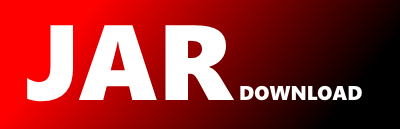
com.zhuang.mica.mqtt.model.MessageOptions Maven / Gradle / Ivy
The newest version!
package com.zhuang.mica.mqtt.model;
import cn.hutool.core.lang.Snowflake;
import cn.hutool.core.util.IdUtil;
import cn.hutool.core.util.StrUtil;
import lombok.Data;
@Data
public class MessageOptions {
public static final int MAX_WAIT_SECONDS = 10 * 60;
public static final int DEFAULT_WAIT_SECONDS = 20;
private String topic;
private String deviceId;
private Integer qos = 2;
private Boolean retain = false;
private boolean waitReply = false;
private Integer waitSeconds = DEFAULT_WAIT_SECONDS;
private boolean saveLog = false;
public Integer getWaitSeconds() {
if (waitSeconds == null) {
waitSeconds = DEFAULT_WAIT_SECONDS;
} else if (waitSeconds > MAX_WAIT_SECONDS) {
return MAX_WAIT_SECONDS;
}
return waitSeconds;
}
public String getMessageId() {
Snowflake snowflake = IdUtil.getSnowflake(1, 1);
String s = snowflake.nextIdStr();
int i = (waitReply ? 1 : 0) | ((saveLog ? 1 : 0) << 1);
String format = String.format("%02x", (byte) i);
return format + ":" + s;
}
public static boolean isWaitReplyByMessageId(String messageId) {
if (StrUtil.isEmpty(messageId) || !messageId.contains(":")) return false;
String[] split = messageId.split("\\:");
String s = split[0];
byte[] bytes = toBytes(s);
int i = bytes[0];
return (i & 1) > 0 ? true : false;
}
public static boolean isSaveLogByMessageId(String messageId) {
if (StrUtil.isEmpty(messageId) || !messageId.contains(":")) return false;
String[] split = messageId.split("\\:");
String s = split[0];
byte[] bytes = toBytes(s);
int i = bytes[0];
return (i & (1 << 1)) > 0 ? true : false;
}
private static byte[] toBytes(String s) {
final int len = s.length();
if (len % 2 != 0)
throw new IllegalArgumentException("hexBinary needs to be even-length: " + s);
byte[] out = new byte[len / 2];
for (int i = 0; i < len; i += 2) {
int h = hexToBin(s.charAt(i));
int l = hexToBin(s.charAt(i + 1));
if (h == -1 || l == -1)
throw new IllegalArgumentException("contains illegal character for hexBinary: " + s);
out[i / 2] = (byte) (h * 16 + l);
}
return out;
}
private static int hexToBin(char ch) {
if ('0' <= ch && ch <= '9') return ch - '0';
if ('A' <= ch && ch <= 'F') return ch - 'A' + 10;
if ('a' <= ch && ch <= 'f') return ch - 'a' + 10;
return -1;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy