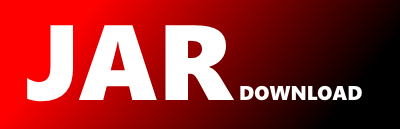
com.zhuang.netty.NettyClient Maven / Gradle / Ivy
package com.zhuang.netty;
import io.netty.bootstrap.Bootstrap;
import io.netty.channel.*;
import io.netty.channel.nio.NioEventLoopGroup;
import io.netty.channel.socket.nio.NioSocketChannel;
import lombok.extern.slf4j.Slf4j;
import java.util.concurrent.TimeUnit;
@Slf4j
public class NettyClient extends NettyBase {
private EventLoopGroup group;
private Bootstrap bootstrap;
private Channel channel;
private final String host;
public NettyClient(String host, int port) {
this.host = host;
this.port = port;
}
@Override
public void start() {
group = new NioEventLoopGroup();
bootstrap = new Bootstrap()
.group(group)
.channel(NioSocketChannel.class)
.option(ChannelOption.CONNECT_TIMEOUT_MILLIS, 5000)
.option(ChannelOption.SO_KEEPALIVE, true)
.handler(createChannelInitializer());
connect();
}
@Override
public void shutdown() {
group.shutdownGracefully();
}
public void connect() {
ChannelFuture channelFuture = bootstrap.connect(host, port);
channelFuture.addListener((ChannelFutureListener) future -> {
if (future.isSuccess()) {
channel = future.channel();
log.info("Client connect success -> port={}", port);
} else {
log.error("Client connect fail -> port={}", port, channelFuture.cause());
future.channel().eventLoop().schedule(this::connect, 5, TimeUnit.SECONDS);
}
});
setChannelFutureSync(channelFuture);
}
public boolean send(Object msg) {
if (channel == null || !channel.isActive()) {
log.error("Client send fail");
return false;
}
channel.writeAndFlush(msg);
return true;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy