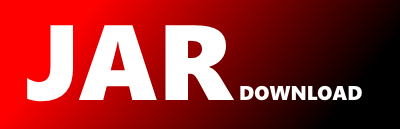
com.zhuang.netty.NettyBase Maven / Gradle / Ivy
package com.zhuang.netty;
import io.netty.channel.ChannelFuture;
import io.netty.channel.ChannelHandler;
import io.netty.channel.ChannelInitializer;
import io.netty.channel.socket.SocketChannel;
import java.util.ArrayList;
import java.util.List;
import java.util.function.Supplier;
abstract public class NettyBase {
protected int port;
protected List
© 2015 - 2025 Weber Informatics LLC | Privacy Policy