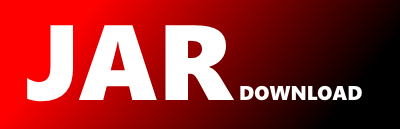
com.zhuang.netty.handler.client.NettyClientHandler Maven / Gradle / Ivy
package com.zhuang.netty.handler.client;
import com.zhuang.netty.NettyClient;
import io.netty.buffer.ByteBuf;
import io.netty.channel.ChannelHandlerContext;
import io.netty.channel.ChannelInboundHandlerAdapter;
import io.netty.util.CharsetUtil;
import lombok.extern.slf4j.Slf4j;
@Slf4j
public class NettyClientHandler extends ChannelInboundHandlerAdapter {
private NettyClient nettyClient;
public NettyClientHandler(NettyClient nettyClient) {
this.nettyClient = nettyClient;
}
@Override
public void channelActive(ChannelHandlerContext ctx) {
log.info("Client channel active");
String message = "Hello, Server!";
ByteBuf buf = ctx.alloc().buffer();
buf.writeBytes(message.getBytes(CharsetUtil.UTF_8));
ctx.writeAndFlush(buf);
}
@Override
public void channelRead(ChannelHandlerContext ctx, Object msg) {
ByteBuf buf = (ByteBuf) msg;
String message = buf.toString(CharsetUtil.UTF_8);
log.info("Client receive -> " + message);
}
@Override
public void channelInactive(ChannelHandlerContext ctx) {
log.info("Client channel inactive");
nettyClient.connect();
}
@Override
public void exceptionCaught(ChannelHandlerContext ctx, Throwable cause) {
log.error("exceptionCaught", cause);
ctx.close();
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy