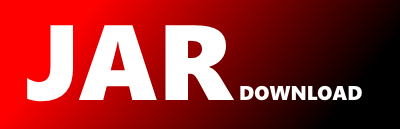
com.github.wendao76.util.LrsJsonMapper Maven / Gradle / Ivy
package com.github.wendao76.util;
import com.fasterxml.jackson.databind.DeserializationFeature;
import com.fasterxml.jackson.databind.ObjectMapper;
import com.fasterxml.jackson.databind.PropertyNamingStrategy;
import com.fasterxml.jackson.databind.SerializationFeature;
import com.fasterxml.jackson.databind.introspect.JacksonAnnotationIntrospector;
import java.io.IOException;
import java.io.InputStream;
import java.util.TimeZone;
/**
*
*
*
* @author wendao76 .
* @version 1.0.0.
*/
public class LrsJsonMapper {
private static ObjectMapper mapper;
static {
mapper = new ObjectMapper();
// 设置将驼峰命名法转换成下划线的方式输入输出
mapper.setPropertyNamingStrategy(PropertyNamingStrategy.SNAKE_CASE);
//设置时区
mapper.setTimeZone(TimeZone.getDefault());
// 设置时间为 ISO-8601 日期
mapper.configure(SerializationFeature.WRITE_DATES_AS_TIMESTAMPS, false);
mapper.configure(SerializationFeature.WRITE_ENUMS_USING_TO_STRING, true);
mapper.configure(SerializationFeature.WRITE_NULL_MAP_VALUES, false);
// 序列化BigDecimal时之间输出原始数字还是科学计数,默认false,即是否以toPlainString()科学计数方式来输出
mapper.configure(SerializationFeature.WRITE_BIGDECIMAL_AS_PLAIN, false);
//允许单引号
//mapper.configure(JsonParser.Feature.ALLOW_SINGLE_QUOTES, true);
//设定是否使用Enum的toString函数来读取Enum, 为False时使用Enum的name()函数来读取Enum,
mapper.configure(DeserializationFeature.READ_ENUMS_USING_TO_STRING, true);
// 如果输入不存在的字段时不会报错
mapper.configure(DeserializationFeature.FAIL_ON_UNKNOWN_PROPERTIES, false);
// 使用默认的Jsckson注解
mapper.setAnnotationIntrospector(new JacksonAnnotationIntrospector());
}
/**
* Gets mapper.
*
* @return the mapper
*/
public static ObjectMapper getMapper() {
return mapper;
}
/**
* Sets mapper.
*
* @param mapper the mapper
*/
public static void setMapper(ObjectMapper mapper) {
LrsJsonMapper.mapper = mapper;
}
/**
* Parse t.
*
* @param the type parameter
* @param json the json
* @param objectType the object type
* @return the t
* @throws IOException the io exception
*/
public static T parse(String json, Class objectType) throws IOException {
if (json == null)
return null;
return mapper.readValue(json, objectType);
}
/**
* Parse t.
*
* @param the type parameter
* @param stream the stream
* @param objectType the object type
* @return the t
* @throws IOException the io exception
*/
public static T parse(InputStream stream, Class objectType) throws IOException {
return mapper.readValue(stream, objectType);
}
/**
* To json string.
*
* @param obj the obj
* @return the string
* @throws IOException the io exception
*/
public static String toJson(Object obj) throws IOException {
return mapper.writeValueAsString(obj);
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy