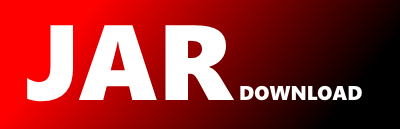
com.zhuang.mica.mqtt.service.BaseMqttService Maven / Gradle / Ivy
The newest version!
package com.zhuang.mica.mqtt.service;
import cn.hutool.core.date.DateUnit;
import cn.hutool.core.date.DateUtil;
import cn.hutool.core.thread.ThreadUtil;
import cn.hutool.core.util.StrUtil;
import cn.hutool.json.JSONUtil;
import com.zhuang.mica.mqtt.enums.DeviceTopics;
import com.zhuang.mica.mqtt.model.*;
import com.zhuang.mica.mqtt.util.JacksonUtils;
import com.zhuang.mica.mqtt.util.MqttCacheUtils;
import com.zhuang.mica.mqtt.util.cache.CacheUtils;
import net.dreamlu.iot.mqtt.codec.MqttQoS;
import org.springframework.beans.factory.annotation.Autowired;
import java.nio.charset.StandardCharsets;
import java.util.Date;
abstract public class BaseMqttService {
@Autowired
private DeviceService deviceService;
protected abstract boolean publish(String topic, byte[] payload, MqttQoS qos, boolean retain);
protected boolean publish(String topic, byte[] payload, MqttQoS qos, boolean retain, String clientId) {
return publish(topic, payload, qos, retain, null);
}
public String sendMessage(T message, MessageOptions options) {
if (StrUtil.isEmpty(message.getMessageId())) {
message.setMessageId(options.getMessageId());
}
String cacheKey = MqttCacheUtils.getReplyCacheKeyByMessageId(message.getMessageId());
boolean publishResult = publish(options.getTopic(), JacksonUtils.toJsonStr(message).getBytes(StandardCharsets.UTF_8),
MqttQoS.valueOf(options.getQos()), options.getRetain(), options.getDeviceId());
if (!publishResult) {
throw new RuntimeException("mqtt消息发送失败!");
} else {
// 记录日志
if (options.isSaveLog()) {
deviceService.addFunctionLog(options.getDeviceId(), JSONUtil.toJsonStr(message), false);
}
}
if (options.isWaitReply()) {
Date begin = new Date();
String msgResult = CacheUtils.get(cacheKey);
int timeoutSeconds = options.getWaitSeconds();
while (StrUtil.isEmpty(msgResult)) {
if (DateUtil.between(begin, new Date(), DateUnit.SECOND) > timeoutSeconds) {
throw new RuntimeException("结果等待超时!");
}
ThreadUtil.sleep(500);
msgResult = CacheUtils.get(cacheKey);
}
return msgResult;
}
return JSONUtil.toJsonStr(new Message());
}
public R invokeFunction(T functionMessage, MessageOptions messageOptions, Class classR) {
String topic = DeviceTopics.FUNCTION.getTopic(deviceService.getProductId(functionMessage.getDeviceId()), functionMessage.getDeviceId());
messageOptions.setTopic(topic);
messageOptions.setDeviceId(functionMessage.getDeviceId());
String s = sendMessage(functionMessage, messageOptions);
return JacksonUtils.toBean(s, classR);
}
public R invokeUpFunction(T functionMessage, MessageOptions messageOptions, Class classR) {
String topic = DeviceTopics.UP_FUNCTION.getTopic(deviceService.getProductId(functionMessage.getDeviceId()), functionMessage.getDeviceId());
messageOptions.setTopic(topic);
messageOptions.setDeviceId(functionMessage.getDeviceId());
String s = sendMessage(functionMessage, messageOptions);
return JacksonUtils.toBean(s, classR);
}
public void invokeFunctionReply(T functionReplyMessage) {
MessageOptions args = new MessageOptions();
args.setTopic(DeviceTopics.FUNCTION_REPLY.getTopic(deviceService.getProductId(functionReplyMessage.getDeviceId()), functionReplyMessage.getDeviceId()));
args.setDeviceId(functionReplyMessage.getDeviceId());
args.setSaveLog(false);
args.setWaitReply(false);
String s = sendMessage(functionReplyMessage, args);
}
public void invokeUpFunctionReply(T functionReplyMessage) {
MessageOptions args = new MessageOptions();
args.setTopic(DeviceTopics.UP_FUNCTION_REPLY.getTopic(deviceService.getProductId(functionReplyMessage.getDeviceId()), functionReplyMessage.getDeviceId()));
args.setDeviceId(functionReplyMessage.getDeviceId());
args.setSaveLog(false);
args.setWaitReply(false);
String s = sendMessage(functionReplyMessage, args);
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy