaving
}
}
// $ANTLR end "T__11"
// $ANTLR start "T__12"
public final void mT__12() throws RecognitionException {
try {
int _type = T__12;
int _channel = DEFAULT_TOKEN_CHANNEL;
// com/github/easydoc/Easydoc.g:12:7: ( '\\\\@\\\\@' )
// com/github/easydoc/Easydoc.g:12:9: '\\\\@\\\\@'
{
match("\\@\\@");
}
state.type = _type;
state.channel = _channel;
}
finally {
// do for sure before leaving
}
}
// $ANTLR end "T__12"
// $ANTLR start "T__13"
public final void mT__13() throws RecognitionException {
try {
int _type = T__13;
int _channel = DEFAULT_TOKEN_CHANNEL;
// com/github/easydoc/Easydoc.g:13:7: ( '\\\\\\\\' )
// com/github/easydoc/Easydoc.g:13:9: '\\\\\\\\'
{
match("\\\\");
}
state.type = _type;
state.channel = _channel;
}
finally {
// do for sure before leaving
}
}
// $ANTLR end "T__13"
// $ANTLR start "WS"
public final void mWS() throws RecognitionException {
try {
int _type = WS;
int _channel = DEFAULT_TOKEN_CHANNEL;
// com/github/easydoc/Easydoc.g:58:3: ( ( ' ' | '\\t' | '\\n' | '\\r' | '\\f' )+ )
// com/github/easydoc/Easydoc.g:58:5: ( ' ' | '\\t' | '\\n' | '\\r' | '\\f' )+
{
// com/github/easydoc/Easydoc.g:58:5: ( ' ' | '\\t' | '\\n' | '\\r' | '\\f' )+
int cnt1=0;
loop1:
do {
int alt1=2;
switch ( input.LA(1) ) {
case '\t':
case '\n':
case '\f':
case '\r':
case ' ':
{
alt1=1;
}
break;
}
switch (alt1) {
case 1 :
// com/github/easydoc/Easydoc.g:
{
if ( (input.LA(1) >= '\t' && input.LA(1) <= '\n')||(input.LA(1) >= '\f' && input.LA(1) <= '\r')||input.LA(1)==' ' ) {
input.consume();
}
else {
MismatchedSetException mse = new MismatchedSetException(null,input);
recover(mse);
throw mse;
}
}
break;
default :
if ( cnt1 >= 1 ) break loop1;
EarlyExitException eee =
new EarlyExitException(1, input);
throw eee;
}
cnt1++;
} while (true);
}
state.type = _type;
state.channel = _channel;
}
finally {
// do for sure before leaving
}
}
// $ANTLR end "WS"
// $ANTLR start "EQ"
public final void mEQ() throws RecognitionException {
try {
int _type = EQ;
int _channel = DEFAULT_TOKEN_CHANNEL;
// com/github/easydoc/Easydoc.g:60:3: ( '=' )
// com/github/easydoc/Easydoc.g:60:5: '='
{
match('=');
}
state.type = _type;
state.channel = _channel;
}
finally {
// do for sure before leaving
}
}
// $ANTLR end "EQ"
// $ANTLR start "COMMA"
public final void mCOMMA() throws RecognitionException {
try {
int _type = COMMA;
int _channel = DEFAULT_TOKEN_CHANNEL;
// com/github/easydoc/Easydoc.g:62:6: ( ',' )
// com/github/easydoc/Easydoc.g:62:8: ','
{
match(',');
}
state.type = _type;
state.channel = _channel;
}
finally {
// do for sure before leaving
}
}
// $ANTLR end "COMMA"
// $ANTLR start "DOUBLEAT"
public final void mDOUBLEAT() throws RecognitionException {
try {
int _type = DOUBLEAT;
int _channel = DEFAULT_TOKEN_CHANNEL;
// com/github/easydoc/Easydoc.g:64:9: ( '@@' )
// com/github/easydoc/Easydoc.g:64:11: '@@'
{
match("@@");
}
state.type = _type;
state.channel = _channel;
}
finally {
// do for sure before leaving
}
}
// $ANTLR end "DOUBLEAT"
// $ANTLR start "ED_START"
public final void mED_START() throws RecognitionException {
try {
int _type = ED_START;
int _channel = DEFAULT_TOKEN_CHANNEL;
// com/github/easydoc/Easydoc.g:66:9: ( '@@easydoc-start' )
// com/github/easydoc/Easydoc.g:66:11: '@@easydoc-start'
{
match("@@easydoc-start");
}
state.type = _type;
state.channel = _channel;
}
finally {
// do for sure before leaving
}
}
// $ANTLR end "ED_START"
// $ANTLR start "ED_END"
public final void mED_END() throws RecognitionException {
try {
int _type = ED_END;
int _channel = DEFAULT_TOKEN_CHANNEL;
// com/github/easydoc/Easydoc.g:68:7: ( '@@easydoc-end@@' )
// com/github/easydoc/Easydoc.g:68:9: '@@easydoc-end@@'
{
match("@@easydoc-end@@");
}
state.type = _type;
state.channel = _channel;
}
finally {
// do for sure before leaving
}
}
// $ANTLR end "ED_END"
// $ANTLR start "CHAR"
public final void mCHAR() throws RecognitionException {
try {
int _type = CHAR;
int _channel = DEFAULT_TOKEN_CHANNEL;
// com/github/easydoc/Easydoc.g:70:5: ( '\\u0000' .. '\\uFFFE' )
// com/github/easydoc/Easydoc.g:
{
if ( (input.LA(1) >= '\u0000' && input.LA(1) <= '\uFFFE') ) {
input.consume();
}
else {
MismatchedSetException mse = new MismatchedSetException(null,input);
recover(mse);
throw mse;
}
}
state.type = _type;
state.channel = _channel;
}
finally {
// do for sure before leaving
}
}
// $ANTLR end "CHAR"
public void mTokens() throws RecognitionException {
// com/github/easydoc/Easydoc.g:1:8: ( T__11 | T__12 | T__13 | WS | EQ | COMMA | DOUBLEAT | ED_START | ED_END | CHAR )
int alt2=10;
int LA2_0 = input.LA(1);
if ( (LA2_0=='\\') ) {
switch ( input.LA(2) ) {
case ',':
{
alt2=1;
}
break;
case '@':
{
alt2=2;
}
break;
case '\\':
{
alt2=3;
}
break;
default:
alt2=10;
}
}
else if ( ((LA2_0 >= '\t' && LA2_0 <= '\n')||(LA2_0 >= '\f' && LA2_0 <= '\r')||LA2_0==' ') ) {
alt2=4;
}
else if ( (LA2_0=='=') ) {
alt2=5;
}
else if ( (LA2_0==',') ) {
alt2=6;
}
else if ( (LA2_0=='@') ) {
switch ( input.LA(2) ) {
case '@':
{
switch ( input.LA(3) ) {
case 'e':
{
switch ( input.LA(4) ) {
case 'a':
{
switch ( input.LA(5) ) {
case 's':
{
switch ( input.LA(6) ) {
case 'y':
{
switch ( input.LA(7) ) {
case 'd':
{
switch ( input.LA(8) ) {
case 'o':
{
switch ( input.LA(9) ) {
case 'c':
{
switch ( input.LA(10) ) {
case '-':
{
switch ( input.LA(11) ) {
case 's':
{
alt2=8;
}
break;
case 'e':
{
alt2=9;
}
break;
default:
NoViableAltException nvae =
new NoViableAltException("", 2, 22, input);
throw nvae;
}
}
break;
default:
NoViableAltException nvae =
new NoViableAltException("", 2, 21, input);
throw nvae;
}
}
break;
default:
NoViableAltException nvae =
new NoViableAltException("", 2, 20, input);
throw nvae;
}
}
break;
default:
NoViableAltException nvae =
new NoViableAltException("", 2, 19, input);
throw nvae;
}
}
break;
default:
NoViableAltException nvae =
new NoViableAltException("", 2, 18, input);
throw nvae;
}
}
break;
default:
NoViableAltException nvae =
new NoViableAltException("", 2, 17, input);
throw nvae;
}
}
break;
default:
NoViableAltException nvae =
new NoViableAltException("", 2, 16, input);
throw nvae;
}
}
break;
default:
NoViableAltException nvae =
new NoViableAltException("", 2, 14, input);
throw nvae;
}
}
break;
default:
alt2=7;
}
}
break;
default:
alt2=10;
}
}
else if ( ((LA2_0 >= '\u0000' && LA2_0 <= '\b')||LA2_0=='\u000B'||(LA2_0 >= '\u000E' && LA2_0 <= '\u001F')||(LA2_0 >= '!' && LA2_0 <= '+')||(LA2_0 >= '-' && LA2_0 <= '<')||(LA2_0 >= '>' && LA2_0 <= '?')||(LA2_0 >= 'A' && LA2_0 <= '[')||(LA2_0 >= ']' && LA2_0 <= '\uFFFE')) ) {
alt2=10;
}
else {
NoViableAltException nvae =
new NoViableAltException("", 2, 0, input);
throw nvae;
}
switch (alt2) {
case 1 :
// com/github/easydoc/Easydoc.g:1:10: T__11
{
mT__11();
}
break;
case 2 :
// com/github/easydoc/Easydoc.g:1:16: T__12
{
mT__12();
}
break;
case 3 :
// com/github/easydoc/Easydoc.g:1:22: T__13
{
mT__13();
}
break;
case 4 :
// com/github/easydoc/Easydoc.g:1:28: WS
{
mWS();
}
break;
case 5 :
// com/github/easydoc/Easydoc.g:1:31: EQ
{
mEQ();
}
break;
case 6 :
// com/github/easydoc/Easydoc.g:1:34: COMMA
{
mCOMMA();
}
break;
case 7 :
// com/github/easydoc/Easydoc.g:1:40: DOUBLEAT
{
mDOUBLEAT();
}
break;
case 8 :
// com/github/easydoc/Easydoc.g:1:49: ED_START
{
mED_START();
}
break;
case 9 :
// com/github/easydoc/Easydoc.g:1:58: ED_END
{
mED_END();
}
break;
case 10 :
// com/github/easydoc/Easydoc.g:1:65: CHAR
{
mCHAR();
}
break;
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy