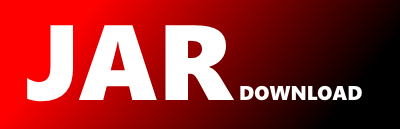
com.zhuang.netty.handler.decoder.SimpleDemoDecoder Maven / Gradle / Ivy
package com.zhuang.netty.handler.decoder;
import io.netty.buffer.ByteBuf;
import io.netty.channel.ChannelHandlerContext;
import io.netty.handler.codec.ByteToMessageDecoder;
import lombok.extern.slf4j.Slf4j;
import java.util.List;
@Slf4j
public class SimpleDemoDecoder extends ByteToMessageDecoder {
// 协议头长度为 4 个字节
private static final int HEADER_LENGTH = 4;
@Override
protected void decode(ChannelHandlerContext ctx, ByteBuf in, List
© 2015 - 2025 Weber Informatics LLC | Privacy Policy