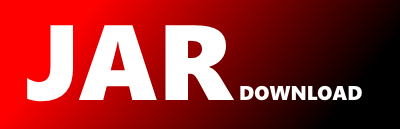
com.github.easydoc.EasydocParser Maven / Gradle / Ivy
// $ANTLR 3.4 com/github/easydoc/Easydoc.g 2012-06-04 19:34:28
package com.github.easydoc;
import java.util.Map;
import java.util.HashMap;
import java.util.List;
import java.util.ArrayList;
import java.io.File;
import com.github.easydoc.model.Doc;
import com.github.easydoc.model.SourceLink;
import com.github.easydoc.model.Directive;
import com.github.easydoc.model.DocItem;
import com.github.easydoc.model.DocTextItem;
import org.antlr.runtime.*;
import java.util.Stack;
import java.util.List;
import java.util.ArrayList;
@SuppressWarnings({"all", "warnings", "unchecked"})
public class EasydocParser extends Parser {
public static final String[] tokenNames = new String[] {
"", "", "", "", "CHAR", "COMMA", "DOUBLEAT", "ED_END", "ED_START", "EQ", "WS", "'\\\\,'", "'\\\\@\\\\@'", "'\\\\\\\\'"
};
public static final int EOF=-1;
public static final int T__11=11;
public static final int T__12=12;
public static final int T__13=13;
public static final int CHAR=4;
public static final int COMMA=5;
public static final int DOUBLEAT=6;
public static final int ED_END=7;
public static final int ED_START=8;
public static final int EQ=9;
public static final int WS=10;
// delegates
public Parser[] getDelegates() {
return new Parser[] {};
}
// delegators
public EasydocParser(TokenStream input) {
this(input, new RecognizerSharedState());
}
public EasydocParser(TokenStream input, RecognizerSharedState state) {
super(input, state);
}
public String[] getTokenNames() { return EasydocParser.tokenNames; }
public String getGrammarFileName() { return "com/github/easydoc/Easydoc.g"; }
private File file;
public EasydocParser(File file) throws java.io.IOException {
super(
new CommonTokenStream(
new EasydocLexer(
new ANTLRFileStream(
file.getAbsolutePath()
)
)
)
);
this.file = file;
}
public EasydocParser(File file, String encoding) throws java.io.IOException {
super(
new CommonTokenStream(
new EasydocLexer(
new ANTLRFileStream(
file.getAbsolutePath(),
encoding
)
)
)
);
this.file = file;
}
public static class easydocStart_return extends ParserRuleReturnScope {
public Map params;
public int line;
public int startColumn;
public int endColumn;
};
// $ANTLR start "easydocStart"
// com/github/easydoc/Easydoc.g:72:1: easydocStart returns [Map params, int line, int startColumn, int endColumn] : ED_START easydocParams DOUBLEAT ;
public final EasydocParser.easydocStart_return easydocStart() throws RecognitionException {
EasydocParser.easydocStart_return retval = new EasydocParser.easydocStart_return();
retval.start = input.LT(1);
Token ED_START1=null;
Token DOUBLEAT3=null;
Map easydocParams2 =null;
try {
// com/github/easydoc/Easydoc.g:72:93: ( ED_START easydocParams DOUBLEAT )
// com/github/easydoc/Easydoc.g:73:2: ED_START easydocParams DOUBLEAT
{
retval.params = new HashMap();
ED_START1=(Token)match(input,ED_START,FOLLOW_ED_START_in_easydocStart125);
retval.line = ED_START1.getLine();
retval.startColumn = ED_START1.getCharPositionInLine();
pushFollow(FOLLOW_easydocParams_in_easydocStart130);
easydocParams2=easydocParams();
state._fsp--;
retval.params = easydocParams2;
DOUBLEAT3=(Token)match(input,DOUBLEAT,FOLLOW_DOUBLEAT_in_easydocStart136);
retval.endColumn = DOUBLEAT3.getCharPositionInLine() + DOUBLEAT3.getText().length();
}
retval.stop = input.LT(-1);
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
// do for sure before leaving
}
return retval;
}
// $ANTLR end "easydocStart"
// $ANTLR start "easydocParams"
// com/github/easydoc/Easydoc.g:82:1: easydocParams returns [Map params] : ( ( WS )* COMMA ( WS )* easydocParam )* ;
public final Map easydocParams() throws RecognitionException {
Map params = null;
EasydocParser.easydocParam_return easydocParam4 =null;
try {
// com/github/easydoc/Easydoc.g:82:52: ( ( ( WS )* COMMA ( WS )* easydocParam )* )
// com/github/easydoc/Easydoc.g:83:2: ( ( WS )* COMMA ( WS )* easydocParam )*
{
params = new HashMap();
// com/github/easydoc/Easydoc.g:84:2: ( ( WS )* COMMA ( WS )* easydocParam )*
loop3:
do {
int alt3=2;
switch ( input.LA(1) ) {
case COMMA:
case WS:
{
alt3=1;
}
break;
}
switch (alt3) {
case 1 :
// com/github/easydoc/Easydoc.g:84:3: ( WS )* COMMA ( WS )* easydocParam
{
// com/github/easydoc/Easydoc.g:84:3: ( WS )*
loop1:
do {
int alt1=2;
switch ( input.LA(1) ) {
case WS:
{
alt1=1;
}
break;
}
switch (alt1) {
case 1 :
// com/github/easydoc/Easydoc.g:84:3: WS
{
match(input,WS,FOLLOW_WS_in_easydocParams159);
}
break;
default :
break loop1;
}
} while (true);
match(input,COMMA,FOLLOW_COMMA_in_easydocParams162);
// com/github/easydoc/Easydoc.g:84:13: ( WS )*
loop2:
do {
int alt2=2;
switch ( input.LA(1) ) {
case WS:
{
alt2=1;
}
break;
}
switch (alt2) {
case 1 :
// com/github/easydoc/Easydoc.g:84:13: WS
{
match(input,WS,FOLLOW_WS_in_easydocParams164);
}
break;
default :
break loop2;
}
} while (true);
pushFollow(FOLLOW_easydocParam_in_easydocParams167);
easydocParam4=easydocParam();
state._fsp--;
params.put((easydocParam4!=null?easydocParam4.name:null), (easydocParam4!=null?easydocParam4.value:null));
}
break;
default :
break loop3;
}
} while (true);
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
// do for sure before leaving
}
return params;
}
// $ANTLR end "easydocParams"
public static class easydocParam_return extends ParserRuleReturnScope {
public String name;
public String value;
};
// $ANTLR start "easydocParam"
// com/github/easydoc/Easydoc.g:87:1: easydocParam returns [String name, String value] : paramName ( ( WS )* EQ ( WS )* paramValue ) ;
public final EasydocParser.easydocParam_return easydocParam() throws RecognitionException {
EasydocParser.easydocParam_return retval = new EasydocParser.easydocParam_return();
retval.start = input.LT(1);
String paramName5 =null;
String paramValue6 =null;
try {
// com/github/easydoc/Easydoc.g:87:50: ( paramName ( ( WS )* EQ ( WS )* paramValue ) )
// com/github/easydoc/Easydoc.g:88:2: paramName ( ( WS )* EQ ( WS )* paramValue )
{
pushFollow(FOLLOW_paramName_in_easydocParam189);
paramName5=paramName();
state._fsp--;
retval.name = paramName5;
// com/github/easydoc/Easydoc.g:89:2: ( ( WS )* EQ ( WS )* paramValue )
// com/github/easydoc/Easydoc.g:89:3: ( WS )* EQ ( WS )* paramValue
{
// com/github/easydoc/Easydoc.g:89:3: ( WS )*
loop4:
do {
int alt4=2;
switch ( input.LA(1) ) {
case WS:
{
alt4=1;
}
break;
}
switch (alt4) {
case 1 :
// com/github/easydoc/Easydoc.g:89:3: WS
{
match(input,WS,FOLLOW_WS_in_easydocParam196);
}
break;
default :
break loop4;
}
} while (true);
match(input,EQ,FOLLOW_EQ_in_easydocParam199);
// com/github/easydoc/Easydoc.g:89:10: ( WS )*
loop5:
do {
int alt5=2;
switch ( input.LA(1) ) {
case WS:
{
alt5=1;
}
break;
}
switch (alt5) {
case 1 :
// com/github/easydoc/Easydoc.g:89:10: WS
{
match(input,WS,FOLLOW_WS_in_easydocParam201);
}
break;
default :
break loop5;
}
} while (true);
pushFollow(FOLLOW_paramValue_in_easydocParam204);
paramValue6=paramValue();
state._fsp--;
retval.value = paramValue6;
}
}
retval.stop = input.LT(-1);
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
// do for sure before leaving
}
return retval;
}
// $ANTLR end "easydocParam"
// $ANTLR start "paramName"
// com/github/easydoc/Easydoc.g:91:1: paramName returns [String text] : ( CHAR )+ ;
public final String paramName() throws RecognitionException {
String text = null;
Token CHAR7=null;
try {
// com/github/easydoc/Easydoc.g:91:33: ( ( CHAR )+ )
// com/github/easydoc/Easydoc.g:92:2: ( CHAR )+
{
StringBuilder sb = new StringBuilder();
// com/github/easydoc/Easydoc.g:93:2: ( CHAR )+
int cnt6=0;
loop6:
do {
int alt6=2;
switch ( input.LA(1) ) {
case CHAR:
{
alt6=1;
}
break;
}
switch (alt6) {
case 1 :
// com/github/easydoc/Easydoc.g:93:3: CHAR
{
CHAR7=(Token)match(input,CHAR,FOLLOW_CHAR_in_paramName226);
sb.append((CHAR7!=null?CHAR7.getText():null));
}
break;
default :
if ( cnt6 >= 1 ) break loop6;
EarlyExitException eee =
new EarlyExitException(6, input);
throw eee;
}
cnt6++;
} while (true);
text = sb.toString();
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
// do for sure before leaving
}
return text;
}
// $ANTLR end "paramName"
// $ANTLR start "paramValue"
// com/github/easydoc/Easydoc.g:96:1: paramValue returns [String text] : ( CHAR | '\\\\,' | '\\\\\\\\' )+ ;
public final String paramValue() throws RecognitionException {
String text = null;
Token CHAR8=null;
try {
// com/github/easydoc/Easydoc.g:96:34: ( ( CHAR | '\\\\,' | '\\\\\\\\' )+ )
// com/github/easydoc/Easydoc.g:97:2: ( CHAR | '\\\\,' | '\\\\\\\\' )+
{
StringBuilder sb = new StringBuilder();
// com/github/easydoc/Easydoc.g:98:2: ( CHAR | '\\\\,' | '\\\\\\\\' )+
int cnt7=0;
loop7:
do {
int alt7=4;
switch ( input.LA(1) ) {
case CHAR:
{
alt7=1;
}
break;
case 11:
{
alt7=2;
}
break;
case 13:
{
alt7=3;
}
break;
}
switch (alt7) {
case 1 :
// com/github/easydoc/Easydoc.g:99:3: CHAR
{
CHAR8=(Token)match(input,CHAR,FOLLOW_CHAR_in_paramValue258);
sb.append((CHAR8!=null?CHAR8.getText():null));
}
break;
case 2 :
// com/github/easydoc/Easydoc.g:100:5: '\\\\,'
{
match(input,11,FOLLOW_11_in_paramValue266);
sb.append(",");
}
break;
case 3 :
// com/github/easydoc/Easydoc.g:101:5: '\\\\\\\\'
{
match(input,13,FOLLOW_13_in_paramValue274);
sb.append("\\");
}
break;
default :
if ( cnt7 >= 1 ) break loop7;
EarlyExitException eee =
new EarlyExitException(7, input);
throw eee;
}
cnt7++;
} while (true);
text = sb.toString();
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
// do for sure before leaving
}
return text;
}
// $ANTLR end "paramValue"
// $ANTLR start "easydocEnd"
// com/github/easydoc/Easydoc.g:105:1: easydocEnd returns [int line] : ED_END ;
public final int easydocEnd() throws RecognitionException {
int line = 0;
Token ED_END9=null;
try {
// com/github/easydoc/Easydoc.g:105:31: ( ED_END )
// com/github/easydoc/Easydoc.g:106:2: ED_END
{
ED_END9=(Token)match(input,ED_END,FOLLOW_ED_END_in_easydocEnd300);
line = ED_END9.getLine();
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
// do for sure before leaving
}
return line;
}
// $ANTLR end "easydocEnd"
// $ANTLR start "easydocDoc"
// com/github/easydoc/Easydoc.g:108:1: easydocDoc returns [Doc result] : easydocStart ( easydocText )? easydocEnd ;
public final Doc easydocDoc() throws RecognitionException {
Doc result = null;
EasydocParser.easydocStart_return easydocStart10 =null;
EasydocParser.easydocText_return easydocText11 =null;
int easydocEnd12 =0;
try {
// com/github/easydoc/Easydoc.g:109:2: ( easydocStart ( easydocText )? easydocEnd )
// com/github/easydoc/Easydoc.g:109:4: easydocStart ( easydocText )? easydocEnd
{
result = new Doc();
pushFollow(FOLLOW_easydocStart_in_easydocDoc319);
easydocStart10=easydocStart();
state._fsp--;
result.setParams((easydocStart10!=null?easydocStart10.params:null));
// com/github/easydoc/Easydoc.g:113:2: ( easydocText )?
int alt8=2;
switch ( input.LA(1) ) {
case CHAR:
case COMMA:
case DOUBLEAT:
case EQ:
case WS:
case 12:
case 13:
{
alt8=1;
}
break;
}
switch (alt8) {
case 1 :
// com/github/easydoc/Easydoc.g:114:3: easydocText
{
pushFollow(FOLLOW_easydocText_in_easydocDoc329);
easydocText11=easydocText();
state._fsp--;
result.setItems((easydocText11!=null?easydocText11.items:null));
result.setDirectives((easydocText11!=null?easydocText11.directives:null));
}
break;
}
pushFollow(FOLLOW_easydocEnd_in_easydocDoc339);
easydocEnd12=easydocEnd();
state._fsp--;
result.setSourceLink(new SourceLink(file, (easydocStart10!=null?easydocStart10.line:0), easydocEnd12));
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
// do for sure before leaving
}
return result;
}
// $ANTLR end "easydocDoc"
// $ANTLR start "easydocDirective"
// com/github/easydoc/Easydoc.g:124:1: easydocDirective returns [Directive result] : DOUBLEAT paramName easydocParams DOUBLEAT ;
public final Directive easydocDirective() throws RecognitionException {
Directive result = null;
String paramName13 =null;
Map easydocParams14 =null;
try {
// com/github/easydoc/Easydoc.g:124:45: ( DOUBLEAT paramName easydocParams DOUBLEAT )
// com/github/easydoc/Easydoc.g:125:2: DOUBLEAT paramName easydocParams DOUBLEAT
{
result = new Directive();
match(input,DOUBLEAT,FOLLOW_DOUBLEAT_in_easydocDirective361);
pushFollow(FOLLOW_paramName_in_easydocDirective364);
paramName13=paramName();
state._fsp--;
result.setName(paramName13);
pushFollow(FOLLOW_easydocParams_in_easydocDirective369);
easydocParams14=easydocParams();
state._fsp--;
result.setParams(easydocParams14);
match(input,DOUBLEAT,FOLLOW_DOUBLEAT_in_easydocDirective374);
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
// do for sure before leaving
}
return result;
}
// $ANTLR end "easydocDirective"
// $ANTLR start "simpleText"
// com/github/easydoc/Easydoc.g:131:1: simpleText returns [String result] : ( CHAR | WS | EQ | COMMA | DOUBLEAT )+ ;
public final String simpleText() throws RecognitionException {
String result = null;
Token CHAR15=null;
Token WS16=null;
Token EQ17=null;
Token COMMA18=null;
try {
// com/github/easydoc/Easydoc.g:131:36: ( ( CHAR | WS | EQ | COMMA | DOUBLEAT )+ )
// com/github/easydoc/Easydoc.g:132:2: ( CHAR | WS | EQ | COMMA | DOUBLEAT )+
{
StringBuffer sb = new StringBuffer();
// com/github/easydoc/Easydoc.g:133:2: ( CHAR | WS | EQ | COMMA | DOUBLEAT )+
int cnt9=0;
loop9:
do {
int alt9=6;
switch ( input.LA(1) ) {
case CHAR:
{
alt9=1;
}
break;
case WS:
{
alt9=2;
}
break;
case EQ:
{
alt9=3;
}
break;
case COMMA:
{
alt9=4;
}
break;
case DOUBLEAT:
{
alt9=5;
}
break;
}
switch (alt9) {
case 1 :
// com/github/easydoc/Easydoc.g:134:3: CHAR
{
CHAR15=(Token)match(input,CHAR,FOLLOW_CHAR_in_simpleText397);
sb.append((CHAR15!=null?CHAR15.getText():null));
}
break;
case 2 :
// com/github/easydoc/Easydoc.g:135:5: WS
{
WS16=(Token)match(input,WS,FOLLOW_WS_in_simpleText406);
sb.append((WS16!=null?WS16.getText():null));
}
break;
case 3 :
// com/github/easydoc/Easydoc.g:136:5: EQ
{
EQ17=(Token)match(input,EQ,FOLLOW_EQ_in_simpleText415);
sb.append((EQ17!=null?EQ17.getText():null));
}
break;
case 4 :
// com/github/easydoc/Easydoc.g:137:5: COMMA
{
COMMA18=(Token)match(input,COMMA,FOLLOW_COMMA_in_simpleText424);
sb.append((COMMA18!=null?COMMA18.getText():null));
}
break;
case 5 :
// com/github/easydoc/Easydoc.g:138:5: DOUBLEAT
{
match(input,DOUBLEAT,FOLLOW_DOUBLEAT_in_simpleText432);
sb.append("@@");
}
break;
default :
if ( cnt9 >= 1 ) break loop9;
EarlyExitException eee =
new EarlyExitException(9, input);
throw eee;
}
cnt9++;
} while (true);
result = sb.toString();
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
// do for sure before leaving
}
return result;
}
// $ANTLR end "simpleText"
public static class easydocText_return extends ParserRuleReturnScope {
public List items;
public List directives;
};
// $ANTLR start "easydocText"
// com/github/easydoc/Easydoc.g:141:1: easydocText returns [List items, List directives] : ( CHAR | WS | EQ | COMMA | '\\\\@\\\\@' | '\\\\\\\\' | easydocDirective )+ ;
public final EasydocParser.easydocText_return easydocText() throws RecognitionException {
EasydocParser.easydocText_return retval = new EasydocParser.easydocText_return();
retval.start = input.LT(1);
Token CHAR19=null;
Token WS20=null;
Token EQ21=null;
Token COMMA22=null;
Directive easydocDirective23 =null;
try {
// com/github/easydoc/Easydoc.g:141:71: ( ( CHAR | WS | EQ | COMMA | '\\\\@\\\\@' | '\\\\\\\\' | easydocDirective )+ )
// com/github/easydoc/Easydoc.g:142:2: ( CHAR | WS | EQ | COMMA | '\\\\@\\\\@' | '\\\\\\\\' | easydocDirective )+
{
StringBuffer sb = new StringBuffer();
retval.directives = new ArrayList();
retval.items = new ArrayList();
// com/github/easydoc/Easydoc.g:147:2: ( CHAR | WS | EQ | COMMA | '\\\\@\\\\@' | '\\\\\\\\' | easydocDirective )+
int cnt10=0;
loop10:
do {
int alt10=8;
switch ( input.LA(1) ) {
case CHAR:
{
alt10=1;
}
break;
case WS:
{
alt10=2;
}
break;
case EQ:
{
alt10=3;
}
break;
case COMMA:
{
alt10=4;
}
break;
case 12:
{
alt10=5;
}
break;
case 13:
{
alt10=6;
}
break;
case DOUBLEAT:
{
alt10=7;
}
break;
}
switch (alt10) {
case 1 :
// com/github/easydoc/Easydoc.g:148:3: CHAR
{
CHAR19=(Token)match(input,CHAR,FOLLOW_CHAR_in_easydocText463);
sb.append((CHAR19!=null?CHAR19.getText():null));
}
break;
case 2 :
// com/github/easydoc/Easydoc.g:149:5: WS
{
WS20=(Token)match(input,WS,FOLLOW_WS_in_easydocText472);
sb.append((WS20!=null?WS20.getText():null));
}
break;
case 3 :
// com/github/easydoc/Easydoc.g:150:5: EQ
{
EQ21=(Token)match(input,EQ,FOLLOW_EQ_in_easydocText481);
sb.append((EQ21!=null?EQ21.getText():null));
}
break;
case 4 :
// com/github/easydoc/Easydoc.g:151:5: COMMA
{
COMMA22=(Token)match(input,COMMA,FOLLOW_COMMA_in_easydocText490);
sb.append((COMMA22!=null?COMMA22.getText():null));
}
break;
case 5 :
// com/github/easydoc/Easydoc.g:152:5: '\\\\@\\\\@'
{
match(input,12,FOLLOW_12_in_easydocText498);
sb.append("@@");
}
break;
case 6 :
// com/github/easydoc/Easydoc.g:153:5: '\\\\\\\\'
{
match(input,13,FOLLOW_13_in_easydocText506);
sb.append("\\");
}
break;
case 7 :
// com/github/easydoc/Easydoc.g:154:5: easydocDirective
{
pushFollow(FOLLOW_easydocDirective_in_easydocText514);
easydocDirective23=easydocDirective();
state._fsp--;
retval.items.add(new DocTextItem(sb.toString()));
sb.setLength(0);
retval.items.add(easydocDirective23);
retval.directives.add(easydocDirective23);
}
break;
default :
if ( cnt10 >= 1 ) break loop10;
EarlyExitException eee =
new EarlyExitException(10, input);
throw eee;
}
cnt10++;
} while (true);
retval.items.add(new DocTextItem(sb.toString()));
}
retval.stop = input.LT(-1);
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
// do for sure before leaving
}
return retval;
}
// $ANTLR end "easydocText"
// $ANTLR start "document"
// com/github/easydoc/Easydoc.g:162:1: document returns [List docs] : ( simpleText | easydocDoc )* ;
public final List document() throws RecognitionException {
List docs = null;
Doc easydocDoc24 =null;
try {
// com/github/easydoc/Easydoc.g:163:2: ( ( simpleText | easydocDoc )* )
// com/github/easydoc/Easydoc.g:164:2: ( simpleText | easydocDoc )*
{
docs = new ArrayList();
// com/github/easydoc/Easydoc.g:165:2: ( simpleText | easydocDoc )*
loop11:
do {
int alt11=3;
switch ( input.LA(1) ) {
case CHAR:
case COMMA:
case DOUBLEAT:
case EQ:
case WS:
{
alt11=1;
}
break;
case ED_START:
{
alt11=2;
}
break;
}
switch (alt11) {
case 1 :
// com/github/easydoc/Easydoc.g:166:3: simpleText
{
pushFollow(FOLLOW_simpleText_in_document545);
simpleText();
state._fsp--;
}
break;
case 2 :
// com/github/easydoc/Easydoc.g:167:5: easydocDoc
{
pushFollow(FOLLOW_easydocDoc_in_document552);
easydocDoc24=easydocDoc();
state._fsp--;
docs.add(easydocDoc24);
}
break;
default :
break loop11;
}
} while (true);
}
}
catch (RecognitionException re) {
reportError(re);
recover(input,re);
}
finally {
// do for sure before leaving
}
return docs;
}
// $ANTLR end "document"
// Delegated rules
public static final BitSet FOLLOW_ED_START_in_easydocStart125 = new BitSet(new long[]{0x0000000000000460L});
public static final BitSet FOLLOW_easydocParams_in_easydocStart130 = new BitSet(new long[]{0x0000000000000040L});
public static final BitSet FOLLOW_DOUBLEAT_in_easydocStart136 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_WS_in_easydocParams159 = new BitSet(new long[]{0x0000000000000420L});
public static final BitSet FOLLOW_COMMA_in_easydocParams162 = new BitSet(new long[]{0x0000000000000410L});
public static final BitSet FOLLOW_WS_in_easydocParams164 = new BitSet(new long[]{0x0000000000000410L});
public static final BitSet FOLLOW_easydocParam_in_easydocParams167 = new BitSet(new long[]{0x0000000000000422L});
public static final BitSet FOLLOW_paramName_in_easydocParam189 = new BitSet(new long[]{0x0000000000000600L});
public static final BitSet FOLLOW_WS_in_easydocParam196 = new BitSet(new long[]{0x0000000000000600L});
public static final BitSet FOLLOW_EQ_in_easydocParam199 = new BitSet(new long[]{0x0000000000002C10L});
public static final BitSet FOLLOW_WS_in_easydocParam201 = new BitSet(new long[]{0x0000000000002C10L});
public static final BitSet FOLLOW_paramValue_in_easydocParam204 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_CHAR_in_paramName226 = new BitSet(new long[]{0x0000000000000012L});
public static final BitSet FOLLOW_CHAR_in_paramValue258 = new BitSet(new long[]{0x0000000000002812L});
public static final BitSet FOLLOW_11_in_paramValue266 = new BitSet(new long[]{0x0000000000002812L});
public static final BitSet FOLLOW_13_in_paramValue274 = new BitSet(new long[]{0x0000000000002812L});
public static final BitSet FOLLOW_ED_END_in_easydocEnd300 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_easydocStart_in_easydocDoc319 = new BitSet(new long[]{0x00000000000036F0L});
public static final BitSet FOLLOW_easydocText_in_easydocDoc329 = new BitSet(new long[]{0x0000000000000080L});
public static final BitSet FOLLOW_easydocEnd_in_easydocDoc339 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_DOUBLEAT_in_easydocDirective361 = new BitSet(new long[]{0x0000000000000010L});
public static final BitSet FOLLOW_paramName_in_easydocDirective364 = new BitSet(new long[]{0x0000000000000460L});
public static final BitSet FOLLOW_easydocParams_in_easydocDirective369 = new BitSet(new long[]{0x0000000000000040L});
public static final BitSet FOLLOW_DOUBLEAT_in_easydocDirective374 = new BitSet(new long[]{0x0000000000000002L});
public static final BitSet FOLLOW_CHAR_in_simpleText397 = new BitSet(new long[]{0x0000000000000672L});
public static final BitSet FOLLOW_WS_in_simpleText406 = new BitSet(new long[]{0x0000000000000672L});
public static final BitSet FOLLOW_EQ_in_simpleText415 = new BitSet(new long[]{0x0000000000000672L});
public static final BitSet FOLLOW_COMMA_in_simpleText424 = new BitSet(new long[]{0x0000000000000672L});
public static final BitSet FOLLOW_DOUBLEAT_in_simpleText432 = new BitSet(new long[]{0x0000000000000672L});
public static final BitSet FOLLOW_CHAR_in_easydocText463 = new BitSet(new long[]{0x0000000000003672L});
public static final BitSet FOLLOW_WS_in_easydocText472 = new BitSet(new long[]{0x0000000000003672L});
public static final BitSet FOLLOW_EQ_in_easydocText481 = new BitSet(new long[]{0x0000000000003672L});
public static final BitSet FOLLOW_COMMA_in_easydocText490 = new BitSet(new long[]{0x0000000000003672L});
public static final BitSet FOLLOW_12_in_easydocText498 = new BitSet(new long[]{0x0000000000003672L});
public static final BitSet FOLLOW_13_in_easydocText506 = new BitSet(new long[]{0x0000000000003672L});
public static final BitSet FOLLOW_easydocDirective_in_easydocText514 = new BitSet(new long[]{0x0000000000003672L});
public static final BitSet FOLLOW_simpleText_in_document545 = new BitSet(new long[]{0x0000000000000772L});
public static final BitSet FOLLOW_easydocDoc_in_document552 = new BitSet(new long[]{0x0000000000000772L});
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy