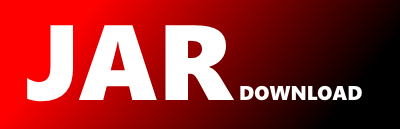
com.github.wenweihu86.raft.proto.RaftMessage Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of raft-java-core Show documentation
Show all versions of raft-java-core Show documentation
another Raft implementation for Java
The newest version!
// Generated by the protocol buffer compiler. DO NOT EDIT!
// source: raft.proto
package com.github.wenweihu86.raft.proto;
public final class RaftMessage {
private RaftMessage() {}
public static void registerAllExtensions(
com.google.protobuf.ExtensionRegistryLite registry) {
}
public static void registerAllExtensions(
com.google.protobuf.ExtensionRegistry registry) {
registerAllExtensions(
(com.google.protobuf.ExtensionRegistryLite) registry);
}
/**
* Protobuf enum {@code raft.ResCode}
*/
public enum ResCode
implements com.google.protobuf.ProtocolMessageEnum {
/**
* RES_CODE_SUCCESS = 0;
*/
RES_CODE_SUCCESS(0),
/**
* RES_CODE_FAIL = 1;
*/
RES_CODE_FAIL(1),
/**
* RES_CODE_NOT_LEADER = 2;
*/
RES_CODE_NOT_LEADER(2),
UNRECOGNIZED(-1),
;
/**
* RES_CODE_SUCCESS = 0;
*/
public static final int RES_CODE_SUCCESS_VALUE = 0;
/**
* RES_CODE_FAIL = 1;
*/
public static final int RES_CODE_FAIL_VALUE = 1;
/**
* RES_CODE_NOT_LEADER = 2;
*/
public static final int RES_CODE_NOT_LEADER_VALUE = 2;
public final int getNumber() {
if (this == UNRECOGNIZED) {
throw new java.lang.IllegalArgumentException(
"Can't get the number of an unknown enum value.");
}
return value;
}
/**
* @deprecated Use {@link #forNumber(int)} instead.
*/
@java.lang.Deprecated
public static ResCode valueOf(int value) {
return forNumber(value);
}
public static ResCode forNumber(int value) {
switch (value) {
case 0: return RES_CODE_SUCCESS;
case 1: return RES_CODE_FAIL;
case 2: return RES_CODE_NOT_LEADER;
default: return null;
}
}
public static com.google.protobuf.Internal.EnumLiteMap
internalGetValueMap() {
return internalValueMap;
}
private static final com.google.protobuf.Internal.EnumLiteMap<
ResCode> internalValueMap =
new com.google.protobuf.Internal.EnumLiteMap() {
public ResCode findValueByNumber(int number) {
return ResCode.forNumber(number);
}
};
public final com.google.protobuf.Descriptors.EnumValueDescriptor
getValueDescriptor() {
return getDescriptor().getValues().get(ordinal());
}
public final com.google.protobuf.Descriptors.EnumDescriptor
getDescriptorForType() {
return getDescriptor();
}
public static final com.google.protobuf.Descriptors.EnumDescriptor
getDescriptor() {
return com.github.wenweihu86.raft.proto.RaftMessage.getDescriptor().getEnumTypes().get(0);
}
private static final ResCode[] VALUES = values();
public static ResCode valueOf(
com.google.protobuf.Descriptors.EnumValueDescriptor desc) {
if (desc.getType() != getDescriptor()) {
throw new java.lang.IllegalArgumentException(
"EnumValueDescriptor is not for this type.");
}
if (desc.getIndex() == -1) {
return UNRECOGNIZED;
}
return VALUES[desc.getIndex()];
}
private final int value;
private ResCode(int value) {
this.value = value;
}
// @@protoc_insertion_point(enum_scope:raft.ResCode)
}
/**
* Protobuf enum {@code raft.EntryType}
*/
public enum EntryType
implements com.google.protobuf.ProtocolMessageEnum {
/**
* ENTRY_TYPE_DATA = 0;
*/
ENTRY_TYPE_DATA(0),
/**
* ENTRY_TYPE_CONFIGURATION = 1;
*/
ENTRY_TYPE_CONFIGURATION(1),
UNRECOGNIZED(-1),
;
/**
* ENTRY_TYPE_DATA = 0;
*/
public static final int ENTRY_TYPE_DATA_VALUE = 0;
/**
* ENTRY_TYPE_CONFIGURATION = 1;
*/
public static final int ENTRY_TYPE_CONFIGURATION_VALUE = 1;
public final int getNumber() {
if (this == UNRECOGNIZED) {
throw new java.lang.IllegalArgumentException(
"Can't get the number of an unknown enum value.");
}
return value;
}
/**
* @deprecated Use {@link #forNumber(int)} instead.
*/
@java.lang.Deprecated
public static EntryType valueOf(int value) {
return forNumber(value);
}
public static EntryType forNumber(int value) {
switch (value) {
case 0: return ENTRY_TYPE_DATA;
case 1: return ENTRY_TYPE_CONFIGURATION;
default: return null;
}
}
public static com.google.protobuf.Internal.EnumLiteMap
internalGetValueMap() {
return internalValueMap;
}
private static final com.google.protobuf.Internal.EnumLiteMap<
EntryType> internalValueMap =
new com.google.protobuf.Internal.EnumLiteMap() {
public EntryType findValueByNumber(int number) {
return EntryType.forNumber(number);
}
};
public final com.google.protobuf.Descriptors.EnumValueDescriptor
getValueDescriptor() {
return getDescriptor().getValues().get(ordinal());
}
public final com.google.protobuf.Descriptors.EnumDescriptor
getDescriptorForType() {
return getDescriptor();
}
public static final com.google.protobuf.Descriptors.EnumDescriptor
getDescriptor() {
return com.github.wenweihu86.raft.proto.RaftMessage.getDescriptor().getEnumTypes().get(1);
}
private static final EntryType[] VALUES = values();
public static EntryType valueOf(
com.google.protobuf.Descriptors.EnumValueDescriptor desc) {
if (desc.getType() != getDescriptor()) {
throw new java.lang.IllegalArgumentException(
"EnumValueDescriptor is not for this type.");
}
if (desc.getIndex() == -1) {
return UNRECOGNIZED;
}
return VALUES[desc.getIndex()];
}
private final int value;
private EntryType(int value) {
this.value = value;
}
// @@protoc_insertion_point(enum_scope:raft.EntryType)
}
public interface EndPointOrBuilder extends
// @@protoc_insertion_point(interface_extends:raft.EndPoint)
com.google.protobuf.MessageOrBuilder {
/**
* optional string host = 1;
*/
java.lang.String getHost();
/**
* optional string host = 1;
*/
com.google.protobuf.ByteString
getHostBytes();
/**
* optional uint32 port = 2;
*/
int getPort();
}
/**
* Protobuf type {@code raft.EndPoint}
*/
public static final class EndPoint extends
com.google.protobuf.GeneratedMessageV3 implements
// @@protoc_insertion_point(message_implements:raft.EndPoint)
EndPointOrBuilder {
// Use EndPoint.newBuilder() to construct.
private EndPoint(com.google.protobuf.GeneratedMessageV3.Builder> builder) {
super(builder);
}
private EndPoint() {
host_ = "";
port_ = 0;
}
@java.lang.Override
public final com.google.protobuf.UnknownFieldSet
getUnknownFields() {
return com.google.protobuf.UnknownFieldSet.getDefaultInstance();
}
private EndPoint(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
this();
int mutable_bitField0_ = 0;
try {
boolean done = false;
while (!done) {
int tag = input.readTag();
switch (tag) {
case 0:
done = true;
break;
default: {
if (!input.skipField(tag)) {
done = true;
}
break;
}
case 10: {
java.lang.String s = input.readStringRequireUtf8();
host_ = s;
break;
}
case 16: {
port_ = input.readUInt32();
break;
}
}
}
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.setUnfinishedMessage(this);
} catch (java.io.IOException e) {
throw new com.google.protobuf.InvalidProtocolBufferException(
e).setUnfinishedMessage(this);
} finally {
makeExtensionsImmutable();
}
}
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return com.github.wenweihu86.raft.proto.RaftMessage.internal_static_raft_EndPoint_descriptor;
}
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return com.github.wenweihu86.raft.proto.RaftMessage.internal_static_raft_EndPoint_fieldAccessorTable
.ensureFieldAccessorsInitialized(
com.github.wenweihu86.raft.proto.RaftMessage.EndPoint.class, com.github.wenweihu86.raft.proto.RaftMessage.EndPoint.Builder.class);
}
public static final int HOST_FIELD_NUMBER = 1;
private volatile java.lang.Object host_;
/**
* optional string host = 1;
*/
public java.lang.String getHost() {
java.lang.Object ref = host_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
host_ = s;
return s;
}
}
/**
* optional string host = 1;
*/
public com.google.protobuf.ByteString
getHostBytes() {
java.lang.Object ref = host_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
host_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
public static final int PORT_FIELD_NUMBER = 2;
private int port_;
/**
* optional uint32 port = 2;
*/
public int getPort() {
return port_;
}
private byte memoizedIsInitialized = -1;
public final boolean isInitialized() {
byte isInitialized = memoizedIsInitialized;
if (isInitialized == 1) return true;
if (isInitialized == 0) return false;
memoizedIsInitialized = 1;
return true;
}
public void writeTo(com.google.protobuf.CodedOutputStream output)
throws java.io.IOException {
if (!getHostBytes().isEmpty()) {
com.google.protobuf.GeneratedMessageV3.writeString(output, 1, host_);
}
if (port_ != 0) {
output.writeUInt32(2, port_);
}
}
public int getSerializedSize() {
int size = memoizedSize;
if (size != -1) return size;
size = 0;
if (!getHostBytes().isEmpty()) {
size += com.google.protobuf.GeneratedMessageV3.computeStringSize(1, host_);
}
if (port_ != 0) {
size += com.google.protobuf.CodedOutputStream
.computeUInt32Size(2, port_);
}
memoizedSize = size;
return size;
}
private static final long serialVersionUID = 0L;
@java.lang.Override
public boolean equals(final java.lang.Object obj) {
if (obj == this) {
return true;
}
if (!(obj instanceof com.github.wenweihu86.raft.proto.RaftMessage.EndPoint)) {
return super.equals(obj);
}
com.github.wenweihu86.raft.proto.RaftMessage.EndPoint other = (com.github.wenweihu86.raft.proto.RaftMessage.EndPoint) obj;
boolean result = true;
result = result && getHost()
.equals(other.getHost());
result = result && (getPort()
== other.getPort());
return result;
}
@java.lang.Override
public int hashCode() {
if (memoizedHashCode != 0) {
return memoizedHashCode;
}
int hash = 41;
hash = (19 * hash) + getDescriptorForType().hashCode();
hash = (37 * hash) + HOST_FIELD_NUMBER;
hash = (53 * hash) + getHost().hashCode();
hash = (37 * hash) + PORT_FIELD_NUMBER;
hash = (53 * hash) + getPort();
hash = (29 * hash) + unknownFields.hashCode();
memoizedHashCode = hash;
return hash;
}
public static com.github.wenweihu86.raft.proto.RaftMessage.EndPoint parseFrom(
com.google.protobuf.ByteString data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static com.github.wenweihu86.raft.proto.RaftMessage.EndPoint parseFrom(
com.google.protobuf.ByteString data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static com.github.wenweihu86.raft.proto.RaftMessage.EndPoint parseFrom(byte[] data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static com.github.wenweihu86.raft.proto.RaftMessage.EndPoint parseFrom(
byte[] data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static com.github.wenweihu86.raft.proto.RaftMessage.EndPoint parseFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static com.github.wenweihu86.raft.proto.RaftMessage.EndPoint parseFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
public static com.github.wenweihu86.raft.proto.RaftMessage.EndPoint parseDelimitedFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input);
}
public static com.github.wenweihu86.raft.proto.RaftMessage.EndPoint parseDelimitedFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input, extensionRegistry);
}
public static com.github.wenweihu86.raft.proto.RaftMessage.EndPoint parseFrom(
com.google.protobuf.CodedInputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static com.github.wenweihu86.raft.proto.RaftMessage.EndPoint parseFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
public Builder newBuilderForType() { return newBuilder(); }
public static Builder newBuilder() {
return DEFAULT_INSTANCE.toBuilder();
}
public static Builder newBuilder(com.github.wenweihu86.raft.proto.RaftMessage.EndPoint prototype) {
return DEFAULT_INSTANCE.toBuilder().mergeFrom(prototype);
}
public Builder toBuilder() {
return this == DEFAULT_INSTANCE
? new Builder() : new Builder().mergeFrom(this);
}
@java.lang.Override
protected Builder newBuilderForType(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
Builder builder = new Builder(parent);
return builder;
}
/**
* Protobuf type {@code raft.EndPoint}
*/
public static final class Builder extends
com.google.protobuf.GeneratedMessageV3.Builder implements
// @@protoc_insertion_point(builder_implements:raft.EndPoint)
com.github.wenweihu86.raft.proto.RaftMessage.EndPointOrBuilder {
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return com.github.wenweihu86.raft.proto.RaftMessage.internal_static_raft_EndPoint_descriptor;
}
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return com.github.wenweihu86.raft.proto.RaftMessage.internal_static_raft_EndPoint_fieldAccessorTable
.ensureFieldAccessorsInitialized(
com.github.wenweihu86.raft.proto.RaftMessage.EndPoint.class, com.github.wenweihu86.raft.proto.RaftMessage.EndPoint.Builder.class);
}
// Construct using com.github.wenweihu86.raft.proto.RaftMessage.EndPoint.newBuilder()
private Builder() {
maybeForceBuilderInitialization();
}
private Builder(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
super(parent);
maybeForceBuilderInitialization();
}
private void maybeForceBuilderInitialization() {
if (com.google.protobuf.GeneratedMessageV3
.alwaysUseFieldBuilders) {
}
}
public Builder clear() {
super.clear();
host_ = "";
port_ = 0;
return this;
}
public com.google.protobuf.Descriptors.Descriptor
getDescriptorForType() {
return com.github.wenweihu86.raft.proto.RaftMessage.internal_static_raft_EndPoint_descriptor;
}
public com.github.wenweihu86.raft.proto.RaftMessage.EndPoint getDefaultInstanceForType() {
return com.github.wenweihu86.raft.proto.RaftMessage.EndPoint.getDefaultInstance();
}
public com.github.wenweihu86.raft.proto.RaftMessage.EndPoint build() {
com.github.wenweihu86.raft.proto.RaftMessage.EndPoint result = buildPartial();
if (!result.isInitialized()) {
throw newUninitializedMessageException(result);
}
return result;
}
public com.github.wenweihu86.raft.proto.RaftMessage.EndPoint buildPartial() {
com.github.wenweihu86.raft.proto.RaftMessage.EndPoint result = new com.github.wenweihu86.raft.proto.RaftMessage.EndPoint(this);
result.host_ = host_;
result.port_ = port_;
onBuilt();
return result;
}
public Builder clone() {
return (Builder) super.clone();
}
public Builder setField(
com.google.protobuf.Descriptors.FieldDescriptor field,
Object value) {
return (Builder) super.setField(field, value);
}
public Builder clearField(
com.google.protobuf.Descriptors.FieldDescriptor field) {
return (Builder) super.clearField(field);
}
public Builder clearOneof(
com.google.protobuf.Descriptors.OneofDescriptor oneof) {
return (Builder) super.clearOneof(oneof);
}
public Builder setRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
int index, Object value) {
return (Builder) super.setRepeatedField(field, index, value);
}
public Builder addRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
Object value) {
return (Builder) super.addRepeatedField(field, value);
}
public Builder mergeFrom(com.google.protobuf.Message other) {
if (other instanceof com.github.wenweihu86.raft.proto.RaftMessage.EndPoint) {
return mergeFrom((com.github.wenweihu86.raft.proto.RaftMessage.EndPoint)other);
} else {
super.mergeFrom(other);
return this;
}
}
public Builder mergeFrom(com.github.wenweihu86.raft.proto.RaftMessage.EndPoint other) {
if (other == com.github.wenweihu86.raft.proto.RaftMessage.EndPoint.getDefaultInstance()) return this;
if (!other.getHost().isEmpty()) {
host_ = other.host_;
onChanged();
}
if (other.getPort() != 0) {
setPort(other.getPort());
}
onChanged();
return this;
}
public final boolean isInitialized() {
return true;
}
public Builder mergeFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
com.github.wenweihu86.raft.proto.RaftMessage.EndPoint parsedMessage = null;
try {
parsedMessage = PARSER.parsePartialFrom(input, extensionRegistry);
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
parsedMessage = (com.github.wenweihu86.raft.proto.RaftMessage.EndPoint) e.getUnfinishedMessage();
throw e.unwrapIOException();
} finally {
if (parsedMessage != null) {
mergeFrom(parsedMessage);
}
}
return this;
}
private java.lang.Object host_ = "";
/**
* optional string host = 1;
*/
public java.lang.String getHost() {
java.lang.Object ref = host_;
if (!(ref instanceof java.lang.String)) {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
host_ = s;
return s;
} else {
return (java.lang.String) ref;
}
}
/**
* optional string host = 1;
*/
public com.google.protobuf.ByteString
getHostBytes() {
java.lang.Object ref = host_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
host_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
* optional string host = 1;
*/
public Builder setHost(
java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
host_ = value;
onChanged();
return this;
}
/**
* optional string host = 1;
*/
public Builder clearHost() {
host_ = getDefaultInstance().getHost();
onChanged();
return this;
}
/**
* optional string host = 1;
*/
public Builder setHostBytes(
com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
checkByteStringIsUtf8(value);
host_ = value;
onChanged();
return this;
}
private int port_ ;
/**
* optional uint32 port = 2;
*/
public int getPort() {
return port_;
}
/**
* optional uint32 port = 2;
*/
public Builder setPort(int value) {
port_ = value;
onChanged();
return this;
}
/**
* optional uint32 port = 2;
*/
public Builder clearPort() {
port_ = 0;
onChanged();
return this;
}
public final Builder setUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return this;
}
public final Builder mergeUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return this;
}
// @@protoc_insertion_point(builder_scope:raft.EndPoint)
}
// @@protoc_insertion_point(class_scope:raft.EndPoint)
private static final com.github.wenweihu86.raft.proto.RaftMessage.EndPoint DEFAULT_INSTANCE;
static {
DEFAULT_INSTANCE = new com.github.wenweihu86.raft.proto.RaftMessage.EndPoint();
}
public static com.github.wenweihu86.raft.proto.RaftMessage.EndPoint getDefaultInstance() {
return DEFAULT_INSTANCE;
}
private static final com.google.protobuf.Parser
PARSER = new com.google.protobuf.AbstractParser() {
public EndPoint parsePartialFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return new EndPoint(input, extensionRegistry);
}
};
public static com.google.protobuf.Parser parser() {
return PARSER;
}
@java.lang.Override
public com.google.protobuf.Parser getParserForType() {
return PARSER;
}
public com.github.wenweihu86.raft.proto.RaftMessage.EndPoint getDefaultInstanceForType() {
return DEFAULT_INSTANCE;
}
}
public interface ServerOrBuilder extends
// @@protoc_insertion_point(interface_extends:raft.Server)
com.google.protobuf.MessageOrBuilder {
/**
* optional uint32 server_id = 1;
*/
int getServerId();
/**
* optional .raft.EndPoint end_point = 2;
*/
boolean hasEndPoint();
/**
* optional .raft.EndPoint end_point = 2;
*/
com.github.wenweihu86.raft.proto.RaftMessage.EndPoint getEndPoint();
/**
* optional .raft.EndPoint end_point = 2;
*/
com.github.wenweihu86.raft.proto.RaftMessage.EndPointOrBuilder getEndPointOrBuilder();
}
/**
* Protobuf type {@code raft.Server}
*/
public static final class Server extends
com.google.protobuf.GeneratedMessageV3 implements
// @@protoc_insertion_point(message_implements:raft.Server)
ServerOrBuilder {
// Use Server.newBuilder() to construct.
private Server(com.google.protobuf.GeneratedMessageV3.Builder> builder) {
super(builder);
}
private Server() {
serverId_ = 0;
}
@java.lang.Override
public final com.google.protobuf.UnknownFieldSet
getUnknownFields() {
return com.google.protobuf.UnknownFieldSet.getDefaultInstance();
}
private Server(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
this();
int mutable_bitField0_ = 0;
try {
boolean done = false;
while (!done) {
int tag = input.readTag();
switch (tag) {
case 0:
done = true;
break;
default: {
if (!input.skipField(tag)) {
done = true;
}
break;
}
case 8: {
serverId_ = input.readUInt32();
break;
}
case 18: {
com.github.wenweihu86.raft.proto.RaftMessage.EndPoint.Builder subBuilder = null;
if (endPoint_ != null) {
subBuilder = endPoint_.toBuilder();
}
endPoint_ = input.readMessage(com.github.wenweihu86.raft.proto.RaftMessage.EndPoint.parser(), extensionRegistry);
if (subBuilder != null) {
subBuilder.mergeFrom(endPoint_);
endPoint_ = subBuilder.buildPartial();
}
break;
}
}
}
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.setUnfinishedMessage(this);
} catch (java.io.IOException e) {
throw new com.google.protobuf.InvalidProtocolBufferException(
e).setUnfinishedMessage(this);
} finally {
makeExtensionsImmutable();
}
}
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return com.github.wenweihu86.raft.proto.RaftMessage.internal_static_raft_Server_descriptor;
}
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return com.github.wenweihu86.raft.proto.RaftMessage.internal_static_raft_Server_fieldAccessorTable
.ensureFieldAccessorsInitialized(
com.github.wenweihu86.raft.proto.RaftMessage.Server.class, com.github.wenweihu86.raft.proto.RaftMessage.Server.Builder.class);
}
public static final int SERVER_ID_FIELD_NUMBER = 1;
private int serverId_;
/**
* optional uint32 server_id = 1;
*/
public int getServerId() {
return serverId_;
}
public static final int END_POINT_FIELD_NUMBER = 2;
private com.github.wenweihu86.raft.proto.RaftMessage.EndPoint endPoint_;
/**
* optional .raft.EndPoint end_point = 2;
*/
public boolean hasEndPoint() {
return endPoint_ != null;
}
/**
* optional .raft.EndPoint end_point = 2;
*/
public com.github.wenweihu86.raft.proto.RaftMessage.EndPoint getEndPoint() {
return endPoint_ == null ? com.github.wenweihu86.raft.proto.RaftMessage.EndPoint.getDefaultInstance() : endPoint_;
}
/**
* optional .raft.EndPoint end_point = 2;
*/
public com.github.wenweihu86.raft.proto.RaftMessage.EndPointOrBuilder getEndPointOrBuilder() {
return getEndPoint();
}
private byte memoizedIsInitialized = -1;
public final boolean isInitialized() {
byte isInitialized = memoizedIsInitialized;
if (isInitialized == 1) return true;
if (isInitialized == 0) return false;
memoizedIsInitialized = 1;
return true;
}
public void writeTo(com.google.protobuf.CodedOutputStream output)
throws java.io.IOException {
if (serverId_ != 0) {
output.writeUInt32(1, serverId_);
}
if (endPoint_ != null) {
output.writeMessage(2, getEndPoint());
}
}
public int getSerializedSize() {
int size = memoizedSize;
if (size != -1) return size;
size = 0;
if (serverId_ != 0) {
size += com.google.protobuf.CodedOutputStream
.computeUInt32Size(1, serverId_);
}
if (endPoint_ != null) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(2, getEndPoint());
}
memoizedSize = size;
return size;
}
private static final long serialVersionUID = 0L;
@java.lang.Override
public boolean equals(final java.lang.Object obj) {
if (obj == this) {
return true;
}
if (!(obj instanceof com.github.wenweihu86.raft.proto.RaftMessage.Server)) {
return super.equals(obj);
}
com.github.wenweihu86.raft.proto.RaftMessage.Server other = (com.github.wenweihu86.raft.proto.RaftMessage.Server) obj;
boolean result = true;
result = result && (getServerId()
== other.getServerId());
result = result && (hasEndPoint() == other.hasEndPoint());
if (hasEndPoint()) {
result = result && getEndPoint()
.equals(other.getEndPoint());
}
return result;
}
@java.lang.Override
public int hashCode() {
if (memoizedHashCode != 0) {
return memoizedHashCode;
}
int hash = 41;
hash = (19 * hash) + getDescriptorForType().hashCode();
hash = (37 * hash) + SERVER_ID_FIELD_NUMBER;
hash = (53 * hash) + getServerId();
if (hasEndPoint()) {
hash = (37 * hash) + END_POINT_FIELD_NUMBER;
hash = (53 * hash) + getEndPoint().hashCode();
}
hash = (29 * hash) + unknownFields.hashCode();
memoizedHashCode = hash;
return hash;
}
public static com.github.wenweihu86.raft.proto.RaftMessage.Server parseFrom(
com.google.protobuf.ByteString data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static com.github.wenweihu86.raft.proto.RaftMessage.Server parseFrom(
com.google.protobuf.ByteString data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static com.github.wenweihu86.raft.proto.RaftMessage.Server parseFrom(byte[] data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static com.github.wenweihu86.raft.proto.RaftMessage.Server parseFrom(
byte[] data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static com.github.wenweihu86.raft.proto.RaftMessage.Server parseFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static com.github.wenweihu86.raft.proto.RaftMessage.Server parseFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
public static com.github.wenweihu86.raft.proto.RaftMessage.Server parseDelimitedFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input);
}
public static com.github.wenweihu86.raft.proto.RaftMessage.Server parseDelimitedFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input, extensionRegistry);
}
public static com.github.wenweihu86.raft.proto.RaftMessage.Server parseFrom(
com.google.protobuf.CodedInputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static com.github.wenweihu86.raft.proto.RaftMessage.Server parseFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
public Builder newBuilderForType() { return newBuilder(); }
public static Builder newBuilder() {
return DEFAULT_INSTANCE.toBuilder();
}
public static Builder newBuilder(com.github.wenweihu86.raft.proto.RaftMessage.Server prototype) {
return DEFAULT_INSTANCE.toBuilder().mergeFrom(prototype);
}
public Builder toBuilder() {
return this == DEFAULT_INSTANCE
? new Builder() : new Builder().mergeFrom(this);
}
@java.lang.Override
protected Builder newBuilderForType(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
Builder builder = new Builder(parent);
return builder;
}
/**
* Protobuf type {@code raft.Server}
*/
public static final class Builder extends
com.google.protobuf.GeneratedMessageV3.Builder implements
// @@protoc_insertion_point(builder_implements:raft.Server)
com.github.wenweihu86.raft.proto.RaftMessage.ServerOrBuilder {
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return com.github.wenweihu86.raft.proto.RaftMessage.internal_static_raft_Server_descriptor;
}
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return com.github.wenweihu86.raft.proto.RaftMessage.internal_static_raft_Server_fieldAccessorTable
.ensureFieldAccessorsInitialized(
com.github.wenweihu86.raft.proto.RaftMessage.Server.class, com.github.wenweihu86.raft.proto.RaftMessage.Server.Builder.class);
}
// Construct using com.github.wenweihu86.raft.proto.RaftMessage.Server.newBuilder()
private Builder() {
maybeForceBuilderInitialization();
}
private Builder(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
super(parent);
maybeForceBuilderInitialization();
}
private void maybeForceBuilderInitialization() {
if (com.google.protobuf.GeneratedMessageV3
.alwaysUseFieldBuilders) {
}
}
public Builder clear() {
super.clear();
serverId_ = 0;
if (endPointBuilder_ == null) {
endPoint_ = null;
} else {
endPoint_ = null;
endPointBuilder_ = null;
}
return this;
}
public com.google.protobuf.Descriptors.Descriptor
getDescriptorForType() {
return com.github.wenweihu86.raft.proto.RaftMessage.internal_static_raft_Server_descriptor;
}
public com.github.wenweihu86.raft.proto.RaftMessage.Server getDefaultInstanceForType() {
return com.github.wenweihu86.raft.proto.RaftMessage.Server.getDefaultInstance();
}
public com.github.wenweihu86.raft.proto.RaftMessage.Server build() {
com.github.wenweihu86.raft.proto.RaftMessage.Server result = buildPartial();
if (!result.isInitialized()) {
throw newUninitializedMessageException(result);
}
return result;
}
public com.github.wenweihu86.raft.proto.RaftMessage.Server buildPartial() {
com.github.wenweihu86.raft.proto.RaftMessage.Server result = new com.github.wenweihu86.raft.proto.RaftMessage.Server(this);
result.serverId_ = serverId_;
if (endPointBuilder_ == null) {
result.endPoint_ = endPoint_;
} else {
result.endPoint_ = endPointBuilder_.build();
}
onBuilt();
return result;
}
public Builder clone() {
return (Builder) super.clone();
}
public Builder setField(
com.google.protobuf.Descriptors.FieldDescriptor field,
Object value) {
return (Builder) super.setField(field, value);
}
public Builder clearField(
com.google.protobuf.Descriptors.FieldDescriptor field) {
return (Builder) super.clearField(field);
}
public Builder clearOneof(
com.google.protobuf.Descriptors.OneofDescriptor oneof) {
return (Builder) super.clearOneof(oneof);
}
public Builder setRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
int index, Object value) {
return (Builder) super.setRepeatedField(field, index, value);
}
public Builder addRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
Object value) {
return (Builder) super.addRepeatedField(field, value);
}
public Builder mergeFrom(com.google.protobuf.Message other) {
if (other instanceof com.github.wenweihu86.raft.proto.RaftMessage.Server) {
return mergeFrom((com.github.wenweihu86.raft.proto.RaftMessage.Server)other);
} else {
super.mergeFrom(other);
return this;
}
}
public Builder mergeFrom(com.github.wenweihu86.raft.proto.RaftMessage.Server other) {
if (other == com.github.wenweihu86.raft.proto.RaftMessage.Server.getDefaultInstance()) return this;
if (other.getServerId() != 0) {
setServerId(other.getServerId());
}
if (other.hasEndPoint()) {
mergeEndPoint(other.getEndPoint());
}
onChanged();
return this;
}
public final boolean isInitialized() {
return true;
}
public Builder mergeFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
com.github.wenweihu86.raft.proto.RaftMessage.Server parsedMessage = null;
try {
parsedMessage = PARSER.parsePartialFrom(input, extensionRegistry);
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
parsedMessage = (com.github.wenweihu86.raft.proto.RaftMessage.Server) e.getUnfinishedMessage();
throw e.unwrapIOException();
} finally {
if (parsedMessage != null) {
mergeFrom(parsedMessage);
}
}
return this;
}
private int serverId_ ;
/**
* optional uint32 server_id = 1;
*/
public int getServerId() {
return serverId_;
}
/**
* optional uint32 server_id = 1;
*/
public Builder setServerId(int value) {
serverId_ = value;
onChanged();
return this;
}
/**
* optional uint32 server_id = 1;
*/
public Builder clearServerId() {
serverId_ = 0;
onChanged();
return this;
}
private com.github.wenweihu86.raft.proto.RaftMessage.EndPoint endPoint_ = null;
private com.google.protobuf.SingleFieldBuilderV3<
com.github.wenweihu86.raft.proto.RaftMessage.EndPoint, com.github.wenweihu86.raft.proto.RaftMessage.EndPoint.Builder, com.github.wenweihu86.raft.proto.RaftMessage.EndPointOrBuilder> endPointBuilder_;
/**
* optional .raft.EndPoint end_point = 2;
*/
public boolean hasEndPoint() {
return endPointBuilder_ != null || endPoint_ != null;
}
/**
* optional .raft.EndPoint end_point = 2;
*/
public com.github.wenweihu86.raft.proto.RaftMessage.EndPoint getEndPoint() {
if (endPointBuilder_ == null) {
return endPoint_ == null ? com.github.wenweihu86.raft.proto.RaftMessage.EndPoint.getDefaultInstance() : endPoint_;
} else {
return endPointBuilder_.getMessage();
}
}
/**
* optional .raft.EndPoint end_point = 2;
*/
public Builder setEndPoint(com.github.wenweihu86.raft.proto.RaftMessage.EndPoint value) {
if (endPointBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
endPoint_ = value;
onChanged();
} else {
endPointBuilder_.setMessage(value);
}
return this;
}
/**
* optional .raft.EndPoint end_point = 2;
*/
public Builder setEndPoint(
com.github.wenweihu86.raft.proto.RaftMessage.EndPoint.Builder builderForValue) {
if (endPointBuilder_ == null) {
endPoint_ = builderForValue.build();
onChanged();
} else {
endPointBuilder_.setMessage(builderForValue.build());
}
return this;
}
/**
* optional .raft.EndPoint end_point = 2;
*/
public Builder mergeEndPoint(com.github.wenweihu86.raft.proto.RaftMessage.EndPoint value) {
if (endPointBuilder_ == null) {
if (endPoint_ != null) {
endPoint_ =
com.github.wenweihu86.raft.proto.RaftMessage.EndPoint.newBuilder(endPoint_).mergeFrom(value).buildPartial();
} else {
endPoint_ = value;
}
onChanged();
} else {
endPointBuilder_.mergeFrom(value);
}
return this;
}
/**
* optional .raft.EndPoint end_point = 2;
*/
public Builder clearEndPoint() {
if (endPointBuilder_ == null) {
endPoint_ = null;
onChanged();
} else {
endPoint_ = null;
endPointBuilder_ = null;
}
return this;
}
/**
* optional .raft.EndPoint end_point = 2;
*/
public com.github.wenweihu86.raft.proto.RaftMessage.EndPoint.Builder getEndPointBuilder() {
onChanged();
return getEndPointFieldBuilder().getBuilder();
}
/**
* optional .raft.EndPoint end_point = 2;
*/
public com.github.wenweihu86.raft.proto.RaftMessage.EndPointOrBuilder getEndPointOrBuilder() {
if (endPointBuilder_ != null) {
return endPointBuilder_.getMessageOrBuilder();
} else {
return endPoint_ == null ?
com.github.wenweihu86.raft.proto.RaftMessage.EndPoint.getDefaultInstance() : endPoint_;
}
}
/**
* optional .raft.EndPoint end_point = 2;
*/
private com.google.protobuf.SingleFieldBuilderV3<
com.github.wenweihu86.raft.proto.RaftMessage.EndPoint, com.github.wenweihu86.raft.proto.RaftMessage.EndPoint.Builder, com.github.wenweihu86.raft.proto.RaftMessage.EndPointOrBuilder>
getEndPointFieldBuilder() {
if (endPointBuilder_ == null) {
endPointBuilder_ = new com.google.protobuf.SingleFieldBuilderV3<
com.github.wenweihu86.raft.proto.RaftMessage.EndPoint, com.github.wenweihu86.raft.proto.RaftMessage.EndPoint.Builder, com.github.wenweihu86.raft.proto.RaftMessage.EndPointOrBuilder>(
getEndPoint(),
getParentForChildren(),
isClean());
endPoint_ = null;
}
return endPointBuilder_;
}
public final Builder setUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return this;
}
public final Builder mergeUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return this;
}
// @@protoc_insertion_point(builder_scope:raft.Server)
}
// @@protoc_insertion_point(class_scope:raft.Server)
private static final com.github.wenweihu86.raft.proto.RaftMessage.Server DEFAULT_INSTANCE;
static {
DEFAULT_INSTANCE = new com.github.wenweihu86.raft.proto.RaftMessage.Server();
}
public static com.github.wenweihu86.raft.proto.RaftMessage.Server getDefaultInstance() {
return DEFAULT_INSTANCE;
}
private static final com.google.protobuf.Parser
PARSER = new com.google.protobuf.AbstractParser() {
public Server parsePartialFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return new Server(input, extensionRegistry);
}
};
public static com.google.protobuf.Parser parser() {
return PARSER;
}
@java.lang.Override
public com.google.protobuf.Parser getParserForType() {
return PARSER;
}
public com.github.wenweihu86.raft.proto.RaftMessage.Server getDefaultInstanceForType() {
return DEFAULT_INSTANCE;
}
}
public interface ConfigurationOrBuilder extends
// @@protoc_insertion_point(interface_extends:raft.Configuration)
com.google.protobuf.MessageOrBuilder {
/**
* repeated .raft.Server servers = 1;
*/
java.util.List
getServersList();
/**
* repeated .raft.Server servers = 1;
*/
com.github.wenweihu86.raft.proto.RaftMessage.Server getServers(int index);
/**
* repeated .raft.Server servers = 1;
*/
int getServersCount();
/**
* repeated .raft.Server servers = 1;
*/
java.util.List extends com.github.wenweihu86.raft.proto.RaftMessage.ServerOrBuilder>
getServersOrBuilderList();
/**
* repeated .raft.Server servers = 1;
*/
com.github.wenweihu86.raft.proto.RaftMessage.ServerOrBuilder getServersOrBuilder(
int index);
}
/**
* Protobuf type {@code raft.Configuration}
*/
public static final class Configuration extends
com.google.protobuf.GeneratedMessageV3 implements
// @@protoc_insertion_point(message_implements:raft.Configuration)
ConfigurationOrBuilder {
// Use Configuration.newBuilder() to construct.
private Configuration(com.google.protobuf.GeneratedMessageV3.Builder> builder) {
super(builder);
}
private Configuration() {
servers_ = java.util.Collections.emptyList();
}
@java.lang.Override
public final com.google.protobuf.UnknownFieldSet
getUnknownFields() {
return com.google.protobuf.UnknownFieldSet.getDefaultInstance();
}
private Configuration(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
this();
int mutable_bitField0_ = 0;
try {
boolean done = false;
while (!done) {
int tag = input.readTag();
switch (tag) {
case 0:
done = true;
break;
default: {
if (!input.skipField(tag)) {
done = true;
}
break;
}
case 10: {
if (!((mutable_bitField0_ & 0x00000001) == 0x00000001)) {
servers_ = new java.util.ArrayList();
mutable_bitField0_ |= 0x00000001;
}
servers_.add(
input.readMessage(com.github.wenweihu86.raft.proto.RaftMessage.Server.parser(), extensionRegistry));
break;
}
}
}
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.setUnfinishedMessage(this);
} catch (java.io.IOException e) {
throw new com.google.protobuf.InvalidProtocolBufferException(
e).setUnfinishedMessage(this);
} finally {
if (((mutable_bitField0_ & 0x00000001) == 0x00000001)) {
servers_ = java.util.Collections.unmodifiableList(servers_);
}
makeExtensionsImmutable();
}
}
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return com.github.wenweihu86.raft.proto.RaftMessage.internal_static_raft_Configuration_descriptor;
}
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return com.github.wenweihu86.raft.proto.RaftMessage.internal_static_raft_Configuration_fieldAccessorTable
.ensureFieldAccessorsInitialized(
com.github.wenweihu86.raft.proto.RaftMessage.Configuration.class, com.github.wenweihu86.raft.proto.RaftMessage.Configuration.Builder.class);
}
public static final int SERVERS_FIELD_NUMBER = 1;
private java.util.List servers_;
/**
* repeated .raft.Server servers = 1;
*/
public java.util.List getServersList() {
return servers_;
}
/**
* repeated .raft.Server servers = 1;
*/
public java.util.List extends com.github.wenweihu86.raft.proto.RaftMessage.ServerOrBuilder>
getServersOrBuilderList() {
return servers_;
}
/**
* repeated .raft.Server servers = 1;
*/
public int getServersCount() {
return servers_.size();
}
/**
* repeated .raft.Server servers = 1;
*/
public com.github.wenweihu86.raft.proto.RaftMessage.Server getServers(int index) {
return servers_.get(index);
}
/**
* repeated .raft.Server servers = 1;
*/
public com.github.wenweihu86.raft.proto.RaftMessage.ServerOrBuilder getServersOrBuilder(
int index) {
return servers_.get(index);
}
private byte memoizedIsInitialized = -1;
public final boolean isInitialized() {
byte isInitialized = memoizedIsInitialized;
if (isInitialized == 1) return true;
if (isInitialized == 0) return false;
memoizedIsInitialized = 1;
return true;
}
public void writeTo(com.google.protobuf.CodedOutputStream output)
throws java.io.IOException {
for (int i = 0; i < servers_.size(); i++) {
output.writeMessage(1, servers_.get(i));
}
}
public int getSerializedSize() {
int size = memoizedSize;
if (size != -1) return size;
size = 0;
for (int i = 0; i < servers_.size(); i++) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(1, servers_.get(i));
}
memoizedSize = size;
return size;
}
private static final long serialVersionUID = 0L;
@java.lang.Override
public boolean equals(final java.lang.Object obj) {
if (obj == this) {
return true;
}
if (!(obj instanceof com.github.wenweihu86.raft.proto.RaftMessage.Configuration)) {
return super.equals(obj);
}
com.github.wenweihu86.raft.proto.RaftMessage.Configuration other = (com.github.wenweihu86.raft.proto.RaftMessage.Configuration) obj;
boolean result = true;
result = result && getServersList()
.equals(other.getServersList());
return result;
}
@java.lang.Override
public int hashCode() {
if (memoizedHashCode != 0) {
return memoizedHashCode;
}
int hash = 41;
hash = (19 * hash) + getDescriptorForType().hashCode();
if (getServersCount() > 0) {
hash = (37 * hash) + SERVERS_FIELD_NUMBER;
hash = (53 * hash) + getServersList().hashCode();
}
hash = (29 * hash) + unknownFields.hashCode();
memoizedHashCode = hash;
return hash;
}
public static com.github.wenweihu86.raft.proto.RaftMessage.Configuration parseFrom(
com.google.protobuf.ByteString data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static com.github.wenweihu86.raft.proto.RaftMessage.Configuration parseFrom(
com.google.protobuf.ByteString data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static com.github.wenweihu86.raft.proto.RaftMessage.Configuration parseFrom(byte[] data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static com.github.wenweihu86.raft.proto.RaftMessage.Configuration parseFrom(
byte[] data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static com.github.wenweihu86.raft.proto.RaftMessage.Configuration parseFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static com.github.wenweihu86.raft.proto.RaftMessage.Configuration parseFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
public static com.github.wenweihu86.raft.proto.RaftMessage.Configuration parseDelimitedFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input);
}
public static com.github.wenweihu86.raft.proto.RaftMessage.Configuration parseDelimitedFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input, extensionRegistry);
}
public static com.github.wenweihu86.raft.proto.RaftMessage.Configuration parseFrom(
com.google.protobuf.CodedInputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static com.github.wenweihu86.raft.proto.RaftMessage.Configuration parseFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
public Builder newBuilderForType() { return newBuilder(); }
public static Builder newBuilder() {
return DEFAULT_INSTANCE.toBuilder();
}
public static Builder newBuilder(com.github.wenweihu86.raft.proto.RaftMessage.Configuration prototype) {
return DEFAULT_INSTANCE.toBuilder().mergeFrom(prototype);
}
public Builder toBuilder() {
return this == DEFAULT_INSTANCE
? new Builder() : new Builder().mergeFrom(this);
}
@java.lang.Override
protected Builder newBuilderForType(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
Builder builder = new Builder(parent);
return builder;
}
/**
* Protobuf type {@code raft.Configuration}
*/
public static final class Builder extends
com.google.protobuf.GeneratedMessageV3.Builder implements
// @@protoc_insertion_point(builder_implements:raft.Configuration)
com.github.wenweihu86.raft.proto.RaftMessage.ConfigurationOrBuilder {
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return com.github.wenweihu86.raft.proto.RaftMessage.internal_static_raft_Configuration_descriptor;
}
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return com.github.wenweihu86.raft.proto.RaftMessage.internal_static_raft_Configuration_fieldAccessorTable
.ensureFieldAccessorsInitialized(
com.github.wenweihu86.raft.proto.RaftMessage.Configuration.class, com.github.wenweihu86.raft.proto.RaftMessage.Configuration.Builder.class);
}
// Construct using com.github.wenweihu86.raft.proto.RaftMessage.Configuration.newBuilder()
private Builder() {
maybeForceBuilderInitialization();
}
private Builder(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
super(parent);
maybeForceBuilderInitialization();
}
private void maybeForceBuilderInitialization() {
if (com.google.protobuf.GeneratedMessageV3
.alwaysUseFieldBuilders) {
getServersFieldBuilder();
}
}
public Builder clear() {
super.clear();
if (serversBuilder_ == null) {
servers_ = java.util.Collections.emptyList();
bitField0_ = (bitField0_ & ~0x00000001);
} else {
serversBuilder_.clear();
}
return this;
}
public com.google.protobuf.Descriptors.Descriptor
getDescriptorForType() {
return com.github.wenweihu86.raft.proto.RaftMessage.internal_static_raft_Configuration_descriptor;
}
public com.github.wenweihu86.raft.proto.RaftMessage.Configuration getDefaultInstanceForType() {
return com.github.wenweihu86.raft.proto.RaftMessage.Configuration.getDefaultInstance();
}
public com.github.wenweihu86.raft.proto.RaftMessage.Configuration build() {
com.github.wenweihu86.raft.proto.RaftMessage.Configuration result = buildPartial();
if (!result.isInitialized()) {
throw newUninitializedMessageException(result);
}
return result;
}
public com.github.wenweihu86.raft.proto.RaftMessage.Configuration buildPartial() {
com.github.wenweihu86.raft.proto.RaftMessage.Configuration result = new com.github.wenweihu86.raft.proto.RaftMessage.Configuration(this);
int from_bitField0_ = bitField0_;
if (serversBuilder_ == null) {
if (((bitField0_ & 0x00000001) == 0x00000001)) {
servers_ = java.util.Collections.unmodifiableList(servers_);
bitField0_ = (bitField0_ & ~0x00000001);
}
result.servers_ = servers_;
} else {
result.servers_ = serversBuilder_.build();
}
onBuilt();
return result;
}
public Builder clone() {
return (Builder) super.clone();
}
public Builder setField(
com.google.protobuf.Descriptors.FieldDescriptor field,
Object value) {
return (Builder) super.setField(field, value);
}
public Builder clearField(
com.google.protobuf.Descriptors.FieldDescriptor field) {
return (Builder) super.clearField(field);
}
public Builder clearOneof(
com.google.protobuf.Descriptors.OneofDescriptor oneof) {
return (Builder) super.clearOneof(oneof);
}
public Builder setRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
int index, Object value) {
return (Builder) super.setRepeatedField(field, index, value);
}
public Builder addRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
Object value) {
return (Builder) super.addRepeatedField(field, value);
}
public Builder mergeFrom(com.google.protobuf.Message other) {
if (other instanceof com.github.wenweihu86.raft.proto.RaftMessage.Configuration) {
return mergeFrom((com.github.wenweihu86.raft.proto.RaftMessage.Configuration)other);
} else {
super.mergeFrom(other);
return this;
}
}
public Builder mergeFrom(com.github.wenweihu86.raft.proto.RaftMessage.Configuration other) {
if (other == com.github.wenweihu86.raft.proto.RaftMessage.Configuration.getDefaultInstance()) return this;
if (serversBuilder_ == null) {
if (!other.servers_.isEmpty()) {
if (servers_.isEmpty()) {
servers_ = other.servers_;
bitField0_ = (bitField0_ & ~0x00000001);
} else {
ensureServersIsMutable();
servers_.addAll(other.servers_);
}
onChanged();
}
} else {
if (!other.servers_.isEmpty()) {
if (serversBuilder_.isEmpty()) {
serversBuilder_.dispose();
serversBuilder_ = null;
servers_ = other.servers_;
bitField0_ = (bitField0_ & ~0x00000001);
serversBuilder_ =
com.google.protobuf.GeneratedMessageV3.alwaysUseFieldBuilders ?
getServersFieldBuilder() : null;
} else {
serversBuilder_.addAllMessages(other.servers_);
}
}
}
onChanged();
return this;
}
public final boolean isInitialized() {
return true;
}
public Builder mergeFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
com.github.wenweihu86.raft.proto.RaftMessage.Configuration parsedMessage = null;
try {
parsedMessage = PARSER.parsePartialFrom(input, extensionRegistry);
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
parsedMessage = (com.github.wenweihu86.raft.proto.RaftMessage.Configuration) e.getUnfinishedMessage();
throw e.unwrapIOException();
} finally {
if (parsedMessage != null) {
mergeFrom(parsedMessage);
}
}
return this;
}
private int bitField0_;
private java.util.List servers_ =
java.util.Collections.emptyList();
private void ensureServersIsMutable() {
if (!((bitField0_ & 0x00000001) == 0x00000001)) {
servers_ = new java.util.ArrayList(servers_);
bitField0_ |= 0x00000001;
}
}
private com.google.protobuf.RepeatedFieldBuilderV3<
com.github.wenweihu86.raft.proto.RaftMessage.Server, com.github.wenweihu86.raft.proto.RaftMessage.Server.Builder, com.github.wenweihu86.raft.proto.RaftMessage.ServerOrBuilder> serversBuilder_;
/**
* repeated .raft.Server servers = 1;
*/
public java.util.List getServersList() {
if (serversBuilder_ == null) {
return java.util.Collections.unmodifiableList(servers_);
} else {
return serversBuilder_.getMessageList();
}
}
/**
* repeated .raft.Server servers = 1;
*/
public int getServersCount() {
if (serversBuilder_ == null) {
return servers_.size();
} else {
return serversBuilder_.getCount();
}
}
/**
* repeated .raft.Server servers = 1;
*/
public com.github.wenweihu86.raft.proto.RaftMessage.Server getServers(int index) {
if (serversBuilder_ == null) {
return servers_.get(index);
} else {
return serversBuilder_.getMessage(index);
}
}
/**
* repeated .raft.Server servers = 1;
*/
public Builder setServers(
int index, com.github.wenweihu86.raft.proto.RaftMessage.Server value) {
if (serversBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
ensureServersIsMutable();
servers_.set(index, value);
onChanged();
} else {
serversBuilder_.setMessage(index, value);
}
return this;
}
/**
* repeated .raft.Server servers = 1;
*/
public Builder setServers(
int index, com.github.wenweihu86.raft.proto.RaftMessage.Server.Builder builderForValue) {
if (serversBuilder_ == null) {
ensureServersIsMutable();
servers_.set(index, builderForValue.build());
onChanged();
} else {
serversBuilder_.setMessage(index, builderForValue.build());
}
return this;
}
/**
* repeated .raft.Server servers = 1;
*/
public Builder addServers(com.github.wenweihu86.raft.proto.RaftMessage.Server value) {
if (serversBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
ensureServersIsMutable();
servers_.add(value);
onChanged();
} else {
serversBuilder_.addMessage(value);
}
return this;
}
/**
* repeated .raft.Server servers = 1;
*/
public Builder addServers(
int index, com.github.wenweihu86.raft.proto.RaftMessage.Server value) {
if (serversBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
ensureServersIsMutable();
servers_.add(index, value);
onChanged();
} else {
serversBuilder_.addMessage(index, value);
}
return this;
}
/**
* repeated .raft.Server servers = 1;
*/
public Builder addServers(
com.github.wenweihu86.raft.proto.RaftMessage.Server.Builder builderForValue) {
if (serversBuilder_ == null) {
ensureServersIsMutable();
servers_.add(builderForValue.build());
onChanged();
} else {
serversBuilder_.addMessage(builderForValue.build());
}
return this;
}
/**
* repeated .raft.Server servers = 1;
*/
public Builder addServers(
int index, com.github.wenweihu86.raft.proto.RaftMessage.Server.Builder builderForValue) {
if (serversBuilder_ == null) {
ensureServersIsMutable();
servers_.add(index, builderForValue.build());
onChanged();
} else {
serversBuilder_.addMessage(index, builderForValue.build());
}
return this;
}
/**
* repeated .raft.Server servers = 1;
*/
public Builder addAllServers(
java.lang.Iterable extends com.github.wenweihu86.raft.proto.RaftMessage.Server> values) {
if (serversBuilder_ == null) {
ensureServersIsMutable();
com.google.protobuf.AbstractMessageLite.Builder.addAll(
values, servers_);
onChanged();
} else {
serversBuilder_.addAllMessages(values);
}
return this;
}
/**
* repeated .raft.Server servers = 1;
*/
public Builder clearServers() {
if (serversBuilder_ == null) {
servers_ = java.util.Collections.emptyList();
bitField0_ = (bitField0_ & ~0x00000001);
onChanged();
} else {
serversBuilder_.clear();
}
return this;
}
/**
* repeated .raft.Server servers = 1;
*/
public Builder removeServers(int index) {
if (serversBuilder_ == null) {
ensureServersIsMutable();
servers_.remove(index);
onChanged();
} else {
serversBuilder_.remove(index);
}
return this;
}
/**
* repeated .raft.Server servers = 1;
*/
public com.github.wenweihu86.raft.proto.RaftMessage.Server.Builder getServersBuilder(
int index) {
return getServersFieldBuilder().getBuilder(index);
}
/**
* repeated .raft.Server servers = 1;
*/
public com.github.wenweihu86.raft.proto.RaftMessage.ServerOrBuilder getServersOrBuilder(
int index) {
if (serversBuilder_ == null) {
return servers_.get(index); } else {
return serversBuilder_.getMessageOrBuilder(index);
}
}
/**
* repeated .raft.Server servers = 1;
*/
public java.util.List extends com.github.wenweihu86.raft.proto.RaftMessage.ServerOrBuilder>
getServersOrBuilderList() {
if (serversBuilder_ != null) {
return serversBuilder_.getMessageOrBuilderList();
} else {
return java.util.Collections.unmodifiableList(servers_);
}
}
/**
* repeated .raft.Server servers = 1;
*/
public com.github.wenweihu86.raft.proto.RaftMessage.Server.Builder addServersBuilder() {
return getServersFieldBuilder().addBuilder(
com.github.wenweihu86.raft.proto.RaftMessage.Server.getDefaultInstance());
}
/**
* repeated .raft.Server servers = 1;
*/
public com.github.wenweihu86.raft.proto.RaftMessage.Server.Builder addServersBuilder(
int index) {
return getServersFieldBuilder().addBuilder(
index, com.github.wenweihu86.raft.proto.RaftMessage.Server.getDefaultInstance());
}
/**
* repeated .raft.Server servers = 1;
*/
public java.util.List
getServersBuilderList() {
return getServersFieldBuilder().getBuilderList();
}
private com.google.protobuf.RepeatedFieldBuilderV3<
com.github.wenweihu86.raft.proto.RaftMessage.Server, com.github.wenweihu86.raft.proto.RaftMessage.Server.Builder, com.github.wenweihu86.raft.proto.RaftMessage.ServerOrBuilder>
getServersFieldBuilder() {
if (serversBuilder_ == null) {
serversBuilder_ = new com.google.protobuf.RepeatedFieldBuilderV3<
com.github.wenweihu86.raft.proto.RaftMessage.Server, com.github.wenweihu86.raft.proto.RaftMessage.Server.Builder, com.github.wenweihu86.raft.proto.RaftMessage.ServerOrBuilder>(
servers_,
((bitField0_ & 0x00000001) == 0x00000001),
getParentForChildren(),
isClean());
servers_ = null;
}
return serversBuilder_;
}
public final Builder setUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return this;
}
public final Builder mergeUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return this;
}
// @@protoc_insertion_point(builder_scope:raft.Configuration)
}
// @@protoc_insertion_point(class_scope:raft.Configuration)
private static final com.github.wenweihu86.raft.proto.RaftMessage.Configuration DEFAULT_INSTANCE;
static {
DEFAULT_INSTANCE = new com.github.wenweihu86.raft.proto.RaftMessage.Configuration();
}
public static com.github.wenweihu86.raft.proto.RaftMessage.Configuration getDefaultInstance() {
return DEFAULT_INSTANCE;
}
private static final com.google.protobuf.Parser
PARSER = new com.google.protobuf.AbstractParser() {
public Configuration parsePartialFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return new Configuration(input, extensionRegistry);
}
};
public static com.google.protobuf.Parser parser() {
return PARSER;
}
@java.lang.Override
public com.google.protobuf.Parser getParserForType() {
return PARSER;
}
public com.github.wenweihu86.raft.proto.RaftMessage.Configuration getDefaultInstanceForType() {
return DEFAULT_INSTANCE;
}
}
public interface LogMetaDataOrBuilder extends
// @@protoc_insertion_point(interface_extends:raft.LogMetaData)
com.google.protobuf.MessageOrBuilder {
/**
* optional uint64 current_term = 1;
*/
long getCurrentTerm();
/**
* optional uint32 voted_for = 2;
*/
int getVotedFor();
/**
* optional uint64 first_log_index = 3;
*/
long getFirstLogIndex();
}
/**
* Protobuf type {@code raft.LogMetaData}
*/
public static final class LogMetaData extends
com.google.protobuf.GeneratedMessageV3 implements
// @@protoc_insertion_point(message_implements:raft.LogMetaData)
LogMetaDataOrBuilder {
// Use LogMetaData.newBuilder() to construct.
private LogMetaData(com.google.protobuf.GeneratedMessageV3.Builder> builder) {
super(builder);
}
private LogMetaData() {
currentTerm_ = 0L;
votedFor_ = 0;
firstLogIndex_ = 0L;
}
@java.lang.Override
public final com.google.protobuf.UnknownFieldSet
getUnknownFields() {
return com.google.protobuf.UnknownFieldSet.getDefaultInstance();
}
private LogMetaData(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
this();
int mutable_bitField0_ = 0;
try {
boolean done = false;
while (!done) {
int tag = input.readTag();
switch (tag) {
case 0:
done = true;
break;
default: {
if (!input.skipField(tag)) {
done = true;
}
break;
}
case 8: {
currentTerm_ = input.readUInt64();
break;
}
case 16: {
votedFor_ = input.readUInt32();
break;
}
case 24: {
firstLogIndex_ = input.readUInt64();
break;
}
}
}
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.setUnfinishedMessage(this);
} catch (java.io.IOException e) {
throw new com.google.protobuf.InvalidProtocolBufferException(
e).setUnfinishedMessage(this);
} finally {
makeExtensionsImmutable();
}
}
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return com.github.wenweihu86.raft.proto.RaftMessage.internal_static_raft_LogMetaData_descriptor;
}
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return com.github.wenweihu86.raft.proto.RaftMessage.internal_static_raft_LogMetaData_fieldAccessorTable
.ensureFieldAccessorsInitialized(
com.github.wenweihu86.raft.proto.RaftMessage.LogMetaData.class, com.github.wenweihu86.raft.proto.RaftMessage.LogMetaData.Builder.class);
}
public static final int CURRENT_TERM_FIELD_NUMBER = 1;
private long currentTerm_;
/**
* optional uint64 current_term = 1;
*/
public long getCurrentTerm() {
return currentTerm_;
}
public static final int VOTED_FOR_FIELD_NUMBER = 2;
private int votedFor_;
/**
* optional uint32 voted_for = 2;
*/
public int getVotedFor() {
return votedFor_;
}
public static final int FIRST_LOG_INDEX_FIELD_NUMBER = 3;
private long firstLogIndex_;
/**
* optional uint64 first_log_index = 3;
*/
public long getFirstLogIndex() {
return firstLogIndex_;
}
private byte memoizedIsInitialized = -1;
public final boolean isInitialized() {
byte isInitialized = memoizedIsInitialized;
if (isInitialized == 1) return true;
if (isInitialized == 0) return false;
memoizedIsInitialized = 1;
return true;
}
public void writeTo(com.google.protobuf.CodedOutputStream output)
throws java.io.IOException {
if (currentTerm_ != 0L) {
output.writeUInt64(1, currentTerm_);
}
if (votedFor_ != 0) {
output.writeUInt32(2, votedFor_);
}
if (firstLogIndex_ != 0L) {
output.writeUInt64(3, firstLogIndex_);
}
}
public int getSerializedSize() {
int size = memoizedSize;
if (size != -1) return size;
size = 0;
if (currentTerm_ != 0L) {
size += com.google.protobuf.CodedOutputStream
.computeUInt64Size(1, currentTerm_);
}
if (votedFor_ != 0) {
size += com.google.protobuf.CodedOutputStream
.computeUInt32Size(2, votedFor_);
}
if (firstLogIndex_ != 0L) {
size += com.google.protobuf.CodedOutputStream
.computeUInt64Size(3, firstLogIndex_);
}
memoizedSize = size;
return size;
}
private static final long serialVersionUID = 0L;
@java.lang.Override
public boolean equals(final java.lang.Object obj) {
if (obj == this) {
return true;
}
if (!(obj instanceof com.github.wenweihu86.raft.proto.RaftMessage.LogMetaData)) {
return super.equals(obj);
}
com.github.wenweihu86.raft.proto.RaftMessage.LogMetaData other = (com.github.wenweihu86.raft.proto.RaftMessage.LogMetaData) obj;
boolean result = true;
result = result && (getCurrentTerm()
== other.getCurrentTerm());
result = result && (getVotedFor()
== other.getVotedFor());
result = result && (getFirstLogIndex()
== other.getFirstLogIndex());
return result;
}
@java.lang.Override
public int hashCode() {
if (memoizedHashCode != 0) {
return memoizedHashCode;
}
int hash = 41;
hash = (19 * hash) + getDescriptorForType().hashCode();
hash = (37 * hash) + CURRENT_TERM_FIELD_NUMBER;
hash = (53 * hash) + com.google.protobuf.Internal.hashLong(
getCurrentTerm());
hash = (37 * hash) + VOTED_FOR_FIELD_NUMBER;
hash = (53 * hash) + getVotedFor();
hash = (37 * hash) + FIRST_LOG_INDEX_FIELD_NUMBER;
hash = (53 * hash) + com.google.protobuf.Internal.hashLong(
getFirstLogIndex());
hash = (29 * hash) + unknownFields.hashCode();
memoizedHashCode = hash;
return hash;
}
public static com.github.wenweihu86.raft.proto.RaftMessage.LogMetaData parseFrom(
com.google.protobuf.ByteString data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static com.github.wenweihu86.raft.proto.RaftMessage.LogMetaData parseFrom(
com.google.protobuf.ByteString data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static com.github.wenweihu86.raft.proto.RaftMessage.LogMetaData parseFrom(byte[] data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static com.github.wenweihu86.raft.proto.RaftMessage.LogMetaData parseFrom(
byte[] data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static com.github.wenweihu86.raft.proto.RaftMessage.LogMetaData parseFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static com.github.wenweihu86.raft.proto.RaftMessage.LogMetaData parseFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
public static com.github.wenweihu86.raft.proto.RaftMessage.LogMetaData parseDelimitedFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input);
}
public static com.github.wenweihu86.raft.proto.RaftMessage.LogMetaData parseDelimitedFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input, extensionRegistry);
}
public static com.github.wenweihu86.raft.proto.RaftMessage.LogMetaData parseFrom(
com.google.protobuf.CodedInputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static com.github.wenweihu86.raft.proto.RaftMessage.LogMetaData parseFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
public Builder newBuilderForType() { return newBuilder(); }
public static Builder newBuilder() {
return DEFAULT_INSTANCE.toBuilder();
}
public static Builder newBuilder(com.github.wenweihu86.raft.proto.RaftMessage.LogMetaData prototype) {
return DEFAULT_INSTANCE.toBuilder().mergeFrom(prototype);
}
public Builder toBuilder() {
return this == DEFAULT_INSTANCE
? new Builder() : new Builder().mergeFrom(this);
}
@java.lang.Override
protected Builder newBuilderForType(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
Builder builder = new Builder(parent);
return builder;
}
/**
* Protobuf type {@code raft.LogMetaData}
*/
public static final class Builder extends
com.google.protobuf.GeneratedMessageV3.Builder implements
// @@protoc_insertion_point(builder_implements:raft.LogMetaData)
com.github.wenweihu86.raft.proto.RaftMessage.LogMetaDataOrBuilder {
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return com.github.wenweihu86.raft.proto.RaftMessage.internal_static_raft_LogMetaData_descriptor;
}
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return com.github.wenweihu86.raft.proto.RaftMessage.internal_static_raft_LogMetaData_fieldAccessorTable
.ensureFieldAccessorsInitialized(
com.github.wenweihu86.raft.proto.RaftMessage.LogMetaData.class, com.github.wenweihu86.raft.proto.RaftMessage.LogMetaData.Builder.class);
}
// Construct using com.github.wenweihu86.raft.proto.RaftMessage.LogMetaData.newBuilder()
private Builder() {
maybeForceBuilderInitialization();
}
private Builder(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
super(parent);
maybeForceBuilderInitialization();
}
private void maybeForceBuilderInitialization() {
if (com.google.protobuf.GeneratedMessageV3
.alwaysUseFieldBuilders) {
}
}
public Builder clear() {
super.clear();
currentTerm_ = 0L;
votedFor_ = 0;
firstLogIndex_ = 0L;
return this;
}
public com.google.protobuf.Descriptors.Descriptor
getDescriptorForType() {
return com.github.wenweihu86.raft.proto.RaftMessage.internal_static_raft_LogMetaData_descriptor;
}
public com.github.wenweihu86.raft.proto.RaftMessage.LogMetaData getDefaultInstanceForType() {
return com.github.wenweihu86.raft.proto.RaftMessage.LogMetaData.getDefaultInstance();
}
public com.github.wenweihu86.raft.proto.RaftMessage.LogMetaData build() {
com.github.wenweihu86.raft.proto.RaftMessage.LogMetaData result = buildPartial();
if (!result.isInitialized()) {
throw newUninitializedMessageException(result);
}
return result;
}
public com.github.wenweihu86.raft.proto.RaftMessage.LogMetaData buildPartial() {
com.github.wenweihu86.raft.proto.RaftMessage.LogMetaData result = new com.github.wenweihu86.raft.proto.RaftMessage.LogMetaData(this);
result.currentTerm_ = currentTerm_;
result.votedFor_ = votedFor_;
result.firstLogIndex_ = firstLogIndex_;
onBuilt();
return result;
}
public Builder clone() {
return (Builder) super.clone();
}
public Builder setField(
com.google.protobuf.Descriptors.FieldDescriptor field,
Object value) {
return (Builder) super.setField(field, value);
}
public Builder clearField(
com.google.protobuf.Descriptors.FieldDescriptor field) {
return (Builder) super.clearField(field);
}
public Builder clearOneof(
com.google.protobuf.Descriptors.OneofDescriptor oneof) {
return (Builder) super.clearOneof(oneof);
}
public Builder setRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
int index, Object value) {
return (Builder) super.setRepeatedField(field, index, value);
}
public Builder addRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
Object value) {
return (Builder) super.addRepeatedField(field, value);
}
public Builder mergeFrom(com.google.protobuf.Message other) {
if (other instanceof com.github.wenweihu86.raft.proto.RaftMessage.LogMetaData) {
return mergeFrom((com.github.wenweihu86.raft.proto.RaftMessage.LogMetaData)other);
} else {
super.mergeFrom(other);
return this;
}
}
public Builder mergeFrom(com.github.wenweihu86.raft.proto.RaftMessage.LogMetaData other) {
if (other == com.github.wenweihu86.raft.proto.RaftMessage.LogMetaData.getDefaultInstance()) return this;
if (other.getCurrentTerm() != 0L) {
setCurrentTerm(other.getCurrentTerm());
}
if (other.getVotedFor() != 0) {
setVotedFor(other.getVotedFor());
}
if (other.getFirstLogIndex() != 0L) {
setFirstLogIndex(other.getFirstLogIndex());
}
onChanged();
return this;
}
public final boolean isInitialized() {
return true;
}
public Builder mergeFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
com.github.wenweihu86.raft.proto.RaftMessage.LogMetaData parsedMessage = null;
try {
parsedMessage = PARSER.parsePartialFrom(input, extensionRegistry);
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
parsedMessage = (com.github.wenweihu86.raft.proto.RaftMessage.LogMetaData) e.getUnfinishedMessage();
throw e.unwrapIOException();
} finally {
if (parsedMessage != null) {
mergeFrom(parsedMessage);
}
}
return this;
}
private long currentTerm_ ;
/**
* optional uint64 current_term = 1;
*/
public long getCurrentTerm() {
return currentTerm_;
}
/**
* optional uint64 current_term = 1;
*/
public Builder setCurrentTerm(long value) {
currentTerm_ = value;
onChanged();
return this;
}
/**
* optional uint64 current_term = 1;
*/
public Builder clearCurrentTerm() {
currentTerm_ = 0L;
onChanged();
return this;
}
private int votedFor_ ;
/**
* optional uint32 voted_for = 2;
*/
public int getVotedFor() {
return votedFor_;
}
/**
* optional uint32 voted_for = 2;
*/
public Builder setVotedFor(int value) {
votedFor_ = value;
onChanged();
return this;
}
/**
* optional uint32 voted_for = 2;
*/
public Builder clearVotedFor() {
votedFor_ = 0;
onChanged();
return this;
}
private long firstLogIndex_ ;
/**
* optional uint64 first_log_index = 3;
*/
public long getFirstLogIndex() {
return firstLogIndex_;
}
/**
* optional uint64 first_log_index = 3;
*/
public Builder setFirstLogIndex(long value) {
firstLogIndex_ = value;
onChanged();
return this;
}
/**
* optional uint64 first_log_index = 3;
*/
public Builder clearFirstLogIndex() {
firstLogIndex_ = 0L;
onChanged();
return this;
}
public final Builder setUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return this;
}
public final Builder mergeUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return this;
}
// @@protoc_insertion_point(builder_scope:raft.LogMetaData)
}
// @@protoc_insertion_point(class_scope:raft.LogMetaData)
private static final com.github.wenweihu86.raft.proto.RaftMessage.LogMetaData DEFAULT_INSTANCE;
static {
DEFAULT_INSTANCE = new com.github.wenweihu86.raft.proto.RaftMessage.LogMetaData();
}
public static com.github.wenweihu86.raft.proto.RaftMessage.LogMetaData getDefaultInstance() {
return DEFAULT_INSTANCE;
}
private static final com.google.protobuf.Parser
PARSER = new com.google.protobuf.AbstractParser() {
public LogMetaData parsePartialFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return new LogMetaData(input, extensionRegistry);
}
};
public static com.google.protobuf.Parser parser() {
return PARSER;
}
@java.lang.Override
public com.google.protobuf.Parser getParserForType() {
return PARSER;
}
public com.github.wenweihu86.raft.proto.RaftMessage.LogMetaData getDefaultInstanceForType() {
return DEFAULT_INSTANCE;
}
}
public interface SnapshotMetaDataOrBuilder extends
// @@protoc_insertion_point(interface_extends:raft.SnapshotMetaData)
com.google.protobuf.MessageOrBuilder {
/**
* optional uint64 last_included_index = 1;
*/
long getLastIncludedIndex();
/**
* optional uint64 last_included_term = 2;
*/
long getLastIncludedTerm();
/**
* optional .raft.Configuration configuration = 3;
*/
boolean hasConfiguration();
/**
* optional .raft.Configuration configuration = 3;
*/
com.github.wenweihu86.raft.proto.RaftMessage.Configuration getConfiguration();
/**
* optional .raft.Configuration configuration = 3;
*/
com.github.wenweihu86.raft.proto.RaftMessage.ConfigurationOrBuilder getConfigurationOrBuilder();
}
/**
* Protobuf type {@code raft.SnapshotMetaData}
*/
public static final class SnapshotMetaData extends
com.google.protobuf.GeneratedMessageV3 implements
// @@protoc_insertion_point(message_implements:raft.SnapshotMetaData)
SnapshotMetaDataOrBuilder {
// Use SnapshotMetaData.newBuilder() to construct.
private SnapshotMetaData(com.google.protobuf.GeneratedMessageV3.Builder> builder) {
super(builder);
}
private SnapshotMetaData() {
lastIncludedIndex_ = 0L;
lastIncludedTerm_ = 0L;
}
@java.lang.Override
public final com.google.protobuf.UnknownFieldSet
getUnknownFields() {
return com.google.protobuf.UnknownFieldSet.getDefaultInstance();
}
private SnapshotMetaData(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
this();
int mutable_bitField0_ = 0;
try {
boolean done = false;
while (!done) {
int tag = input.readTag();
switch (tag) {
case 0:
done = true;
break;
default: {
if (!input.skipField(tag)) {
done = true;
}
break;
}
case 8: {
lastIncludedIndex_ = input.readUInt64();
break;
}
case 16: {
lastIncludedTerm_ = input.readUInt64();
break;
}
case 26: {
com.github.wenweihu86.raft.proto.RaftMessage.Configuration.Builder subBuilder = null;
if (configuration_ != null) {
subBuilder = configuration_.toBuilder();
}
configuration_ = input.readMessage(com.github.wenweihu86.raft.proto.RaftMessage.Configuration.parser(), extensionRegistry);
if (subBuilder != null) {
subBuilder.mergeFrom(configuration_);
configuration_ = subBuilder.buildPartial();
}
break;
}
}
}
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.setUnfinishedMessage(this);
} catch (java.io.IOException e) {
throw new com.google.protobuf.InvalidProtocolBufferException(
e).setUnfinishedMessage(this);
} finally {
makeExtensionsImmutable();
}
}
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return com.github.wenweihu86.raft.proto.RaftMessage.internal_static_raft_SnapshotMetaData_descriptor;
}
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return com.github.wenweihu86.raft.proto.RaftMessage.internal_static_raft_SnapshotMetaData_fieldAccessorTable
.ensureFieldAccessorsInitialized(
com.github.wenweihu86.raft.proto.RaftMessage.SnapshotMetaData.class, com.github.wenweihu86.raft.proto.RaftMessage.SnapshotMetaData.Builder.class);
}
public static final int LAST_INCLUDED_INDEX_FIELD_NUMBER = 1;
private long lastIncludedIndex_;
/**
* optional uint64 last_included_index = 1;
*/
public long getLastIncludedIndex() {
return lastIncludedIndex_;
}
public static final int LAST_INCLUDED_TERM_FIELD_NUMBER = 2;
private long lastIncludedTerm_;
/**
* optional uint64 last_included_term = 2;
*/
public long getLastIncludedTerm() {
return lastIncludedTerm_;
}
public static final int CONFIGURATION_FIELD_NUMBER = 3;
private com.github.wenweihu86.raft.proto.RaftMessage.Configuration configuration_;
/**
* optional .raft.Configuration configuration = 3;
*/
public boolean hasConfiguration() {
return configuration_ != null;
}
/**
* optional .raft.Configuration configuration = 3;
*/
public com.github.wenweihu86.raft.proto.RaftMessage.Configuration getConfiguration() {
return configuration_ == null ? com.github.wenweihu86.raft.proto.RaftMessage.Configuration.getDefaultInstance() : configuration_;
}
/**
* optional .raft.Configuration configuration = 3;
*/
public com.github.wenweihu86.raft.proto.RaftMessage.ConfigurationOrBuilder getConfigurationOrBuilder() {
return getConfiguration();
}
private byte memoizedIsInitialized = -1;
public final boolean isInitialized() {
byte isInitialized = memoizedIsInitialized;
if (isInitialized == 1) return true;
if (isInitialized == 0) return false;
memoizedIsInitialized = 1;
return true;
}
public void writeTo(com.google.protobuf.CodedOutputStream output)
throws java.io.IOException {
if (lastIncludedIndex_ != 0L) {
output.writeUInt64(1, lastIncludedIndex_);
}
if (lastIncludedTerm_ != 0L) {
output.writeUInt64(2, lastIncludedTerm_);
}
if (configuration_ != null) {
output.writeMessage(3, getConfiguration());
}
}
public int getSerializedSize() {
int size = memoizedSize;
if (size != -1) return size;
size = 0;
if (lastIncludedIndex_ != 0L) {
size += com.google.protobuf.CodedOutputStream
.computeUInt64Size(1, lastIncludedIndex_);
}
if (lastIncludedTerm_ != 0L) {
size += com.google.protobuf.CodedOutputStream
.computeUInt64Size(2, lastIncludedTerm_);
}
if (configuration_ != null) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(3, getConfiguration());
}
memoizedSize = size;
return size;
}
private static final long serialVersionUID = 0L;
@java.lang.Override
public boolean equals(final java.lang.Object obj) {
if (obj == this) {
return true;
}
if (!(obj instanceof com.github.wenweihu86.raft.proto.RaftMessage.SnapshotMetaData)) {
return super.equals(obj);
}
com.github.wenweihu86.raft.proto.RaftMessage.SnapshotMetaData other = (com.github.wenweihu86.raft.proto.RaftMessage.SnapshotMetaData) obj;
boolean result = true;
result = result && (getLastIncludedIndex()
== other.getLastIncludedIndex());
result = result && (getLastIncludedTerm()
== other.getLastIncludedTerm());
result = result && (hasConfiguration() == other.hasConfiguration());
if (hasConfiguration()) {
result = result && getConfiguration()
.equals(other.getConfiguration());
}
return result;
}
@java.lang.Override
public int hashCode() {
if (memoizedHashCode != 0) {
return memoizedHashCode;
}
int hash = 41;
hash = (19 * hash) + getDescriptorForType().hashCode();
hash = (37 * hash) + LAST_INCLUDED_INDEX_FIELD_NUMBER;
hash = (53 * hash) + com.google.protobuf.Internal.hashLong(
getLastIncludedIndex());
hash = (37 * hash) + LAST_INCLUDED_TERM_FIELD_NUMBER;
hash = (53 * hash) + com.google.protobuf.Internal.hashLong(
getLastIncludedTerm());
if (hasConfiguration()) {
hash = (37 * hash) + CONFIGURATION_FIELD_NUMBER;
hash = (53 * hash) + getConfiguration().hashCode();
}
hash = (29 * hash) + unknownFields.hashCode();
memoizedHashCode = hash;
return hash;
}
public static com.github.wenweihu86.raft.proto.RaftMessage.SnapshotMetaData parseFrom(
com.google.protobuf.ByteString data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static com.github.wenweihu86.raft.proto.RaftMessage.SnapshotMetaData parseFrom(
com.google.protobuf.ByteString data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static com.github.wenweihu86.raft.proto.RaftMessage.SnapshotMetaData parseFrom(byte[] data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static com.github.wenweihu86.raft.proto.RaftMessage.SnapshotMetaData parseFrom(
byte[] data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static com.github.wenweihu86.raft.proto.RaftMessage.SnapshotMetaData parseFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static com.github.wenweihu86.raft.proto.RaftMessage.SnapshotMetaData parseFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
public static com.github.wenweihu86.raft.proto.RaftMessage.SnapshotMetaData parseDelimitedFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input);
}
public static com.github.wenweihu86.raft.proto.RaftMessage.SnapshotMetaData parseDelimitedFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input, extensionRegistry);
}
public static com.github.wenweihu86.raft.proto.RaftMessage.SnapshotMetaData parseFrom(
com.google.protobuf.CodedInputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static com.github.wenweihu86.raft.proto.RaftMessage.SnapshotMetaData parseFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
public Builder newBuilderForType() { return newBuilder(); }
public static Builder newBuilder() {
return DEFAULT_INSTANCE.toBuilder();
}
public static Builder newBuilder(com.github.wenweihu86.raft.proto.RaftMessage.SnapshotMetaData prototype) {
return DEFAULT_INSTANCE.toBuilder().mergeFrom(prototype);
}
public Builder toBuilder() {
return this == DEFAULT_INSTANCE
? new Builder() : new Builder().mergeFrom(this);
}
@java.lang.Override
protected Builder newBuilderForType(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
Builder builder = new Builder(parent);
return builder;
}
/**
* Protobuf type {@code raft.SnapshotMetaData}
*/
public static final class Builder extends
com.google.protobuf.GeneratedMessageV3.Builder implements
// @@protoc_insertion_point(builder_implements:raft.SnapshotMetaData)
com.github.wenweihu86.raft.proto.RaftMessage.SnapshotMetaDataOrBuilder {
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return com.github.wenweihu86.raft.proto.RaftMessage.internal_static_raft_SnapshotMetaData_descriptor;
}
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return com.github.wenweihu86.raft.proto.RaftMessage.internal_static_raft_SnapshotMetaData_fieldAccessorTable
.ensureFieldAccessorsInitialized(
com.github.wenweihu86.raft.proto.RaftMessage.SnapshotMetaData.class, com.github.wenweihu86.raft.proto.RaftMessage.SnapshotMetaData.Builder.class);
}
// Construct using com.github.wenweihu86.raft.proto.RaftMessage.SnapshotMetaData.newBuilder()
private Builder() {
maybeForceBuilderInitialization();
}
private Builder(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
super(parent);
maybeForceBuilderInitialization();
}
private void maybeForceBuilderInitialization() {
if (com.google.protobuf.GeneratedMessageV3
.alwaysUseFieldBuilders) {
}
}
public Builder clear() {
super.clear();
lastIncludedIndex_ = 0L;
lastIncludedTerm_ = 0L;
if (configurationBuilder_ == null) {
configuration_ = null;
} else {
configuration_ = null;
configurationBuilder_ = null;
}
return this;
}
public com.google.protobuf.Descriptors.Descriptor
getDescriptorForType() {
return com.github.wenweihu86.raft.proto.RaftMessage.internal_static_raft_SnapshotMetaData_descriptor;
}
public com.github.wenweihu86.raft.proto.RaftMessage.SnapshotMetaData getDefaultInstanceForType() {
return com.github.wenweihu86.raft.proto.RaftMessage.SnapshotMetaData.getDefaultInstance();
}
public com.github.wenweihu86.raft.proto.RaftMessage.SnapshotMetaData build() {
com.github.wenweihu86.raft.proto.RaftMessage.SnapshotMetaData result = buildPartial();
if (!result.isInitialized()) {
throw newUninitializedMessageException(result);
}
return result;
}
public com.github.wenweihu86.raft.proto.RaftMessage.SnapshotMetaData buildPartial() {
com.github.wenweihu86.raft.proto.RaftMessage.SnapshotMetaData result = new com.github.wenweihu86.raft.proto.RaftMessage.SnapshotMetaData(this);
result.lastIncludedIndex_ = lastIncludedIndex_;
result.lastIncludedTerm_ = lastIncludedTerm_;
if (configurationBuilder_ == null) {
result.configuration_ = configuration_;
} else {
result.configuration_ = configurationBuilder_.build();
}
onBuilt();
return result;
}
public Builder clone() {
return (Builder) super.clone();
}
public Builder setField(
com.google.protobuf.Descriptors.FieldDescriptor field,
Object value) {
return (Builder) super.setField(field, value);
}
public Builder clearField(
com.google.protobuf.Descriptors.FieldDescriptor field) {
return (Builder) super.clearField(field);
}
public Builder clearOneof(
com.google.protobuf.Descriptors.OneofDescriptor oneof) {
return (Builder) super.clearOneof(oneof);
}
public Builder setRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
int index, Object value) {
return (Builder) super.setRepeatedField(field, index, value);
}
public Builder addRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
Object value) {
return (Builder) super.addRepeatedField(field, value);
}
public Builder mergeFrom(com.google.protobuf.Message other) {
if (other instanceof com.github.wenweihu86.raft.proto.RaftMessage.SnapshotMetaData) {
return mergeFrom((com.github.wenweihu86.raft.proto.RaftMessage.SnapshotMetaData)other);
} else {
super.mergeFrom(other);
return this;
}
}
public Builder mergeFrom(com.github.wenweihu86.raft.proto.RaftMessage.SnapshotMetaData other) {
if (other == com.github.wenweihu86.raft.proto.RaftMessage.SnapshotMetaData.getDefaultInstance()) return this;
if (other.getLastIncludedIndex() != 0L) {
setLastIncludedIndex(other.getLastIncludedIndex());
}
if (other.getLastIncludedTerm() != 0L) {
setLastIncludedTerm(other.getLastIncludedTerm());
}
if (other.hasConfiguration()) {
mergeConfiguration(other.getConfiguration());
}
onChanged();
return this;
}
public final boolean isInitialized() {
return true;
}
public Builder mergeFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
com.github.wenweihu86.raft.proto.RaftMessage.SnapshotMetaData parsedMessage = null;
try {
parsedMessage = PARSER.parsePartialFrom(input, extensionRegistry);
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
parsedMessage = (com.github.wenweihu86.raft.proto.RaftMessage.SnapshotMetaData) e.getUnfinishedMessage();
throw e.unwrapIOException();
} finally {
if (parsedMessage != null) {
mergeFrom(parsedMessage);
}
}
return this;
}
private long lastIncludedIndex_ ;
/**
* optional uint64 last_included_index = 1;
*/
public long getLastIncludedIndex() {
return lastIncludedIndex_;
}
/**
* optional uint64 last_included_index = 1;
*/
public Builder setLastIncludedIndex(long value) {
lastIncludedIndex_ = value;
onChanged();
return this;
}
/**
* optional uint64 last_included_index = 1;
*/
public Builder clearLastIncludedIndex() {
lastIncludedIndex_ = 0L;
onChanged();
return this;
}
private long lastIncludedTerm_ ;
/**
* optional uint64 last_included_term = 2;
*/
public long getLastIncludedTerm() {
return lastIncludedTerm_;
}
/**
* optional uint64 last_included_term = 2;
*/
public Builder setLastIncludedTerm(long value) {
lastIncludedTerm_ = value;
onChanged();
return this;
}
/**
* optional uint64 last_included_term = 2;
*/
public Builder clearLastIncludedTerm() {
lastIncludedTerm_ = 0L;
onChanged();
return this;
}
private com.github.wenweihu86.raft.proto.RaftMessage.Configuration configuration_ = null;
private com.google.protobuf.SingleFieldBuilderV3<
com.github.wenweihu86.raft.proto.RaftMessage.Configuration, com.github.wenweihu86.raft.proto.RaftMessage.Configuration.Builder, com.github.wenweihu86.raft.proto.RaftMessage.ConfigurationOrBuilder> configurationBuilder_;
/**
* optional .raft.Configuration configuration = 3;
*/
public boolean hasConfiguration() {
return configurationBuilder_ != null || configuration_ != null;
}
/**
* optional .raft.Configuration configuration = 3;
*/
public com.github.wenweihu86.raft.proto.RaftMessage.Configuration getConfiguration() {
if (configurationBuilder_ == null) {
return configuration_ == null ? com.github.wenweihu86.raft.proto.RaftMessage.Configuration.getDefaultInstance() : configuration_;
} else {
return configurationBuilder_.getMessage();
}
}
/**
* optional .raft.Configuration configuration = 3;
*/
public Builder setConfiguration(com.github.wenweihu86.raft.proto.RaftMessage.Configuration value) {
if (configurationBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
configuration_ = value;
onChanged();
} else {
configurationBuilder_.setMessage(value);
}
return this;
}
/**
* optional .raft.Configuration configuration = 3;
*/
public Builder setConfiguration(
com.github.wenweihu86.raft.proto.RaftMessage.Configuration.Builder builderForValue) {
if (configurationBuilder_ == null) {
configuration_ = builderForValue.build();
onChanged();
} else {
configurationBuilder_.setMessage(builderForValue.build());
}
return this;
}
/**
* optional .raft.Configuration configuration = 3;
*/
public Builder mergeConfiguration(com.github.wenweihu86.raft.proto.RaftMessage.Configuration value) {
if (configurationBuilder_ == null) {
if (configuration_ != null) {
configuration_ =
com.github.wenweihu86.raft.proto.RaftMessage.Configuration.newBuilder(configuration_).mergeFrom(value).buildPartial();
} else {
configuration_ = value;
}
onChanged();
} else {
configurationBuilder_.mergeFrom(value);
}
return this;
}
/**
* optional .raft.Configuration configuration = 3;
*/
public Builder clearConfiguration() {
if (configurationBuilder_ == null) {
configuration_ = null;
onChanged();
} else {
configuration_ = null;
configurationBuilder_ = null;
}
return this;
}
/**
* optional .raft.Configuration configuration = 3;
*/
public com.github.wenweihu86.raft.proto.RaftMessage.Configuration.Builder getConfigurationBuilder() {
onChanged();
return getConfigurationFieldBuilder().getBuilder();
}
/**
* optional .raft.Configuration configuration = 3;
*/
public com.github.wenweihu86.raft.proto.RaftMessage.ConfigurationOrBuilder getConfigurationOrBuilder() {
if (configurationBuilder_ != null) {
return configurationBuilder_.getMessageOrBuilder();
} else {
return configuration_ == null ?
com.github.wenweihu86.raft.proto.RaftMessage.Configuration.getDefaultInstance() : configuration_;
}
}
/**
* optional .raft.Configuration configuration = 3;
*/
private com.google.protobuf.SingleFieldBuilderV3<
com.github.wenweihu86.raft.proto.RaftMessage.Configuration, com.github.wenweihu86.raft.proto.RaftMessage.Configuration.Builder, com.github.wenweihu86.raft.proto.RaftMessage.ConfigurationOrBuilder>
getConfigurationFieldBuilder() {
if (configurationBuilder_ == null) {
configurationBuilder_ = new com.google.protobuf.SingleFieldBuilderV3<
com.github.wenweihu86.raft.proto.RaftMessage.Configuration, com.github.wenweihu86.raft.proto.RaftMessage.Configuration.Builder, com.github.wenweihu86.raft.proto.RaftMessage.ConfigurationOrBuilder>(
getConfiguration(),
getParentForChildren(),
isClean());
configuration_ = null;
}
return configurationBuilder_;
}
public final Builder setUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return this;
}
public final Builder mergeUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return this;
}
// @@protoc_insertion_point(builder_scope:raft.SnapshotMetaData)
}
// @@protoc_insertion_point(class_scope:raft.SnapshotMetaData)
private static final com.github.wenweihu86.raft.proto.RaftMessage.SnapshotMetaData DEFAULT_INSTANCE;
static {
DEFAULT_INSTANCE = new com.github.wenweihu86.raft.proto.RaftMessage.SnapshotMetaData();
}
public static com.github.wenweihu86.raft.proto.RaftMessage.SnapshotMetaData getDefaultInstance() {
return DEFAULT_INSTANCE;
}
private static final com.google.protobuf.Parser
PARSER = new com.google.protobuf.AbstractParser() {
public SnapshotMetaData parsePartialFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return new SnapshotMetaData(input, extensionRegistry);
}
};
public static com.google.protobuf.Parser parser() {
return PARSER;
}
@java.lang.Override
public com.google.protobuf.Parser getParserForType() {
return PARSER;
}
public com.github.wenweihu86.raft.proto.RaftMessage.SnapshotMetaData getDefaultInstanceForType() {
return DEFAULT_INSTANCE;
}
}
public interface LogEntryOrBuilder extends
// @@protoc_insertion_point(interface_extends:raft.LogEntry)
com.google.protobuf.MessageOrBuilder {
/**
* optional uint64 term = 1;
*/
long getTerm();
/**
* optional uint64 index = 2;
*/
long getIndex();
/**
* optional .raft.EntryType type = 3;
*/
int getTypeValue();
/**
* optional .raft.EntryType type = 3;
*/
com.github.wenweihu86.raft.proto.RaftMessage.EntryType getType();
/**
* optional bytes data = 4;
*/
com.google.protobuf.ByteString getData();
}
/**
* Protobuf type {@code raft.LogEntry}
*/
public static final class LogEntry extends
com.google.protobuf.GeneratedMessageV3 implements
// @@protoc_insertion_point(message_implements:raft.LogEntry)
LogEntryOrBuilder {
// Use LogEntry.newBuilder() to construct.
private LogEntry(com.google.protobuf.GeneratedMessageV3.Builder> builder) {
super(builder);
}
private LogEntry() {
term_ = 0L;
index_ = 0L;
type_ = 0;
data_ = com.google.protobuf.ByteString.EMPTY;
}
@java.lang.Override
public final com.google.protobuf.UnknownFieldSet
getUnknownFields() {
return com.google.protobuf.UnknownFieldSet.getDefaultInstance();
}
private LogEntry(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
this();
int mutable_bitField0_ = 0;
try {
boolean done = false;
while (!done) {
int tag = input.readTag();
switch (tag) {
case 0:
done = true;
break;
default: {
if (!input.skipField(tag)) {
done = true;
}
break;
}
case 8: {
term_ = input.readUInt64();
break;
}
case 16: {
index_ = input.readUInt64();
break;
}
case 24: {
int rawValue = input.readEnum();
type_ = rawValue;
break;
}
case 34: {
data_ = input.readBytes();
break;
}
}
}
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.setUnfinishedMessage(this);
} catch (java.io.IOException e) {
throw new com.google.protobuf.InvalidProtocolBufferException(
e).setUnfinishedMessage(this);
} finally {
makeExtensionsImmutable();
}
}
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return com.github.wenweihu86.raft.proto.RaftMessage.internal_static_raft_LogEntry_descriptor;
}
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return com.github.wenweihu86.raft.proto.RaftMessage.internal_static_raft_LogEntry_fieldAccessorTable
.ensureFieldAccessorsInitialized(
com.github.wenweihu86.raft.proto.RaftMessage.LogEntry.class, com.github.wenweihu86.raft.proto.RaftMessage.LogEntry.Builder.class);
}
public static final int TERM_FIELD_NUMBER = 1;
private long term_;
/**
* optional uint64 term = 1;
*/
public long getTerm() {
return term_;
}
public static final int INDEX_FIELD_NUMBER = 2;
private long index_;
/**
* optional uint64 index = 2;
*/
public long getIndex() {
return index_;
}
public static final int TYPE_FIELD_NUMBER = 3;
private int type_;
/**
* optional .raft.EntryType type = 3;
*/
public int getTypeValue() {
return type_;
}
/**
* optional .raft.EntryType type = 3;
*/
public com.github.wenweihu86.raft.proto.RaftMessage.EntryType getType() {
com.github.wenweihu86.raft.proto.RaftMessage.EntryType result = com.github.wenweihu86.raft.proto.RaftMessage.EntryType.valueOf(type_);
return result == null ? com.github.wenweihu86.raft.proto.RaftMessage.EntryType.UNRECOGNIZED : result;
}
public static final int DATA_FIELD_NUMBER = 4;
private com.google.protobuf.ByteString data_;
/**
* optional bytes data = 4;
*/
public com.google.protobuf.ByteString getData() {
return data_;
}
private byte memoizedIsInitialized = -1;
public final boolean isInitialized() {
byte isInitialized = memoizedIsInitialized;
if (isInitialized == 1) return true;
if (isInitialized == 0) return false;
memoizedIsInitialized = 1;
return true;
}
public void writeTo(com.google.protobuf.CodedOutputStream output)
throws java.io.IOException {
if (term_ != 0L) {
output.writeUInt64(1, term_);
}
if (index_ != 0L) {
output.writeUInt64(2, index_);
}
if (type_ != com.github.wenweihu86.raft.proto.RaftMessage.EntryType.ENTRY_TYPE_DATA.getNumber()) {
output.writeEnum(3, type_);
}
if (!data_.isEmpty()) {
output.writeBytes(4, data_);
}
}
public int getSerializedSize() {
int size = memoizedSize;
if (size != -1) return size;
size = 0;
if (term_ != 0L) {
size += com.google.protobuf.CodedOutputStream
.computeUInt64Size(1, term_);
}
if (index_ != 0L) {
size += com.google.protobuf.CodedOutputStream
.computeUInt64Size(2, index_);
}
if (type_ != com.github.wenweihu86.raft.proto.RaftMessage.EntryType.ENTRY_TYPE_DATA.getNumber()) {
size += com.google.protobuf.CodedOutputStream
.computeEnumSize(3, type_);
}
if (!data_.isEmpty()) {
size += com.google.protobuf.CodedOutputStream
.computeBytesSize(4, data_);
}
memoizedSize = size;
return size;
}
private static final long serialVersionUID = 0L;
@java.lang.Override
public boolean equals(final java.lang.Object obj) {
if (obj == this) {
return true;
}
if (!(obj instanceof com.github.wenweihu86.raft.proto.RaftMessage.LogEntry)) {
return super.equals(obj);
}
com.github.wenweihu86.raft.proto.RaftMessage.LogEntry other = (com.github.wenweihu86.raft.proto.RaftMessage.LogEntry) obj;
boolean result = true;
result = result && (getTerm()
== other.getTerm());
result = result && (getIndex()
== other.getIndex());
result = result && type_ == other.type_;
result = result && getData()
.equals(other.getData());
return result;
}
@java.lang.Override
public int hashCode() {
if (memoizedHashCode != 0) {
return memoizedHashCode;
}
int hash = 41;
hash = (19 * hash) + getDescriptorForType().hashCode();
hash = (37 * hash) + TERM_FIELD_NUMBER;
hash = (53 * hash) + com.google.protobuf.Internal.hashLong(
getTerm());
hash = (37 * hash) + INDEX_FIELD_NUMBER;
hash = (53 * hash) + com.google.protobuf.Internal.hashLong(
getIndex());
hash = (37 * hash) + TYPE_FIELD_NUMBER;
hash = (53 * hash) + type_;
hash = (37 * hash) + DATA_FIELD_NUMBER;
hash = (53 * hash) + getData().hashCode();
hash = (29 * hash) + unknownFields.hashCode();
memoizedHashCode = hash;
return hash;
}
public static com.github.wenweihu86.raft.proto.RaftMessage.LogEntry parseFrom(
com.google.protobuf.ByteString data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static com.github.wenweihu86.raft.proto.RaftMessage.LogEntry parseFrom(
com.google.protobuf.ByteString data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static com.github.wenweihu86.raft.proto.RaftMessage.LogEntry parseFrom(byte[] data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static com.github.wenweihu86.raft.proto.RaftMessage.LogEntry parseFrom(
byte[] data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static com.github.wenweihu86.raft.proto.RaftMessage.LogEntry parseFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static com.github.wenweihu86.raft.proto.RaftMessage.LogEntry parseFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
public static com.github.wenweihu86.raft.proto.RaftMessage.LogEntry parseDelimitedFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input);
}
public static com.github.wenweihu86.raft.proto.RaftMessage.LogEntry parseDelimitedFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input, extensionRegistry);
}
public static com.github.wenweihu86.raft.proto.RaftMessage.LogEntry parseFrom(
com.google.protobuf.CodedInputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static com.github.wenweihu86.raft.proto.RaftMessage.LogEntry parseFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
public Builder newBuilderForType() { return newBuilder(); }
public static Builder newBuilder() {
return DEFAULT_INSTANCE.toBuilder();
}
public static Builder newBuilder(com.github.wenweihu86.raft.proto.RaftMessage.LogEntry prototype) {
return DEFAULT_INSTANCE.toBuilder().mergeFrom(prototype);
}
public Builder toBuilder() {
return this == DEFAULT_INSTANCE
? new Builder() : new Builder().mergeFrom(this);
}
@java.lang.Override
protected Builder newBuilderForType(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
Builder builder = new Builder(parent);
return builder;
}
/**
* Protobuf type {@code raft.LogEntry}
*/
public static final class Builder extends
com.google.protobuf.GeneratedMessageV3.Builder implements
// @@protoc_insertion_point(builder_implements:raft.LogEntry)
com.github.wenweihu86.raft.proto.RaftMessage.LogEntryOrBuilder {
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return com.github.wenweihu86.raft.proto.RaftMessage.internal_static_raft_LogEntry_descriptor;
}
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return com.github.wenweihu86.raft.proto.RaftMessage.internal_static_raft_LogEntry_fieldAccessorTable
.ensureFieldAccessorsInitialized(
com.github.wenweihu86.raft.proto.RaftMessage.LogEntry.class, com.github.wenweihu86.raft.proto.RaftMessage.LogEntry.Builder.class);
}
// Construct using com.github.wenweihu86.raft.proto.RaftMessage.LogEntry.newBuilder()
private Builder() {
maybeForceBuilderInitialization();
}
private Builder(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
super(parent);
maybeForceBuilderInitialization();
}
private void maybeForceBuilderInitialization() {
if (com.google.protobuf.GeneratedMessageV3
.alwaysUseFieldBuilders) {
}
}
public Builder clear() {
super.clear();
term_ = 0L;
index_ = 0L;
type_ = 0;
data_ = com.google.protobuf.ByteString.EMPTY;
return this;
}
public com.google.protobuf.Descriptors.Descriptor
getDescriptorForType() {
return com.github.wenweihu86.raft.proto.RaftMessage.internal_static_raft_LogEntry_descriptor;
}
public com.github.wenweihu86.raft.proto.RaftMessage.LogEntry getDefaultInstanceForType() {
return com.github.wenweihu86.raft.proto.RaftMessage.LogEntry.getDefaultInstance();
}
public com.github.wenweihu86.raft.proto.RaftMessage.LogEntry build() {
com.github.wenweihu86.raft.proto.RaftMessage.LogEntry result = buildPartial();
if (!result.isInitialized()) {
throw newUninitializedMessageException(result);
}
return result;
}
public com.github.wenweihu86.raft.proto.RaftMessage.LogEntry buildPartial() {
com.github.wenweihu86.raft.proto.RaftMessage.LogEntry result = new com.github.wenweihu86.raft.proto.RaftMessage.LogEntry(this);
result.term_ = term_;
result.index_ = index_;
result.type_ = type_;
result.data_ = data_;
onBuilt();
return result;
}
public Builder clone() {
return (Builder) super.clone();
}
public Builder setField(
com.google.protobuf.Descriptors.FieldDescriptor field,
Object value) {
return (Builder) super.setField(field, value);
}
public Builder clearField(
com.google.protobuf.Descriptors.FieldDescriptor field) {
return (Builder) super.clearField(field);
}
public Builder clearOneof(
com.google.protobuf.Descriptors.OneofDescriptor oneof) {
return (Builder) super.clearOneof(oneof);
}
public Builder setRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
int index, Object value) {
return (Builder) super.setRepeatedField(field, index, value);
}
public Builder addRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
Object value) {
return (Builder) super.addRepeatedField(field, value);
}
public Builder mergeFrom(com.google.protobuf.Message other) {
if (other instanceof com.github.wenweihu86.raft.proto.RaftMessage.LogEntry) {
return mergeFrom((com.github.wenweihu86.raft.proto.RaftMessage.LogEntry)other);
} else {
super.mergeFrom(other);
return this;
}
}
public Builder mergeFrom(com.github.wenweihu86.raft.proto.RaftMessage.LogEntry other) {
if (other == com.github.wenweihu86.raft.proto.RaftMessage.LogEntry.getDefaultInstance()) return this;
if (other.getTerm() != 0L) {
setTerm(other.getTerm());
}
if (other.getIndex() != 0L) {
setIndex(other.getIndex());
}
if (other.type_ != 0) {
setTypeValue(other.getTypeValue());
}
if (other.getData() != com.google.protobuf.ByteString.EMPTY) {
setData(other.getData());
}
onChanged();
return this;
}
public final boolean isInitialized() {
return true;
}
public Builder mergeFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
com.github.wenweihu86.raft.proto.RaftMessage.LogEntry parsedMessage = null;
try {
parsedMessage = PARSER.parsePartialFrom(input, extensionRegistry);
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
parsedMessage = (com.github.wenweihu86.raft.proto.RaftMessage.LogEntry) e.getUnfinishedMessage();
throw e.unwrapIOException();
} finally {
if (parsedMessage != null) {
mergeFrom(parsedMessage);
}
}
return this;
}
private long term_ ;
/**
* optional uint64 term = 1;
*/
public long getTerm() {
return term_;
}
/**
* optional uint64 term = 1;
*/
public Builder setTerm(long value) {
term_ = value;
onChanged();
return this;
}
/**
* optional uint64 term = 1;
*/
public Builder clearTerm() {
term_ = 0L;
onChanged();
return this;
}
private long index_ ;
/**
* optional uint64 index = 2;
*/
public long getIndex() {
return index_;
}
/**
* optional uint64 index = 2;
*/
public Builder setIndex(long value) {
index_ = value;
onChanged();
return this;
}
/**
* optional uint64 index = 2;
*/
public Builder clearIndex() {
index_ = 0L;
onChanged();
return this;
}
private int type_ = 0;
/**
* optional .raft.EntryType type = 3;
*/
public int getTypeValue() {
return type_;
}
/**
* optional .raft.EntryType type = 3;
*/
public Builder setTypeValue(int value) {
type_ = value;
onChanged();
return this;
}
/**
* optional .raft.EntryType type = 3;
*/
public com.github.wenweihu86.raft.proto.RaftMessage.EntryType getType() {
com.github.wenweihu86.raft.proto.RaftMessage.EntryType result = com.github.wenweihu86.raft.proto.RaftMessage.EntryType.valueOf(type_);
return result == null ? com.github.wenweihu86.raft.proto.RaftMessage.EntryType.UNRECOGNIZED : result;
}
/**
* optional .raft.EntryType type = 3;
*/
public Builder setType(com.github.wenweihu86.raft.proto.RaftMessage.EntryType value) {
if (value == null) {
throw new NullPointerException();
}
type_ = value.getNumber();
onChanged();
return this;
}
/**
* optional .raft.EntryType type = 3;
*/
public Builder clearType() {
type_ = 0;
onChanged();
return this;
}
private com.google.protobuf.ByteString data_ = com.google.protobuf.ByteString.EMPTY;
/**
* optional bytes data = 4;
*/
public com.google.protobuf.ByteString getData() {
return data_;
}
/**
* optional bytes data = 4;
*/
public Builder setData(com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
data_ = value;
onChanged();
return this;
}
/**
* optional bytes data = 4;
*/
public Builder clearData() {
data_ = getDefaultInstance().getData();
onChanged();
return this;
}
public final Builder setUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return this;
}
public final Builder mergeUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return this;
}
// @@protoc_insertion_point(builder_scope:raft.LogEntry)
}
// @@protoc_insertion_point(class_scope:raft.LogEntry)
private static final com.github.wenweihu86.raft.proto.RaftMessage.LogEntry DEFAULT_INSTANCE;
static {
DEFAULT_INSTANCE = new com.github.wenweihu86.raft.proto.RaftMessage.LogEntry();
}
public static com.github.wenweihu86.raft.proto.RaftMessage.LogEntry getDefaultInstance() {
return DEFAULT_INSTANCE;
}
private static final com.google.protobuf.Parser
PARSER = new com.google.protobuf.AbstractParser() {
public LogEntry parsePartialFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return new LogEntry(input, extensionRegistry);
}
};
public static com.google.protobuf.Parser parser() {
return PARSER;
}
@java.lang.Override
public com.google.protobuf.Parser getParserForType() {
return PARSER;
}
public com.github.wenweihu86.raft.proto.RaftMessage.LogEntry getDefaultInstanceForType() {
return DEFAULT_INSTANCE;
}
}
public interface VoteRequestOrBuilder extends
// @@protoc_insertion_point(interface_extends:raft.VoteRequest)
com.google.protobuf.MessageOrBuilder {
/**
*
* 请求选票的候选人的 Id
*
*
* optional uint32 server_id = 1;
*/
int getServerId();
/**
*
* 候选人的任期号
*
*
* optional uint64 term = 2;
*/
long getTerm();
/**
*
* 候选人的最后日志条目的索引值
*
*
* optional uint64 last_log_term = 3;
*/
long getLastLogTerm();
/**
*
* 候选人最后日志条目的任期号
*
*
* optional uint64 last_log_index = 4;
*/
long getLastLogIndex();
}
/**
* Protobuf type {@code raft.VoteRequest}
*/
public static final class VoteRequest extends
com.google.protobuf.GeneratedMessageV3 implements
// @@protoc_insertion_point(message_implements:raft.VoteRequest)
VoteRequestOrBuilder {
// Use VoteRequest.newBuilder() to construct.
private VoteRequest(com.google.protobuf.GeneratedMessageV3.Builder> builder) {
super(builder);
}
private VoteRequest() {
serverId_ = 0;
term_ = 0L;
lastLogTerm_ = 0L;
lastLogIndex_ = 0L;
}
@java.lang.Override
public final com.google.protobuf.UnknownFieldSet
getUnknownFields() {
return com.google.protobuf.UnknownFieldSet.getDefaultInstance();
}
private VoteRequest(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
this();
int mutable_bitField0_ = 0;
try {
boolean done = false;
while (!done) {
int tag = input.readTag();
switch (tag) {
case 0:
done = true;
break;
default: {
if (!input.skipField(tag)) {
done = true;
}
break;
}
case 8: {
serverId_ = input.readUInt32();
break;
}
case 16: {
term_ = input.readUInt64();
break;
}
case 24: {
lastLogTerm_ = input.readUInt64();
break;
}
case 32: {
lastLogIndex_ = input.readUInt64();
break;
}
}
}
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.setUnfinishedMessage(this);
} catch (java.io.IOException e) {
throw new com.google.protobuf.InvalidProtocolBufferException(
e).setUnfinishedMessage(this);
} finally {
makeExtensionsImmutable();
}
}
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return com.github.wenweihu86.raft.proto.RaftMessage.internal_static_raft_VoteRequest_descriptor;
}
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return com.github.wenweihu86.raft.proto.RaftMessage.internal_static_raft_VoteRequest_fieldAccessorTable
.ensureFieldAccessorsInitialized(
com.github.wenweihu86.raft.proto.RaftMessage.VoteRequest.class, com.github.wenweihu86.raft.proto.RaftMessage.VoteRequest.Builder.class);
}
public static final int SERVER_ID_FIELD_NUMBER = 1;
private int serverId_;
/**
*
* 请求选票的候选人的 Id
*
*
* optional uint32 server_id = 1;
*/
public int getServerId() {
return serverId_;
}
public static final int TERM_FIELD_NUMBER = 2;
private long term_;
/**
*
* 候选人的任期号
*
*
* optional uint64 term = 2;
*/
public long getTerm() {
return term_;
}
public static final int LAST_LOG_TERM_FIELD_NUMBER = 3;
private long lastLogTerm_;
/**
*
* 候选人的最后日志条目的索引值
*
*
* optional uint64 last_log_term = 3;
*/
public long getLastLogTerm() {
return lastLogTerm_;
}
public static final int LAST_LOG_INDEX_FIELD_NUMBER = 4;
private long lastLogIndex_;
/**
*
* 候选人最后日志条目的任期号
*
*
* optional uint64 last_log_index = 4;
*/
public long getLastLogIndex() {
return lastLogIndex_;
}
private byte memoizedIsInitialized = -1;
public final boolean isInitialized() {
byte isInitialized = memoizedIsInitialized;
if (isInitialized == 1) return true;
if (isInitialized == 0) return false;
memoizedIsInitialized = 1;
return true;
}
public void writeTo(com.google.protobuf.CodedOutputStream output)
throws java.io.IOException {
if (serverId_ != 0) {
output.writeUInt32(1, serverId_);
}
if (term_ != 0L) {
output.writeUInt64(2, term_);
}
if (lastLogTerm_ != 0L) {
output.writeUInt64(3, lastLogTerm_);
}
if (lastLogIndex_ != 0L) {
output.writeUInt64(4, lastLogIndex_);
}
}
public int getSerializedSize() {
int size = memoizedSize;
if (size != -1) return size;
size = 0;
if (serverId_ != 0) {
size += com.google.protobuf.CodedOutputStream
.computeUInt32Size(1, serverId_);
}
if (term_ != 0L) {
size += com.google.protobuf.CodedOutputStream
.computeUInt64Size(2, term_);
}
if (lastLogTerm_ != 0L) {
size += com.google.protobuf.CodedOutputStream
.computeUInt64Size(3, lastLogTerm_);
}
if (lastLogIndex_ != 0L) {
size += com.google.protobuf.CodedOutputStream
.computeUInt64Size(4, lastLogIndex_);
}
memoizedSize = size;
return size;
}
private static final long serialVersionUID = 0L;
@java.lang.Override
public boolean equals(final java.lang.Object obj) {
if (obj == this) {
return true;
}
if (!(obj instanceof com.github.wenweihu86.raft.proto.RaftMessage.VoteRequest)) {
return super.equals(obj);
}
com.github.wenweihu86.raft.proto.RaftMessage.VoteRequest other = (com.github.wenweihu86.raft.proto.RaftMessage.VoteRequest) obj;
boolean result = true;
result = result && (getServerId()
== other.getServerId());
result = result && (getTerm()
== other.getTerm());
result = result && (getLastLogTerm()
== other.getLastLogTerm());
result = result && (getLastLogIndex()
== other.getLastLogIndex());
return result;
}
@java.lang.Override
public int hashCode() {
if (memoizedHashCode != 0) {
return memoizedHashCode;
}
int hash = 41;
hash = (19 * hash) + getDescriptorForType().hashCode();
hash = (37 * hash) + SERVER_ID_FIELD_NUMBER;
hash = (53 * hash) + getServerId();
hash = (37 * hash) + TERM_FIELD_NUMBER;
hash = (53 * hash) + com.google.protobuf.Internal.hashLong(
getTerm());
hash = (37 * hash) + LAST_LOG_TERM_FIELD_NUMBER;
hash = (53 * hash) + com.google.protobuf.Internal.hashLong(
getLastLogTerm());
hash = (37 * hash) + LAST_LOG_INDEX_FIELD_NUMBER;
hash = (53 * hash) + com.google.protobuf.Internal.hashLong(
getLastLogIndex());
hash = (29 * hash) + unknownFields.hashCode();
memoizedHashCode = hash;
return hash;
}
public static com.github.wenweihu86.raft.proto.RaftMessage.VoteRequest parseFrom(
com.google.protobuf.ByteString data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static com.github.wenweihu86.raft.proto.RaftMessage.VoteRequest parseFrom(
com.google.protobuf.ByteString data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static com.github.wenweihu86.raft.proto.RaftMessage.VoteRequest parseFrom(byte[] data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static com.github.wenweihu86.raft.proto.RaftMessage.VoteRequest parseFrom(
byte[] data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static com.github.wenweihu86.raft.proto.RaftMessage.VoteRequest parseFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static com.github.wenweihu86.raft.proto.RaftMessage.VoteRequest parseFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
public static com.github.wenweihu86.raft.proto.RaftMessage.VoteRequest parseDelimitedFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input);
}
public static com.github.wenweihu86.raft.proto.RaftMessage.VoteRequest parseDelimitedFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input, extensionRegistry);
}
public static com.github.wenweihu86.raft.proto.RaftMessage.VoteRequest parseFrom(
com.google.protobuf.CodedInputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static com.github.wenweihu86.raft.proto.RaftMessage.VoteRequest parseFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
public Builder newBuilderForType() { return newBuilder(); }
public static Builder newBuilder() {
return DEFAULT_INSTANCE.toBuilder();
}
public static Builder newBuilder(com.github.wenweihu86.raft.proto.RaftMessage.VoteRequest prototype) {
return DEFAULT_INSTANCE.toBuilder().mergeFrom(prototype);
}
public Builder toBuilder() {
return this == DEFAULT_INSTANCE
? new Builder() : new Builder().mergeFrom(this);
}
@java.lang.Override
protected Builder newBuilderForType(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
Builder builder = new Builder(parent);
return builder;
}
/**
* Protobuf type {@code raft.VoteRequest}
*/
public static final class Builder extends
com.google.protobuf.GeneratedMessageV3.Builder implements
// @@protoc_insertion_point(builder_implements:raft.VoteRequest)
com.github.wenweihu86.raft.proto.RaftMessage.VoteRequestOrBuilder {
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return com.github.wenweihu86.raft.proto.RaftMessage.internal_static_raft_VoteRequest_descriptor;
}
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return com.github.wenweihu86.raft.proto.RaftMessage.internal_static_raft_VoteRequest_fieldAccessorTable
.ensureFieldAccessorsInitialized(
com.github.wenweihu86.raft.proto.RaftMessage.VoteRequest.class, com.github.wenweihu86.raft.proto.RaftMessage.VoteRequest.Builder.class);
}
// Construct using com.github.wenweihu86.raft.proto.RaftMessage.VoteRequest.newBuilder()
private Builder() {
maybeForceBuilderInitialization();
}
private Builder(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
super(parent);
maybeForceBuilderInitialization();
}
private void maybeForceBuilderInitialization() {
if (com.google.protobuf.GeneratedMessageV3
.alwaysUseFieldBuilders) {
}
}
public Builder clear() {
super.clear();
serverId_ = 0;
term_ = 0L;
lastLogTerm_ = 0L;
lastLogIndex_ = 0L;
return this;
}
public com.google.protobuf.Descriptors.Descriptor
getDescriptorForType() {
return com.github.wenweihu86.raft.proto.RaftMessage.internal_static_raft_VoteRequest_descriptor;
}
public com.github.wenweihu86.raft.proto.RaftMessage.VoteRequest getDefaultInstanceForType() {
return com.github.wenweihu86.raft.proto.RaftMessage.VoteRequest.getDefaultInstance();
}
public com.github.wenweihu86.raft.proto.RaftMessage.VoteRequest build() {
com.github.wenweihu86.raft.proto.RaftMessage.VoteRequest result = buildPartial();
if (!result.isInitialized()) {
throw newUninitializedMessageException(result);
}
return result;
}
public com.github.wenweihu86.raft.proto.RaftMessage.VoteRequest buildPartial() {
com.github.wenweihu86.raft.proto.RaftMessage.VoteRequest result = new com.github.wenweihu86.raft.proto.RaftMessage.VoteRequest(this);
result.serverId_ = serverId_;
result.term_ = term_;
result.lastLogTerm_ = lastLogTerm_;
result.lastLogIndex_ = lastLogIndex_;
onBuilt();
return result;
}
public Builder clone() {
return (Builder) super.clone();
}
public Builder setField(
com.google.protobuf.Descriptors.FieldDescriptor field,
Object value) {
return (Builder) super.setField(field, value);
}
public Builder clearField(
com.google.protobuf.Descriptors.FieldDescriptor field) {
return (Builder) super.clearField(field);
}
public Builder clearOneof(
com.google.protobuf.Descriptors.OneofDescriptor oneof) {
return (Builder) super.clearOneof(oneof);
}
public Builder setRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
int index, Object value) {
return (Builder) super.setRepeatedField(field, index, value);
}
public Builder addRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
Object value) {
return (Builder) super.addRepeatedField(field, value);
}
public Builder mergeFrom(com.google.protobuf.Message other) {
if (other instanceof com.github.wenweihu86.raft.proto.RaftMessage.VoteRequest) {
return mergeFrom((com.github.wenweihu86.raft.proto.RaftMessage.VoteRequest)other);
} else {
super.mergeFrom(other);
return this;
}
}
public Builder mergeFrom(com.github.wenweihu86.raft.proto.RaftMessage.VoteRequest other) {
if (other == com.github.wenweihu86.raft.proto.RaftMessage.VoteRequest.getDefaultInstance()) return this;
if (other.getServerId() != 0) {
setServerId(other.getServerId());
}
if (other.getTerm() != 0L) {
setTerm(other.getTerm());
}
if (other.getLastLogTerm() != 0L) {
setLastLogTerm(other.getLastLogTerm());
}
if (other.getLastLogIndex() != 0L) {
setLastLogIndex(other.getLastLogIndex());
}
onChanged();
return this;
}
public final boolean isInitialized() {
return true;
}
public Builder mergeFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
com.github.wenweihu86.raft.proto.RaftMessage.VoteRequest parsedMessage = null;
try {
parsedMessage = PARSER.parsePartialFrom(input, extensionRegistry);
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
parsedMessage = (com.github.wenweihu86.raft.proto.RaftMessage.VoteRequest) e.getUnfinishedMessage();
throw e.unwrapIOException();
} finally {
if (parsedMessage != null) {
mergeFrom(parsedMessage);
}
}
return this;
}
private int serverId_ ;
/**
*
* 请求选票的候选人的 Id
*
*
* optional uint32 server_id = 1;
*/
public int getServerId() {
return serverId_;
}
/**
*
* 请求选票的候选人的 Id
*
*
* optional uint32 server_id = 1;
*/
public Builder setServerId(int value) {
serverId_ = value;
onChanged();
return this;
}
/**
*
* 请求选票的候选人的 Id
*
*
* optional uint32 server_id = 1;
*/
public Builder clearServerId() {
serverId_ = 0;
onChanged();
return this;
}
private long term_ ;
/**
*
* 候选人的任期号
*
*
* optional uint64 term = 2;
*/
public long getTerm() {
return term_;
}
/**
*
* 候选人的任期号
*
*
* optional uint64 term = 2;
*/
public Builder setTerm(long value) {
term_ = value;
onChanged();
return this;
}
/**
*
* 候选人的任期号
*
*
* optional uint64 term = 2;
*/
public Builder clearTerm() {
term_ = 0L;
onChanged();
return this;
}
private long lastLogTerm_ ;
/**
*
* 候选人的最后日志条目的索引值
*
*
* optional uint64 last_log_term = 3;
*/
public long getLastLogTerm() {
return lastLogTerm_;
}
/**
*
* 候选人的最后日志条目的索引值
*
*
* optional uint64 last_log_term = 3;
*/
public Builder setLastLogTerm(long value) {
lastLogTerm_ = value;
onChanged();
return this;
}
/**
*
* 候选人的最后日志条目的索引值
*
*
* optional uint64 last_log_term = 3;
*/
public Builder clearLastLogTerm() {
lastLogTerm_ = 0L;
onChanged();
return this;
}
private long lastLogIndex_ ;
/**
*
* 候选人最后日志条目的任期号
*
*
* optional uint64 last_log_index = 4;
*/
public long getLastLogIndex() {
return lastLogIndex_;
}
/**
*
* 候选人最后日志条目的任期号
*
*
* optional uint64 last_log_index = 4;
*/
public Builder setLastLogIndex(long value) {
lastLogIndex_ = value;
onChanged();
return this;
}
/**
*
* 候选人最后日志条目的任期号
*
*
* optional uint64 last_log_index = 4;
*/
public Builder clearLastLogIndex() {
lastLogIndex_ = 0L;
onChanged();
return this;
}
public final Builder setUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return this;
}
public final Builder mergeUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return this;
}
// @@protoc_insertion_point(builder_scope:raft.VoteRequest)
}
// @@protoc_insertion_point(class_scope:raft.VoteRequest)
private static final com.github.wenweihu86.raft.proto.RaftMessage.VoteRequest DEFAULT_INSTANCE;
static {
DEFAULT_INSTANCE = new com.github.wenweihu86.raft.proto.RaftMessage.VoteRequest();
}
public static com.github.wenweihu86.raft.proto.RaftMessage.VoteRequest getDefaultInstance() {
return DEFAULT_INSTANCE;
}
private static final com.google.protobuf.Parser
PARSER = new com.google.protobuf.AbstractParser() {
public VoteRequest parsePartialFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return new VoteRequest(input, extensionRegistry);
}
};
public static com.google.protobuf.Parser parser() {
return PARSER;
}
@java.lang.Override
public com.google.protobuf.Parser getParserForType() {
return PARSER;
}
public com.github.wenweihu86.raft.proto.RaftMessage.VoteRequest getDefaultInstanceForType() {
return DEFAULT_INSTANCE;
}
}
public interface VoteResponseOrBuilder extends
// @@protoc_insertion_point(interface_extends:raft.VoteResponse)
com.google.protobuf.MessageOrBuilder {
/**
*
* 当前任期号,以便于候选人去更新自己的任期号
*
*
* optional uint64 term = 1;
*/
long getTerm();
/**
*
* 候选人赢得了此张选票时为真
*
*
* optional bool granted = 2;
*/
boolean getGranted();
}
/**
* Protobuf type {@code raft.VoteResponse}
*/
public static final class VoteResponse extends
com.google.protobuf.GeneratedMessageV3 implements
// @@protoc_insertion_point(message_implements:raft.VoteResponse)
VoteResponseOrBuilder {
// Use VoteResponse.newBuilder() to construct.
private VoteResponse(com.google.protobuf.GeneratedMessageV3.Builder> builder) {
super(builder);
}
private VoteResponse() {
term_ = 0L;
granted_ = false;
}
@java.lang.Override
public final com.google.protobuf.UnknownFieldSet
getUnknownFields() {
return com.google.protobuf.UnknownFieldSet.getDefaultInstance();
}
private VoteResponse(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
this();
int mutable_bitField0_ = 0;
try {
boolean done = false;
while (!done) {
int tag = input.readTag();
switch (tag) {
case 0:
done = true;
break;
default: {
if (!input.skipField(tag)) {
done = true;
}
break;
}
case 8: {
term_ = input.readUInt64();
break;
}
case 16: {
granted_ = input.readBool();
break;
}
}
}
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.setUnfinishedMessage(this);
} catch (java.io.IOException e) {
throw new com.google.protobuf.InvalidProtocolBufferException(
e).setUnfinishedMessage(this);
} finally {
makeExtensionsImmutable();
}
}
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return com.github.wenweihu86.raft.proto.RaftMessage.internal_static_raft_VoteResponse_descriptor;
}
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return com.github.wenweihu86.raft.proto.RaftMessage.internal_static_raft_VoteResponse_fieldAccessorTable
.ensureFieldAccessorsInitialized(
com.github.wenweihu86.raft.proto.RaftMessage.VoteResponse.class, com.github.wenweihu86.raft.proto.RaftMessage.VoteResponse.Builder.class);
}
public static final int TERM_FIELD_NUMBER = 1;
private long term_;
/**
*
* 当前任期号,以便于候选人去更新自己的任期号
*
*
* optional uint64 term = 1;
*/
public long getTerm() {
return term_;
}
public static final int GRANTED_FIELD_NUMBER = 2;
private boolean granted_;
/**
*
* 候选人赢得了此张选票时为真
*
*
* optional bool granted = 2;
*/
public boolean getGranted() {
return granted_;
}
private byte memoizedIsInitialized = -1;
public final boolean isInitialized() {
byte isInitialized = memoizedIsInitialized;
if (isInitialized == 1) return true;
if (isInitialized == 0) return false;
memoizedIsInitialized = 1;
return true;
}
public void writeTo(com.google.protobuf.CodedOutputStream output)
throws java.io.IOException {
if (term_ != 0L) {
output.writeUInt64(1, term_);
}
if (granted_ != false) {
output.writeBool(2, granted_);
}
}
public int getSerializedSize() {
int size = memoizedSize;
if (size != -1) return size;
size = 0;
if (term_ != 0L) {
size += com.google.protobuf.CodedOutputStream
.computeUInt64Size(1, term_);
}
if (granted_ != false) {
size += com.google.protobuf.CodedOutputStream
.computeBoolSize(2, granted_);
}
memoizedSize = size;
return size;
}
private static final long serialVersionUID = 0L;
@java.lang.Override
public boolean equals(final java.lang.Object obj) {
if (obj == this) {
return true;
}
if (!(obj instanceof com.github.wenweihu86.raft.proto.RaftMessage.VoteResponse)) {
return super.equals(obj);
}
com.github.wenweihu86.raft.proto.RaftMessage.VoteResponse other = (com.github.wenweihu86.raft.proto.RaftMessage.VoteResponse) obj;
boolean result = true;
result = result && (getTerm()
== other.getTerm());
result = result && (getGranted()
== other.getGranted());
return result;
}
@java.lang.Override
public int hashCode() {
if (memoizedHashCode != 0) {
return memoizedHashCode;
}
int hash = 41;
hash = (19 * hash) + getDescriptorForType().hashCode();
hash = (37 * hash) + TERM_FIELD_NUMBER;
hash = (53 * hash) + com.google.protobuf.Internal.hashLong(
getTerm());
hash = (37 * hash) + GRANTED_FIELD_NUMBER;
hash = (53 * hash) + com.google.protobuf.Internal.hashBoolean(
getGranted());
hash = (29 * hash) + unknownFields.hashCode();
memoizedHashCode = hash;
return hash;
}
public static com.github.wenweihu86.raft.proto.RaftMessage.VoteResponse parseFrom(
com.google.protobuf.ByteString data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static com.github.wenweihu86.raft.proto.RaftMessage.VoteResponse parseFrom(
com.google.protobuf.ByteString data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static com.github.wenweihu86.raft.proto.RaftMessage.VoteResponse parseFrom(byte[] data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static com.github.wenweihu86.raft.proto.RaftMessage.VoteResponse parseFrom(
byte[] data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static com.github.wenweihu86.raft.proto.RaftMessage.VoteResponse parseFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static com.github.wenweihu86.raft.proto.RaftMessage.VoteResponse parseFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
public static com.github.wenweihu86.raft.proto.RaftMessage.VoteResponse parseDelimitedFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input);
}
public static com.github.wenweihu86.raft.proto.RaftMessage.VoteResponse parseDelimitedFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input, extensionRegistry);
}
public static com.github.wenweihu86.raft.proto.RaftMessage.VoteResponse parseFrom(
com.google.protobuf.CodedInputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static com.github.wenweihu86.raft.proto.RaftMessage.VoteResponse parseFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
public Builder newBuilderForType() { return newBuilder(); }
public static Builder newBuilder() {
return DEFAULT_INSTANCE.toBuilder();
}
public static Builder newBuilder(com.github.wenweihu86.raft.proto.RaftMessage.VoteResponse prototype) {
return DEFAULT_INSTANCE.toBuilder().mergeFrom(prototype);
}
public Builder toBuilder() {
return this == DEFAULT_INSTANCE
? new Builder() : new Builder().mergeFrom(this);
}
@java.lang.Override
protected Builder newBuilderForType(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
Builder builder = new Builder(parent);
return builder;
}
/**
* Protobuf type {@code raft.VoteResponse}
*/
public static final class Builder extends
com.google.protobuf.GeneratedMessageV3.Builder implements
// @@protoc_insertion_point(builder_implements:raft.VoteResponse)
com.github.wenweihu86.raft.proto.RaftMessage.VoteResponseOrBuilder {
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return com.github.wenweihu86.raft.proto.RaftMessage.internal_static_raft_VoteResponse_descriptor;
}
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return com.github.wenweihu86.raft.proto.RaftMessage.internal_static_raft_VoteResponse_fieldAccessorTable
.ensureFieldAccessorsInitialized(
com.github.wenweihu86.raft.proto.RaftMessage.VoteResponse.class, com.github.wenweihu86.raft.proto.RaftMessage.VoteResponse.Builder.class);
}
// Construct using com.github.wenweihu86.raft.proto.RaftMessage.VoteResponse.newBuilder()
private Builder() {
maybeForceBuilderInitialization();
}
private Builder(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
super(parent);
maybeForceBuilderInitialization();
}
private void maybeForceBuilderInitialization() {
if (com.google.protobuf.GeneratedMessageV3
.alwaysUseFieldBuilders) {
}
}
public Builder clear() {
super.clear();
term_ = 0L;
granted_ = false;
return this;
}
public com.google.protobuf.Descriptors.Descriptor
getDescriptorForType() {
return com.github.wenweihu86.raft.proto.RaftMessage.internal_static_raft_VoteResponse_descriptor;
}
public com.github.wenweihu86.raft.proto.RaftMessage.VoteResponse getDefaultInstanceForType() {
return com.github.wenweihu86.raft.proto.RaftMessage.VoteResponse.getDefaultInstance();
}
public com.github.wenweihu86.raft.proto.RaftMessage.VoteResponse build() {
com.github.wenweihu86.raft.proto.RaftMessage.VoteResponse result = buildPartial();
if (!result.isInitialized()) {
throw newUninitializedMessageException(result);
}
return result;
}
public com.github.wenweihu86.raft.proto.RaftMessage.VoteResponse buildPartial() {
com.github.wenweihu86.raft.proto.RaftMessage.VoteResponse result = new com.github.wenweihu86.raft.proto.RaftMessage.VoteResponse(this);
result.term_ = term_;
result.granted_ = granted_;
onBuilt();
return result;
}
public Builder clone() {
return (Builder) super.clone();
}
public Builder setField(
com.google.protobuf.Descriptors.FieldDescriptor field,
Object value) {
return (Builder) super.setField(field, value);
}
public Builder clearField(
com.google.protobuf.Descriptors.FieldDescriptor field) {
return (Builder) super.clearField(field);
}
public Builder clearOneof(
com.google.protobuf.Descriptors.OneofDescriptor oneof) {
return (Builder) super.clearOneof(oneof);
}
public Builder setRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
int index, Object value) {
return (Builder) super.setRepeatedField(field, index, value);
}
public Builder addRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
Object value) {
return (Builder) super.addRepeatedField(field, value);
}
public Builder mergeFrom(com.google.protobuf.Message other) {
if (other instanceof com.github.wenweihu86.raft.proto.RaftMessage.VoteResponse) {
return mergeFrom((com.github.wenweihu86.raft.proto.RaftMessage.VoteResponse)other);
} else {
super.mergeFrom(other);
return this;
}
}
public Builder mergeFrom(com.github.wenweihu86.raft.proto.RaftMessage.VoteResponse other) {
if (other == com.github.wenweihu86.raft.proto.RaftMessage.VoteResponse.getDefaultInstance()) return this;
if (other.getTerm() != 0L) {
setTerm(other.getTerm());
}
if (other.getGranted() != false) {
setGranted(other.getGranted());
}
onChanged();
return this;
}
public final boolean isInitialized() {
return true;
}
public Builder mergeFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
com.github.wenweihu86.raft.proto.RaftMessage.VoteResponse parsedMessage = null;
try {
parsedMessage = PARSER.parsePartialFrom(input, extensionRegistry);
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
parsedMessage = (com.github.wenweihu86.raft.proto.RaftMessage.VoteResponse) e.getUnfinishedMessage();
throw e.unwrapIOException();
} finally {
if (parsedMessage != null) {
mergeFrom(parsedMessage);
}
}
return this;
}
private long term_ ;
/**
*
* 当前任期号,以便于候选人去更新自己的任期号
*
*
* optional uint64 term = 1;
*/
public long getTerm() {
return term_;
}
/**
*
* 当前任期号,以便于候选人去更新自己的任期号
*
*
* optional uint64 term = 1;
*/
public Builder setTerm(long value) {
term_ = value;
onChanged();
return this;
}
/**
*
* 当前任期号,以便于候选人去更新自己的任期号
*
*
* optional uint64 term = 1;
*/
public Builder clearTerm() {
term_ = 0L;
onChanged();
return this;
}
private boolean granted_ ;
/**
*
* 候选人赢得了此张选票时为真
*
*
* optional bool granted = 2;
*/
public boolean getGranted() {
return granted_;
}
/**
*
* 候选人赢得了此张选票时为真
*
*
* optional bool granted = 2;
*/
public Builder setGranted(boolean value) {
granted_ = value;
onChanged();
return this;
}
/**
*
* 候选人赢得了此张选票时为真
*
*
* optional bool granted = 2;
*/
public Builder clearGranted() {
granted_ = false;
onChanged();
return this;
}
public final Builder setUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return this;
}
public final Builder mergeUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return this;
}
// @@protoc_insertion_point(builder_scope:raft.VoteResponse)
}
// @@protoc_insertion_point(class_scope:raft.VoteResponse)
private static final com.github.wenweihu86.raft.proto.RaftMessage.VoteResponse DEFAULT_INSTANCE;
static {
DEFAULT_INSTANCE = new com.github.wenweihu86.raft.proto.RaftMessage.VoteResponse();
}
public static com.github.wenweihu86.raft.proto.RaftMessage.VoteResponse getDefaultInstance() {
return DEFAULT_INSTANCE;
}
private static final com.google.protobuf.Parser
PARSER = new com.google.protobuf.AbstractParser() {
public VoteResponse parsePartialFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return new VoteResponse(input, extensionRegistry);
}
};
public static com.google.protobuf.Parser parser() {
return PARSER;
}
@java.lang.Override
public com.google.protobuf.Parser getParserForType() {
return PARSER;
}
public com.github.wenweihu86.raft.proto.RaftMessage.VoteResponse getDefaultInstanceForType() {
return DEFAULT_INSTANCE;
}
}
public interface AppendEntriesRequestOrBuilder extends
// @@protoc_insertion_point(interface_extends:raft.AppendEntriesRequest)
com.google.protobuf.MessageOrBuilder {
/**
*
* 领导人的Id
*
*
* optional uint32 server_id = 1;
*/
int getServerId();
/**
*
* 领导人的任期号
*
*
* optional uint64 term = 2;
*/
long getTerm();
/**
*
* 新的日志条目紧随之前的索引值
*
*
* optional uint64 prev_log_index = 3;
*/
long getPrevLogIndex();
/**
*
* prev_log_index条目的任期号
*
*
* optional uint64 prev_log_term = 4;
*/
long getPrevLogTerm();
/**
*
* 准备存储的日志条目(表示心跳时为空)
*
*
* repeated .raft.LogEntry entries = 5;
*/
java.util.List
getEntriesList();
/**
*
* 准备存储的日志条目(表示心跳时为空)
*
*
* repeated .raft.LogEntry entries = 5;
*/
com.github.wenweihu86.raft.proto.RaftMessage.LogEntry getEntries(int index);
/**
*
* 准备存储的日志条目(表示心跳时为空)
*
*
* repeated .raft.LogEntry entries = 5;
*/
int getEntriesCount();
/**
*
* 准备存储的日志条目(表示心跳时为空)
*
*
* repeated .raft.LogEntry entries = 5;
*/
java.util.List extends com.github.wenweihu86.raft.proto.RaftMessage.LogEntryOrBuilder>
getEntriesOrBuilderList();
/**
*
* 准备存储的日志条目(表示心跳时为空)
*
*
* repeated .raft.LogEntry entries = 5;
*/
com.github.wenweihu86.raft.proto.RaftMessage.LogEntryOrBuilder getEntriesOrBuilder(
int index);
/**
*
* 领导人已经提交的日志的索引值
*
*
* optional uint64 commit_index = 6;
*/
long getCommitIndex();
}
/**
* Protobuf type {@code raft.AppendEntriesRequest}
*/
public static final class AppendEntriesRequest extends
com.google.protobuf.GeneratedMessageV3 implements
// @@protoc_insertion_point(message_implements:raft.AppendEntriesRequest)
AppendEntriesRequestOrBuilder {
// Use AppendEntriesRequest.newBuilder() to construct.
private AppendEntriesRequest(com.google.protobuf.GeneratedMessageV3.Builder> builder) {
super(builder);
}
private AppendEntriesRequest() {
serverId_ = 0;
term_ = 0L;
prevLogIndex_ = 0L;
prevLogTerm_ = 0L;
entries_ = java.util.Collections.emptyList();
commitIndex_ = 0L;
}
@java.lang.Override
public final com.google.protobuf.UnknownFieldSet
getUnknownFields() {
return com.google.protobuf.UnknownFieldSet.getDefaultInstance();
}
private AppendEntriesRequest(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
this();
int mutable_bitField0_ = 0;
try {
boolean done = false;
while (!done) {
int tag = input.readTag();
switch (tag) {
case 0:
done = true;
break;
default: {
if (!input.skipField(tag)) {
done = true;
}
break;
}
case 8: {
serverId_ = input.readUInt32();
break;
}
case 16: {
term_ = input.readUInt64();
break;
}
case 24: {
prevLogIndex_ = input.readUInt64();
break;
}
case 32: {
prevLogTerm_ = input.readUInt64();
break;
}
case 42: {
if (!((mutable_bitField0_ & 0x00000010) == 0x00000010)) {
entries_ = new java.util.ArrayList();
mutable_bitField0_ |= 0x00000010;
}
entries_.add(
input.readMessage(com.github.wenweihu86.raft.proto.RaftMessage.LogEntry.parser(), extensionRegistry));
break;
}
case 48: {
commitIndex_ = input.readUInt64();
break;
}
}
}
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.setUnfinishedMessage(this);
} catch (java.io.IOException e) {
throw new com.google.protobuf.InvalidProtocolBufferException(
e).setUnfinishedMessage(this);
} finally {
if (((mutable_bitField0_ & 0x00000010) == 0x00000010)) {
entries_ = java.util.Collections.unmodifiableList(entries_);
}
makeExtensionsImmutable();
}
}
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return com.github.wenweihu86.raft.proto.RaftMessage.internal_static_raft_AppendEntriesRequest_descriptor;
}
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return com.github.wenweihu86.raft.proto.RaftMessage.internal_static_raft_AppendEntriesRequest_fieldAccessorTable
.ensureFieldAccessorsInitialized(
com.github.wenweihu86.raft.proto.RaftMessage.AppendEntriesRequest.class, com.github.wenweihu86.raft.proto.RaftMessage.AppendEntriesRequest.Builder.class);
}
private int bitField0_;
public static final int SERVER_ID_FIELD_NUMBER = 1;
private int serverId_;
/**
*
* 领导人的Id
*
*
* optional uint32 server_id = 1;
*/
public int getServerId() {
return serverId_;
}
public static final int TERM_FIELD_NUMBER = 2;
private long term_;
/**
*
* 领导人的任期号
*
*
* optional uint64 term = 2;
*/
public long getTerm() {
return term_;
}
public static final int PREV_LOG_INDEX_FIELD_NUMBER = 3;
private long prevLogIndex_;
/**
*
* 新的日志条目紧随之前的索引值
*
*
* optional uint64 prev_log_index = 3;
*/
public long getPrevLogIndex() {
return prevLogIndex_;
}
public static final int PREV_LOG_TERM_FIELD_NUMBER = 4;
private long prevLogTerm_;
/**
*
* prev_log_index条目的任期号
*
*
* optional uint64 prev_log_term = 4;
*/
public long getPrevLogTerm() {
return prevLogTerm_;
}
public static final int ENTRIES_FIELD_NUMBER = 5;
private java.util.List entries_;
/**
*
* 准备存储的日志条目(表示心跳时为空)
*
*
* repeated .raft.LogEntry entries = 5;
*/
public java.util.List getEntriesList() {
return entries_;
}
/**
*
* 准备存储的日志条目(表示心跳时为空)
*
*
* repeated .raft.LogEntry entries = 5;
*/
public java.util.List extends com.github.wenweihu86.raft.proto.RaftMessage.LogEntryOrBuilder>
getEntriesOrBuilderList() {
return entries_;
}
/**
*
* 准备存储的日志条目(表示心跳时为空)
*
*
* repeated .raft.LogEntry entries = 5;
*/
public int getEntriesCount() {
return entries_.size();
}
/**
*
* 准备存储的日志条目(表示心跳时为空)
*
*
* repeated .raft.LogEntry entries = 5;
*/
public com.github.wenweihu86.raft.proto.RaftMessage.LogEntry getEntries(int index) {
return entries_.get(index);
}
/**
*
* 准备存储的日志条目(表示心跳时为空)
*
*
* repeated .raft.LogEntry entries = 5;
*/
public com.github.wenweihu86.raft.proto.RaftMessage.LogEntryOrBuilder getEntriesOrBuilder(
int index) {
return entries_.get(index);
}
public static final int COMMIT_INDEX_FIELD_NUMBER = 6;
private long commitIndex_;
/**
*
* 领导人已经提交的日志的索引值
*
*
* optional uint64 commit_index = 6;
*/
public long getCommitIndex() {
return commitIndex_;
}
private byte memoizedIsInitialized = -1;
public final boolean isInitialized() {
byte isInitialized = memoizedIsInitialized;
if (isInitialized == 1) return true;
if (isInitialized == 0) return false;
memoizedIsInitialized = 1;
return true;
}
public void writeTo(com.google.protobuf.CodedOutputStream output)
throws java.io.IOException {
if (serverId_ != 0) {
output.writeUInt32(1, serverId_);
}
if (term_ != 0L) {
output.writeUInt64(2, term_);
}
if (prevLogIndex_ != 0L) {
output.writeUInt64(3, prevLogIndex_);
}
if (prevLogTerm_ != 0L) {
output.writeUInt64(4, prevLogTerm_);
}
for (int i = 0; i < entries_.size(); i++) {
output.writeMessage(5, entries_.get(i));
}
if (commitIndex_ != 0L) {
output.writeUInt64(6, commitIndex_);
}
}
public int getSerializedSize() {
int size = memoizedSize;
if (size != -1) return size;
size = 0;
if (serverId_ != 0) {
size += com.google.protobuf.CodedOutputStream
.computeUInt32Size(1, serverId_);
}
if (term_ != 0L) {
size += com.google.protobuf.CodedOutputStream
.computeUInt64Size(2, term_);
}
if (prevLogIndex_ != 0L) {
size += com.google.protobuf.CodedOutputStream
.computeUInt64Size(3, prevLogIndex_);
}
if (prevLogTerm_ != 0L) {
size += com.google.protobuf.CodedOutputStream
.computeUInt64Size(4, prevLogTerm_);
}
for (int i = 0; i < entries_.size(); i++) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(5, entries_.get(i));
}
if (commitIndex_ != 0L) {
size += com.google.protobuf.CodedOutputStream
.computeUInt64Size(6, commitIndex_);
}
memoizedSize = size;
return size;
}
private static final long serialVersionUID = 0L;
@java.lang.Override
public boolean equals(final java.lang.Object obj) {
if (obj == this) {
return true;
}
if (!(obj instanceof com.github.wenweihu86.raft.proto.RaftMessage.AppendEntriesRequest)) {
return super.equals(obj);
}
com.github.wenweihu86.raft.proto.RaftMessage.AppendEntriesRequest other = (com.github.wenweihu86.raft.proto.RaftMessage.AppendEntriesRequest) obj;
boolean result = true;
result = result && (getServerId()
== other.getServerId());
result = result && (getTerm()
== other.getTerm());
result = result && (getPrevLogIndex()
== other.getPrevLogIndex());
result = result && (getPrevLogTerm()
== other.getPrevLogTerm());
result = result && getEntriesList()
.equals(other.getEntriesList());
result = result && (getCommitIndex()
== other.getCommitIndex());
return result;
}
@java.lang.Override
public int hashCode() {
if (memoizedHashCode != 0) {
return memoizedHashCode;
}
int hash = 41;
hash = (19 * hash) + getDescriptorForType().hashCode();
hash = (37 * hash) + SERVER_ID_FIELD_NUMBER;
hash = (53 * hash) + getServerId();
hash = (37 * hash) + TERM_FIELD_NUMBER;
hash = (53 * hash) + com.google.protobuf.Internal.hashLong(
getTerm());
hash = (37 * hash) + PREV_LOG_INDEX_FIELD_NUMBER;
hash = (53 * hash) + com.google.protobuf.Internal.hashLong(
getPrevLogIndex());
hash = (37 * hash) + PREV_LOG_TERM_FIELD_NUMBER;
hash = (53 * hash) + com.google.protobuf.Internal.hashLong(
getPrevLogTerm());
if (getEntriesCount() > 0) {
hash = (37 * hash) + ENTRIES_FIELD_NUMBER;
hash = (53 * hash) + getEntriesList().hashCode();
}
hash = (37 * hash) + COMMIT_INDEX_FIELD_NUMBER;
hash = (53 * hash) + com.google.protobuf.Internal.hashLong(
getCommitIndex());
hash = (29 * hash) + unknownFields.hashCode();
memoizedHashCode = hash;
return hash;
}
public static com.github.wenweihu86.raft.proto.RaftMessage.AppendEntriesRequest parseFrom(
com.google.protobuf.ByteString data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static com.github.wenweihu86.raft.proto.RaftMessage.AppendEntriesRequest parseFrom(
com.google.protobuf.ByteString data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static com.github.wenweihu86.raft.proto.RaftMessage.AppendEntriesRequest parseFrom(byte[] data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static com.github.wenweihu86.raft.proto.RaftMessage.AppendEntriesRequest parseFrom(
byte[] data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static com.github.wenweihu86.raft.proto.RaftMessage.AppendEntriesRequest parseFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static com.github.wenweihu86.raft.proto.RaftMessage.AppendEntriesRequest parseFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
public static com.github.wenweihu86.raft.proto.RaftMessage.AppendEntriesRequest parseDelimitedFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input);
}
public static com.github.wenweihu86.raft.proto.RaftMessage.AppendEntriesRequest parseDelimitedFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input, extensionRegistry);
}
public static com.github.wenweihu86.raft.proto.RaftMessage.AppendEntriesRequest parseFrom(
com.google.protobuf.CodedInputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static com.github.wenweihu86.raft.proto.RaftMessage.AppendEntriesRequest parseFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
public Builder newBuilderForType() { return newBuilder(); }
public static Builder newBuilder() {
return DEFAULT_INSTANCE.toBuilder();
}
public static Builder newBuilder(com.github.wenweihu86.raft.proto.RaftMessage.AppendEntriesRequest prototype) {
return DEFAULT_INSTANCE.toBuilder().mergeFrom(prototype);
}
public Builder toBuilder() {
return this == DEFAULT_INSTANCE
? new Builder() : new Builder().mergeFrom(this);
}
@java.lang.Override
protected Builder newBuilderForType(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
Builder builder = new Builder(parent);
return builder;
}
/**
* Protobuf type {@code raft.AppendEntriesRequest}
*/
public static final class Builder extends
com.google.protobuf.GeneratedMessageV3.Builder implements
// @@protoc_insertion_point(builder_implements:raft.AppendEntriesRequest)
com.github.wenweihu86.raft.proto.RaftMessage.AppendEntriesRequestOrBuilder {
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return com.github.wenweihu86.raft.proto.RaftMessage.internal_static_raft_AppendEntriesRequest_descriptor;
}
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return com.github.wenweihu86.raft.proto.RaftMessage.internal_static_raft_AppendEntriesRequest_fieldAccessorTable
.ensureFieldAccessorsInitialized(
com.github.wenweihu86.raft.proto.RaftMessage.AppendEntriesRequest.class, com.github.wenweihu86.raft.proto.RaftMessage.AppendEntriesRequest.Builder.class);
}
// Construct using com.github.wenweihu86.raft.proto.RaftMessage.AppendEntriesRequest.newBuilder()
private Builder() {
maybeForceBuilderInitialization();
}
private Builder(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
super(parent);
maybeForceBuilderInitialization();
}
private void maybeForceBuilderInitialization() {
if (com.google.protobuf.GeneratedMessageV3
.alwaysUseFieldBuilders) {
getEntriesFieldBuilder();
}
}
public Builder clear() {
super.clear();
serverId_ = 0;
term_ = 0L;
prevLogIndex_ = 0L;
prevLogTerm_ = 0L;
if (entriesBuilder_ == null) {
entries_ = java.util.Collections.emptyList();
bitField0_ = (bitField0_ & ~0x00000010);
} else {
entriesBuilder_.clear();
}
commitIndex_ = 0L;
return this;
}
public com.google.protobuf.Descriptors.Descriptor
getDescriptorForType() {
return com.github.wenweihu86.raft.proto.RaftMessage.internal_static_raft_AppendEntriesRequest_descriptor;
}
public com.github.wenweihu86.raft.proto.RaftMessage.AppendEntriesRequest getDefaultInstanceForType() {
return com.github.wenweihu86.raft.proto.RaftMessage.AppendEntriesRequest.getDefaultInstance();
}
public com.github.wenweihu86.raft.proto.RaftMessage.AppendEntriesRequest build() {
com.github.wenweihu86.raft.proto.RaftMessage.AppendEntriesRequest result = buildPartial();
if (!result.isInitialized()) {
throw newUninitializedMessageException(result);
}
return result;
}
public com.github.wenweihu86.raft.proto.RaftMessage.AppendEntriesRequest buildPartial() {
com.github.wenweihu86.raft.proto.RaftMessage.AppendEntriesRequest result = new com.github.wenweihu86.raft.proto.RaftMessage.AppendEntriesRequest(this);
int from_bitField0_ = bitField0_;
int to_bitField0_ = 0;
result.serverId_ = serverId_;
result.term_ = term_;
result.prevLogIndex_ = prevLogIndex_;
result.prevLogTerm_ = prevLogTerm_;
if (entriesBuilder_ == null) {
if (((bitField0_ & 0x00000010) == 0x00000010)) {
entries_ = java.util.Collections.unmodifiableList(entries_);
bitField0_ = (bitField0_ & ~0x00000010);
}
result.entries_ = entries_;
} else {
result.entries_ = entriesBuilder_.build();
}
result.commitIndex_ = commitIndex_;
result.bitField0_ = to_bitField0_;
onBuilt();
return result;
}
public Builder clone() {
return (Builder) super.clone();
}
public Builder setField(
com.google.protobuf.Descriptors.FieldDescriptor field,
Object value) {
return (Builder) super.setField(field, value);
}
public Builder clearField(
com.google.protobuf.Descriptors.FieldDescriptor field) {
return (Builder) super.clearField(field);
}
public Builder clearOneof(
com.google.protobuf.Descriptors.OneofDescriptor oneof) {
return (Builder) super.clearOneof(oneof);
}
public Builder setRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
int index, Object value) {
return (Builder) super.setRepeatedField(field, index, value);
}
public Builder addRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
Object value) {
return (Builder) super.addRepeatedField(field, value);
}
public Builder mergeFrom(com.google.protobuf.Message other) {
if (other instanceof com.github.wenweihu86.raft.proto.RaftMessage.AppendEntriesRequest) {
return mergeFrom((com.github.wenweihu86.raft.proto.RaftMessage.AppendEntriesRequest)other);
} else {
super.mergeFrom(other);
return this;
}
}
public Builder mergeFrom(com.github.wenweihu86.raft.proto.RaftMessage.AppendEntriesRequest other) {
if (other == com.github.wenweihu86.raft.proto.RaftMessage.AppendEntriesRequest.getDefaultInstance()) return this;
if (other.getServerId() != 0) {
setServerId(other.getServerId());
}
if (other.getTerm() != 0L) {
setTerm(other.getTerm());
}
if (other.getPrevLogIndex() != 0L) {
setPrevLogIndex(other.getPrevLogIndex());
}
if (other.getPrevLogTerm() != 0L) {
setPrevLogTerm(other.getPrevLogTerm());
}
if (entriesBuilder_ == null) {
if (!other.entries_.isEmpty()) {
if (entries_.isEmpty()) {
entries_ = other.entries_;
bitField0_ = (bitField0_ & ~0x00000010);
} else {
ensureEntriesIsMutable();
entries_.addAll(other.entries_);
}
onChanged();
}
} else {
if (!other.entries_.isEmpty()) {
if (entriesBuilder_.isEmpty()) {
entriesBuilder_.dispose();
entriesBuilder_ = null;
entries_ = other.entries_;
bitField0_ = (bitField0_ & ~0x00000010);
entriesBuilder_ =
com.google.protobuf.GeneratedMessageV3.alwaysUseFieldBuilders ?
getEntriesFieldBuilder() : null;
} else {
entriesBuilder_.addAllMessages(other.entries_);
}
}
}
if (other.getCommitIndex() != 0L) {
setCommitIndex(other.getCommitIndex());
}
onChanged();
return this;
}
public final boolean isInitialized() {
return true;
}
public Builder mergeFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
com.github.wenweihu86.raft.proto.RaftMessage.AppendEntriesRequest parsedMessage = null;
try {
parsedMessage = PARSER.parsePartialFrom(input, extensionRegistry);
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
parsedMessage = (com.github.wenweihu86.raft.proto.RaftMessage.AppendEntriesRequest) e.getUnfinishedMessage();
throw e.unwrapIOException();
} finally {
if (parsedMessage != null) {
mergeFrom(parsedMessage);
}
}
return this;
}
private int bitField0_;
private int serverId_ ;
/**
*
* 领导人的Id
*
*
* optional uint32 server_id = 1;
*/
public int getServerId() {
return serverId_;
}
/**
*
* 领导人的Id
*
*
* optional uint32 server_id = 1;
*/
public Builder setServerId(int value) {
serverId_ = value;
onChanged();
return this;
}
/**
*
* 领导人的Id
*
*
* optional uint32 server_id = 1;
*/
public Builder clearServerId() {
serverId_ = 0;
onChanged();
return this;
}
private long term_ ;
/**
*
* 领导人的任期号
*
*
* optional uint64 term = 2;
*/
public long getTerm() {
return term_;
}
/**
*
* 领导人的任期号
*
*
* optional uint64 term = 2;
*/
public Builder setTerm(long value) {
term_ = value;
onChanged();
return this;
}
/**
*
* 领导人的任期号
*
*
* optional uint64 term = 2;
*/
public Builder clearTerm() {
term_ = 0L;
onChanged();
return this;
}
private long prevLogIndex_ ;
/**
*
* 新的日志条目紧随之前的索引值
*
*
* optional uint64 prev_log_index = 3;
*/
public long getPrevLogIndex() {
return prevLogIndex_;
}
/**
*
* 新的日志条目紧随之前的索引值
*
*
* optional uint64 prev_log_index = 3;
*/
public Builder setPrevLogIndex(long value) {
prevLogIndex_ = value;
onChanged();
return this;
}
/**
*
* 新的日志条目紧随之前的索引值
*
*
* optional uint64 prev_log_index = 3;
*/
public Builder clearPrevLogIndex() {
prevLogIndex_ = 0L;
onChanged();
return this;
}
private long prevLogTerm_ ;
/**
*
* prev_log_index条目的任期号
*
*
* optional uint64 prev_log_term = 4;
*/
public long getPrevLogTerm() {
return prevLogTerm_;
}
/**
*
* prev_log_index条目的任期号
*
*
* optional uint64 prev_log_term = 4;
*/
public Builder setPrevLogTerm(long value) {
prevLogTerm_ = value;
onChanged();
return this;
}
/**
*
* prev_log_index条目的任期号
*
*
* optional uint64 prev_log_term = 4;
*/
public Builder clearPrevLogTerm() {
prevLogTerm_ = 0L;
onChanged();
return this;
}
private java.util.List entries_ =
java.util.Collections.emptyList();
private void ensureEntriesIsMutable() {
if (!((bitField0_ & 0x00000010) == 0x00000010)) {
entries_ = new java.util.ArrayList(entries_);
bitField0_ |= 0x00000010;
}
}
private com.google.protobuf.RepeatedFieldBuilderV3<
com.github.wenweihu86.raft.proto.RaftMessage.LogEntry, com.github.wenweihu86.raft.proto.RaftMessage.LogEntry.Builder, com.github.wenweihu86.raft.proto.RaftMessage.LogEntryOrBuilder> entriesBuilder_;
/**
*
* 准备存储的日志条目(表示心跳时为空)
*
*
* repeated .raft.LogEntry entries = 5;
*/
public java.util.List getEntriesList() {
if (entriesBuilder_ == null) {
return java.util.Collections.unmodifiableList(entries_);
} else {
return entriesBuilder_.getMessageList();
}
}
/**
*
* 准备存储的日志条目(表示心跳时为空)
*
*
* repeated .raft.LogEntry entries = 5;
*/
public int getEntriesCount() {
if (entriesBuilder_ == null) {
return entries_.size();
} else {
return entriesBuilder_.getCount();
}
}
/**
*
* 准备存储的日志条目(表示心跳时为空)
*
*
* repeated .raft.LogEntry entries = 5;
*/
public com.github.wenweihu86.raft.proto.RaftMessage.LogEntry getEntries(int index) {
if (entriesBuilder_ == null) {
return entries_.get(index);
} else {
return entriesBuilder_.getMessage(index);
}
}
/**
*
* 准备存储的日志条目(表示心跳时为空)
*
*
* repeated .raft.LogEntry entries = 5;
*/
public Builder setEntries(
int index, com.github.wenweihu86.raft.proto.RaftMessage.LogEntry value) {
if (entriesBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
ensureEntriesIsMutable();
entries_.set(index, value);
onChanged();
} else {
entriesBuilder_.setMessage(index, value);
}
return this;
}
/**
*
* 准备存储的日志条目(表示心跳时为空)
*
*
* repeated .raft.LogEntry entries = 5;
*/
public Builder setEntries(
int index, com.github.wenweihu86.raft.proto.RaftMessage.LogEntry.Builder builderForValue) {
if (entriesBuilder_ == null) {
ensureEntriesIsMutable();
entries_.set(index, builderForValue.build());
onChanged();
} else {
entriesBuilder_.setMessage(index, builderForValue.build());
}
return this;
}
/**
*
* 准备存储的日志条目(表示心跳时为空)
*
*
* repeated .raft.LogEntry entries = 5;
*/
public Builder addEntries(com.github.wenweihu86.raft.proto.RaftMessage.LogEntry value) {
if (entriesBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
ensureEntriesIsMutable();
entries_.add(value);
onChanged();
} else {
entriesBuilder_.addMessage(value);
}
return this;
}
/**
*
* 准备存储的日志条目(表示心跳时为空)
*
*
* repeated .raft.LogEntry entries = 5;
*/
public Builder addEntries(
int index, com.github.wenweihu86.raft.proto.RaftMessage.LogEntry value) {
if (entriesBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
ensureEntriesIsMutable();
entries_.add(index, value);
onChanged();
} else {
entriesBuilder_.addMessage(index, value);
}
return this;
}
/**
*
* 准备存储的日志条目(表示心跳时为空)
*
*
* repeated .raft.LogEntry entries = 5;
*/
public Builder addEntries(
com.github.wenweihu86.raft.proto.RaftMessage.LogEntry.Builder builderForValue) {
if (entriesBuilder_ == null) {
ensureEntriesIsMutable();
entries_.add(builderForValue.build());
onChanged();
} else {
entriesBuilder_.addMessage(builderForValue.build());
}
return this;
}
/**
*
* 准备存储的日志条目(表示心跳时为空)
*
*
* repeated .raft.LogEntry entries = 5;
*/
public Builder addEntries(
int index, com.github.wenweihu86.raft.proto.RaftMessage.LogEntry.Builder builderForValue) {
if (entriesBuilder_ == null) {
ensureEntriesIsMutable();
entries_.add(index, builderForValue.build());
onChanged();
} else {
entriesBuilder_.addMessage(index, builderForValue.build());
}
return this;
}
/**
*
* 准备存储的日志条目(表示心跳时为空)
*
*
* repeated .raft.LogEntry entries = 5;
*/
public Builder addAllEntries(
java.lang.Iterable extends com.github.wenweihu86.raft.proto.RaftMessage.LogEntry> values) {
if (entriesBuilder_ == null) {
ensureEntriesIsMutable();
com.google.protobuf.AbstractMessageLite.Builder.addAll(
values, entries_);
onChanged();
} else {
entriesBuilder_.addAllMessages(values);
}
return this;
}
/**
*
* 准备存储的日志条目(表示心跳时为空)
*
*
* repeated .raft.LogEntry entries = 5;
*/
public Builder clearEntries() {
if (entriesBuilder_ == null) {
entries_ = java.util.Collections.emptyList();
bitField0_ = (bitField0_ & ~0x00000010);
onChanged();
} else {
entriesBuilder_.clear();
}
return this;
}
/**
*
* 准备存储的日志条目(表示心跳时为空)
*
*
* repeated .raft.LogEntry entries = 5;
*/
public Builder removeEntries(int index) {
if (entriesBuilder_ == null) {
ensureEntriesIsMutable();
entries_.remove(index);
onChanged();
} else {
entriesBuilder_.remove(index);
}
return this;
}
/**
*
* 准备存储的日志条目(表示心跳时为空)
*
*
* repeated .raft.LogEntry entries = 5;
*/
public com.github.wenweihu86.raft.proto.RaftMessage.LogEntry.Builder getEntriesBuilder(
int index) {
return getEntriesFieldBuilder().getBuilder(index);
}
/**
*
* 准备存储的日志条目(表示心跳时为空)
*
*
* repeated .raft.LogEntry entries = 5;
*/
public com.github.wenweihu86.raft.proto.RaftMessage.LogEntryOrBuilder getEntriesOrBuilder(
int index) {
if (entriesBuilder_ == null) {
return entries_.get(index); } else {
return entriesBuilder_.getMessageOrBuilder(index);
}
}
/**
*
* 准备存储的日志条目(表示心跳时为空)
*
*
* repeated .raft.LogEntry entries = 5;
*/
public java.util.List extends com.github.wenweihu86.raft.proto.RaftMessage.LogEntryOrBuilder>
getEntriesOrBuilderList() {
if (entriesBuilder_ != null) {
return entriesBuilder_.getMessageOrBuilderList();
} else {
return java.util.Collections.unmodifiableList(entries_);
}
}
/**
*
* 准备存储的日志条目(表示心跳时为空)
*
*
* repeated .raft.LogEntry entries = 5;
*/
public com.github.wenweihu86.raft.proto.RaftMessage.LogEntry.Builder addEntriesBuilder() {
return getEntriesFieldBuilder().addBuilder(
com.github.wenweihu86.raft.proto.RaftMessage.LogEntry.getDefaultInstance());
}
/**
*
* 准备存储的日志条目(表示心跳时为空)
*
*
* repeated .raft.LogEntry entries = 5;
*/
public com.github.wenweihu86.raft.proto.RaftMessage.LogEntry.Builder addEntriesBuilder(
int index) {
return getEntriesFieldBuilder().addBuilder(
index, com.github.wenweihu86.raft.proto.RaftMessage.LogEntry.getDefaultInstance());
}
/**
*
* 准备存储的日志条目(表示心跳时为空)
*
*
* repeated .raft.LogEntry entries = 5;
*/
public java.util.List
getEntriesBuilderList() {
return getEntriesFieldBuilder().getBuilderList();
}
private com.google.protobuf.RepeatedFieldBuilderV3<
com.github.wenweihu86.raft.proto.RaftMessage.LogEntry, com.github.wenweihu86.raft.proto.RaftMessage.LogEntry.Builder, com.github.wenweihu86.raft.proto.RaftMessage.LogEntryOrBuilder>
getEntriesFieldBuilder() {
if (entriesBuilder_ == null) {
entriesBuilder_ = new com.google.protobuf.RepeatedFieldBuilderV3<
com.github.wenweihu86.raft.proto.RaftMessage.LogEntry, com.github.wenweihu86.raft.proto.RaftMessage.LogEntry.Builder, com.github.wenweihu86.raft.proto.RaftMessage.LogEntryOrBuilder>(
entries_,
((bitField0_ & 0x00000010) == 0x00000010),
getParentForChildren(),
isClean());
entries_ = null;
}
return entriesBuilder_;
}
private long commitIndex_ ;
/**
*
* 领导人已经提交的日志的索引值
*
*
* optional uint64 commit_index = 6;
*/
public long getCommitIndex() {
return commitIndex_;
}
/**
*
* 领导人已经提交的日志的索引值
*
*
* optional uint64 commit_index = 6;
*/
public Builder setCommitIndex(long value) {
commitIndex_ = value;
onChanged();
return this;
}
/**
*
* 领导人已经提交的日志的索引值
*
*
* optional uint64 commit_index = 6;
*/
public Builder clearCommitIndex() {
commitIndex_ = 0L;
onChanged();
return this;
}
public final Builder setUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return this;
}
public final Builder mergeUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return this;
}
// @@protoc_insertion_point(builder_scope:raft.AppendEntriesRequest)
}
// @@protoc_insertion_point(class_scope:raft.AppendEntriesRequest)
private static final com.github.wenweihu86.raft.proto.RaftMessage.AppendEntriesRequest DEFAULT_INSTANCE;
static {
DEFAULT_INSTANCE = new com.github.wenweihu86.raft.proto.RaftMessage.AppendEntriesRequest();
}
public static com.github.wenweihu86.raft.proto.RaftMessage.AppendEntriesRequest getDefaultInstance() {
return DEFAULT_INSTANCE;
}
private static final com.google.protobuf.Parser
PARSER = new com.google.protobuf.AbstractParser() {
public AppendEntriesRequest parsePartialFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return new AppendEntriesRequest(input, extensionRegistry);
}
};
public static com.google.protobuf.Parser parser() {
return PARSER;
}
@java.lang.Override
public com.google.protobuf.Parser getParserForType() {
return PARSER;
}
public com.github.wenweihu86.raft.proto.RaftMessage.AppendEntriesRequest getDefaultInstanceForType() {
return DEFAULT_INSTANCE;
}
}
public interface AppendEntriesResponseOrBuilder extends
// @@protoc_insertion_point(interface_extends:raft.AppendEntriesResponse)
com.google.protobuf.MessageOrBuilder {
/**
*
* 跟随者包含了匹配上 prevLogIndex 和 prevLogTerm 的日志时为真
*
*
* optional .raft.ResCode res_code = 1;
*/
int getResCodeValue();
/**
*
* 跟随者包含了匹配上 prevLogIndex 和 prevLogTerm 的日志时为真
*
*
* optional .raft.ResCode res_code = 1;
*/
com.github.wenweihu86.raft.proto.RaftMessage.ResCode getResCode();
/**
*
* 当前的任期号,用于领导人去更新自己
*
*
* optional uint64 term = 2;
*/
long getTerm();
/**
* optional uint64 last_log_index = 3;
*/
long getLastLogIndex();
}
/**
* Protobuf type {@code raft.AppendEntriesResponse}
*/
public static final class AppendEntriesResponse extends
com.google.protobuf.GeneratedMessageV3 implements
// @@protoc_insertion_point(message_implements:raft.AppendEntriesResponse)
AppendEntriesResponseOrBuilder {
// Use AppendEntriesResponse.newBuilder() to construct.
private AppendEntriesResponse(com.google.protobuf.GeneratedMessageV3.Builder> builder) {
super(builder);
}
private AppendEntriesResponse() {
resCode_ = 0;
term_ = 0L;
lastLogIndex_ = 0L;
}
@java.lang.Override
public final com.google.protobuf.UnknownFieldSet
getUnknownFields() {
return com.google.protobuf.UnknownFieldSet.getDefaultInstance();
}
private AppendEntriesResponse(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
this();
int mutable_bitField0_ = 0;
try {
boolean done = false;
while (!done) {
int tag = input.readTag();
switch (tag) {
case 0:
done = true;
break;
default: {
if (!input.skipField(tag)) {
done = true;
}
break;
}
case 8: {
int rawValue = input.readEnum();
resCode_ = rawValue;
break;
}
case 16: {
term_ = input.readUInt64();
break;
}
case 24: {
lastLogIndex_ = input.readUInt64();
break;
}
}
}
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.setUnfinishedMessage(this);
} catch (java.io.IOException e) {
throw new com.google.protobuf.InvalidProtocolBufferException(
e).setUnfinishedMessage(this);
} finally {
makeExtensionsImmutable();
}
}
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return com.github.wenweihu86.raft.proto.RaftMessage.internal_static_raft_AppendEntriesResponse_descriptor;
}
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return com.github.wenweihu86.raft.proto.RaftMessage.internal_static_raft_AppendEntriesResponse_fieldAccessorTable
.ensureFieldAccessorsInitialized(
com.github.wenweihu86.raft.proto.RaftMessage.AppendEntriesResponse.class, com.github.wenweihu86.raft.proto.RaftMessage.AppendEntriesResponse.Builder.class);
}
public static final int RES_CODE_FIELD_NUMBER = 1;
private int resCode_;
/**
*
* 跟随者包含了匹配上 prevLogIndex 和 prevLogTerm 的日志时为真
*
*
* optional .raft.ResCode res_code = 1;
*/
public int getResCodeValue() {
return resCode_;
}
/**
*
* 跟随者包含了匹配上 prevLogIndex 和 prevLogTerm 的日志时为真
*
*
* optional .raft.ResCode res_code = 1;
*/
public com.github.wenweihu86.raft.proto.RaftMessage.ResCode getResCode() {
com.github.wenweihu86.raft.proto.RaftMessage.ResCode result = com.github.wenweihu86.raft.proto.RaftMessage.ResCode.valueOf(resCode_);
return result == null ? com.github.wenweihu86.raft.proto.RaftMessage.ResCode.UNRECOGNIZED : result;
}
public static final int TERM_FIELD_NUMBER = 2;
private long term_;
/**
*
* 当前的任期号,用于领导人去更新自己
*
*
* optional uint64 term = 2;
*/
public long getTerm() {
return term_;
}
public static final int LAST_LOG_INDEX_FIELD_NUMBER = 3;
private long lastLogIndex_;
/**
* optional uint64 last_log_index = 3;
*/
public long getLastLogIndex() {
return lastLogIndex_;
}
private byte memoizedIsInitialized = -1;
public final boolean isInitialized() {
byte isInitialized = memoizedIsInitialized;
if (isInitialized == 1) return true;
if (isInitialized == 0) return false;
memoizedIsInitialized = 1;
return true;
}
public void writeTo(com.google.protobuf.CodedOutputStream output)
throws java.io.IOException {
if (resCode_ != com.github.wenweihu86.raft.proto.RaftMessage.ResCode.RES_CODE_SUCCESS.getNumber()) {
output.writeEnum(1, resCode_);
}
if (term_ != 0L) {
output.writeUInt64(2, term_);
}
if (lastLogIndex_ != 0L) {
output.writeUInt64(3, lastLogIndex_);
}
}
public int getSerializedSize() {
int size = memoizedSize;
if (size != -1) return size;
size = 0;
if (resCode_ != com.github.wenweihu86.raft.proto.RaftMessage.ResCode.RES_CODE_SUCCESS.getNumber()) {
size += com.google.protobuf.CodedOutputStream
.computeEnumSize(1, resCode_);
}
if (term_ != 0L) {
size += com.google.protobuf.CodedOutputStream
.computeUInt64Size(2, term_);
}
if (lastLogIndex_ != 0L) {
size += com.google.protobuf.CodedOutputStream
.computeUInt64Size(3, lastLogIndex_);
}
memoizedSize = size;
return size;
}
private static final long serialVersionUID = 0L;
@java.lang.Override
public boolean equals(final java.lang.Object obj) {
if (obj == this) {
return true;
}
if (!(obj instanceof com.github.wenweihu86.raft.proto.RaftMessage.AppendEntriesResponse)) {
return super.equals(obj);
}
com.github.wenweihu86.raft.proto.RaftMessage.AppendEntriesResponse other = (com.github.wenweihu86.raft.proto.RaftMessage.AppendEntriesResponse) obj;
boolean result = true;
result = result && resCode_ == other.resCode_;
result = result && (getTerm()
== other.getTerm());
result = result && (getLastLogIndex()
== other.getLastLogIndex());
return result;
}
@java.lang.Override
public int hashCode() {
if (memoizedHashCode != 0) {
return memoizedHashCode;
}
int hash = 41;
hash = (19 * hash) + getDescriptorForType().hashCode();
hash = (37 * hash) + RES_CODE_FIELD_NUMBER;
hash = (53 * hash) + resCode_;
hash = (37 * hash) + TERM_FIELD_NUMBER;
hash = (53 * hash) + com.google.protobuf.Internal.hashLong(
getTerm());
hash = (37 * hash) + LAST_LOG_INDEX_FIELD_NUMBER;
hash = (53 * hash) + com.google.protobuf.Internal.hashLong(
getLastLogIndex());
hash = (29 * hash) + unknownFields.hashCode();
memoizedHashCode = hash;
return hash;
}
public static com.github.wenweihu86.raft.proto.RaftMessage.AppendEntriesResponse parseFrom(
com.google.protobuf.ByteString data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static com.github.wenweihu86.raft.proto.RaftMessage.AppendEntriesResponse parseFrom(
com.google.protobuf.ByteString data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static com.github.wenweihu86.raft.proto.RaftMessage.AppendEntriesResponse parseFrom(byte[] data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static com.github.wenweihu86.raft.proto.RaftMessage.AppendEntriesResponse parseFrom(
byte[] data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static com.github.wenweihu86.raft.proto.RaftMessage.AppendEntriesResponse parseFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static com.github.wenweihu86.raft.proto.RaftMessage.AppendEntriesResponse parseFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
public static com.github.wenweihu86.raft.proto.RaftMessage.AppendEntriesResponse parseDelimitedFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input);
}
public static com.github.wenweihu86.raft.proto.RaftMessage.AppendEntriesResponse parseDelimitedFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input, extensionRegistry);
}
public static com.github.wenweihu86.raft.proto.RaftMessage.AppendEntriesResponse parseFrom(
com.google.protobuf.CodedInputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static com.github.wenweihu86.raft.proto.RaftMessage.AppendEntriesResponse parseFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
public Builder newBuilderForType() { return newBuilder(); }
public static Builder newBuilder() {
return DEFAULT_INSTANCE.toBuilder();
}
public static Builder newBuilder(com.github.wenweihu86.raft.proto.RaftMessage.AppendEntriesResponse prototype) {
return DEFAULT_INSTANCE.toBuilder().mergeFrom(prototype);
}
public Builder toBuilder() {
return this == DEFAULT_INSTANCE
? new Builder() : new Builder().mergeFrom(this);
}
@java.lang.Override
protected Builder newBuilderForType(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
Builder builder = new Builder(parent);
return builder;
}
/**
* Protobuf type {@code raft.AppendEntriesResponse}
*/
public static final class Builder extends
com.google.protobuf.GeneratedMessageV3.Builder implements
// @@protoc_insertion_point(builder_implements:raft.AppendEntriesResponse)
com.github.wenweihu86.raft.proto.RaftMessage.AppendEntriesResponseOrBuilder {
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return com.github.wenweihu86.raft.proto.RaftMessage.internal_static_raft_AppendEntriesResponse_descriptor;
}
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return com.github.wenweihu86.raft.proto.RaftMessage.internal_static_raft_AppendEntriesResponse_fieldAccessorTable
.ensureFieldAccessorsInitialized(
com.github.wenweihu86.raft.proto.RaftMessage.AppendEntriesResponse.class, com.github.wenweihu86.raft.proto.RaftMessage.AppendEntriesResponse.Builder.class);
}
// Construct using com.github.wenweihu86.raft.proto.RaftMessage.AppendEntriesResponse.newBuilder()
private Builder() {
maybeForceBuilderInitialization();
}
private Builder(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
super(parent);
maybeForceBuilderInitialization();
}
private void maybeForceBuilderInitialization() {
if (com.google.protobuf.GeneratedMessageV3
.alwaysUseFieldBuilders) {
}
}
public Builder clear() {
super.clear();
resCode_ = 0;
term_ = 0L;
lastLogIndex_ = 0L;
return this;
}
public com.google.protobuf.Descriptors.Descriptor
getDescriptorForType() {
return com.github.wenweihu86.raft.proto.RaftMessage.internal_static_raft_AppendEntriesResponse_descriptor;
}
public com.github.wenweihu86.raft.proto.RaftMessage.AppendEntriesResponse getDefaultInstanceForType() {
return com.github.wenweihu86.raft.proto.RaftMessage.AppendEntriesResponse.getDefaultInstance();
}
public com.github.wenweihu86.raft.proto.RaftMessage.AppendEntriesResponse build() {
com.github.wenweihu86.raft.proto.RaftMessage.AppendEntriesResponse result = buildPartial();
if (!result.isInitialized()) {
throw newUninitializedMessageException(result);
}
return result;
}
public com.github.wenweihu86.raft.proto.RaftMessage.AppendEntriesResponse buildPartial() {
com.github.wenweihu86.raft.proto.RaftMessage.AppendEntriesResponse result = new com.github.wenweihu86.raft.proto.RaftMessage.AppendEntriesResponse(this);
result.resCode_ = resCode_;
result.term_ = term_;
result.lastLogIndex_ = lastLogIndex_;
onBuilt();
return result;
}
public Builder clone() {
return (Builder) super.clone();
}
public Builder setField(
com.google.protobuf.Descriptors.FieldDescriptor field,
Object value) {
return (Builder) super.setField(field, value);
}
public Builder clearField(
com.google.protobuf.Descriptors.FieldDescriptor field) {
return (Builder) super.clearField(field);
}
public Builder clearOneof(
com.google.protobuf.Descriptors.OneofDescriptor oneof) {
return (Builder) super.clearOneof(oneof);
}
public Builder setRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
int index, Object value) {
return (Builder) super.setRepeatedField(field, index, value);
}
public Builder addRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
Object value) {
return (Builder) super.addRepeatedField(field, value);
}
public Builder mergeFrom(com.google.protobuf.Message other) {
if (other instanceof com.github.wenweihu86.raft.proto.RaftMessage.AppendEntriesResponse) {
return mergeFrom((com.github.wenweihu86.raft.proto.RaftMessage.AppendEntriesResponse)other);
} else {
super.mergeFrom(other);
return this;
}
}
public Builder mergeFrom(com.github.wenweihu86.raft.proto.RaftMessage.AppendEntriesResponse other) {
if (other == com.github.wenweihu86.raft.proto.RaftMessage.AppendEntriesResponse.getDefaultInstance()) return this;
if (other.resCode_ != 0) {
setResCodeValue(other.getResCodeValue());
}
if (other.getTerm() != 0L) {
setTerm(other.getTerm());
}
if (other.getLastLogIndex() != 0L) {
setLastLogIndex(other.getLastLogIndex());
}
onChanged();
return this;
}
public final boolean isInitialized() {
return true;
}
public Builder mergeFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
com.github.wenweihu86.raft.proto.RaftMessage.AppendEntriesResponse parsedMessage = null;
try {
parsedMessage = PARSER.parsePartialFrom(input, extensionRegistry);
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
parsedMessage = (com.github.wenweihu86.raft.proto.RaftMessage.AppendEntriesResponse) e.getUnfinishedMessage();
throw e.unwrapIOException();
} finally {
if (parsedMessage != null) {
mergeFrom(parsedMessage);
}
}
return this;
}
private int resCode_ = 0;
/**
*
* 跟随者包含了匹配上 prevLogIndex 和 prevLogTerm 的日志时为真
*
*
* optional .raft.ResCode res_code = 1;
*/
public int getResCodeValue() {
return resCode_;
}
/**
*
* 跟随者包含了匹配上 prevLogIndex 和 prevLogTerm 的日志时为真
*
*
* optional .raft.ResCode res_code = 1;
*/
public Builder setResCodeValue(int value) {
resCode_ = value;
onChanged();
return this;
}
/**
*
* 跟随者包含了匹配上 prevLogIndex 和 prevLogTerm 的日志时为真
*
*
* optional .raft.ResCode res_code = 1;
*/
public com.github.wenweihu86.raft.proto.RaftMessage.ResCode getResCode() {
com.github.wenweihu86.raft.proto.RaftMessage.ResCode result = com.github.wenweihu86.raft.proto.RaftMessage.ResCode.valueOf(resCode_);
return result == null ? com.github.wenweihu86.raft.proto.RaftMessage.ResCode.UNRECOGNIZED : result;
}
/**
*
* 跟随者包含了匹配上 prevLogIndex 和 prevLogTerm 的日志时为真
*
*
* optional .raft.ResCode res_code = 1;
*/
public Builder setResCode(com.github.wenweihu86.raft.proto.RaftMessage.ResCode value) {
if (value == null) {
throw new NullPointerException();
}
resCode_ = value.getNumber();
onChanged();
return this;
}
/**
*
* 跟随者包含了匹配上 prevLogIndex 和 prevLogTerm 的日志时为真
*
*
* optional .raft.ResCode res_code = 1;
*/
public Builder clearResCode() {
resCode_ = 0;
onChanged();
return this;
}
private long term_ ;
/**
*
* 当前的任期号,用于领导人去更新自己
*
*
* optional uint64 term = 2;
*/
public long getTerm() {
return term_;
}
/**
*
* 当前的任期号,用于领导人去更新自己
*
*
* optional uint64 term = 2;
*/
public Builder setTerm(long value) {
term_ = value;
onChanged();
return this;
}
/**
*
* 当前的任期号,用于领导人去更新自己
*
*
* optional uint64 term = 2;
*/
public Builder clearTerm() {
term_ = 0L;
onChanged();
return this;
}
private long lastLogIndex_ ;
/**
* optional uint64 last_log_index = 3;
*/
public long getLastLogIndex() {
return lastLogIndex_;
}
/**
* optional uint64 last_log_index = 3;
*/
public Builder setLastLogIndex(long value) {
lastLogIndex_ = value;
onChanged();
return this;
}
/**
* optional uint64 last_log_index = 3;
*/
public Builder clearLastLogIndex() {
lastLogIndex_ = 0L;
onChanged();
return this;
}
public final Builder setUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return this;
}
public final Builder mergeUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return this;
}
// @@protoc_insertion_point(builder_scope:raft.AppendEntriesResponse)
}
// @@protoc_insertion_point(class_scope:raft.AppendEntriesResponse)
private static final com.github.wenweihu86.raft.proto.RaftMessage.AppendEntriesResponse DEFAULT_INSTANCE;
static {
DEFAULT_INSTANCE = new com.github.wenweihu86.raft.proto.RaftMessage.AppendEntriesResponse();
}
public static com.github.wenweihu86.raft.proto.RaftMessage.AppendEntriesResponse getDefaultInstance() {
return DEFAULT_INSTANCE;
}
private static final com.google.protobuf.Parser
PARSER = new com.google.protobuf.AbstractParser() {
public AppendEntriesResponse parsePartialFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return new AppendEntriesResponse(input, extensionRegistry);
}
};
public static com.google.protobuf.Parser parser() {
return PARSER;
}
@java.lang.Override
public com.google.protobuf.Parser getParserForType() {
return PARSER;
}
public com.github.wenweihu86.raft.proto.RaftMessage.AppendEntriesResponse getDefaultInstanceForType() {
return DEFAULT_INSTANCE;
}
}
public interface InstallSnapshotRequestOrBuilder extends
// @@protoc_insertion_point(interface_extends:raft.InstallSnapshotRequest)
com.google.protobuf.MessageOrBuilder {
/**
* optional uint32 server_id = 1;
*/
int getServerId();
/**
* optional uint64 term = 2;
*/
long getTerm();
/**
* optional .raft.SnapshotMetaData snapshot_meta_data = 3;
*/
boolean hasSnapshotMetaData();
/**
* optional .raft.SnapshotMetaData snapshot_meta_data = 3;
*/
com.github.wenweihu86.raft.proto.RaftMessage.SnapshotMetaData getSnapshotMetaData();
/**
* optional .raft.SnapshotMetaData snapshot_meta_data = 3;
*/
com.github.wenweihu86.raft.proto.RaftMessage.SnapshotMetaDataOrBuilder getSnapshotMetaDataOrBuilder();
/**
* optional string file_name = 4;
*/
java.lang.String getFileName();
/**
* optional string file_name = 4;
*/
com.google.protobuf.ByteString
getFileNameBytes();
/**
* optional uint64 offset = 5;
*/
long getOffset();
/**
* optional bytes data = 6;
*/
com.google.protobuf.ByteString getData();
/**
* optional bool is_first = 7;
*/
boolean getIsFirst();
/**
* optional bool is_last = 8;
*/
boolean getIsLast();
}
/**
* Protobuf type {@code raft.InstallSnapshotRequest}
*/
public static final class InstallSnapshotRequest extends
com.google.protobuf.GeneratedMessageV3 implements
// @@protoc_insertion_point(message_implements:raft.InstallSnapshotRequest)
InstallSnapshotRequestOrBuilder {
// Use InstallSnapshotRequest.newBuilder() to construct.
private InstallSnapshotRequest(com.google.protobuf.GeneratedMessageV3.Builder> builder) {
super(builder);
}
private InstallSnapshotRequest() {
serverId_ = 0;
term_ = 0L;
fileName_ = "";
offset_ = 0L;
data_ = com.google.protobuf.ByteString.EMPTY;
isFirst_ = false;
isLast_ = false;
}
@java.lang.Override
public final com.google.protobuf.UnknownFieldSet
getUnknownFields() {
return com.google.protobuf.UnknownFieldSet.getDefaultInstance();
}
private InstallSnapshotRequest(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
this();
int mutable_bitField0_ = 0;
try {
boolean done = false;
while (!done) {
int tag = input.readTag();
switch (tag) {
case 0:
done = true;
break;
default: {
if (!input.skipField(tag)) {
done = true;
}
break;
}
case 8: {
serverId_ = input.readUInt32();
break;
}
case 16: {
term_ = input.readUInt64();
break;
}
case 26: {
com.github.wenweihu86.raft.proto.RaftMessage.SnapshotMetaData.Builder subBuilder = null;
if (snapshotMetaData_ != null) {
subBuilder = snapshotMetaData_.toBuilder();
}
snapshotMetaData_ = input.readMessage(com.github.wenweihu86.raft.proto.RaftMessage.SnapshotMetaData.parser(), extensionRegistry);
if (subBuilder != null) {
subBuilder.mergeFrom(snapshotMetaData_);
snapshotMetaData_ = subBuilder.buildPartial();
}
break;
}
case 34: {
java.lang.String s = input.readStringRequireUtf8();
fileName_ = s;
break;
}
case 40: {
offset_ = input.readUInt64();
break;
}
case 50: {
data_ = input.readBytes();
break;
}
case 56: {
isFirst_ = input.readBool();
break;
}
case 64: {
isLast_ = input.readBool();
break;
}
}
}
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.setUnfinishedMessage(this);
} catch (java.io.IOException e) {
throw new com.google.protobuf.InvalidProtocolBufferException(
e).setUnfinishedMessage(this);
} finally {
makeExtensionsImmutable();
}
}
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return com.github.wenweihu86.raft.proto.RaftMessage.internal_static_raft_InstallSnapshotRequest_descriptor;
}
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return com.github.wenweihu86.raft.proto.RaftMessage.internal_static_raft_InstallSnapshotRequest_fieldAccessorTable
.ensureFieldAccessorsInitialized(
com.github.wenweihu86.raft.proto.RaftMessage.InstallSnapshotRequest.class, com.github.wenweihu86.raft.proto.RaftMessage.InstallSnapshotRequest.Builder.class);
}
public static final int SERVER_ID_FIELD_NUMBER = 1;
private int serverId_;
/**
* optional uint32 server_id = 1;
*/
public int getServerId() {
return serverId_;
}
public static final int TERM_FIELD_NUMBER = 2;
private long term_;
/**
* optional uint64 term = 2;
*/
public long getTerm() {
return term_;
}
public static final int SNAPSHOT_META_DATA_FIELD_NUMBER = 3;
private com.github.wenweihu86.raft.proto.RaftMessage.SnapshotMetaData snapshotMetaData_;
/**
* optional .raft.SnapshotMetaData snapshot_meta_data = 3;
*/
public boolean hasSnapshotMetaData() {
return snapshotMetaData_ != null;
}
/**
* optional .raft.SnapshotMetaData snapshot_meta_data = 3;
*/
public com.github.wenweihu86.raft.proto.RaftMessage.SnapshotMetaData getSnapshotMetaData() {
return snapshotMetaData_ == null ? com.github.wenweihu86.raft.proto.RaftMessage.SnapshotMetaData.getDefaultInstance() : snapshotMetaData_;
}
/**
* optional .raft.SnapshotMetaData snapshot_meta_data = 3;
*/
public com.github.wenweihu86.raft.proto.RaftMessage.SnapshotMetaDataOrBuilder getSnapshotMetaDataOrBuilder() {
return getSnapshotMetaData();
}
public static final int FILE_NAME_FIELD_NUMBER = 4;
private volatile java.lang.Object fileName_;
/**
* optional string file_name = 4;
*/
public java.lang.String getFileName() {
java.lang.Object ref = fileName_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
fileName_ = s;
return s;
}
}
/**
* optional string file_name = 4;
*/
public com.google.protobuf.ByteString
getFileNameBytes() {
java.lang.Object ref = fileName_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
fileName_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
public static final int OFFSET_FIELD_NUMBER = 5;
private long offset_;
/**
* optional uint64 offset = 5;
*/
public long getOffset() {
return offset_;
}
public static final int DATA_FIELD_NUMBER = 6;
private com.google.protobuf.ByteString data_;
/**
* optional bytes data = 6;
*/
public com.google.protobuf.ByteString getData() {
return data_;
}
public static final int IS_FIRST_FIELD_NUMBER = 7;
private boolean isFirst_;
/**
* optional bool is_first = 7;
*/
public boolean getIsFirst() {
return isFirst_;
}
public static final int IS_LAST_FIELD_NUMBER = 8;
private boolean isLast_;
/**
* optional bool is_last = 8;
*/
public boolean getIsLast() {
return isLast_;
}
private byte memoizedIsInitialized = -1;
public final boolean isInitialized() {
byte isInitialized = memoizedIsInitialized;
if (isInitialized == 1) return true;
if (isInitialized == 0) return false;
memoizedIsInitialized = 1;
return true;
}
public void writeTo(com.google.protobuf.CodedOutputStream output)
throws java.io.IOException {
if (serverId_ != 0) {
output.writeUInt32(1, serverId_);
}
if (term_ != 0L) {
output.writeUInt64(2, term_);
}
if (snapshotMetaData_ != null) {
output.writeMessage(3, getSnapshotMetaData());
}
if (!getFileNameBytes().isEmpty()) {
com.google.protobuf.GeneratedMessageV3.writeString(output, 4, fileName_);
}
if (offset_ != 0L) {
output.writeUInt64(5, offset_);
}
if (!data_.isEmpty()) {
output.writeBytes(6, data_);
}
if (isFirst_ != false) {
output.writeBool(7, isFirst_);
}
if (isLast_ != false) {
output.writeBool(8, isLast_);
}
}
public int getSerializedSize() {
int size = memoizedSize;
if (size != -1) return size;
size = 0;
if (serverId_ != 0) {
size += com.google.protobuf.CodedOutputStream
.computeUInt32Size(1, serverId_);
}
if (term_ != 0L) {
size += com.google.protobuf.CodedOutputStream
.computeUInt64Size(2, term_);
}
if (snapshotMetaData_ != null) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(3, getSnapshotMetaData());
}
if (!getFileNameBytes().isEmpty()) {
size += com.google.protobuf.GeneratedMessageV3.computeStringSize(4, fileName_);
}
if (offset_ != 0L) {
size += com.google.protobuf.CodedOutputStream
.computeUInt64Size(5, offset_);
}
if (!data_.isEmpty()) {
size += com.google.protobuf.CodedOutputStream
.computeBytesSize(6, data_);
}
if (isFirst_ != false) {
size += com.google.protobuf.CodedOutputStream
.computeBoolSize(7, isFirst_);
}
if (isLast_ != false) {
size += com.google.protobuf.CodedOutputStream
.computeBoolSize(8, isLast_);
}
memoizedSize = size;
return size;
}
private static final long serialVersionUID = 0L;
@java.lang.Override
public boolean equals(final java.lang.Object obj) {
if (obj == this) {
return true;
}
if (!(obj instanceof com.github.wenweihu86.raft.proto.RaftMessage.InstallSnapshotRequest)) {
return super.equals(obj);
}
com.github.wenweihu86.raft.proto.RaftMessage.InstallSnapshotRequest other = (com.github.wenweihu86.raft.proto.RaftMessage.InstallSnapshotRequest) obj;
boolean result = true;
result = result && (getServerId()
== other.getServerId());
result = result && (getTerm()
== other.getTerm());
result = result && (hasSnapshotMetaData() == other.hasSnapshotMetaData());
if (hasSnapshotMetaData()) {
result = result && getSnapshotMetaData()
.equals(other.getSnapshotMetaData());
}
result = result && getFileName()
.equals(other.getFileName());
result = result && (getOffset()
== other.getOffset());
result = result && getData()
.equals(other.getData());
result = result && (getIsFirst()
== other.getIsFirst());
result = result && (getIsLast()
== other.getIsLast());
return result;
}
@java.lang.Override
public int hashCode() {
if (memoizedHashCode != 0) {
return memoizedHashCode;
}
int hash = 41;
hash = (19 * hash) + getDescriptorForType().hashCode();
hash = (37 * hash) + SERVER_ID_FIELD_NUMBER;
hash = (53 * hash) + getServerId();
hash = (37 * hash) + TERM_FIELD_NUMBER;
hash = (53 * hash) + com.google.protobuf.Internal.hashLong(
getTerm());
if (hasSnapshotMetaData()) {
hash = (37 * hash) + SNAPSHOT_META_DATA_FIELD_NUMBER;
hash = (53 * hash) + getSnapshotMetaData().hashCode();
}
hash = (37 * hash) + FILE_NAME_FIELD_NUMBER;
hash = (53 * hash) + getFileName().hashCode();
hash = (37 * hash) + OFFSET_FIELD_NUMBER;
hash = (53 * hash) + com.google.protobuf.Internal.hashLong(
getOffset());
hash = (37 * hash) + DATA_FIELD_NUMBER;
hash = (53 * hash) + getData().hashCode();
hash = (37 * hash) + IS_FIRST_FIELD_NUMBER;
hash = (53 * hash) + com.google.protobuf.Internal.hashBoolean(
getIsFirst());
hash = (37 * hash) + IS_LAST_FIELD_NUMBER;
hash = (53 * hash) + com.google.protobuf.Internal.hashBoolean(
getIsLast());
hash = (29 * hash) + unknownFields.hashCode();
memoizedHashCode = hash;
return hash;
}
public static com.github.wenweihu86.raft.proto.RaftMessage.InstallSnapshotRequest parseFrom(
com.google.protobuf.ByteString data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static com.github.wenweihu86.raft.proto.RaftMessage.InstallSnapshotRequest parseFrom(
com.google.protobuf.ByteString data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static com.github.wenweihu86.raft.proto.RaftMessage.InstallSnapshotRequest parseFrom(byte[] data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static com.github.wenweihu86.raft.proto.RaftMessage.InstallSnapshotRequest parseFrom(
byte[] data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static com.github.wenweihu86.raft.proto.RaftMessage.InstallSnapshotRequest parseFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static com.github.wenweihu86.raft.proto.RaftMessage.InstallSnapshotRequest parseFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
public static com.github.wenweihu86.raft.proto.RaftMessage.InstallSnapshotRequest parseDelimitedFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input);
}
public static com.github.wenweihu86.raft.proto.RaftMessage.InstallSnapshotRequest parseDelimitedFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input, extensionRegistry);
}
public static com.github.wenweihu86.raft.proto.RaftMessage.InstallSnapshotRequest parseFrom(
com.google.protobuf.CodedInputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static com.github.wenweihu86.raft.proto.RaftMessage.InstallSnapshotRequest parseFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
public Builder newBuilderForType() { return newBuilder(); }
public static Builder newBuilder() {
return DEFAULT_INSTANCE.toBuilder();
}
public static Builder newBuilder(com.github.wenweihu86.raft.proto.RaftMessage.InstallSnapshotRequest prototype) {
return DEFAULT_INSTANCE.toBuilder().mergeFrom(prototype);
}
public Builder toBuilder() {
return this == DEFAULT_INSTANCE
? new Builder() : new Builder().mergeFrom(this);
}
@java.lang.Override
protected Builder newBuilderForType(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
Builder builder = new Builder(parent);
return builder;
}
/**
* Protobuf type {@code raft.InstallSnapshotRequest}
*/
public static final class Builder extends
com.google.protobuf.GeneratedMessageV3.Builder implements
// @@protoc_insertion_point(builder_implements:raft.InstallSnapshotRequest)
com.github.wenweihu86.raft.proto.RaftMessage.InstallSnapshotRequestOrBuilder {
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return com.github.wenweihu86.raft.proto.RaftMessage.internal_static_raft_InstallSnapshotRequest_descriptor;
}
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return com.github.wenweihu86.raft.proto.RaftMessage.internal_static_raft_InstallSnapshotRequest_fieldAccessorTable
.ensureFieldAccessorsInitialized(
com.github.wenweihu86.raft.proto.RaftMessage.InstallSnapshotRequest.class, com.github.wenweihu86.raft.proto.RaftMessage.InstallSnapshotRequest.Builder.class);
}
// Construct using com.github.wenweihu86.raft.proto.RaftMessage.InstallSnapshotRequest.newBuilder()
private Builder() {
maybeForceBuilderInitialization();
}
private Builder(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
super(parent);
maybeForceBuilderInitialization();
}
private void maybeForceBuilderInitialization() {
if (com.google.protobuf.GeneratedMessageV3
.alwaysUseFieldBuilders) {
}
}
public Builder clear() {
super.clear();
serverId_ = 0;
term_ = 0L;
if (snapshotMetaDataBuilder_ == null) {
snapshotMetaData_ = null;
} else {
snapshotMetaData_ = null;
snapshotMetaDataBuilder_ = null;
}
fileName_ = "";
offset_ = 0L;
data_ = com.google.protobuf.ByteString.EMPTY;
isFirst_ = false;
isLast_ = false;
return this;
}
public com.google.protobuf.Descriptors.Descriptor
getDescriptorForType() {
return com.github.wenweihu86.raft.proto.RaftMessage.internal_static_raft_InstallSnapshotRequest_descriptor;
}
public com.github.wenweihu86.raft.proto.RaftMessage.InstallSnapshotRequest getDefaultInstanceForType() {
return com.github.wenweihu86.raft.proto.RaftMessage.InstallSnapshotRequest.getDefaultInstance();
}
public com.github.wenweihu86.raft.proto.RaftMessage.InstallSnapshotRequest build() {
com.github.wenweihu86.raft.proto.RaftMessage.InstallSnapshotRequest result = buildPartial();
if (!result.isInitialized()) {
throw newUninitializedMessageException(result);
}
return result;
}
public com.github.wenweihu86.raft.proto.RaftMessage.InstallSnapshotRequest buildPartial() {
com.github.wenweihu86.raft.proto.RaftMessage.InstallSnapshotRequest result = new com.github.wenweihu86.raft.proto.RaftMessage.InstallSnapshotRequest(this);
result.serverId_ = serverId_;
result.term_ = term_;
if (snapshotMetaDataBuilder_ == null) {
result.snapshotMetaData_ = snapshotMetaData_;
} else {
result.snapshotMetaData_ = snapshotMetaDataBuilder_.build();
}
result.fileName_ = fileName_;
result.offset_ = offset_;
result.data_ = data_;
result.isFirst_ = isFirst_;
result.isLast_ = isLast_;
onBuilt();
return result;
}
public Builder clone() {
return (Builder) super.clone();
}
public Builder setField(
com.google.protobuf.Descriptors.FieldDescriptor field,
Object value) {
return (Builder) super.setField(field, value);
}
public Builder clearField(
com.google.protobuf.Descriptors.FieldDescriptor field) {
return (Builder) super.clearField(field);
}
public Builder clearOneof(
com.google.protobuf.Descriptors.OneofDescriptor oneof) {
return (Builder) super.clearOneof(oneof);
}
public Builder setRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
int index, Object value) {
return (Builder) super.setRepeatedField(field, index, value);
}
public Builder addRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
Object value) {
return (Builder) super.addRepeatedField(field, value);
}
public Builder mergeFrom(com.google.protobuf.Message other) {
if (other instanceof com.github.wenweihu86.raft.proto.RaftMessage.InstallSnapshotRequest) {
return mergeFrom((com.github.wenweihu86.raft.proto.RaftMessage.InstallSnapshotRequest)other);
} else {
super.mergeFrom(other);
return this;
}
}
public Builder mergeFrom(com.github.wenweihu86.raft.proto.RaftMessage.InstallSnapshotRequest other) {
if (other == com.github.wenweihu86.raft.proto.RaftMessage.InstallSnapshotRequest.getDefaultInstance()) return this;
if (other.getServerId() != 0) {
setServerId(other.getServerId());
}
if (other.getTerm() != 0L) {
setTerm(other.getTerm());
}
if (other.hasSnapshotMetaData()) {
mergeSnapshotMetaData(other.getSnapshotMetaData());
}
if (!other.getFileName().isEmpty()) {
fileName_ = other.fileName_;
onChanged();
}
if (other.getOffset() != 0L) {
setOffset(other.getOffset());
}
if (other.getData() != com.google.protobuf.ByteString.EMPTY) {
setData(other.getData());
}
if (other.getIsFirst() != false) {
setIsFirst(other.getIsFirst());
}
if (other.getIsLast() != false) {
setIsLast(other.getIsLast());
}
onChanged();
return this;
}
public final boolean isInitialized() {
return true;
}
public Builder mergeFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
com.github.wenweihu86.raft.proto.RaftMessage.InstallSnapshotRequest parsedMessage = null;
try {
parsedMessage = PARSER.parsePartialFrom(input, extensionRegistry);
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
parsedMessage = (com.github.wenweihu86.raft.proto.RaftMessage.InstallSnapshotRequest) e.getUnfinishedMessage();
throw e.unwrapIOException();
} finally {
if (parsedMessage != null) {
mergeFrom(parsedMessage);
}
}
return this;
}
private int serverId_ ;
/**
* optional uint32 server_id = 1;
*/
public int getServerId() {
return serverId_;
}
/**
* optional uint32 server_id = 1;
*/
public Builder setServerId(int value) {
serverId_ = value;
onChanged();
return this;
}
/**
* optional uint32 server_id = 1;
*/
public Builder clearServerId() {
serverId_ = 0;
onChanged();
return this;
}
private long term_ ;
/**
* optional uint64 term = 2;
*/
public long getTerm() {
return term_;
}
/**
* optional uint64 term = 2;
*/
public Builder setTerm(long value) {
term_ = value;
onChanged();
return this;
}
/**
* optional uint64 term = 2;
*/
public Builder clearTerm() {
term_ = 0L;
onChanged();
return this;
}
private com.github.wenweihu86.raft.proto.RaftMessage.SnapshotMetaData snapshotMetaData_ = null;
private com.google.protobuf.SingleFieldBuilderV3<
com.github.wenweihu86.raft.proto.RaftMessage.SnapshotMetaData, com.github.wenweihu86.raft.proto.RaftMessage.SnapshotMetaData.Builder, com.github.wenweihu86.raft.proto.RaftMessage.SnapshotMetaDataOrBuilder> snapshotMetaDataBuilder_;
/**
* optional .raft.SnapshotMetaData snapshot_meta_data = 3;
*/
public boolean hasSnapshotMetaData() {
return snapshotMetaDataBuilder_ != null || snapshotMetaData_ != null;
}
/**
* optional .raft.SnapshotMetaData snapshot_meta_data = 3;
*/
public com.github.wenweihu86.raft.proto.RaftMessage.SnapshotMetaData getSnapshotMetaData() {
if (snapshotMetaDataBuilder_ == null) {
return snapshotMetaData_ == null ? com.github.wenweihu86.raft.proto.RaftMessage.SnapshotMetaData.getDefaultInstance() : snapshotMetaData_;
} else {
return snapshotMetaDataBuilder_.getMessage();
}
}
/**
* optional .raft.SnapshotMetaData snapshot_meta_data = 3;
*/
public Builder setSnapshotMetaData(com.github.wenweihu86.raft.proto.RaftMessage.SnapshotMetaData value) {
if (snapshotMetaDataBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
snapshotMetaData_ = value;
onChanged();
} else {
snapshotMetaDataBuilder_.setMessage(value);
}
return this;
}
/**
* optional .raft.SnapshotMetaData snapshot_meta_data = 3;
*/
public Builder setSnapshotMetaData(
com.github.wenweihu86.raft.proto.RaftMessage.SnapshotMetaData.Builder builderForValue) {
if (snapshotMetaDataBuilder_ == null) {
snapshotMetaData_ = builderForValue.build();
onChanged();
} else {
snapshotMetaDataBuilder_.setMessage(builderForValue.build());
}
return this;
}
/**
* optional .raft.SnapshotMetaData snapshot_meta_data = 3;
*/
public Builder mergeSnapshotMetaData(com.github.wenweihu86.raft.proto.RaftMessage.SnapshotMetaData value) {
if (snapshotMetaDataBuilder_ == null) {
if (snapshotMetaData_ != null) {
snapshotMetaData_ =
com.github.wenweihu86.raft.proto.RaftMessage.SnapshotMetaData.newBuilder(snapshotMetaData_).mergeFrom(value).buildPartial();
} else {
snapshotMetaData_ = value;
}
onChanged();
} else {
snapshotMetaDataBuilder_.mergeFrom(value);
}
return this;
}
/**
* optional .raft.SnapshotMetaData snapshot_meta_data = 3;
*/
public Builder clearSnapshotMetaData() {
if (snapshotMetaDataBuilder_ == null) {
snapshotMetaData_ = null;
onChanged();
} else {
snapshotMetaData_ = null;
snapshotMetaDataBuilder_ = null;
}
return this;
}
/**
* optional .raft.SnapshotMetaData snapshot_meta_data = 3;
*/
public com.github.wenweihu86.raft.proto.RaftMessage.SnapshotMetaData.Builder getSnapshotMetaDataBuilder() {
onChanged();
return getSnapshotMetaDataFieldBuilder().getBuilder();
}
/**
* optional .raft.SnapshotMetaData snapshot_meta_data = 3;
*/
public com.github.wenweihu86.raft.proto.RaftMessage.SnapshotMetaDataOrBuilder getSnapshotMetaDataOrBuilder() {
if (snapshotMetaDataBuilder_ != null) {
return snapshotMetaDataBuilder_.getMessageOrBuilder();
} else {
return snapshotMetaData_ == null ?
com.github.wenweihu86.raft.proto.RaftMessage.SnapshotMetaData.getDefaultInstance() : snapshotMetaData_;
}
}
/**
* optional .raft.SnapshotMetaData snapshot_meta_data = 3;
*/
private com.google.protobuf.SingleFieldBuilderV3<
com.github.wenweihu86.raft.proto.RaftMessage.SnapshotMetaData, com.github.wenweihu86.raft.proto.RaftMessage.SnapshotMetaData.Builder, com.github.wenweihu86.raft.proto.RaftMessage.SnapshotMetaDataOrBuilder>
getSnapshotMetaDataFieldBuilder() {
if (snapshotMetaDataBuilder_ == null) {
snapshotMetaDataBuilder_ = new com.google.protobuf.SingleFieldBuilderV3<
com.github.wenweihu86.raft.proto.RaftMessage.SnapshotMetaData, com.github.wenweihu86.raft.proto.RaftMessage.SnapshotMetaData.Builder, com.github.wenweihu86.raft.proto.RaftMessage.SnapshotMetaDataOrBuilder>(
getSnapshotMetaData(),
getParentForChildren(),
isClean());
snapshotMetaData_ = null;
}
return snapshotMetaDataBuilder_;
}
private java.lang.Object fileName_ = "";
/**
* optional string file_name = 4;
*/
public java.lang.String getFileName() {
java.lang.Object ref = fileName_;
if (!(ref instanceof java.lang.String)) {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
fileName_ = s;
return s;
} else {
return (java.lang.String) ref;
}
}
/**
* optional string file_name = 4;
*/
public com.google.protobuf.ByteString
getFileNameBytes() {
java.lang.Object ref = fileName_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
fileName_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
* optional string file_name = 4;
*/
public Builder setFileName(
java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
fileName_ = value;
onChanged();
return this;
}
/**
* optional string file_name = 4;
*/
public Builder clearFileName() {
fileName_ = getDefaultInstance().getFileName();
onChanged();
return this;
}
/**
* optional string file_name = 4;
*/
public Builder setFileNameBytes(
com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
checkByteStringIsUtf8(value);
fileName_ = value;
onChanged();
return this;
}
private long offset_ ;
/**
* optional uint64 offset = 5;
*/
public long getOffset() {
return offset_;
}
/**
* optional uint64 offset = 5;
*/
public Builder setOffset(long value) {
offset_ = value;
onChanged();
return this;
}
/**
* optional uint64 offset = 5;
*/
public Builder clearOffset() {
offset_ = 0L;
onChanged();
return this;
}
private com.google.protobuf.ByteString data_ = com.google.protobuf.ByteString.EMPTY;
/**
* optional bytes data = 6;
*/
public com.google.protobuf.ByteString getData() {
return data_;
}
/**
* optional bytes data = 6;
*/
public Builder setData(com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
data_ = value;
onChanged();
return this;
}
/**
* optional bytes data = 6;
*/
public Builder clearData() {
data_ = getDefaultInstance().getData();
onChanged();
return this;
}
private boolean isFirst_ ;
/**
* optional bool is_first = 7;
*/
public boolean getIsFirst() {
return isFirst_;
}
/**
* optional bool is_first = 7;
*/
public Builder setIsFirst(boolean value) {
isFirst_ = value;
onChanged();
return this;
}
/**
* optional bool is_first = 7;
*/
public Builder clearIsFirst() {
isFirst_ = false;
onChanged();
return this;
}
private boolean isLast_ ;
/**
* optional bool is_last = 8;
*/
public boolean getIsLast() {
return isLast_;
}
/**
* optional bool is_last = 8;
*/
public Builder setIsLast(boolean value) {
isLast_ = value;
onChanged();
return this;
}
/**
* optional bool is_last = 8;
*/
public Builder clearIsLast() {
isLast_ = false;
onChanged();
return this;
}
public final Builder setUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return this;
}
public final Builder mergeUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return this;
}
// @@protoc_insertion_point(builder_scope:raft.InstallSnapshotRequest)
}
// @@protoc_insertion_point(class_scope:raft.InstallSnapshotRequest)
private static final com.github.wenweihu86.raft.proto.RaftMessage.InstallSnapshotRequest DEFAULT_INSTANCE;
static {
DEFAULT_INSTANCE = new com.github.wenweihu86.raft.proto.RaftMessage.InstallSnapshotRequest();
}
public static com.github.wenweihu86.raft.proto.RaftMessage.InstallSnapshotRequest getDefaultInstance() {
return DEFAULT_INSTANCE;
}
private static final com.google.protobuf.Parser
PARSER = new com.google.protobuf.AbstractParser() {
public InstallSnapshotRequest parsePartialFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return new InstallSnapshotRequest(input, extensionRegistry);
}
};
public static com.google.protobuf.Parser parser() {
return PARSER;
}
@java.lang.Override
public com.google.protobuf.Parser getParserForType() {
return PARSER;
}
public com.github.wenweihu86.raft.proto.RaftMessage.InstallSnapshotRequest getDefaultInstanceForType() {
return DEFAULT_INSTANCE;
}
}
public interface InstallSnapshotResponseOrBuilder extends
// @@protoc_insertion_point(interface_extends:raft.InstallSnapshotResponse)
com.google.protobuf.MessageOrBuilder {
/**
* optional .raft.ResCode res_code = 1;
*/
int getResCodeValue();
/**
* optional .raft.ResCode res_code = 1;
*/
com.github.wenweihu86.raft.proto.RaftMessage.ResCode getResCode();
/**
* optional uint64 term = 2;
*/
long getTerm();
}
/**
* Protobuf type {@code raft.InstallSnapshotResponse}
*/
public static final class InstallSnapshotResponse extends
com.google.protobuf.GeneratedMessageV3 implements
// @@protoc_insertion_point(message_implements:raft.InstallSnapshotResponse)
InstallSnapshotResponseOrBuilder {
// Use InstallSnapshotResponse.newBuilder() to construct.
private InstallSnapshotResponse(com.google.protobuf.GeneratedMessageV3.Builder> builder) {
super(builder);
}
private InstallSnapshotResponse() {
resCode_ = 0;
term_ = 0L;
}
@java.lang.Override
public final com.google.protobuf.UnknownFieldSet
getUnknownFields() {
return com.google.protobuf.UnknownFieldSet.getDefaultInstance();
}
private InstallSnapshotResponse(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
this();
int mutable_bitField0_ = 0;
try {
boolean done = false;
while (!done) {
int tag = input.readTag();
switch (tag) {
case 0:
done = true;
break;
default: {
if (!input.skipField(tag)) {
done = true;
}
break;
}
case 8: {
int rawValue = input.readEnum();
resCode_ = rawValue;
break;
}
case 16: {
term_ = input.readUInt64();
break;
}
}
}
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.setUnfinishedMessage(this);
} catch (java.io.IOException e) {
throw new com.google.protobuf.InvalidProtocolBufferException(
e).setUnfinishedMessage(this);
} finally {
makeExtensionsImmutable();
}
}
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return com.github.wenweihu86.raft.proto.RaftMessage.internal_static_raft_InstallSnapshotResponse_descriptor;
}
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return com.github.wenweihu86.raft.proto.RaftMessage.internal_static_raft_InstallSnapshotResponse_fieldAccessorTable
.ensureFieldAccessorsInitialized(
com.github.wenweihu86.raft.proto.RaftMessage.InstallSnapshotResponse.class, com.github.wenweihu86.raft.proto.RaftMessage.InstallSnapshotResponse.Builder.class);
}
public static final int RES_CODE_FIELD_NUMBER = 1;
private int resCode_;
/**
* optional .raft.ResCode res_code = 1;
*/
public int getResCodeValue() {
return resCode_;
}
/**
* optional .raft.ResCode res_code = 1;
*/
public com.github.wenweihu86.raft.proto.RaftMessage.ResCode getResCode() {
com.github.wenweihu86.raft.proto.RaftMessage.ResCode result = com.github.wenweihu86.raft.proto.RaftMessage.ResCode.valueOf(resCode_);
return result == null ? com.github.wenweihu86.raft.proto.RaftMessage.ResCode.UNRECOGNIZED : result;
}
public static final int TERM_FIELD_NUMBER = 2;
private long term_;
/**
* optional uint64 term = 2;
*/
public long getTerm() {
return term_;
}
private byte memoizedIsInitialized = -1;
public final boolean isInitialized() {
byte isInitialized = memoizedIsInitialized;
if (isInitialized == 1) return true;
if (isInitialized == 0) return false;
memoizedIsInitialized = 1;
return true;
}
public void writeTo(com.google.protobuf.CodedOutputStream output)
throws java.io.IOException {
if (resCode_ != com.github.wenweihu86.raft.proto.RaftMessage.ResCode.RES_CODE_SUCCESS.getNumber()) {
output.writeEnum(1, resCode_);
}
if (term_ != 0L) {
output.writeUInt64(2, term_);
}
}
public int getSerializedSize() {
int size = memoizedSize;
if (size != -1) return size;
size = 0;
if (resCode_ != com.github.wenweihu86.raft.proto.RaftMessage.ResCode.RES_CODE_SUCCESS.getNumber()) {
size += com.google.protobuf.CodedOutputStream
.computeEnumSize(1, resCode_);
}
if (term_ != 0L) {
size += com.google.protobuf.CodedOutputStream
.computeUInt64Size(2, term_);
}
memoizedSize = size;
return size;
}
private static final long serialVersionUID = 0L;
@java.lang.Override
public boolean equals(final java.lang.Object obj) {
if (obj == this) {
return true;
}
if (!(obj instanceof com.github.wenweihu86.raft.proto.RaftMessage.InstallSnapshotResponse)) {
return super.equals(obj);
}
com.github.wenweihu86.raft.proto.RaftMessage.InstallSnapshotResponse other = (com.github.wenweihu86.raft.proto.RaftMessage.InstallSnapshotResponse) obj;
boolean result = true;
result = result && resCode_ == other.resCode_;
result = result && (getTerm()
== other.getTerm());
return result;
}
@java.lang.Override
public int hashCode() {
if (memoizedHashCode != 0) {
return memoizedHashCode;
}
int hash = 41;
hash = (19 * hash) + getDescriptorForType().hashCode();
hash = (37 * hash) + RES_CODE_FIELD_NUMBER;
hash = (53 * hash) + resCode_;
hash = (37 * hash) + TERM_FIELD_NUMBER;
hash = (53 * hash) + com.google.protobuf.Internal.hashLong(
getTerm());
hash = (29 * hash) + unknownFields.hashCode();
memoizedHashCode = hash;
return hash;
}
public static com.github.wenweihu86.raft.proto.RaftMessage.InstallSnapshotResponse parseFrom(
com.google.protobuf.ByteString data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static com.github.wenweihu86.raft.proto.RaftMessage.InstallSnapshotResponse parseFrom(
com.google.protobuf.ByteString data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static com.github.wenweihu86.raft.proto.RaftMessage.InstallSnapshotResponse parseFrom(byte[] data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static com.github.wenweihu86.raft.proto.RaftMessage.InstallSnapshotResponse parseFrom(
byte[] data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static com.github.wenweihu86.raft.proto.RaftMessage.InstallSnapshotResponse parseFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static com.github.wenweihu86.raft.proto.RaftMessage.InstallSnapshotResponse parseFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
public static com.github.wenweihu86.raft.proto.RaftMessage.InstallSnapshotResponse parseDelimitedFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input);
}
public static com.github.wenweihu86.raft.proto.RaftMessage.InstallSnapshotResponse parseDelimitedFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input, extensionRegistry);
}
public static com.github.wenweihu86.raft.proto.RaftMessage.InstallSnapshotResponse parseFrom(
com.google.protobuf.CodedInputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static com.github.wenweihu86.raft.proto.RaftMessage.InstallSnapshotResponse parseFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
public Builder newBuilderForType() { return newBuilder(); }
public static Builder newBuilder() {
return DEFAULT_INSTANCE.toBuilder();
}
public static Builder newBuilder(com.github.wenweihu86.raft.proto.RaftMessage.InstallSnapshotResponse prototype) {
return DEFAULT_INSTANCE.toBuilder().mergeFrom(prototype);
}
public Builder toBuilder() {
return this == DEFAULT_INSTANCE
? new Builder() : new Builder().mergeFrom(this);
}
@java.lang.Override
protected Builder newBuilderForType(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
Builder builder = new Builder(parent);
return builder;
}
/**
* Protobuf type {@code raft.InstallSnapshotResponse}
*/
public static final class Builder extends
com.google.protobuf.GeneratedMessageV3.Builder implements
// @@protoc_insertion_point(builder_implements:raft.InstallSnapshotResponse)
com.github.wenweihu86.raft.proto.RaftMessage.InstallSnapshotResponseOrBuilder {
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return com.github.wenweihu86.raft.proto.RaftMessage.internal_static_raft_InstallSnapshotResponse_descriptor;
}
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return com.github.wenweihu86.raft.proto.RaftMessage.internal_static_raft_InstallSnapshotResponse_fieldAccessorTable
.ensureFieldAccessorsInitialized(
com.github.wenweihu86.raft.proto.RaftMessage.InstallSnapshotResponse.class, com.github.wenweihu86.raft.proto.RaftMessage.InstallSnapshotResponse.Builder.class);
}
// Construct using com.github.wenweihu86.raft.proto.RaftMessage.InstallSnapshotResponse.newBuilder()
private Builder() {
maybeForceBuilderInitialization();
}
private Builder(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
super(parent);
maybeForceBuilderInitialization();
}
private void maybeForceBuilderInitialization() {
if (com.google.protobuf.GeneratedMessageV3
.alwaysUseFieldBuilders) {
}
}
public Builder clear() {
super.clear();
resCode_ = 0;
term_ = 0L;
return this;
}
public com.google.protobuf.Descriptors.Descriptor
getDescriptorForType() {
return com.github.wenweihu86.raft.proto.RaftMessage.internal_static_raft_InstallSnapshotResponse_descriptor;
}
public com.github.wenweihu86.raft.proto.RaftMessage.InstallSnapshotResponse getDefaultInstanceForType() {
return com.github.wenweihu86.raft.proto.RaftMessage.InstallSnapshotResponse.getDefaultInstance();
}
public com.github.wenweihu86.raft.proto.RaftMessage.InstallSnapshotResponse build() {
com.github.wenweihu86.raft.proto.RaftMessage.InstallSnapshotResponse result = buildPartial();
if (!result.isInitialized()) {
throw newUninitializedMessageException(result);
}
return result;
}
public com.github.wenweihu86.raft.proto.RaftMessage.InstallSnapshotResponse buildPartial() {
com.github.wenweihu86.raft.proto.RaftMessage.InstallSnapshotResponse result = new com.github.wenweihu86.raft.proto.RaftMessage.InstallSnapshotResponse(this);
result.resCode_ = resCode_;
result.term_ = term_;
onBuilt();
return result;
}
public Builder clone() {
return (Builder) super.clone();
}
public Builder setField(
com.google.protobuf.Descriptors.FieldDescriptor field,
Object value) {
return (Builder) super.setField(field, value);
}
public Builder clearField(
com.google.protobuf.Descriptors.FieldDescriptor field) {
return (Builder) super.clearField(field);
}
public Builder clearOneof(
com.google.protobuf.Descriptors.OneofDescriptor oneof) {
return (Builder) super.clearOneof(oneof);
}
public Builder setRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
int index, Object value) {
return (Builder) super.setRepeatedField(field, index, value);
}
public Builder addRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
Object value) {
return (Builder) super.addRepeatedField(field, value);
}
public Builder mergeFrom(com.google.protobuf.Message other) {
if (other instanceof com.github.wenweihu86.raft.proto.RaftMessage.InstallSnapshotResponse) {
return mergeFrom((com.github.wenweihu86.raft.proto.RaftMessage.InstallSnapshotResponse)other);
} else {
super.mergeFrom(other);
return this;
}
}
public Builder mergeFrom(com.github.wenweihu86.raft.proto.RaftMessage.InstallSnapshotResponse other) {
if (other == com.github.wenweihu86.raft.proto.RaftMessage.InstallSnapshotResponse.getDefaultInstance()) return this;
if (other.resCode_ != 0) {
setResCodeValue(other.getResCodeValue());
}
if (other.getTerm() != 0L) {
setTerm(other.getTerm());
}
onChanged();
return this;
}
public final boolean isInitialized() {
return true;
}
public Builder mergeFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
com.github.wenweihu86.raft.proto.RaftMessage.InstallSnapshotResponse parsedMessage = null;
try {
parsedMessage = PARSER.parsePartialFrom(input, extensionRegistry);
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
parsedMessage = (com.github.wenweihu86.raft.proto.RaftMessage.InstallSnapshotResponse) e.getUnfinishedMessage();
throw e.unwrapIOException();
} finally {
if (parsedMessage != null) {
mergeFrom(parsedMessage);
}
}
return this;
}
private int resCode_ = 0;
/**
* optional .raft.ResCode res_code = 1;
*/
public int getResCodeValue() {
return resCode_;
}
/**
* optional .raft.ResCode res_code = 1;
*/
public Builder setResCodeValue(int value) {
resCode_ = value;
onChanged();
return this;
}
/**
* optional .raft.ResCode res_code = 1;
*/
public com.github.wenweihu86.raft.proto.RaftMessage.ResCode getResCode() {
com.github.wenweihu86.raft.proto.RaftMessage.ResCode result = com.github.wenweihu86.raft.proto.RaftMessage.ResCode.valueOf(resCode_);
return result == null ? com.github.wenweihu86.raft.proto.RaftMessage.ResCode.UNRECOGNIZED : result;
}
/**
* optional .raft.ResCode res_code = 1;
*/
public Builder setResCode(com.github.wenweihu86.raft.proto.RaftMessage.ResCode value) {
if (value == null) {
throw new NullPointerException();
}
resCode_ = value.getNumber();
onChanged();
return this;
}
/**
* optional .raft.ResCode res_code = 1;
*/
public Builder clearResCode() {
resCode_ = 0;
onChanged();
return this;
}
private long term_ ;
/**
* optional uint64 term = 2;
*/
public long getTerm() {
return term_;
}
/**
* optional uint64 term = 2;
*/
public Builder setTerm(long value) {
term_ = value;
onChanged();
return this;
}
/**
* optional uint64 term = 2;
*/
public Builder clearTerm() {
term_ = 0L;
onChanged();
return this;
}
public final Builder setUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return this;
}
public final Builder mergeUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return this;
}
// @@protoc_insertion_point(builder_scope:raft.InstallSnapshotResponse)
}
// @@protoc_insertion_point(class_scope:raft.InstallSnapshotResponse)
private static final com.github.wenweihu86.raft.proto.RaftMessage.InstallSnapshotResponse DEFAULT_INSTANCE;
static {
DEFAULT_INSTANCE = new com.github.wenweihu86.raft.proto.RaftMessage.InstallSnapshotResponse();
}
public static com.github.wenweihu86.raft.proto.RaftMessage.InstallSnapshotResponse getDefaultInstance() {
return DEFAULT_INSTANCE;
}
private static final com.google.protobuf.Parser
PARSER = new com.google.protobuf.AbstractParser() {
public InstallSnapshotResponse parsePartialFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return new InstallSnapshotResponse(input, extensionRegistry);
}
};
public static com.google.protobuf.Parser parser() {
return PARSER;
}
@java.lang.Override
public com.google.protobuf.Parser getParserForType() {
return PARSER;
}
public com.github.wenweihu86.raft.proto.RaftMessage.InstallSnapshotResponse getDefaultInstanceForType() {
return DEFAULT_INSTANCE;
}
}
public interface GetLeaderRequestOrBuilder extends
// @@protoc_insertion_point(interface_extends:raft.GetLeaderRequest)
com.google.protobuf.MessageOrBuilder {
}
/**
* Protobuf type {@code raft.GetLeaderRequest}
*/
public static final class GetLeaderRequest extends
com.google.protobuf.GeneratedMessageV3 implements
// @@protoc_insertion_point(message_implements:raft.GetLeaderRequest)
GetLeaderRequestOrBuilder {
// Use GetLeaderRequest.newBuilder() to construct.
private GetLeaderRequest(com.google.protobuf.GeneratedMessageV3.Builder> builder) {
super(builder);
}
private GetLeaderRequest() {
}
@java.lang.Override
public final com.google.protobuf.UnknownFieldSet
getUnknownFields() {
return com.google.protobuf.UnknownFieldSet.getDefaultInstance();
}
private GetLeaderRequest(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
this();
try {
boolean done = false;
while (!done) {
int tag = input.readTag();
switch (tag) {
case 0:
done = true;
break;
default: {
if (!input.skipField(tag)) {
done = true;
}
break;
}
}
}
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.setUnfinishedMessage(this);
} catch (java.io.IOException e) {
throw new com.google.protobuf.InvalidProtocolBufferException(
e).setUnfinishedMessage(this);
} finally {
makeExtensionsImmutable();
}
}
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return com.github.wenweihu86.raft.proto.RaftMessage.internal_static_raft_GetLeaderRequest_descriptor;
}
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return com.github.wenweihu86.raft.proto.RaftMessage.internal_static_raft_GetLeaderRequest_fieldAccessorTable
.ensureFieldAccessorsInitialized(
com.github.wenweihu86.raft.proto.RaftMessage.GetLeaderRequest.class, com.github.wenweihu86.raft.proto.RaftMessage.GetLeaderRequest.Builder.class);
}
private byte memoizedIsInitialized = -1;
public final boolean isInitialized() {
byte isInitialized = memoizedIsInitialized;
if (isInitialized == 1) return true;
if (isInitialized == 0) return false;
memoizedIsInitialized = 1;
return true;
}
public void writeTo(com.google.protobuf.CodedOutputStream output)
throws java.io.IOException {
}
public int getSerializedSize() {
int size = memoizedSize;
if (size != -1) return size;
size = 0;
memoizedSize = size;
return size;
}
private static final long serialVersionUID = 0L;
@java.lang.Override
public boolean equals(final java.lang.Object obj) {
if (obj == this) {
return true;
}
if (!(obj instanceof com.github.wenweihu86.raft.proto.RaftMessage.GetLeaderRequest)) {
return super.equals(obj);
}
com.github.wenweihu86.raft.proto.RaftMessage.GetLeaderRequest other = (com.github.wenweihu86.raft.proto.RaftMessage.GetLeaderRequest) obj;
boolean result = true;
return result;
}
@java.lang.Override
public int hashCode() {
if (memoizedHashCode != 0) {
return memoizedHashCode;
}
int hash = 41;
hash = (19 * hash) + getDescriptorForType().hashCode();
hash = (29 * hash) + unknownFields.hashCode();
memoizedHashCode = hash;
return hash;
}
public static com.github.wenweihu86.raft.proto.RaftMessage.GetLeaderRequest parseFrom(
com.google.protobuf.ByteString data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static com.github.wenweihu86.raft.proto.RaftMessage.GetLeaderRequest parseFrom(
com.google.protobuf.ByteString data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static com.github.wenweihu86.raft.proto.RaftMessage.GetLeaderRequest parseFrom(byte[] data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static com.github.wenweihu86.raft.proto.RaftMessage.GetLeaderRequest parseFrom(
byte[] data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static com.github.wenweihu86.raft.proto.RaftMessage.GetLeaderRequest parseFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static com.github.wenweihu86.raft.proto.RaftMessage.GetLeaderRequest parseFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
public static com.github.wenweihu86.raft.proto.RaftMessage.GetLeaderRequest parseDelimitedFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input);
}
public static com.github.wenweihu86.raft.proto.RaftMessage.GetLeaderRequest parseDelimitedFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input, extensionRegistry);
}
public static com.github.wenweihu86.raft.proto.RaftMessage.GetLeaderRequest parseFrom(
com.google.protobuf.CodedInputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static com.github.wenweihu86.raft.proto.RaftMessage.GetLeaderRequest parseFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
public Builder newBuilderForType() { return newBuilder(); }
public static Builder newBuilder() {
return DEFAULT_INSTANCE.toBuilder();
}
public static Builder newBuilder(com.github.wenweihu86.raft.proto.RaftMessage.GetLeaderRequest prototype) {
return DEFAULT_INSTANCE.toBuilder().mergeFrom(prototype);
}
public Builder toBuilder() {
return this == DEFAULT_INSTANCE
? new Builder() : new Builder().mergeFrom(this);
}
@java.lang.Override
protected Builder newBuilderForType(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
Builder builder = new Builder(parent);
return builder;
}
/**
* Protobuf type {@code raft.GetLeaderRequest}
*/
public static final class Builder extends
com.google.protobuf.GeneratedMessageV3.Builder implements
// @@protoc_insertion_point(builder_implements:raft.GetLeaderRequest)
com.github.wenweihu86.raft.proto.RaftMessage.GetLeaderRequestOrBuilder {
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return com.github.wenweihu86.raft.proto.RaftMessage.internal_static_raft_GetLeaderRequest_descriptor;
}
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return com.github.wenweihu86.raft.proto.RaftMessage.internal_static_raft_GetLeaderRequest_fieldAccessorTable
.ensureFieldAccessorsInitialized(
com.github.wenweihu86.raft.proto.RaftMessage.GetLeaderRequest.class, com.github.wenweihu86.raft.proto.RaftMessage.GetLeaderRequest.Builder.class);
}
// Construct using com.github.wenweihu86.raft.proto.RaftMessage.GetLeaderRequest.newBuilder()
private Builder() {
maybeForceBuilderInitialization();
}
private Builder(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
super(parent);
maybeForceBuilderInitialization();
}
private void maybeForceBuilderInitialization() {
if (com.google.protobuf.GeneratedMessageV3
.alwaysUseFieldBuilders) {
}
}
public Builder clear() {
super.clear();
return this;
}
public com.google.protobuf.Descriptors.Descriptor
getDescriptorForType() {
return com.github.wenweihu86.raft.proto.RaftMessage.internal_static_raft_GetLeaderRequest_descriptor;
}
public com.github.wenweihu86.raft.proto.RaftMessage.GetLeaderRequest getDefaultInstanceForType() {
return com.github.wenweihu86.raft.proto.RaftMessage.GetLeaderRequest.getDefaultInstance();
}
public com.github.wenweihu86.raft.proto.RaftMessage.GetLeaderRequest build() {
com.github.wenweihu86.raft.proto.RaftMessage.GetLeaderRequest result = buildPartial();
if (!result.isInitialized()) {
throw newUninitializedMessageException(result);
}
return result;
}
public com.github.wenweihu86.raft.proto.RaftMessage.GetLeaderRequest buildPartial() {
com.github.wenweihu86.raft.proto.RaftMessage.GetLeaderRequest result = new com.github.wenweihu86.raft.proto.RaftMessage.GetLeaderRequest(this);
onBuilt();
return result;
}
public Builder clone() {
return (Builder) super.clone();
}
public Builder setField(
com.google.protobuf.Descriptors.FieldDescriptor field,
Object value) {
return (Builder) super.setField(field, value);
}
public Builder clearField(
com.google.protobuf.Descriptors.FieldDescriptor field) {
return (Builder) super.clearField(field);
}
public Builder clearOneof(
com.google.protobuf.Descriptors.OneofDescriptor oneof) {
return (Builder) super.clearOneof(oneof);
}
public Builder setRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
int index, Object value) {
return (Builder) super.setRepeatedField(field, index, value);
}
public Builder addRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
Object value) {
return (Builder) super.addRepeatedField(field, value);
}
public Builder mergeFrom(com.google.protobuf.Message other) {
if (other instanceof com.github.wenweihu86.raft.proto.RaftMessage.GetLeaderRequest) {
return mergeFrom((com.github.wenweihu86.raft.proto.RaftMessage.GetLeaderRequest)other);
} else {
super.mergeFrom(other);
return this;
}
}
public Builder mergeFrom(com.github.wenweihu86.raft.proto.RaftMessage.GetLeaderRequest other) {
if (other == com.github.wenweihu86.raft.proto.RaftMessage.GetLeaderRequest.getDefaultInstance()) return this;
onChanged();
return this;
}
public final boolean isInitialized() {
return true;
}
public Builder mergeFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
com.github.wenweihu86.raft.proto.RaftMessage.GetLeaderRequest parsedMessage = null;
try {
parsedMessage = PARSER.parsePartialFrom(input, extensionRegistry);
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
parsedMessage = (com.github.wenweihu86.raft.proto.RaftMessage.GetLeaderRequest) e.getUnfinishedMessage();
throw e.unwrapIOException();
} finally {
if (parsedMessage != null) {
mergeFrom(parsedMessage);
}
}
return this;
}
public final Builder setUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return this;
}
public final Builder mergeUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return this;
}
// @@protoc_insertion_point(builder_scope:raft.GetLeaderRequest)
}
// @@protoc_insertion_point(class_scope:raft.GetLeaderRequest)
private static final com.github.wenweihu86.raft.proto.RaftMessage.GetLeaderRequest DEFAULT_INSTANCE;
static {
DEFAULT_INSTANCE = new com.github.wenweihu86.raft.proto.RaftMessage.GetLeaderRequest();
}
public static com.github.wenweihu86.raft.proto.RaftMessage.GetLeaderRequest getDefaultInstance() {
return DEFAULT_INSTANCE;
}
private static final com.google.protobuf.Parser
PARSER = new com.google.protobuf.AbstractParser() {
public GetLeaderRequest parsePartialFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return new GetLeaderRequest(input, extensionRegistry);
}
};
public static com.google.protobuf.Parser parser() {
return PARSER;
}
@java.lang.Override
public com.google.protobuf.Parser getParserForType() {
return PARSER;
}
public com.github.wenweihu86.raft.proto.RaftMessage.GetLeaderRequest getDefaultInstanceForType() {
return DEFAULT_INSTANCE;
}
}
public interface GetLeaderResponseOrBuilder extends
// @@protoc_insertion_point(interface_extends:raft.GetLeaderResponse)
com.google.protobuf.MessageOrBuilder {
/**
* optional .raft.ResCode res_code = 1;
*/
int getResCodeValue();
/**
* optional .raft.ResCode res_code = 1;
*/
com.github.wenweihu86.raft.proto.RaftMessage.ResCode getResCode();
/**
* optional string res_msg = 2;
*/
java.lang.String getResMsg();
/**
* optional string res_msg = 2;
*/
com.google.protobuf.ByteString
getResMsgBytes();
/**
* optional .raft.EndPoint leader = 3;
*/
boolean hasLeader();
/**
* optional .raft.EndPoint leader = 3;
*/
com.github.wenweihu86.raft.proto.RaftMessage.EndPoint getLeader();
/**
* optional .raft.EndPoint leader = 3;
*/
com.github.wenweihu86.raft.proto.RaftMessage.EndPointOrBuilder getLeaderOrBuilder();
}
/**
* Protobuf type {@code raft.GetLeaderResponse}
*/
public static final class GetLeaderResponse extends
com.google.protobuf.GeneratedMessageV3 implements
// @@protoc_insertion_point(message_implements:raft.GetLeaderResponse)
GetLeaderResponseOrBuilder {
// Use GetLeaderResponse.newBuilder() to construct.
private GetLeaderResponse(com.google.protobuf.GeneratedMessageV3.Builder> builder) {
super(builder);
}
private GetLeaderResponse() {
resCode_ = 0;
resMsg_ = "";
}
@java.lang.Override
public final com.google.protobuf.UnknownFieldSet
getUnknownFields() {
return com.google.protobuf.UnknownFieldSet.getDefaultInstance();
}
private GetLeaderResponse(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
this();
int mutable_bitField0_ = 0;
try {
boolean done = false;
while (!done) {
int tag = input.readTag();
switch (tag) {
case 0:
done = true;
break;
default: {
if (!input.skipField(tag)) {
done = true;
}
break;
}
case 8: {
int rawValue = input.readEnum();
resCode_ = rawValue;
break;
}
case 18: {
java.lang.String s = input.readStringRequireUtf8();
resMsg_ = s;
break;
}
case 26: {
com.github.wenweihu86.raft.proto.RaftMessage.EndPoint.Builder subBuilder = null;
if (leader_ != null) {
subBuilder = leader_.toBuilder();
}
leader_ = input.readMessage(com.github.wenweihu86.raft.proto.RaftMessage.EndPoint.parser(), extensionRegistry);
if (subBuilder != null) {
subBuilder.mergeFrom(leader_);
leader_ = subBuilder.buildPartial();
}
break;
}
}
}
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.setUnfinishedMessage(this);
} catch (java.io.IOException e) {
throw new com.google.protobuf.InvalidProtocolBufferException(
e).setUnfinishedMessage(this);
} finally {
makeExtensionsImmutable();
}
}
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return com.github.wenweihu86.raft.proto.RaftMessage.internal_static_raft_GetLeaderResponse_descriptor;
}
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return com.github.wenweihu86.raft.proto.RaftMessage.internal_static_raft_GetLeaderResponse_fieldAccessorTable
.ensureFieldAccessorsInitialized(
com.github.wenweihu86.raft.proto.RaftMessage.GetLeaderResponse.class, com.github.wenweihu86.raft.proto.RaftMessage.GetLeaderResponse.Builder.class);
}
public static final int RES_CODE_FIELD_NUMBER = 1;
private int resCode_;
/**
* optional .raft.ResCode res_code = 1;
*/
public int getResCodeValue() {
return resCode_;
}
/**
* optional .raft.ResCode res_code = 1;
*/
public com.github.wenweihu86.raft.proto.RaftMessage.ResCode getResCode() {
com.github.wenweihu86.raft.proto.RaftMessage.ResCode result = com.github.wenweihu86.raft.proto.RaftMessage.ResCode.valueOf(resCode_);
return result == null ? com.github.wenweihu86.raft.proto.RaftMessage.ResCode.UNRECOGNIZED : result;
}
public static final int RES_MSG_FIELD_NUMBER = 2;
private volatile java.lang.Object resMsg_;
/**
* optional string res_msg = 2;
*/
public java.lang.String getResMsg() {
java.lang.Object ref = resMsg_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
resMsg_ = s;
return s;
}
}
/**
* optional string res_msg = 2;
*/
public com.google.protobuf.ByteString
getResMsgBytes() {
java.lang.Object ref = resMsg_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
resMsg_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
public static final int LEADER_FIELD_NUMBER = 3;
private com.github.wenweihu86.raft.proto.RaftMessage.EndPoint leader_;
/**
* optional .raft.EndPoint leader = 3;
*/
public boolean hasLeader() {
return leader_ != null;
}
/**
* optional .raft.EndPoint leader = 3;
*/
public com.github.wenweihu86.raft.proto.RaftMessage.EndPoint getLeader() {
return leader_ == null ? com.github.wenweihu86.raft.proto.RaftMessage.EndPoint.getDefaultInstance() : leader_;
}
/**
* optional .raft.EndPoint leader = 3;
*/
public com.github.wenweihu86.raft.proto.RaftMessage.EndPointOrBuilder getLeaderOrBuilder() {
return getLeader();
}
private byte memoizedIsInitialized = -1;
public final boolean isInitialized() {
byte isInitialized = memoizedIsInitialized;
if (isInitialized == 1) return true;
if (isInitialized == 0) return false;
memoizedIsInitialized = 1;
return true;
}
public void writeTo(com.google.protobuf.CodedOutputStream output)
throws java.io.IOException {
if (resCode_ != com.github.wenweihu86.raft.proto.RaftMessage.ResCode.RES_CODE_SUCCESS.getNumber()) {
output.writeEnum(1, resCode_);
}
if (!getResMsgBytes().isEmpty()) {
com.google.protobuf.GeneratedMessageV3.writeString(output, 2, resMsg_);
}
if (leader_ != null) {
output.writeMessage(3, getLeader());
}
}
public int getSerializedSize() {
int size = memoizedSize;
if (size != -1) return size;
size = 0;
if (resCode_ != com.github.wenweihu86.raft.proto.RaftMessage.ResCode.RES_CODE_SUCCESS.getNumber()) {
size += com.google.protobuf.CodedOutputStream
.computeEnumSize(1, resCode_);
}
if (!getResMsgBytes().isEmpty()) {
size += com.google.protobuf.GeneratedMessageV3.computeStringSize(2, resMsg_);
}
if (leader_ != null) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(3, getLeader());
}
memoizedSize = size;
return size;
}
private static final long serialVersionUID = 0L;
@java.lang.Override
public boolean equals(final java.lang.Object obj) {
if (obj == this) {
return true;
}
if (!(obj instanceof com.github.wenweihu86.raft.proto.RaftMessage.GetLeaderResponse)) {
return super.equals(obj);
}
com.github.wenweihu86.raft.proto.RaftMessage.GetLeaderResponse other = (com.github.wenweihu86.raft.proto.RaftMessage.GetLeaderResponse) obj;
boolean result = true;
result = result && resCode_ == other.resCode_;
result = result && getResMsg()
.equals(other.getResMsg());
result = result && (hasLeader() == other.hasLeader());
if (hasLeader()) {
result = result && getLeader()
.equals(other.getLeader());
}
return result;
}
@java.lang.Override
public int hashCode() {
if (memoizedHashCode != 0) {
return memoizedHashCode;
}
int hash = 41;
hash = (19 * hash) + getDescriptorForType().hashCode();
hash = (37 * hash) + RES_CODE_FIELD_NUMBER;
hash = (53 * hash) + resCode_;
hash = (37 * hash) + RES_MSG_FIELD_NUMBER;
hash = (53 * hash) + getResMsg().hashCode();
if (hasLeader()) {
hash = (37 * hash) + LEADER_FIELD_NUMBER;
hash = (53 * hash) + getLeader().hashCode();
}
hash = (29 * hash) + unknownFields.hashCode();
memoizedHashCode = hash;
return hash;
}
public static com.github.wenweihu86.raft.proto.RaftMessage.GetLeaderResponse parseFrom(
com.google.protobuf.ByteString data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static com.github.wenweihu86.raft.proto.RaftMessage.GetLeaderResponse parseFrom(
com.google.protobuf.ByteString data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static com.github.wenweihu86.raft.proto.RaftMessage.GetLeaderResponse parseFrom(byte[] data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static com.github.wenweihu86.raft.proto.RaftMessage.GetLeaderResponse parseFrom(
byte[] data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static com.github.wenweihu86.raft.proto.RaftMessage.GetLeaderResponse parseFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static com.github.wenweihu86.raft.proto.RaftMessage.GetLeaderResponse parseFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
public static com.github.wenweihu86.raft.proto.RaftMessage.GetLeaderResponse parseDelimitedFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input);
}
public static com.github.wenweihu86.raft.proto.RaftMessage.GetLeaderResponse parseDelimitedFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input, extensionRegistry);
}
public static com.github.wenweihu86.raft.proto.RaftMessage.GetLeaderResponse parseFrom(
com.google.protobuf.CodedInputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static com.github.wenweihu86.raft.proto.RaftMessage.GetLeaderResponse parseFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
public Builder newBuilderForType() { return newBuilder(); }
public static Builder newBuilder() {
return DEFAULT_INSTANCE.toBuilder();
}
public static Builder newBuilder(com.github.wenweihu86.raft.proto.RaftMessage.GetLeaderResponse prototype) {
return DEFAULT_INSTANCE.toBuilder().mergeFrom(prototype);
}
public Builder toBuilder() {
return this == DEFAULT_INSTANCE
? new Builder() : new Builder().mergeFrom(this);
}
@java.lang.Override
protected Builder newBuilderForType(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
Builder builder = new Builder(parent);
return builder;
}
/**
* Protobuf type {@code raft.GetLeaderResponse}
*/
public static final class Builder extends
com.google.protobuf.GeneratedMessageV3.Builder implements
// @@protoc_insertion_point(builder_implements:raft.GetLeaderResponse)
com.github.wenweihu86.raft.proto.RaftMessage.GetLeaderResponseOrBuilder {
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return com.github.wenweihu86.raft.proto.RaftMessage.internal_static_raft_GetLeaderResponse_descriptor;
}
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return com.github.wenweihu86.raft.proto.RaftMessage.internal_static_raft_GetLeaderResponse_fieldAccessorTable
.ensureFieldAccessorsInitialized(
com.github.wenweihu86.raft.proto.RaftMessage.GetLeaderResponse.class, com.github.wenweihu86.raft.proto.RaftMessage.GetLeaderResponse.Builder.class);
}
// Construct using com.github.wenweihu86.raft.proto.RaftMessage.GetLeaderResponse.newBuilder()
private Builder() {
maybeForceBuilderInitialization();
}
private Builder(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
super(parent);
maybeForceBuilderInitialization();
}
private void maybeForceBuilderInitialization() {
if (com.google.protobuf.GeneratedMessageV3
.alwaysUseFieldBuilders) {
}
}
public Builder clear() {
super.clear();
resCode_ = 0;
resMsg_ = "";
if (leaderBuilder_ == null) {
leader_ = null;
} else {
leader_ = null;
leaderBuilder_ = null;
}
return this;
}
public com.google.protobuf.Descriptors.Descriptor
getDescriptorForType() {
return com.github.wenweihu86.raft.proto.RaftMessage.internal_static_raft_GetLeaderResponse_descriptor;
}
public com.github.wenweihu86.raft.proto.RaftMessage.GetLeaderResponse getDefaultInstanceForType() {
return com.github.wenweihu86.raft.proto.RaftMessage.GetLeaderResponse.getDefaultInstance();
}
public com.github.wenweihu86.raft.proto.RaftMessage.GetLeaderResponse build() {
com.github.wenweihu86.raft.proto.RaftMessage.GetLeaderResponse result = buildPartial();
if (!result.isInitialized()) {
throw newUninitializedMessageException(result);
}
return result;
}
public com.github.wenweihu86.raft.proto.RaftMessage.GetLeaderResponse buildPartial() {
com.github.wenweihu86.raft.proto.RaftMessage.GetLeaderResponse result = new com.github.wenweihu86.raft.proto.RaftMessage.GetLeaderResponse(this);
result.resCode_ = resCode_;
result.resMsg_ = resMsg_;
if (leaderBuilder_ == null) {
result.leader_ = leader_;
} else {
result.leader_ = leaderBuilder_.build();
}
onBuilt();
return result;
}
public Builder clone() {
return (Builder) super.clone();
}
public Builder setField(
com.google.protobuf.Descriptors.FieldDescriptor field,
Object value) {
return (Builder) super.setField(field, value);
}
public Builder clearField(
com.google.protobuf.Descriptors.FieldDescriptor field) {
return (Builder) super.clearField(field);
}
public Builder clearOneof(
com.google.protobuf.Descriptors.OneofDescriptor oneof) {
return (Builder) super.clearOneof(oneof);
}
public Builder setRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
int index, Object value) {
return (Builder) super.setRepeatedField(field, index, value);
}
public Builder addRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
Object value) {
return (Builder) super.addRepeatedField(field, value);
}
public Builder mergeFrom(com.google.protobuf.Message other) {
if (other instanceof com.github.wenweihu86.raft.proto.RaftMessage.GetLeaderResponse) {
return mergeFrom((com.github.wenweihu86.raft.proto.RaftMessage.GetLeaderResponse)other);
} else {
super.mergeFrom(other);
return this;
}
}
public Builder mergeFrom(com.github.wenweihu86.raft.proto.RaftMessage.GetLeaderResponse other) {
if (other == com.github.wenweihu86.raft.proto.RaftMessage.GetLeaderResponse.getDefaultInstance()) return this;
if (other.resCode_ != 0) {
setResCodeValue(other.getResCodeValue());
}
if (!other.getResMsg().isEmpty()) {
resMsg_ = other.resMsg_;
onChanged();
}
if (other.hasLeader()) {
mergeLeader(other.getLeader());
}
onChanged();
return this;
}
public final boolean isInitialized() {
return true;
}
public Builder mergeFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
com.github.wenweihu86.raft.proto.RaftMessage.GetLeaderResponse parsedMessage = null;
try {
parsedMessage = PARSER.parsePartialFrom(input, extensionRegistry);
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
parsedMessage = (com.github.wenweihu86.raft.proto.RaftMessage.GetLeaderResponse) e.getUnfinishedMessage();
throw e.unwrapIOException();
} finally {
if (parsedMessage != null) {
mergeFrom(parsedMessage);
}
}
return this;
}
private int resCode_ = 0;
/**
* optional .raft.ResCode res_code = 1;
*/
public int getResCodeValue() {
return resCode_;
}
/**
* optional .raft.ResCode res_code = 1;
*/
public Builder setResCodeValue(int value) {
resCode_ = value;
onChanged();
return this;
}
/**
* optional .raft.ResCode res_code = 1;
*/
public com.github.wenweihu86.raft.proto.RaftMessage.ResCode getResCode() {
com.github.wenweihu86.raft.proto.RaftMessage.ResCode result = com.github.wenweihu86.raft.proto.RaftMessage.ResCode.valueOf(resCode_);
return result == null ? com.github.wenweihu86.raft.proto.RaftMessage.ResCode.UNRECOGNIZED : result;
}
/**
* optional .raft.ResCode res_code = 1;
*/
public Builder setResCode(com.github.wenweihu86.raft.proto.RaftMessage.ResCode value) {
if (value == null) {
throw new NullPointerException();
}
resCode_ = value.getNumber();
onChanged();
return this;
}
/**
* optional .raft.ResCode res_code = 1;
*/
public Builder clearResCode() {
resCode_ = 0;
onChanged();
return this;
}
private java.lang.Object resMsg_ = "";
/**
* optional string res_msg = 2;
*/
public java.lang.String getResMsg() {
java.lang.Object ref = resMsg_;
if (!(ref instanceof java.lang.String)) {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
resMsg_ = s;
return s;
} else {
return (java.lang.String) ref;
}
}
/**
* optional string res_msg = 2;
*/
public com.google.protobuf.ByteString
getResMsgBytes() {
java.lang.Object ref = resMsg_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
resMsg_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
* optional string res_msg = 2;
*/
public Builder setResMsg(
java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
resMsg_ = value;
onChanged();
return this;
}
/**
* optional string res_msg = 2;
*/
public Builder clearResMsg() {
resMsg_ = getDefaultInstance().getResMsg();
onChanged();
return this;
}
/**
* optional string res_msg = 2;
*/
public Builder setResMsgBytes(
com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
checkByteStringIsUtf8(value);
resMsg_ = value;
onChanged();
return this;
}
private com.github.wenweihu86.raft.proto.RaftMessage.EndPoint leader_ = null;
private com.google.protobuf.SingleFieldBuilderV3<
com.github.wenweihu86.raft.proto.RaftMessage.EndPoint, com.github.wenweihu86.raft.proto.RaftMessage.EndPoint.Builder, com.github.wenweihu86.raft.proto.RaftMessage.EndPointOrBuilder> leaderBuilder_;
/**
* optional .raft.EndPoint leader = 3;
*/
public boolean hasLeader() {
return leaderBuilder_ != null || leader_ != null;
}
/**
* optional .raft.EndPoint leader = 3;
*/
public com.github.wenweihu86.raft.proto.RaftMessage.EndPoint getLeader() {
if (leaderBuilder_ == null) {
return leader_ == null ? com.github.wenweihu86.raft.proto.RaftMessage.EndPoint.getDefaultInstance() : leader_;
} else {
return leaderBuilder_.getMessage();
}
}
/**
* optional .raft.EndPoint leader = 3;
*/
public Builder setLeader(com.github.wenweihu86.raft.proto.RaftMessage.EndPoint value) {
if (leaderBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
leader_ = value;
onChanged();
} else {
leaderBuilder_.setMessage(value);
}
return this;
}
/**
* optional .raft.EndPoint leader = 3;
*/
public Builder setLeader(
com.github.wenweihu86.raft.proto.RaftMessage.EndPoint.Builder builderForValue) {
if (leaderBuilder_ == null) {
leader_ = builderForValue.build();
onChanged();
} else {
leaderBuilder_.setMessage(builderForValue.build());
}
return this;
}
/**
* optional .raft.EndPoint leader = 3;
*/
public Builder mergeLeader(com.github.wenweihu86.raft.proto.RaftMessage.EndPoint value) {
if (leaderBuilder_ == null) {
if (leader_ != null) {
leader_ =
com.github.wenweihu86.raft.proto.RaftMessage.EndPoint.newBuilder(leader_).mergeFrom(value).buildPartial();
} else {
leader_ = value;
}
onChanged();
} else {
leaderBuilder_.mergeFrom(value);
}
return this;
}
/**
* optional .raft.EndPoint leader = 3;
*/
public Builder clearLeader() {
if (leaderBuilder_ == null) {
leader_ = null;
onChanged();
} else {
leader_ = null;
leaderBuilder_ = null;
}
return this;
}
/**
* optional .raft.EndPoint leader = 3;
*/
public com.github.wenweihu86.raft.proto.RaftMessage.EndPoint.Builder getLeaderBuilder() {
onChanged();
return getLeaderFieldBuilder().getBuilder();
}
/**
* optional .raft.EndPoint leader = 3;
*/
public com.github.wenweihu86.raft.proto.RaftMessage.EndPointOrBuilder getLeaderOrBuilder() {
if (leaderBuilder_ != null) {
return leaderBuilder_.getMessageOrBuilder();
} else {
return leader_ == null ?
com.github.wenweihu86.raft.proto.RaftMessage.EndPoint.getDefaultInstance() : leader_;
}
}
/**
* optional .raft.EndPoint leader = 3;
*/
private com.google.protobuf.SingleFieldBuilderV3<
com.github.wenweihu86.raft.proto.RaftMessage.EndPoint, com.github.wenweihu86.raft.proto.RaftMessage.EndPoint.Builder, com.github.wenweihu86.raft.proto.RaftMessage.EndPointOrBuilder>
getLeaderFieldBuilder() {
if (leaderBuilder_ == null) {
leaderBuilder_ = new com.google.protobuf.SingleFieldBuilderV3<
com.github.wenweihu86.raft.proto.RaftMessage.EndPoint, com.github.wenweihu86.raft.proto.RaftMessage.EndPoint.Builder, com.github.wenweihu86.raft.proto.RaftMessage.EndPointOrBuilder>(
getLeader(),
getParentForChildren(),
isClean());
leader_ = null;
}
return leaderBuilder_;
}
public final Builder setUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return this;
}
public final Builder mergeUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return this;
}
// @@protoc_insertion_point(builder_scope:raft.GetLeaderResponse)
}
// @@protoc_insertion_point(class_scope:raft.GetLeaderResponse)
private static final com.github.wenweihu86.raft.proto.RaftMessage.GetLeaderResponse DEFAULT_INSTANCE;
static {
DEFAULT_INSTANCE = new com.github.wenweihu86.raft.proto.RaftMessage.GetLeaderResponse();
}
public static com.github.wenweihu86.raft.proto.RaftMessage.GetLeaderResponse getDefaultInstance() {
return DEFAULT_INSTANCE;
}
private static final com.google.protobuf.Parser
PARSER = new com.google.protobuf.AbstractParser() {
public GetLeaderResponse parsePartialFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return new GetLeaderResponse(input, extensionRegistry);
}
};
public static com.google.protobuf.Parser parser() {
return PARSER;
}
@java.lang.Override
public com.google.protobuf.Parser getParserForType() {
return PARSER;
}
public com.github.wenweihu86.raft.proto.RaftMessage.GetLeaderResponse getDefaultInstanceForType() {
return DEFAULT_INSTANCE;
}
}
public interface AddPeersRequestOrBuilder extends
// @@protoc_insertion_point(interface_extends:raft.AddPeersRequest)
com.google.protobuf.MessageOrBuilder {
/**
* repeated .raft.Server servers = 1;
*/
java.util.List
getServersList();
/**
* repeated .raft.Server servers = 1;
*/
com.github.wenweihu86.raft.proto.RaftMessage.Server getServers(int index);
/**
* repeated .raft.Server servers = 1;
*/
int getServersCount();
/**
* repeated .raft.Server servers = 1;
*/
java.util.List extends com.github.wenweihu86.raft.proto.RaftMessage.ServerOrBuilder>
getServersOrBuilderList();
/**
* repeated .raft.Server servers = 1;
*/
com.github.wenweihu86.raft.proto.RaftMessage.ServerOrBuilder getServersOrBuilder(
int index);
}
/**
* Protobuf type {@code raft.AddPeersRequest}
*/
public static final class AddPeersRequest extends
com.google.protobuf.GeneratedMessageV3 implements
// @@protoc_insertion_point(message_implements:raft.AddPeersRequest)
AddPeersRequestOrBuilder {
// Use AddPeersRequest.newBuilder() to construct.
private AddPeersRequest(com.google.protobuf.GeneratedMessageV3.Builder> builder) {
super(builder);
}
private AddPeersRequest() {
servers_ = java.util.Collections.emptyList();
}
@java.lang.Override
public final com.google.protobuf.UnknownFieldSet
getUnknownFields() {
return com.google.protobuf.UnknownFieldSet.getDefaultInstance();
}
private AddPeersRequest(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
this();
int mutable_bitField0_ = 0;
try {
boolean done = false;
while (!done) {
int tag = input.readTag();
switch (tag) {
case 0:
done = true;
break;
default: {
if (!input.skipField(tag)) {
done = true;
}
break;
}
case 10: {
if (!((mutable_bitField0_ & 0x00000001) == 0x00000001)) {
servers_ = new java.util.ArrayList();
mutable_bitField0_ |= 0x00000001;
}
servers_.add(
input.readMessage(com.github.wenweihu86.raft.proto.RaftMessage.Server.parser(), extensionRegistry));
break;
}
}
}
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.setUnfinishedMessage(this);
} catch (java.io.IOException e) {
throw new com.google.protobuf.InvalidProtocolBufferException(
e).setUnfinishedMessage(this);
} finally {
if (((mutable_bitField0_ & 0x00000001) == 0x00000001)) {
servers_ = java.util.Collections.unmodifiableList(servers_);
}
makeExtensionsImmutable();
}
}
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return com.github.wenweihu86.raft.proto.RaftMessage.internal_static_raft_AddPeersRequest_descriptor;
}
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return com.github.wenweihu86.raft.proto.RaftMessage.internal_static_raft_AddPeersRequest_fieldAccessorTable
.ensureFieldAccessorsInitialized(
com.github.wenweihu86.raft.proto.RaftMessage.AddPeersRequest.class, com.github.wenweihu86.raft.proto.RaftMessage.AddPeersRequest.Builder.class);
}
public static final int SERVERS_FIELD_NUMBER = 1;
private java.util.List servers_;
/**
* repeated .raft.Server servers = 1;
*/
public java.util.List getServersList() {
return servers_;
}
/**
* repeated .raft.Server servers = 1;
*/
public java.util.List extends com.github.wenweihu86.raft.proto.RaftMessage.ServerOrBuilder>
getServersOrBuilderList() {
return servers_;
}
/**
* repeated .raft.Server servers = 1;
*/
public int getServersCount() {
return servers_.size();
}
/**
* repeated .raft.Server servers = 1;
*/
public com.github.wenweihu86.raft.proto.RaftMessage.Server getServers(int index) {
return servers_.get(index);
}
/**
* repeated .raft.Server servers = 1;
*/
public com.github.wenweihu86.raft.proto.RaftMessage.ServerOrBuilder getServersOrBuilder(
int index) {
return servers_.get(index);
}
private byte memoizedIsInitialized = -1;
public final boolean isInitialized() {
byte isInitialized = memoizedIsInitialized;
if (isInitialized == 1) return true;
if (isInitialized == 0) return false;
memoizedIsInitialized = 1;
return true;
}
public void writeTo(com.google.protobuf.CodedOutputStream output)
throws java.io.IOException {
for (int i = 0; i < servers_.size(); i++) {
output.writeMessage(1, servers_.get(i));
}
}
public int getSerializedSize() {
int size = memoizedSize;
if (size != -1) return size;
size = 0;
for (int i = 0; i < servers_.size(); i++) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(1, servers_.get(i));
}
memoizedSize = size;
return size;
}
private static final long serialVersionUID = 0L;
@java.lang.Override
public boolean equals(final java.lang.Object obj) {
if (obj == this) {
return true;
}
if (!(obj instanceof com.github.wenweihu86.raft.proto.RaftMessage.AddPeersRequest)) {
return super.equals(obj);
}
com.github.wenweihu86.raft.proto.RaftMessage.AddPeersRequest other = (com.github.wenweihu86.raft.proto.RaftMessage.AddPeersRequest) obj;
boolean result = true;
result = result && getServersList()
.equals(other.getServersList());
return result;
}
@java.lang.Override
public int hashCode() {
if (memoizedHashCode != 0) {
return memoizedHashCode;
}
int hash = 41;
hash = (19 * hash) + getDescriptorForType().hashCode();
if (getServersCount() > 0) {
hash = (37 * hash) + SERVERS_FIELD_NUMBER;
hash = (53 * hash) + getServersList().hashCode();
}
hash = (29 * hash) + unknownFields.hashCode();
memoizedHashCode = hash;
return hash;
}
public static com.github.wenweihu86.raft.proto.RaftMessage.AddPeersRequest parseFrom(
com.google.protobuf.ByteString data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static com.github.wenweihu86.raft.proto.RaftMessage.AddPeersRequest parseFrom(
com.google.protobuf.ByteString data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static com.github.wenweihu86.raft.proto.RaftMessage.AddPeersRequest parseFrom(byte[] data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static com.github.wenweihu86.raft.proto.RaftMessage.AddPeersRequest parseFrom(
byte[] data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static com.github.wenweihu86.raft.proto.RaftMessage.AddPeersRequest parseFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static com.github.wenweihu86.raft.proto.RaftMessage.AddPeersRequest parseFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
public static com.github.wenweihu86.raft.proto.RaftMessage.AddPeersRequest parseDelimitedFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input);
}
public static com.github.wenweihu86.raft.proto.RaftMessage.AddPeersRequest parseDelimitedFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input, extensionRegistry);
}
public static com.github.wenweihu86.raft.proto.RaftMessage.AddPeersRequest parseFrom(
com.google.protobuf.CodedInputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static com.github.wenweihu86.raft.proto.RaftMessage.AddPeersRequest parseFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
public Builder newBuilderForType() { return newBuilder(); }
public static Builder newBuilder() {
return DEFAULT_INSTANCE.toBuilder();
}
public static Builder newBuilder(com.github.wenweihu86.raft.proto.RaftMessage.AddPeersRequest prototype) {
return DEFAULT_INSTANCE.toBuilder().mergeFrom(prototype);
}
public Builder toBuilder() {
return this == DEFAULT_INSTANCE
? new Builder() : new Builder().mergeFrom(this);
}
@java.lang.Override
protected Builder newBuilderForType(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
Builder builder = new Builder(parent);
return builder;
}
/**
* Protobuf type {@code raft.AddPeersRequest}
*/
public static final class Builder extends
com.google.protobuf.GeneratedMessageV3.Builder implements
// @@protoc_insertion_point(builder_implements:raft.AddPeersRequest)
com.github.wenweihu86.raft.proto.RaftMessage.AddPeersRequestOrBuilder {
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return com.github.wenweihu86.raft.proto.RaftMessage.internal_static_raft_AddPeersRequest_descriptor;
}
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return com.github.wenweihu86.raft.proto.RaftMessage.internal_static_raft_AddPeersRequest_fieldAccessorTable
.ensureFieldAccessorsInitialized(
com.github.wenweihu86.raft.proto.RaftMessage.AddPeersRequest.class, com.github.wenweihu86.raft.proto.RaftMessage.AddPeersRequest.Builder.class);
}
// Construct using com.github.wenweihu86.raft.proto.RaftMessage.AddPeersRequest.newBuilder()
private Builder() {
maybeForceBuilderInitialization();
}
private Builder(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
super(parent);
maybeForceBuilderInitialization();
}
private void maybeForceBuilderInitialization() {
if (com.google.protobuf.GeneratedMessageV3
.alwaysUseFieldBuilders) {
getServersFieldBuilder();
}
}
public Builder clear() {
super.clear();
if (serversBuilder_ == null) {
servers_ = java.util.Collections.emptyList();
bitField0_ = (bitField0_ & ~0x00000001);
} else {
serversBuilder_.clear();
}
return this;
}
public com.google.protobuf.Descriptors.Descriptor
getDescriptorForType() {
return com.github.wenweihu86.raft.proto.RaftMessage.internal_static_raft_AddPeersRequest_descriptor;
}
public com.github.wenweihu86.raft.proto.RaftMessage.AddPeersRequest getDefaultInstanceForType() {
return com.github.wenweihu86.raft.proto.RaftMessage.AddPeersRequest.getDefaultInstance();
}
public com.github.wenweihu86.raft.proto.RaftMessage.AddPeersRequest build() {
com.github.wenweihu86.raft.proto.RaftMessage.AddPeersRequest result = buildPartial();
if (!result.isInitialized()) {
throw newUninitializedMessageException(result);
}
return result;
}
public com.github.wenweihu86.raft.proto.RaftMessage.AddPeersRequest buildPartial() {
com.github.wenweihu86.raft.proto.RaftMessage.AddPeersRequest result = new com.github.wenweihu86.raft.proto.RaftMessage.AddPeersRequest(this);
int from_bitField0_ = bitField0_;
if (serversBuilder_ == null) {
if (((bitField0_ & 0x00000001) == 0x00000001)) {
servers_ = java.util.Collections.unmodifiableList(servers_);
bitField0_ = (bitField0_ & ~0x00000001);
}
result.servers_ = servers_;
} else {
result.servers_ = serversBuilder_.build();
}
onBuilt();
return result;
}
public Builder clone() {
return (Builder) super.clone();
}
public Builder setField(
com.google.protobuf.Descriptors.FieldDescriptor field,
Object value) {
return (Builder) super.setField(field, value);
}
public Builder clearField(
com.google.protobuf.Descriptors.FieldDescriptor field) {
return (Builder) super.clearField(field);
}
public Builder clearOneof(
com.google.protobuf.Descriptors.OneofDescriptor oneof) {
return (Builder) super.clearOneof(oneof);
}
public Builder setRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
int index, Object value) {
return (Builder) super.setRepeatedField(field, index, value);
}
public Builder addRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
Object value) {
return (Builder) super.addRepeatedField(field, value);
}
public Builder mergeFrom(com.google.protobuf.Message other) {
if (other instanceof com.github.wenweihu86.raft.proto.RaftMessage.AddPeersRequest) {
return mergeFrom((com.github.wenweihu86.raft.proto.RaftMessage.AddPeersRequest)other);
} else {
super.mergeFrom(other);
return this;
}
}
public Builder mergeFrom(com.github.wenweihu86.raft.proto.RaftMessage.AddPeersRequest other) {
if (other == com.github.wenweihu86.raft.proto.RaftMessage.AddPeersRequest.getDefaultInstance()) return this;
if (serversBuilder_ == null) {
if (!other.servers_.isEmpty()) {
if (servers_.isEmpty()) {
servers_ = other.servers_;
bitField0_ = (bitField0_ & ~0x00000001);
} else {
ensureServersIsMutable();
servers_.addAll(other.servers_);
}
onChanged();
}
} else {
if (!other.servers_.isEmpty()) {
if (serversBuilder_.isEmpty()) {
serversBuilder_.dispose();
serversBuilder_ = null;
servers_ = other.servers_;
bitField0_ = (bitField0_ & ~0x00000001);
serversBuilder_ =
com.google.protobuf.GeneratedMessageV3.alwaysUseFieldBuilders ?
getServersFieldBuilder() : null;
} else {
serversBuilder_.addAllMessages(other.servers_);
}
}
}
onChanged();
return this;
}
public final boolean isInitialized() {
return true;
}
public Builder mergeFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
com.github.wenweihu86.raft.proto.RaftMessage.AddPeersRequest parsedMessage = null;
try {
parsedMessage = PARSER.parsePartialFrom(input, extensionRegistry);
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
parsedMessage = (com.github.wenweihu86.raft.proto.RaftMessage.AddPeersRequest) e.getUnfinishedMessage();
throw e.unwrapIOException();
} finally {
if (parsedMessage != null) {
mergeFrom(parsedMessage);
}
}
return this;
}
private int bitField0_;
private java.util.List servers_ =
java.util.Collections.emptyList();
private void ensureServersIsMutable() {
if (!((bitField0_ & 0x00000001) == 0x00000001)) {
servers_ = new java.util.ArrayList(servers_);
bitField0_ |= 0x00000001;
}
}
private com.google.protobuf.RepeatedFieldBuilderV3<
com.github.wenweihu86.raft.proto.RaftMessage.Server, com.github.wenweihu86.raft.proto.RaftMessage.Server.Builder, com.github.wenweihu86.raft.proto.RaftMessage.ServerOrBuilder> serversBuilder_;
/**
* repeated .raft.Server servers = 1;
*/
public java.util.List getServersList() {
if (serversBuilder_ == null) {
return java.util.Collections.unmodifiableList(servers_);
} else {
return serversBuilder_.getMessageList();
}
}
/**
* repeated .raft.Server servers = 1;
*/
public int getServersCount() {
if (serversBuilder_ == null) {
return servers_.size();
} else {
return serversBuilder_.getCount();
}
}
/**
* repeated .raft.Server servers = 1;
*/
public com.github.wenweihu86.raft.proto.RaftMessage.Server getServers(int index) {
if (serversBuilder_ == null) {
return servers_.get(index);
} else {
return serversBuilder_.getMessage(index);
}
}
/**
* repeated .raft.Server servers = 1;
*/
public Builder setServers(
int index, com.github.wenweihu86.raft.proto.RaftMessage.Server value) {
if (serversBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
ensureServersIsMutable();
servers_.set(index, value);
onChanged();
} else {
serversBuilder_.setMessage(index, value);
}
return this;
}
/**
* repeated .raft.Server servers = 1;
*/
public Builder setServers(
int index, com.github.wenweihu86.raft.proto.RaftMessage.Server.Builder builderForValue) {
if (serversBuilder_ == null) {
ensureServersIsMutable();
servers_.set(index, builderForValue.build());
onChanged();
} else {
serversBuilder_.setMessage(index, builderForValue.build());
}
return this;
}
/**
* repeated .raft.Server servers = 1;
*/
public Builder addServers(com.github.wenweihu86.raft.proto.RaftMessage.Server value) {
if (serversBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
ensureServersIsMutable();
servers_.add(value);
onChanged();
} else {
serversBuilder_.addMessage(value);
}
return this;
}
/**
* repeated .raft.Server servers = 1;
*/
public Builder addServers(
int index, com.github.wenweihu86.raft.proto.RaftMessage.Server value) {
if (serversBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
ensureServersIsMutable();
servers_.add(index, value);
onChanged();
} else {
serversBuilder_.addMessage(index, value);
}
return this;
}
/**
* repeated .raft.Server servers = 1;
*/
public Builder addServers(
com.github.wenweihu86.raft.proto.RaftMessage.Server.Builder builderForValue) {
if (serversBuilder_ == null) {
ensureServersIsMutable();
servers_.add(builderForValue.build());
onChanged();
} else {
serversBuilder_.addMessage(builderForValue.build());
}
return this;
}
/**
* repeated .raft.Server servers = 1;
*/
public Builder addServers(
int index, com.github.wenweihu86.raft.proto.RaftMessage.Server.Builder builderForValue) {
if (serversBuilder_ == null) {
ensureServersIsMutable();
servers_.add(index, builderForValue.build());
onChanged();
} else {
serversBuilder_.addMessage(index, builderForValue.build());
}
return this;
}
/**
* repeated .raft.Server servers = 1;
*/
public Builder addAllServers(
java.lang.Iterable extends com.github.wenweihu86.raft.proto.RaftMessage.Server> values) {
if (serversBuilder_ == null) {
ensureServersIsMutable();
com.google.protobuf.AbstractMessageLite.Builder.addAll(
values, servers_);
onChanged();
} else {
serversBuilder_.addAllMessages(values);
}
return this;
}
/**
* repeated .raft.Server servers = 1;
*/
public Builder clearServers() {
if (serversBuilder_ == null) {
servers_ = java.util.Collections.emptyList();
bitField0_ = (bitField0_ & ~0x00000001);
onChanged();
} else {
serversBuilder_.clear();
}
return this;
}
/**
* repeated .raft.Server servers = 1;
*/
public Builder removeServers(int index) {
if (serversBuilder_ == null) {
ensureServersIsMutable();
servers_.remove(index);
onChanged();
} else {
serversBuilder_.remove(index);
}
return this;
}
/**
* repeated .raft.Server servers = 1;
*/
public com.github.wenweihu86.raft.proto.RaftMessage.Server.Builder getServersBuilder(
int index) {
return getServersFieldBuilder().getBuilder(index);
}
/**
* repeated .raft.Server servers = 1;
*/
public com.github.wenweihu86.raft.proto.RaftMessage.ServerOrBuilder getServersOrBuilder(
int index) {
if (serversBuilder_ == null) {
return servers_.get(index); } else {
return serversBuilder_.getMessageOrBuilder(index);
}
}
/**
* repeated .raft.Server servers = 1;
*/
public java.util.List extends com.github.wenweihu86.raft.proto.RaftMessage.ServerOrBuilder>
getServersOrBuilderList() {
if (serversBuilder_ != null) {
return serversBuilder_.getMessageOrBuilderList();
} else {
return java.util.Collections.unmodifiableList(servers_);
}
}
/**
* repeated .raft.Server servers = 1;
*/
public com.github.wenweihu86.raft.proto.RaftMessage.Server.Builder addServersBuilder() {
return getServersFieldBuilder().addBuilder(
com.github.wenweihu86.raft.proto.RaftMessage.Server.getDefaultInstance());
}
/**
* repeated .raft.Server servers = 1;
*/
public com.github.wenweihu86.raft.proto.RaftMessage.Server.Builder addServersBuilder(
int index) {
return getServersFieldBuilder().addBuilder(
index, com.github.wenweihu86.raft.proto.RaftMessage.Server.getDefaultInstance());
}
/**
* repeated .raft.Server servers = 1;
*/
public java.util.List
getServersBuilderList() {
return getServersFieldBuilder().getBuilderList();
}
private com.google.protobuf.RepeatedFieldBuilderV3<
com.github.wenweihu86.raft.proto.RaftMessage.Server, com.github.wenweihu86.raft.proto.RaftMessage.Server.Builder, com.github.wenweihu86.raft.proto.RaftMessage.ServerOrBuilder>
getServersFieldBuilder() {
if (serversBuilder_ == null) {
serversBuilder_ = new com.google.protobuf.RepeatedFieldBuilderV3<
com.github.wenweihu86.raft.proto.RaftMessage.Server, com.github.wenweihu86.raft.proto.RaftMessage.Server.Builder, com.github.wenweihu86.raft.proto.RaftMessage.ServerOrBuilder>(
servers_,
((bitField0_ & 0x00000001) == 0x00000001),
getParentForChildren(),
isClean());
servers_ = null;
}
return serversBuilder_;
}
public final Builder setUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return this;
}
public final Builder mergeUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return this;
}
// @@protoc_insertion_point(builder_scope:raft.AddPeersRequest)
}
// @@protoc_insertion_point(class_scope:raft.AddPeersRequest)
private static final com.github.wenweihu86.raft.proto.RaftMessage.AddPeersRequest DEFAULT_INSTANCE;
static {
DEFAULT_INSTANCE = new com.github.wenweihu86.raft.proto.RaftMessage.AddPeersRequest();
}
public static com.github.wenweihu86.raft.proto.RaftMessage.AddPeersRequest getDefaultInstance() {
return DEFAULT_INSTANCE;
}
private static final com.google.protobuf.Parser
PARSER = new com.google.protobuf.AbstractParser() {
public AddPeersRequest parsePartialFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return new AddPeersRequest(input, extensionRegistry);
}
};
public static com.google.protobuf.Parser parser() {
return PARSER;
}
@java.lang.Override
public com.google.protobuf.Parser getParserForType() {
return PARSER;
}
public com.github.wenweihu86.raft.proto.RaftMessage.AddPeersRequest getDefaultInstanceForType() {
return DEFAULT_INSTANCE;
}
}
public interface AddPeersResponseOrBuilder extends
// @@protoc_insertion_point(interface_extends:raft.AddPeersResponse)
com.google.protobuf.MessageOrBuilder {
/**
* optional .raft.ResCode res_code = 1;
*/
int getResCodeValue();
/**
* optional .raft.ResCode res_code = 1;
*/
com.github.wenweihu86.raft.proto.RaftMessage.ResCode getResCode();
/**
* optional string res_msg = 2;
*/
java.lang.String getResMsg();
/**
* optional string res_msg = 2;
*/
com.google.protobuf.ByteString
getResMsgBytes();
}
/**
* Protobuf type {@code raft.AddPeersResponse}
*/
public static final class AddPeersResponse extends
com.google.protobuf.GeneratedMessageV3 implements
// @@protoc_insertion_point(message_implements:raft.AddPeersResponse)
AddPeersResponseOrBuilder {
// Use AddPeersResponse.newBuilder() to construct.
private AddPeersResponse(com.google.protobuf.GeneratedMessageV3.Builder> builder) {
super(builder);
}
private AddPeersResponse() {
resCode_ = 0;
resMsg_ = "";
}
@java.lang.Override
public final com.google.protobuf.UnknownFieldSet
getUnknownFields() {
return com.google.protobuf.UnknownFieldSet.getDefaultInstance();
}
private AddPeersResponse(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
this();
int mutable_bitField0_ = 0;
try {
boolean done = false;
while (!done) {
int tag = input.readTag();
switch (tag) {
case 0:
done = true;
break;
default: {
if (!input.skipField(tag)) {
done = true;
}
break;
}
case 8: {
int rawValue = input.readEnum();
resCode_ = rawValue;
break;
}
case 18: {
java.lang.String s = input.readStringRequireUtf8();
resMsg_ = s;
break;
}
}
}
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.setUnfinishedMessage(this);
} catch (java.io.IOException e) {
throw new com.google.protobuf.InvalidProtocolBufferException(
e).setUnfinishedMessage(this);
} finally {
makeExtensionsImmutable();
}
}
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return com.github.wenweihu86.raft.proto.RaftMessage.internal_static_raft_AddPeersResponse_descriptor;
}
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return com.github.wenweihu86.raft.proto.RaftMessage.internal_static_raft_AddPeersResponse_fieldAccessorTable
.ensureFieldAccessorsInitialized(
com.github.wenweihu86.raft.proto.RaftMessage.AddPeersResponse.class, com.github.wenweihu86.raft.proto.RaftMessage.AddPeersResponse.Builder.class);
}
public static final int RES_CODE_FIELD_NUMBER = 1;
private int resCode_;
/**
* optional .raft.ResCode res_code = 1;
*/
public int getResCodeValue() {
return resCode_;
}
/**
* optional .raft.ResCode res_code = 1;
*/
public com.github.wenweihu86.raft.proto.RaftMessage.ResCode getResCode() {
com.github.wenweihu86.raft.proto.RaftMessage.ResCode result = com.github.wenweihu86.raft.proto.RaftMessage.ResCode.valueOf(resCode_);
return result == null ? com.github.wenweihu86.raft.proto.RaftMessage.ResCode.UNRECOGNIZED : result;
}
public static final int RES_MSG_FIELD_NUMBER = 2;
private volatile java.lang.Object resMsg_;
/**
* optional string res_msg = 2;
*/
public java.lang.String getResMsg() {
java.lang.Object ref = resMsg_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
resMsg_ = s;
return s;
}
}
/**
* optional string res_msg = 2;
*/
public com.google.protobuf.ByteString
getResMsgBytes() {
java.lang.Object ref = resMsg_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
resMsg_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
private byte memoizedIsInitialized = -1;
public final boolean isInitialized() {
byte isInitialized = memoizedIsInitialized;
if (isInitialized == 1) return true;
if (isInitialized == 0) return false;
memoizedIsInitialized = 1;
return true;
}
public void writeTo(com.google.protobuf.CodedOutputStream output)
throws java.io.IOException {
if (resCode_ != com.github.wenweihu86.raft.proto.RaftMessage.ResCode.RES_CODE_SUCCESS.getNumber()) {
output.writeEnum(1, resCode_);
}
if (!getResMsgBytes().isEmpty()) {
com.google.protobuf.GeneratedMessageV3.writeString(output, 2, resMsg_);
}
}
public int getSerializedSize() {
int size = memoizedSize;
if (size != -1) return size;
size = 0;
if (resCode_ != com.github.wenweihu86.raft.proto.RaftMessage.ResCode.RES_CODE_SUCCESS.getNumber()) {
size += com.google.protobuf.CodedOutputStream
.computeEnumSize(1, resCode_);
}
if (!getResMsgBytes().isEmpty()) {
size += com.google.protobuf.GeneratedMessageV3.computeStringSize(2, resMsg_);
}
memoizedSize = size;
return size;
}
private static final long serialVersionUID = 0L;
@java.lang.Override
public boolean equals(final java.lang.Object obj) {
if (obj == this) {
return true;
}
if (!(obj instanceof com.github.wenweihu86.raft.proto.RaftMessage.AddPeersResponse)) {
return super.equals(obj);
}
com.github.wenweihu86.raft.proto.RaftMessage.AddPeersResponse other = (com.github.wenweihu86.raft.proto.RaftMessage.AddPeersResponse) obj;
boolean result = true;
result = result && resCode_ == other.resCode_;
result = result && getResMsg()
.equals(other.getResMsg());
return result;
}
@java.lang.Override
public int hashCode() {
if (memoizedHashCode != 0) {
return memoizedHashCode;
}
int hash = 41;
hash = (19 * hash) + getDescriptorForType().hashCode();
hash = (37 * hash) + RES_CODE_FIELD_NUMBER;
hash = (53 * hash) + resCode_;
hash = (37 * hash) + RES_MSG_FIELD_NUMBER;
hash = (53 * hash) + getResMsg().hashCode();
hash = (29 * hash) + unknownFields.hashCode();
memoizedHashCode = hash;
return hash;
}
public static com.github.wenweihu86.raft.proto.RaftMessage.AddPeersResponse parseFrom(
com.google.protobuf.ByteString data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static com.github.wenweihu86.raft.proto.RaftMessage.AddPeersResponse parseFrom(
com.google.protobuf.ByteString data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static com.github.wenweihu86.raft.proto.RaftMessage.AddPeersResponse parseFrom(byte[] data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static com.github.wenweihu86.raft.proto.RaftMessage.AddPeersResponse parseFrom(
byte[] data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static com.github.wenweihu86.raft.proto.RaftMessage.AddPeersResponse parseFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static com.github.wenweihu86.raft.proto.RaftMessage.AddPeersResponse parseFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
public static com.github.wenweihu86.raft.proto.RaftMessage.AddPeersResponse parseDelimitedFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input);
}
public static com.github.wenweihu86.raft.proto.RaftMessage.AddPeersResponse parseDelimitedFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input, extensionRegistry);
}
public static com.github.wenweihu86.raft.proto.RaftMessage.AddPeersResponse parseFrom(
com.google.protobuf.CodedInputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static com.github.wenweihu86.raft.proto.RaftMessage.AddPeersResponse parseFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
public Builder newBuilderForType() { return newBuilder(); }
public static Builder newBuilder() {
return DEFAULT_INSTANCE.toBuilder();
}
public static Builder newBuilder(com.github.wenweihu86.raft.proto.RaftMessage.AddPeersResponse prototype) {
return DEFAULT_INSTANCE.toBuilder().mergeFrom(prototype);
}
public Builder toBuilder() {
return this == DEFAULT_INSTANCE
? new Builder() : new Builder().mergeFrom(this);
}
@java.lang.Override
protected Builder newBuilderForType(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
Builder builder = new Builder(parent);
return builder;
}
/**
* Protobuf type {@code raft.AddPeersResponse}
*/
public static final class Builder extends
com.google.protobuf.GeneratedMessageV3.Builder implements
// @@protoc_insertion_point(builder_implements:raft.AddPeersResponse)
com.github.wenweihu86.raft.proto.RaftMessage.AddPeersResponseOrBuilder {
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return com.github.wenweihu86.raft.proto.RaftMessage.internal_static_raft_AddPeersResponse_descriptor;
}
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return com.github.wenweihu86.raft.proto.RaftMessage.internal_static_raft_AddPeersResponse_fieldAccessorTable
.ensureFieldAccessorsInitialized(
com.github.wenweihu86.raft.proto.RaftMessage.AddPeersResponse.class, com.github.wenweihu86.raft.proto.RaftMessage.AddPeersResponse.Builder.class);
}
// Construct using com.github.wenweihu86.raft.proto.RaftMessage.AddPeersResponse.newBuilder()
private Builder() {
maybeForceBuilderInitialization();
}
private Builder(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
super(parent);
maybeForceBuilderInitialization();
}
private void maybeForceBuilderInitialization() {
if (com.google.protobuf.GeneratedMessageV3
.alwaysUseFieldBuilders) {
}
}
public Builder clear() {
super.clear();
resCode_ = 0;
resMsg_ = "";
return this;
}
public com.google.protobuf.Descriptors.Descriptor
getDescriptorForType() {
return com.github.wenweihu86.raft.proto.RaftMessage.internal_static_raft_AddPeersResponse_descriptor;
}
public com.github.wenweihu86.raft.proto.RaftMessage.AddPeersResponse getDefaultInstanceForType() {
return com.github.wenweihu86.raft.proto.RaftMessage.AddPeersResponse.getDefaultInstance();
}
public com.github.wenweihu86.raft.proto.RaftMessage.AddPeersResponse build() {
com.github.wenweihu86.raft.proto.RaftMessage.AddPeersResponse result = buildPartial();
if (!result.isInitialized()) {
throw newUninitializedMessageException(result);
}
return result;
}
public com.github.wenweihu86.raft.proto.RaftMessage.AddPeersResponse buildPartial() {
com.github.wenweihu86.raft.proto.RaftMessage.AddPeersResponse result = new com.github.wenweihu86.raft.proto.RaftMessage.AddPeersResponse(this);
result.resCode_ = resCode_;
result.resMsg_ = resMsg_;
onBuilt();
return result;
}
public Builder clone() {
return (Builder) super.clone();
}
public Builder setField(
com.google.protobuf.Descriptors.FieldDescriptor field,
Object value) {
return (Builder) super.setField(field, value);
}
public Builder clearField(
com.google.protobuf.Descriptors.FieldDescriptor field) {
return (Builder) super.clearField(field);
}
public Builder clearOneof(
com.google.protobuf.Descriptors.OneofDescriptor oneof) {
return (Builder) super.clearOneof(oneof);
}
public Builder setRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
int index, Object value) {
return (Builder) super.setRepeatedField(field, index, value);
}
public Builder addRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
Object value) {
return (Builder) super.addRepeatedField(field, value);
}
public Builder mergeFrom(com.google.protobuf.Message other) {
if (other instanceof com.github.wenweihu86.raft.proto.RaftMessage.AddPeersResponse) {
return mergeFrom((com.github.wenweihu86.raft.proto.RaftMessage.AddPeersResponse)other);
} else {
super.mergeFrom(other);
return this;
}
}
public Builder mergeFrom(com.github.wenweihu86.raft.proto.RaftMessage.AddPeersResponse other) {
if (other == com.github.wenweihu86.raft.proto.RaftMessage.AddPeersResponse.getDefaultInstance()) return this;
if (other.resCode_ != 0) {
setResCodeValue(other.getResCodeValue());
}
if (!other.getResMsg().isEmpty()) {
resMsg_ = other.resMsg_;
onChanged();
}
onChanged();
return this;
}
public final boolean isInitialized() {
return true;
}
public Builder mergeFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
com.github.wenweihu86.raft.proto.RaftMessage.AddPeersResponse parsedMessage = null;
try {
parsedMessage = PARSER.parsePartialFrom(input, extensionRegistry);
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
parsedMessage = (com.github.wenweihu86.raft.proto.RaftMessage.AddPeersResponse) e.getUnfinishedMessage();
throw e.unwrapIOException();
} finally {
if (parsedMessage != null) {
mergeFrom(parsedMessage);
}
}
return this;
}
private int resCode_ = 0;
/**
* optional .raft.ResCode res_code = 1;
*/
public int getResCodeValue() {
return resCode_;
}
/**
* optional .raft.ResCode res_code = 1;
*/
public Builder setResCodeValue(int value) {
resCode_ = value;
onChanged();
return this;
}
/**
* optional .raft.ResCode res_code = 1;
*/
public com.github.wenweihu86.raft.proto.RaftMessage.ResCode getResCode() {
com.github.wenweihu86.raft.proto.RaftMessage.ResCode result = com.github.wenweihu86.raft.proto.RaftMessage.ResCode.valueOf(resCode_);
return result == null ? com.github.wenweihu86.raft.proto.RaftMessage.ResCode.UNRECOGNIZED : result;
}
/**
* optional .raft.ResCode res_code = 1;
*/
public Builder setResCode(com.github.wenweihu86.raft.proto.RaftMessage.ResCode value) {
if (value == null) {
throw new NullPointerException();
}
resCode_ = value.getNumber();
onChanged();
return this;
}
/**
* optional .raft.ResCode res_code = 1;
*/
public Builder clearResCode() {
resCode_ = 0;
onChanged();
return this;
}
private java.lang.Object resMsg_ = "";
/**
* optional string res_msg = 2;
*/
public java.lang.String getResMsg() {
java.lang.Object ref = resMsg_;
if (!(ref instanceof java.lang.String)) {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
resMsg_ = s;
return s;
} else {
return (java.lang.String) ref;
}
}
/**
* optional string res_msg = 2;
*/
public com.google.protobuf.ByteString
getResMsgBytes() {
java.lang.Object ref = resMsg_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
resMsg_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
* optional string res_msg = 2;
*/
public Builder setResMsg(
java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
resMsg_ = value;
onChanged();
return this;
}
/**
* optional string res_msg = 2;
*/
public Builder clearResMsg() {
resMsg_ = getDefaultInstance().getResMsg();
onChanged();
return this;
}
/**
* optional string res_msg = 2;
*/
public Builder setResMsgBytes(
com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
checkByteStringIsUtf8(value);
resMsg_ = value;
onChanged();
return this;
}
public final Builder setUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return this;
}
public final Builder mergeUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return this;
}
// @@protoc_insertion_point(builder_scope:raft.AddPeersResponse)
}
// @@protoc_insertion_point(class_scope:raft.AddPeersResponse)
private static final com.github.wenweihu86.raft.proto.RaftMessage.AddPeersResponse DEFAULT_INSTANCE;
static {
DEFAULT_INSTANCE = new com.github.wenweihu86.raft.proto.RaftMessage.AddPeersResponse();
}
public static com.github.wenweihu86.raft.proto.RaftMessage.AddPeersResponse getDefaultInstance() {
return DEFAULT_INSTANCE;
}
private static final com.google.protobuf.Parser
PARSER = new com.google.protobuf.AbstractParser() {
public AddPeersResponse parsePartialFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return new AddPeersResponse(input, extensionRegistry);
}
};
public static com.google.protobuf.Parser parser() {
return PARSER;
}
@java.lang.Override
public com.google.protobuf.Parser getParserForType() {
return PARSER;
}
public com.github.wenweihu86.raft.proto.RaftMessage.AddPeersResponse getDefaultInstanceForType() {
return DEFAULT_INSTANCE;
}
}
public interface RemovePeersRequestOrBuilder extends
// @@protoc_insertion_point(interface_extends:raft.RemovePeersRequest)
com.google.protobuf.MessageOrBuilder {
/**
* repeated .raft.Server servers = 1;
*/
java.util.List
getServersList();
/**
* repeated .raft.Server servers = 1;
*/
com.github.wenweihu86.raft.proto.RaftMessage.Server getServers(int index);
/**
* repeated .raft.Server servers = 1;
*/
int getServersCount();
/**
* repeated .raft.Server servers = 1;
*/
java.util.List extends com.github.wenweihu86.raft.proto.RaftMessage.ServerOrBuilder>
getServersOrBuilderList();
/**
* repeated .raft.Server servers = 1;
*/
com.github.wenweihu86.raft.proto.RaftMessage.ServerOrBuilder getServersOrBuilder(
int index);
}
/**
* Protobuf type {@code raft.RemovePeersRequest}
*/
public static final class RemovePeersRequest extends
com.google.protobuf.GeneratedMessageV3 implements
// @@protoc_insertion_point(message_implements:raft.RemovePeersRequest)
RemovePeersRequestOrBuilder {
// Use RemovePeersRequest.newBuilder() to construct.
private RemovePeersRequest(com.google.protobuf.GeneratedMessageV3.Builder> builder) {
super(builder);
}
private RemovePeersRequest() {
servers_ = java.util.Collections.emptyList();
}
@java.lang.Override
public final com.google.protobuf.UnknownFieldSet
getUnknownFields() {
return com.google.protobuf.UnknownFieldSet.getDefaultInstance();
}
private RemovePeersRequest(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
this();
int mutable_bitField0_ = 0;
try {
boolean done = false;
while (!done) {
int tag = input.readTag();
switch (tag) {
case 0:
done = true;
break;
default: {
if (!input.skipField(tag)) {
done = true;
}
break;
}
case 10: {
if (!((mutable_bitField0_ & 0x00000001) == 0x00000001)) {
servers_ = new java.util.ArrayList();
mutable_bitField0_ |= 0x00000001;
}
servers_.add(
input.readMessage(com.github.wenweihu86.raft.proto.RaftMessage.Server.parser(), extensionRegistry));
break;
}
}
}
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.setUnfinishedMessage(this);
} catch (java.io.IOException e) {
throw new com.google.protobuf.InvalidProtocolBufferException(
e).setUnfinishedMessage(this);
} finally {
if (((mutable_bitField0_ & 0x00000001) == 0x00000001)) {
servers_ = java.util.Collections.unmodifiableList(servers_);
}
makeExtensionsImmutable();
}
}
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return com.github.wenweihu86.raft.proto.RaftMessage.internal_static_raft_RemovePeersRequest_descriptor;
}
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return com.github.wenweihu86.raft.proto.RaftMessage.internal_static_raft_RemovePeersRequest_fieldAccessorTable
.ensureFieldAccessorsInitialized(
com.github.wenweihu86.raft.proto.RaftMessage.RemovePeersRequest.class, com.github.wenweihu86.raft.proto.RaftMessage.RemovePeersRequest.Builder.class);
}
public static final int SERVERS_FIELD_NUMBER = 1;
private java.util.List servers_;
/**
* repeated .raft.Server servers = 1;
*/
public java.util.List getServersList() {
return servers_;
}
/**
* repeated .raft.Server servers = 1;
*/
public java.util.List extends com.github.wenweihu86.raft.proto.RaftMessage.ServerOrBuilder>
getServersOrBuilderList() {
return servers_;
}
/**
* repeated .raft.Server servers = 1;
*/
public int getServersCount() {
return servers_.size();
}
/**
* repeated .raft.Server servers = 1;
*/
public com.github.wenweihu86.raft.proto.RaftMessage.Server getServers(int index) {
return servers_.get(index);
}
/**
* repeated .raft.Server servers = 1;
*/
public com.github.wenweihu86.raft.proto.RaftMessage.ServerOrBuilder getServersOrBuilder(
int index) {
return servers_.get(index);
}
private byte memoizedIsInitialized = -1;
public final boolean isInitialized() {
byte isInitialized = memoizedIsInitialized;
if (isInitialized == 1) return true;
if (isInitialized == 0) return false;
memoizedIsInitialized = 1;
return true;
}
public void writeTo(com.google.protobuf.CodedOutputStream output)
throws java.io.IOException {
for (int i = 0; i < servers_.size(); i++) {
output.writeMessage(1, servers_.get(i));
}
}
public int getSerializedSize() {
int size = memoizedSize;
if (size != -1) return size;
size = 0;
for (int i = 0; i < servers_.size(); i++) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(1, servers_.get(i));
}
memoizedSize = size;
return size;
}
private static final long serialVersionUID = 0L;
@java.lang.Override
public boolean equals(final java.lang.Object obj) {
if (obj == this) {
return true;
}
if (!(obj instanceof com.github.wenweihu86.raft.proto.RaftMessage.RemovePeersRequest)) {
return super.equals(obj);
}
com.github.wenweihu86.raft.proto.RaftMessage.RemovePeersRequest other = (com.github.wenweihu86.raft.proto.RaftMessage.RemovePeersRequest) obj;
boolean result = true;
result = result && getServersList()
.equals(other.getServersList());
return result;
}
@java.lang.Override
public int hashCode() {
if (memoizedHashCode != 0) {
return memoizedHashCode;
}
int hash = 41;
hash = (19 * hash) + getDescriptorForType().hashCode();
if (getServersCount() > 0) {
hash = (37 * hash) + SERVERS_FIELD_NUMBER;
hash = (53 * hash) + getServersList().hashCode();
}
hash = (29 * hash) + unknownFields.hashCode();
memoizedHashCode = hash;
return hash;
}
public static com.github.wenweihu86.raft.proto.RaftMessage.RemovePeersRequest parseFrom(
com.google.protobuf.ByteString data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static com.github.wenweihu86.raft.proto.RaftMessage.RemovePeersRequest parseFrom(
com.google.protobuf.ByteString data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static com.github.wenweihu86.raft.proto.RaftMessage.RemovePeersRequest parseFrom(byte[] data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static com.github.wenweihu86.raft.proto.RaftMessage.RemovePeersRequest parseFrom(
byte[] data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static com.github.wenweihu86.raft.proto.RaftMessage.RemovePeersRequest parseFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static com.github.wenweihu86.raft.proto.RaftMessage.RemovePeersRequest parseFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
public static com.github.wenweihu86.raft.proto.RaftMessage.RemovePeersRequest parseDelimitedFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input);
}
public static com.github.wenweihu86.raft.proto.RaftMessage.RemovePeersRequest parseDelimitedFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input, extensionRegistry);
}
public static com.github.wenweihu86.raft.proto.RaftMessage.RemovePeersRequest parseFrom(
com.google.protobuf.CodedInputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static com.github.wenweihu86.raft.proto.RaftMessage.RemovePeersRequest parseFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
public Builder newBuilderForType() { return newBuilder(); }
public static Builder newBuilder() {
return DEFAULT_INSTANCE.toBuilder();
}
public static Builder newBuilder(com.github.wenweihu86.raft.proto.RaftMessage.RemovePeersRequest prototype) {
return DEFAULT_INSTANCE.toBuilder().mergeFrom(prototype);
}
public Builder toBuilder() {
return this == DEFAULT_INSTANCE
? new Builder() : new Builder().mergeFrom(this);
}
@java.lang.Override
protected Builder newBuilderForType(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
Builder builder = new Builder(parent);
return builder;
}
/**
* Protobuf type {@code raft.RemovePeersRequest}
*/
public static final class Builder extends
com.google.protobuf.GeneratedMessageV3.Builder implements
// @@protoc_insertion_point(builder_implements:raft.RemovePeersRequest)
com.github.wenweihu86.raft.proto.RaftMessage.RemovePeersRequestOrBuilder {
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return com.github.wenweihu86.raft.proto.RaftMessage.internal_static_raft_RemovePeersRequest_descriptor;
}
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return com.github.wenweihu86.raft.proto.RaftMessage.internal_static_raft_RemovePeersRequest_fieldAccessorTable
.ensureFieldAccessorsInitialized(
com.github.wenweihu86.raft.proto.RaftMessage.RemovePeersRequest.class, com.github.wenweihu86.raft.proto.RaftMessage.RemovePeersRequest.Builder.class);
}
// Construct using com.github.wenweihu86.raft.proto.RaftMessage.RemovePeersRequest.newBuilder()
private Builder() {
maybeForceBuilderInitialization();
}
private Builder(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
super(parent);
maybeForceBuilderInitialization();
}
private void maybeForceBuilderInitialization() {
if (com.google.protobuf.GeneratedMessageV3
.alwaysUseFieldBuilders) {
getServersFieldBuilder();
}
}
public Builder clear() {
super.clear();
if (serversBuilder_ == null) {
servers_ = java.util.Collections.emptyList();
bitField0_ = (bitField0_ & ~0x00000001);
} else {
serversBuilder_.clear();
}
return this;
}
public com.google.protobuf.Descriptors.Descriptor
getDescriptorForType() {
return com.github.wenweihu86.raft.proto.RaftMessage.internal_static_raft_RemovePeersRequest_descriptor;
}
public com.github.wenweihu86.raft.proto.RaftMessage.RemovePeersRequest getDefaultInstanceForType() {
return com.github.wenweihu86.raft.proto.RaftMessage.RemovePeersRequest.getDefaultInstance();
}
public com.github.wenweihu86.raft.proto.RaftMessage.RemovePeersRequest build() {
com.github.wenweihu86.raft.proto.RaftMessage.RemovePeersRequest result = buildPartial();
if (!result.isInitialized()) {
throw newUninitializedMessageException(result);
}
return result;
}
public com.github.wenweihu86.raft.proto.RaftMessage.RemovePeersRequest buildPartial() {
com.github.wenweihu86.raft.proto.RaftMessage.RemovePeersRequest result = new com.github.wenweihu86.raft.proto.RaftMessage.RemovePeersRequest(this);
int from_bitField0_ = bitField0_;
if (serversBuilder_ == null) {
if (((bitField0_ & 0x00000001) == 0x00000001)) {
servers_ = java.util.Collections.unmodifiableList(servers_);
bitField0_ = (bitField0_ & ~0x00000001);
}
result.servers_ = servers_;
} else {
result.servers_ = serversBuilder_.build();
}
onBuilt();
return result;
}
public Builder clone() {
return (Builder) super.clone();
}
public Builder setField(
com.google.protobuf.Descriptors.FieldDescriptor field,
Object value) {
return (Builder) super.setField(field, value);
}
public Builder clearField(
com.google.protobuf.Descriptors.FieldDescriptor field) {
return (Builder) super.clearField(field);
}
public Builder clearOneof(
com.google.protobuf.Descriptors.OneofDescriptor oneof) {
return (Builder) super.clearOneof(oneof);
}
public Builder setRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
int index, Object value) {
return (Builder) super.setRepeatedField(field, index, value);
}
public Builder addRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
Object value) {
return (Builder) super.addRepeatedField(field, value);
}
public Builder mergeFrom(com.google.protobuf.Message other) {
if (other instanceof com.github.wenweihu86.raft.proto.RaftMessage.RemovePeersRequest) {
return mergeFrom((com.github.wenweihu86.raft.proto.RaftMessage.RemovePeersRequest)other);
} else {
super.mergeFrom(other);
return this;
}
}
public Builder mergeFrom(com.github.wenweihu86.raft.proto.RaftMessage.RemovePeersRequest other) {
if (other == com.github.wenweihu86.raft.proto.RaftMessage.RemovePeersRequest.getDefaultInstance()) return this;
if (serversBuilder_ == null) {
if (!other.servers_.isEmpty()) {
if (servers_.isEmpty()) {
servers_ = other.servers_;
bitField0_ = (bitField0_ & ~0x00000001);
} else {
ensureServersIsMutable();
servers_.addAll(other.servers_);
}
onChanged();
}
} else {
if (!other.servers_.isEmpty()) {
if (serversBuilder_.isEmpty()) {
serversBuilder_.dispose();
serversBuilder_ = null;
servers_ = other.servers_;
bitField0_ = (bitField0_ & ~0x00000001);
serversBuilder_ =
com.google.protobuf.GeneratedMessageV3.alwaysUseFieldBuilders ?
getServersFieldBuilder() : null;
} else {
serversBuilder_.addAllMessages(other.servers_);
}
}
}
onChanged();
return this;
}
public final boolean isInitialized() {
return true;
}
public Builder mergeFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
com.github.wenweihu86.raft.proto.RaftMessage.RemovePeersRequest parsedMessage = null;
try {
parsedMessage = PARSER.parsePartialFrom(input, extensionRegistry);
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
parsedMessage = (com.github.wenweihu86.raft.proto.RaftMessage.RemovePeersRequest) e.getUnfinishedMessage();
throw e.unwrapIOException();
} finally {
if (parsedMessage != null) {
mergeFrom(parsedMessage);
}
}
return this;
}
private int bitField0_;
private java.util.List servers_ =
java.util.Collections.emptyList();
private void ensureServersIsMutable() {
if (!((bitField0_ & 0x00000001) == 0x00000001)) {
servers_ = new java.util.ArrayList(servers_);
bitField0_ |= 0x00000001;
}
}
private com.google.protobuf.RepeatedFieldBuilderV3<
com.github.wenweihu86.raft.proto.RaftMessage.Server, com.github.wenweihu86.raft.proto.RaftMessage.Server.Builder, com.github.wenweihu86.raft.proto.RaftMessage.ServerOrBuilder> serversBuilder_;
/**
* repeated .raft.Server servers = 1;
*/
public java.util.List getServersList() {
if (serversBuilder_ == null) {
return java.util.Collections.unmodifiableList(servers_);
} else {
return serversBuilder_.getMessageList();
}
}
/**
* repeated .raft.Server servers = 1;
*/
public int getServersCount() {
if (serversBuilder_ == null) {
return servers_.size();
} else {
return serversBuilder_.getCount();
}
}
/**
* repeated .raft.Server servers = 1;
*/
public com.github.wenweihu86.raft.proto.RaftMessage.Server getServers(int index) {
if (serversBuilder_ == null) {
return servers_.get(index);
} else {
return serversBuilder_.getMessage(index);
}
}
/**
* repeated .raft.Server servers = 1;
*/
public Builder setServers(
int index, com.github.wenweihu86.raft.proto.RaftMessage.Server value) {
if (serversBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
ensureServersIsMutable();
servers_.set(index, value);
onChanged();
} else {
serversBuilder_.setMessage(index, value);
}
return this;
}
/**
* repeated .raft.Server servers = 1;
*/
public Builder setServers(
int index, com.github.wenweihu86.raft.proto.RaftMessage.Server.Builder builderForValue) {
if (serversBuilder_ == null) {
ensureServersIsMutable();
servers_.set(index, builderForValue.build());
onChanged();
} else {
serversBuilder_.setMessage(index, builderForValue.build());
}
return this;
}
/**
* repeated .raft.Server servers = 1;
*/
public Builder addServers(com.github.wenweihu86.raft.proto.RaftMessage.Server value) {
if (serversBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
ensureServersIsMutable();
servers_.add(value);
onChanged();
} else {
serversBuilder_.addMessage(value);
}
return this;
}
/**
* repeated .raft.Server servers = 1;
*/
public Builder addServers(
int index, com.github.wenweihu86.raft.proto.RaftMessage.Server value) {
if (serversBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
ensureServersIsMutable();
servers_.add(index, value);
onChanged();
} else {
serversBuilder_.addMessage(index, value);
}
return this;
}
/**
* repeated .raft.Server servers = 1;
*/
public Builder addServers(
com.github.wenweihu86.raft.proto.RaftMessage.Server.Builder builderForValue) {
if (serversBuilder_ == null) {
ensureServersIsMutable();
servers_.add(builderForValue.build());
onChanged();
} else {
serversBuilder_.addMessage(builderForValue.build());
}
return this;
}
/**
* repeated .raft.Server servers = 1;
*/
public Builder addServers(
int index, com.github.wenweihu86.raft.proto.RaftMessage.Server.Builder builderForValue) {
if (serversBuilder_ == null) {
ensureServersIsMutable();
servers_.add(index, builderForValue.build());
onChanged();
} else {
serversBuilder_.addMessage(index, builderForValue.build());
}
return this;
}
/**
* repeated .raft.Server servers = 1;
*/
public Builder addAllServers(
java.lang.Iterable extends com.github.wenweihu86.raft.proto.RaftMessage.Server> values) {
if (serversBuilder_ == null) {
ensureServersIsMutable();
com.google.protobuf.AbstractMessageLite.Builder.addAll(
values, servers_);
onChanged();
} else {
serversBuilder_.addAllMessages(values);
}
return this;
}
/**
* repeated .raft.Server servers = 1;
*/
public Builder clearServers() {
if (serversBuilder_ == null) {
servers_ = java.util.Collections.emptyList();
bitField0_ = (bitField0_ & ~0x00000001);
onChanged();
} else {
serversBuilder_.clear();
}
return this;
}
/**
* repeated .raft.Server servers = 1;
*/
public Builder removeServers(int index) {
if (serversBuilder_ == null) {
ensureServersIsMutable();
servers_.remove(index);
onChanged();
} else {
serversBuilder_.remove(index);
}
return this;
}
/**
* repeated .raft.Server servers = 1;
*/
public com.github.wenweihu86.raft.proto.RaftMessage.Server.Builder getServersBuilder(
int index) {
return getServersFieldBuilder().getBuilder(index);
}
/**
* repeated .raft.Server servers = 1;
*/
public com.github.wenweihu86.raft.proto.RaftMessage.ServerOrBuilder getServersOrBuilder(
int index) {
if (serversBuilder_ == null) {
return servers_.get(index); } else {
return serversBuilder_.getMessageOrBuilder(index);
}
}
/**
* repeated .raft.Server servers = 1;
*/
public java.util.List extends com.github.wenweihu86.raft.proto.RaftMessage.ServerOrBuilder>
getServersOrBuilderList() {
if (serversBuilder_ != null) {
return serversBuilder_.getMessageOrBuilderList();
} else {
return java.util.Collections.unmodifiableList(servers_);
}
}
/**
* repeated .raft.Server servers = 1;
*/
public com.github.wenweihu86.raft.proto.RaftMessage.Server.Builder addServersBuilder() {
return getServersFieldBuilder().addBuilder(
com.github.wenweihu86.raft.proto.RaftMessage.Server.getDefaultInstance());
}
/**
* repeated .raft.Server servers = 1;
*/
public com.github.wenweihu86.raft.proto.RaftMessage.Server.Builder addServersBuilder(
int index) {
return getServersFieldBuilder().addBuilder(
index, com.github.wenweihu86.raft.proto.RaftMessage.Server.getDefaultInstance());
}
/**
* repeated .raft.Server servers = 1;
*/
public java.util.List
getServersBuilderList() {
return getServersFieldBuilder().getBuilderList();
}
private com.google.protobuf.RepeatedFieldBuilderV3<
com.github.wenweihu86.raft.proto.RaftMessage.Server, com.github.wenweihu86.raft.proto.RaftMessage.Server.Builder, com.github.wenweihu86.raft.proto.RaftMessage.ServerOrBuilder>
getServersFieldBuilder() {
if (serversBuilder_ == null) {
serversBuilder_ = new com.google.protobuf.RepeatedFieldBuilderV3<
com.github.wenweihu86.raft.proto.RaftMessage.Server, com.github.wenweihu86.raft.proto.RaftMessage.Server.Builder, com.github.wenweihu86.raft.proto.RaftMessage.ServerOrBuilder>(
servers_,
((bitField0_ & 0x00000001) == 0x00000001),
getParentForChildren(),
isClean());
servers_ = null;
}
return serversBuilder_;
}
public final Builder setUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return this;
}
public final Builder mergeUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return this;
}
// @@protoc_insertion_point(builder_scope:raft.RemovePeersRequest)
}
// @@protoc_insertion_point(class_scope:raft.RemovePeersRequest)
private static final com.github.wenweihu86.raft.proto.RaftMessage.RemovePeersRequest DEFAULT_INSTANCE;
static {
DEFAULT_INSTANCE = new com.github.wenweihu86.raft.proto.RaftMessage.RemovePeersRequest();
}
public static com.github.wenweihu86.raft.proto.RaftMessage.RemovePeersRequest getDefaultInstance() {
return DEFAULT_INSTANCE;
}
private static final com.google.protobuf.Parser
PARSER = new com.google.protobuf.AbstractParser() {
public RemovePeersRequest parsePartialFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return new RemovePeersRequest(input, extensionRegistry);
}
};
public static com.google.protobuf.Parser parser() {
return PARSER;
}
@java.lang.Override
public com.google.protobuf.Parser getParserForType() {
return PARSER;
}
public com.github.wenweihu86.raft.proto.RaftMessage.RemovePeersRequest getDefaultInstanceForType() {
return DEFAULT_INSTANCE;
}
}
public interface RemovePeersResponseOrBuilder extends
// @@protoc_insertion_point(interface_extends:raft.RemovePeersResponse)
com.google.protobuf.MessageOrBuilder {
/**
* optional .raft.ResCode res_code = 1;
*/
int getResCodeValue();
/**
* optional .raft.ResCode res_code = 1;
*/
com.github.wenweihu86.raft.proto.RaftMessage.ResCode getResCode();
/**
* optional string res_msg = 2;
*/
java.lang.String getResMsg();
/**
* optional string res_msg = 2;
*/
com.google.protobuf.ByteString
getResMsgBytes();
}
/**
* Protobuf type {@code raft.RemovePeersResponse}
*/
public static final class RemovePeersResponse extends
com.google.protobuf.GeneratedMessageV3 implements
// @@protoc_insertion_point(message_implements:raft.RemovePeersResponse)
RemovePeersResponseOrBuilder {
// Use RemovePeersResponse.newBuilder() to construct.
private RemovePeersResponse(com.google.protobuf.GeneratedMessageV3.Builder> builder) {
super(builder);
}
private RemovePeersResponse() {
resCode_ = 0;
resMsg_ = "";
}
@java.lang.Override
public final com.google.protobuf.UnknownFieldSet
getUnknownFields() {
return com.google.protobuf.UnknownFieldSet.getDefaultInstance();
}
private RemovePeersResponse(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
this();
int mutable_bitField0_ = 0;
try {
boolean done = false;
while (!done) {
int tag = input.readTag();
switch (tag) {
case 0:
done = true;
break;
default: {
if (!input.skipField(tag)) {
done = true;
}
break;
}
case 8: {
int rawValue = input.readEnum();
resCode_ = rawValue;
break;
}
case 18: {
java.lang.String s = input.readStringRequireUtf8();
resMsg_ = s;
break;
}
}
}
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.setUnfinishedMessage(this);
} catch (java.io.IOException e) {
throw new com.google.protobuf.InvalidProtocolBufferException(
e).setUnfinishedMessage(this);
} finally {
makeExtensionsImmutable();
}
}
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return com.github.wenweihu86.raft.proto.RaftMessage.internal_static_raft_RemovePeersResponse_descriptor;
}
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return com.github.wenweihu86.raft.proto.RaftMessage.internal_static_raft_RemovePeersResponse_fieldAccessorTable
.ensureFieldAccessorsInitialized(
com.github.wenweihu86.raft.proto.RaftMessage.RemovePeersResponse.class, com.github.wenweihu86.raft.proto.RaftMessage.RemovePeersResponse.Builder.class);
}
public static final int RES_CODE_FIELD_NUMBER = 1;
private int resCode_;
/**
* optional .raft.ResCode res_code = 1;
*/
public int getResCodeValue() {
return resCode_;
}
/**
* optional .raft.ResCode res_code = 1;
*/
public com.github.wenweihu86.raft.proto.RaftMessage.ResCode getResCode() {
com.github.wenweihu86.raft.proto.RaftMessage.ResCode result = com.github.wenweihu86.raft.proto.RaftMessage.ResCode.valueOf(resCode_);
return result == null ? com.github.wenweihu86.raft.proto.RaftMessage.ResCode.UNRECOGNIZED : result;
}
public static final int RES_MSG_FIELD_NUMBER = 2;
private volatile java.lang.Object resMsg_;
/**
* optional string res_msg = 2;
*/
public java.lang.String getResMsg() {
java.lang.Object ref = resMsg_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
resMsg_ = s;
return s;
}
}
/**
* optional string res_msg = 2;
*/
public com.google.protobuf.ByteString
getResMsgBytes() {
java.lang.Object ref = resMsg_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
resMsg_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
private byte memoizedIsInitialized = -1;
public final boolean isInitialized() {
byte isInitialized = memoizedIsInitialized;
if (isInitialized == 1) return true;
if (isInitialized == 0) return false;
memoizedIsInitialized = 1;
return true;
}
public void writeTo(com.google.protobuf.CodedOutputStream output)
throws java.io.IOException {
if (resCode_ != com.github.wenweihu86.raft.proto.RaftMessage.ResCode.RES_CODE_SUCCESS.getNumber()) {
output.writeEnum(1, resCode_);
}
if (!getResMsgBytes().isEmpty()) {
com.google.protobuf.GeneratedMessageV3.writeString(output, 2, resMsg_);
}
}
public int getSerializedSize() {
int size = memoizedSize;
if (size != -1) return size;
size = 0;
if (resCode_ != com.github.wenweihu86.raft.proto.RaftMessage.ResCode.RES_CODE_SUCCESS.getNumber()) {
size += com.google.protobuf.CodedOutputStream
.computeEnumSize(1, resCode_);
}
if (!getResMsgBytes().isEmpty()) {
size += com.google.protobuf.GeneratedMessageV3.computeStringSize(2, resMsg_);
}
memoizedSize = size;
return size;
}
private static final long serialVersionUID = 0L;
@java.lang.Override
public boolean equals(final java.lang.Object obj) {
if (obj == this) {
return true;
}
if (!(obj instanceof com.github.wenweihu86.raft.proto.RaftMessage.RemovePeersResponse)) {
return super.equals(obj);
}
com.github.wenweihu86.raft.proto.RaftMessage.RemovePeersResponse other = (com.github.wenweihu86.raft.proto.RaftMessage.RemovePeersResponse) obj;
boolean result = true;
result = result && resCode_ == other.resCode_;
result = result && getResMsg()
.equals(other.getResMsg());
return result;
}
@java.lang.Override
public int hashCode() {
if (memoizedHashCode != 0) {
return memoizedHashCode;
}
int hash = 41;
hash = (19 * hash) + getDescriptorForType().hashCode();
hash = (37 * hash) + RES_CODE_FIELD_NUMBER;
hash = (53 * hash) + resCode_;
hash = (37 * hash) + RES_MSG_FIELD_NUMBER;
hash = (53 * hash) + getResMsg().hashCode();
hash = (29 * hash) + unknownFields.hashCode();
memoizedHashCode = hash;
return hash;
}
public static com.github.wenweihu86.raft.proto.RaftMessage.RemovePeersResponse parseFrom(
com.google.protobuf.ByteString data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static com.github.wenweihu86.raft.proto.RaftMessage.RemovePeersResponse parseFrom(
com.google.protobuf.ByteString data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static com.github.wenweihu86.raft.proto.RaftMessage.RemovePeersResponse parseFrom(byte[] data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static com.github.wenweihu86.raft.proto.RaftMessage.RemovePeersResponse parseFrom(
byte[] data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static com.github.wenweihu86.raft.proto.RaftMessage.RemovePeersResponse parseFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static com.github.wenweihu86.raft.proto.RaftMessage.RemovePeersResponse parseFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
public static com.github.wenweihu86.raft.proto.RaftMessage.RemovePeersResponse parseDelimitedFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input);
}
public static com.github.wenweihu86.raft.proto.RaftMessage.RemovePeersResponse parseDelimitedFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input, extensionRegistry);
}
public static com.github.wenweihu86.raft.proto.RaftMessage.RemovePeersResponse parseFrom(
com.google.protobuf.CodedInputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static com.github.wenweihu86.raft.proto.RaftMessage.RemovePeersResponse parseFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
public Builder newBuilderForType() { return newBuilder(); }
public static Builder newBuilder() {
return DEFAULT_INSTANCE.toBuilder();
}
public static Builder newBuilder(com.github.wenweihu86.raft.proto.RaftMessage.RemovePeersResponse prototype) {
return DEFAULT_INSTANCE.toBuilder().mergeFrom(prototype);
}
public Builder toBuilder() {
return this == DEFAULT_INSTANCE
? new Builder() : new Builder().mergeFrom(this);
}
@java.lang.Override
protected Builder newBuilderForType(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
Builder builder = new Builder(parent);
return builder;
}
/**
* Protobuf type {@code raft.RemovePeersResponse}
*/
public static final class Builder extends
com.google.protobuf.GeneratedMessageV3.Builder implements
// @@protoc_insertion_point(builder_implements:raft.RemovePeersResponse)
com.github.wenweihu86.raft.proto.RaftMessage.RemovePeersResponseOrBuilder {
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return com.github.wenweihu86.raft.proto.RaftMessage.internal_static_raft_RemovePeersResponse_descriptor;
}
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return com.github.wenweihu86.raft.proto.RaftMessage.internal_static_raft_RemovePeersResponse_fieldAccessorTable
.ensureFieldAccessorsInitialized(
com.github.wenweihu86.raft.proto.RaftMessage.RemovePeersResponse.class, com.github.wenweihu86.raft.proto.RaftMessage.RemovePeersResponse.Builder.class);
}
// Construct using com.github.wenweihu86.raft.proto.RaftMessage.RemovePeersResponse.newBuilder()
private Builder() {
maybeForceBuilderInitialization();
}
private Builder(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
super(parent);
maybeForceBuilderInitialization();
}
private void maybeForceBuilderInitialization() {
if (com.google.protobuf.GeneratedMessageV3
.alwaysUseFieldBuilders) {
}
}
public Builder clear() {
super.clear();
resCode_ = 0;
resMsg_ = "";
return this;
}
public com.google.protobuf.Descriptors.Descriptor
getDescriptorForType() {
return com.github.wenweihu86.raft.proto.RaftMessage.internal_static_raft_RemovePeersResponse_descriptor;
}
public com.github.wenweihu86.raft.proto.RaftMessage.RemovePeersResponse getDefaultInstanceForType() {
return com.github.wenweihu86.raft.proto.RaftMessage.RemovePeersResponse.getDefaultInstance();
}
public com.github.wenweihu86.raft.proto.RaftMessage.RemovePeersResponse build() {
com.github.wenweihu86.raft.proto.RaftMessage.RemovePeersResponse result = buildPartial();
if (!result.isInitialized()) {
throw newUninitializedMessageException(result);
}
return result;
}
public com.github.wenweihu86.raft.proto.RaftMessage.RemovePeersResponse buildPartial() {
com.github.wenweihu86.raft.proto.RaftMessage.RemovePeersResponse result = new com.github.wenweihu86.raft.proto.RaftMessage.RemovePeersResponse(this);
result.resCode_ = resCode_;
result.resMsg_ = resMsg_;
onBuilt();
return result;
}
public Builder clone() {
return (Builder) super.clone();
}
public Builder setField(
com.google.protobuf.Descriptors.FieldDescriptor field,
Object value) {
return (Builder) super.setField(field, value);
}
public Builder clearField(
com.google.protobuf.Descriptors.FieldDescriptor field) {
return (Builder) super.clearField(field);
}
public Builder clearOneof(
com.google.protobuf.Descriptors.OneofDescriptor oneof) {
return (Builder) super.clearOneof(oneof);
}
public Builder setRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
int index, Object value) {
return (Builder) super.setRepeatedField(field, index, value);
}
public Builder addRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
Object value) {
return (Builder) super.addRepeatedField(field, value);
}
public Builder mergeFrom(com.google.protobuf.Message other) {
if (other instanceof com.github.wenweihu86.raft.proto.RaftMessage.RemovePeersResponse) {
return mergeFrom((com.github.wenweihu86.raft.proto.RaftMessage.RemovePeersResponse)other);
} else {
super.mergeFrom(other);
return this;
}
}
public Builder mergeFrom(com.github.wenweihu86.raft.proto.RaftMessage.RemovePeersResponse other) {
if (other == com.github.wenweihu86.raft.proto.RaftMessage.RemovePeersResponse.getDefaultInstance()) return this;
if (other.resCode_ != 0) {
setResCodeValue(other.getResCodeValue());
}
if (!other.getResMsg().isEmpty()) {
resMsg_ = other.resMsg_;
onChanged();
}
onChanged();
return this;
}
public final boolean isInitialized() {
return true;
}
public Builder mergeFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
com.github.wenweihu86.raft.proto.RaftMessage.RemovePeersResponse parsedMessage = null;
try {
parsedMessage = PARSER.parsePartialFrom(input, extensionRegistry);
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
parsedMessage = (com.github.wenweihu86.raft.proto.RaftMessage.RemovePeersResponse) e.getUnfinishedMessage();
throw e.unwrapIOException();
} finally {
if (parsedMessage != null) {
mergeFrom(parsedMessage);
}
}
return this;
}
private int resCode_ = 0;
/**
* optional .raft.ResCode res_code = 1;
*/
public int getResCodeValue() {
return resCode_;
}
/**
* optional .raft.ResCode res_code = 1;
*/
public Builder setResCodeValue(int value) {
resCode_ = value;
onChanged();
return this;
}
/**
* optional .raft.ResCode res_code = 1;
*/
public com.github.wenweihu86.raft.proto.RaftMessage.ResCode getResCode() {
com.github.wenweihu86.raft.proto.RaftMessage.ResCode result = com.github.wenweihu86.raft.proto.RaftMessage.ResCode.valueOf(resCode_);
return result == null ? com.github.wenweihu86.raft.proto.RaftMessage.ResCode.UNRECOGNIZED : result;
}
/**
* optional .raft.ResCode res_code = 1;
*/
public Builder setResCode(com.github.wenweihu86.raft.proto.RaftMessage.ResCode value) {
if (value == null) {
throw new NullPointerException();
}
resCode_ = value.getNumber();
onChanged();
return this;
}
/**
* optional .raft.ResCode res_code = 1;
*/
public Builder clearResCode() {
resCode_ = 0;
onChanged();
return this;
}
private java.lang.Object resMsg_ = "";
/**
* optional string res_msg = 2;
*/
public java.lang.String getResMsg() {
java.lang.Object ref = resMsg_;
if (!(ref instanceof java.lang.String)) {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
resMsg_ = s;
return s;
} else {
return (java.lang.String) ref;
}
}
/**
* optional string res_msg = 2;
*/
public com.google.protobuf.ByteString
getResMsgBytes() {
java.lang.Object ref = resMsg_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
resMsg_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
* optional string res_msg = 2;
*/
public Builder setResMsg(
java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
resMsg_ = value;
onChanged();
return this;
}
/**
* optional string res_msg = 2;
*/
public Builder clearResMsg() {
resMsg_ = getDefaultInstance().getResMsg();
onChanged();
return this;
}
/**
* optional string res_msg = 2;
*/
public Builder setResMsgBytes(
com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
checkByteStringIsUtf8(value);
resMsg_ = value;
onChanged();
return this;
}
public final Builder setUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return this;
}
public final Builder mergeUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return this;
}
// @@protoc_insertion_point(builder_scope:raft.RemovePeersResponse)
}
// @@protoc_insertion_point(class_scope:raft.RemovePeersResponse)
private static final com.github.wenweihu86.raft.proto.RaftMessage.RemovePeersResponse DEFAULT_INSTANCE;
static {
DEFAULT_INSTANCE = new com.github.wenweihu86.raft.proto.RaftMessage.RemovePeersResponse();
}
public static com.github.wenweihu86.raft.proto.RaftMessage.RemovePeersResponse getDefaultInstance() {
return DEFAULT_INSTANCE;
}
private static final com.google.protobuf.Parser
PARSER = new com.google.protobuf.AbstractParser() {
public RemovePeersResponse parsePartialFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return new RemovePeersResponse(input, extensionRegistry);
}
};
public static com.google.protobuf.Parser parser() {
return PARSER;
}
@java.lang.Override
public com.google.protobuf.Parser getParserForType() {
return PARSER;
}
public com.github.wenweihu86.raft.proto.RaftMessage.RemovePeersResponse getDefaultInstanceForType() {
return DEFAULT_INSTANCE;
}
}
public interface GetConfigurationRequestOrBuilder extends
// @@protoc_insertion_point(interface_extends:raft.GetConfigurationRequest)
com.google.protobuf.MessageOrBuilder {
}
/**
* Protobuf type {@code raft.GetConfigurationRequest}
*/
public static final class GetConfigurationRequest extends
com.google.protobuf.GeneratedMessageV3 implements
// @@protoc_insertion_point(message_implements:raft.GetConfigurationRequest)
GetConfigurationRequestOrBuilder {
// Use GetConfigurationRequest.newBuilder() to construct.
private GetConfigurationRequest(com.google.protobuf.GeneratedMessageV3.Builder> builder) {
super(builder);
}
private GetConfigurationRequest() {
}
@java.lang.Override
public final com.google.protobuf.UnknownFieldSet
getUnknownFields() {
return com.google.protobuf.UnknownFieldSet.getDefaultInstance();
}
private GetConfigurationRequest(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
this();
try {
boolean done = false;
while (!done) {
int tag = input.readTag();
switch (tag) {
case 0:
done = true;
break;
default: {
if (!input.skipField(tag)) {
done = true;
}
break;
}
}
}
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.setUnfinishedMessage(this);
} catch (java.io.IOException e) {
throw new com.google.protobuf.InvalidProtocolBufferException(
e).setUnfinishedMessage(this);
} finally {
makeExtensionsImmutable();
}
}
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return com.github.wenweihu86.raft.proto.RaftMessage.internal_static_raft_GetConfigurationRequest_descriptor;
}
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return com.github.wenweihu86.raft.proto.RaftMessage.internal_static_raft_GetConfigurationRequest_fieldAccessorTable
.ensureFieldAccessorsInitialized(
com.github.wenweihu86.raft.proto.RaftMessage.GetConfigurationRequest.class, com.github.wenweihu86.raft.proto.RaftMessage.GetConfigurationRequest.Builder.class);
}
private byte memoizedIsInitialized = -1;
public final boolean isInitialized() {
byte isInitialized = memoizedIsInitialized;
if (isInitialized == 1) return true;
if (isInitialized == 0) return false;
memoizedIsInitialized = 1;
return true;
}
public void writeTo(com.google.protobuf.CodedOutputStream output)
throws java.io.IOException {
}
public int getSerializedSize() {
int size = memoizedSize;
if (size != -1) return size;
size = 0;
memoizedSize = size;
return size;
}
private static final long serialVersionUID = 0L;
@java.lang.Override
public boolean equals(final java.lang.Object obj) {
if (obj == this) {
return true;
}
if (!(obj instanceof com.github.wenweihu86.raft.proto.RaftMessage.GetConfigurationRequest)) {
return super.equals(obj);
}
com.github.wenweihu86.raft.proto.RaftMessage.GetConfigurationRequest other = (com.github.wenweihu86.raft.proto.RaftMessage.GetConfigurationRequest) obj;
boolean result = true;
return result;
}
@java.lang.Override
public int hashCode() {
if (memoizedHashCode != 0) {
return memoizedHashCode;
}
int hash = 41;
hash = (19 * hash) + getDescriptorForType().hashCode();
hash = (29 * hash) + unknownFields.hashCode();
memoizedHashCode = hash;
return hash;
}
public static com.github.wenweihu86.raft.proto.RaftMessage.GetConfigurationRequest parseFrom(
com.google.protobuf.ByteString data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static com.github.wenweihu86.raft.proto.RaftMessage.GetConfigurationRequest parseFrom(
com.google.protobuf.ByteString data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static com.github.wenweihu86.raft.proto.RaftMessage.GetConfigurationRequest parseFrom(byte[] data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static com.github.wenweihu86.raft.proto.RaftMessage.GetConfigurationRequest parseFrom(
byte[] data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static com.github.wenweihu86.raft.proto.RaftMessage.GetConfigurationRequest parseFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static com.github.wenweihu86.raft.proto.RaftMessage.GetConfigurationRequest parseFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
public static com.github.wenweihu86.raft.proto.RaftMessage.GetConfigurationRequest parseDelimitedFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input);
}
public static com.github.wenweihu86.raft.proto.RaftMessage.GetConfigurationRequest parseDelimitedFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input, extensionRegistry);
}
public static com.github.wenweihu86.raft.proto.RaftMessage.GetConfigurationRequest parseFrom(
com.google.protobuf.CodedInputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static com.github.wenweihu86.raft.proto.RaftMessage.GetConfigurationRequest parseFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
public Builder newBuilderForType() { return newBuilder(); }
public static Builder newBuilder() {
return DEFAULT_INSTANCE.toBuilder();
}
public static Builder newBuilder(com.github.wenweihu86.raft.proto.RaftMessage.GetConfigurationRequest prototype) {
return DEFAULT_INSTANCE.toBuilder().mergeFrom(prototype);
}
public Builder toBuilder() {
return this == DEFAULT_INSTANCE
? new Builder() : new Builder().mergeFrom(this);
}
@java.lang.Override
protected Builder newBuilderForType(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
Builder builder = new Builder(parent);
return builder;
}
/**
* Protobuf type {@code raft.GetConfigurationRequest}
*/
public static final class Builder extends
com.google.protobuf.GeneratedMessageV3.Builder implements
// @@protoc_insertion_point(builder_implements:raft.GetConfigurationRequest)
com.github.wenweihu86.raft.proto.RaftMessage.GetConfigurationRequestOrBuilder {
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return com.github.wenweihu86.raft.proto.RaftMessage.internal_static_raft_GetConfigurationRequest_descriptor;
}
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return com.github.wenweihu86.raft.proto.RaftMessage.internal_static_raft_GetConfigurationRequest_fieldAccessorTable
.ensureFieldAccessorsInitialized(
com.github.wenweihu86.raft.proto.RaftMessage.GetConfigurationRequest.class, com.github.wenweihu86.raft.proto.RaftMessage.GetConfigurationRequest.Builder.class);
}
// Construct using com.github.wenweihu86.raft.proto.RaftMessage.GetConfigurationRequest.newBuilder()
private Builder() {
maybeForceBuilderInitialization();
}
private Builder(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
super(parent);
maybeForceBuilderInitialization();
}
private void maybeForceBuilderInitialization() {
if (com.google.protobuf.GeneratedMessageV3
.alwaysUseFieldBuilders) {
}
}
public Builder clear() {
super.clear();
return this;
}
public com.google.protobuf.Descriptors.Descriptor
getDescriptorForType() {
return com.github.wenweihu86.raft.proto.RaftMessage.internal_static_raft_GetConfigurationRequest_descriptor;
}
public com.github.wenweihu86.raft.proto.RaftMessage.GetConfigurationRequest getDefaultInstanceForType() {
return com.github.wenweihu86.raft.proto.RaftMessage.GetConfigurationRequest.getDefaultInstance();
}
public com.github.wenweihu86.raft.proto.RaftMessage.GetConfigurationRequest build() {
com.github.wenweihu86.raft.proto.RaftMessage.GetConfigurationRequest result = buildPartial();
if (!result.isInitialized()) {
throw newUninitializedMessageException(result);
}
return result;
}
public com.github.wenweihu86.raft.proto.RaftMessage.GetConfigurationRequest buildPartial() {
com.github.wenweihu86.raft.proto.RaftMessage.GetConfigurationRequest result = new com.github.wenweihu86.raft.proto.RaftMessage.GetConfigurationRequest(this);
onBuilt();
return result;
}
public Builder clone() {
return (Builder) super.clone();
}
public Builder setField(
com.google.protobuf.Descriptors.FieldDescriptor field,
Object value) {
return (Builder) super.setField(field, value);
}
public Builder clearField(
com.google.protobuf.Descriptors.FieldDescriptor field) {
return (Builder) super.clearField(field);
}
public Builder clearOneof(
com.google.protobuf.Descriptors.OneofDescriptor oneof) {
return (Builder) super.clearOneof(oneof);
}
public Builder setRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
int index, Object value) {
return (Builder) super.setRepeatedField(field, index, value);
}
public Builder addRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
Object value) {
return (Builder) super.addRepeatedField(field, value);
}
public Builder mergeFrom(com.google.protobuf.Message other) {
if (other instanceof com.github.wenweihu86.raft.proto.RaftMessage.GetConfigurationRequest) {
return mergeFrom((com.github.wenweihu86.raft.proto.RaftMessage.GetConfigurationRequest)other);
} else {
super.mergeFrom(other);
return this;
}
}
public Builder mergeFrom(com.github.wenweihu86.raft.proto.RaftMessage.GetConfigurationRequest other) {
if (other == com.github.wenweihu86.raft.proto.RaftMessage.GetConfigurationRequest.getDefaultInstance()) return this;
onChanged();
return this;
}
public final boolean isInitialized() {
return true;
}
public Builder mergeFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
com.github.wenweihu86.raft.proto.RaftMessage.GetConfigurationRequest parsedMessage = null;
try {
parsedMessage = PARSER.parsePartialFrom(input, extensionRegistry);
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
parsedMessage = (com.github.wenweihu86.raft.proto.RaftMessage.GetConfigurationRequest) e.getUnfinishedMessage();
throw e.unwrapIOException();
} finally {
if (parsedMessage != null) {
mergeFrom(parsedMessage);
}
}
return this;
}
public final Builder setUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return this;
}
public final Builder mergeUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return this;
}
// @@protoc_insertion_point(builder_scope:raft.GetConfigurationRequest)
}
// @@protoc_insertion_point(class_scope:raft.GetConfigurationRequest)
private static final com.github.wenweihu86.raft.proto.RaftMessage.GetConfigurationRequest DEFAULT_INSTANCE;
static {
DEFAULT_INSTANCE = new com.github.wenweihu86.raft.proto.RaftMessage.GetConfigurationRequest();
}
public static com.github.wenweihu86.raft.proto.RaftMessage.GetConfigurationRequest getDefaultInstance() {
return DEFAULT_INSTANCE;
}
private static final com.google.protobuf.Parser
PARSER = new com.google.protobuf.AbstractParser() {
public GetConfigurationRequest parsePartialFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return new GetConfigurationRequest(input, extensionRegistry);
}
};
public static com.google.protobuf.Parser parser() {
return PARSER;
}
@java.lang.Override
public com.google.protobuf.Parser getParserForType() {
return PARSER;
}
public com.github.wenweihu86.raft.proto.RaftMessage.GetConfigurationRequest getDefaultInstanceForType() {
return DEFAULT_INSTANCE;
}
}
public interface GetConfigurationResponseOrBuilder extends
// @@protoc_insertion_point(interface_extends:raft.GetConfigurationResponse)
com.google.protobuf.MessageOrBuilder {
/**
* optional .raft.ResCode res_code = 1;
*/
int getResCodeValue();
/**
* optional .raft.ResCode res_code = 1;
*/
com.github.wenweihu86.raft.proto.RaftMessage.ResCode getResCode();
/**
* optional string res_msg = 2;
*/
java.lang.String getResMsg();
/**
* optional string res_msg = 2;
*/
com.google.protobuf.ByteString
getResMsgBytes();
/**
* repeated .raft.Server servers = 3;
*/
java.util.List
getServersList();
/**
* repeated .raft.Server servers = 3;
*/
com.github.wenweihu86.raft.proto.RaftMessage.Server getServers(int index);
/**
* repeated .raft.Server servers = 3;
*/
int getServersCount();
/**
* repeated .raft.Server servers = 3;
*/
java.util.List extends com.github.wenweihu86.raft.proto.RaftMessage.ServerOrBuilder>
getServersOrBuilderList();
/**
* repeated .raft.Server servers = 3;
*/
com.github.wenweihu86.raft.proto.RaftMessage.ServerOrBuilder getServersOrBuilder(
int index);
/**
* optional .raft.Server leader = 4;
*/
boolean hasLeader();
/**
* optional .raft.Server leader = 4;
*/
com.github.wenweihu86.raft.proto.RaftMessage.Server getLeader();
/**
* optional .raft.Server leader = 4;
*/
com.github.wenweihu86.raft.proto.RaftMessage.ServerOrBuilder getLeaderOrBuilder();
}
/**
* Protobuf type {@code raft.GetConfigurationResponse}
*/
public static final class GetConfigurationResponse extends
com.google.protobuf.GeneratedMessageV3 implements
// @@protoc_insertion_point(message_implements:raft.GetConfigurationResponse)
GetConfigurationResponseOrBuilder {
// Use GetConfigurationResponse.newBuilder() to construct.
private GetConfigurationResponse(com.google.protobuf.GeneratedMessageV3.Builder> builder) {
super(builder);
}
private GetConfigurationResponse() {
resCode_ = 0;
resMsg_ = "";
servers_ = java.util.Collections.emptyList();
}
@java.lang.Override
public final com.google.protobuf.UnknownFieldSet
getUnknownFields() {
return com.google.protobuf.UnknownFieldSet.getDefaultInstance();
}
private GetConfigurationResponse(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
this();
int mutable_bitField0_ = 0;
try {
boolean done = false;
while (!done) {
int tag = input.readTag();
switch (tag) {
case 0:
done = true;
break;
default: {
if (!input.skipField(tag)) {
done = true;
}
break;
}
case 8: {
int rawValue = input.readEnum();
resCode_ = rawValue;
break;
}
case 18: {
java.lang.String s = input.readStringRequireUtf8();
resMsg_ = s;
break;
}
case 26: {
if (!((mutable_bitField0_ & 0x00000004) == 0x00000004)) {
servers_ = new java.util.ArrayList();
mutable_bitField0_ |= 0x00000004;
}
servers_.add(
input.readMessage(com.github.wenweihu86.raft.proto.RaftMessage.Server.parser(), extensionRegistry));
break;
}
case 34: {
com.github.wenweihu86.raft.proto.RaftMessage.Server.Builder subBuilder = null;
if (leader_ != null) {
subBuilder = leader_.toBuilder();
}
leader_ = input.readMessage(com.github.wenweihu86.raft.proto.RaftMessage.Server.parser(), extensionRegistry);
if (subBuilder != null) {
subBuilder.mergeFrom(leader_);
leader_ = subBuilder.buildPartial();
}
break;
}
}
}
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.setUnfinishedMessage(this);
} catch (java.io.IOException e) {
throw new com.google.protobuf.InvalidProtocolBufferException(
e).setUnfinishedMessage(this);
} finally {
if (((mutable_bitField0_ & 0x00000004) == 0x00000004)) {
servers_ = java.util.Collections.unmodifiableList(servers_);
}
makeExtensionsImmutable();
}
}
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return com.github.wenweihu86.raft.proto.RaftMessage.internal_static_raft_GetConfigurationResponse_descriptor;
}
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return com.github.wenweihu86.raft.proto.RaftMessage.internal_static_raft_GetConfigurationResponse_fieldAccessorTable
.ensureFieldAccessorsInitialized(
com.github.wenweihu86.raft.proto.RaftMessage.GetConfigurationResponse.class, com.github.wenweihu86.raft.proto.RaftMessage.GetConfigurationResponse.Builder.class);
}
private int bitField0_;
public static final int RES_CODE_FIELD_NUMBER = 1;
private int resCode_;
/**
* optional .raft.ResCode res_code = 1;
*/
public int getResCodeValue() {
return resCode_;
}
/**
* optional .raft.ResCode res_code = 1;
*/
public com.github.wenweihu86.raft.proto.RaftMessage.ResCode getResCode() {
com.github.wenweihu86.raft.proto.RaftMessage.ResCode result = com.github.wenweihu86.raft.proto.RaftMessage.ResCode.valueOf(resCode_);
return result == null ? com.github.wenweihu86.raft.proto.RaftMessage.ResCode.UNRECOGNIZED : result;
}
public static final int RES_MSG_FIELD_NUMBER = 2;
private volatile java.lang.Object resMsg_;
/**
* optional string res_msg = 2;
*/
public java.lang.String getResMsg() {
java.lang.Object ref = resMsg_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
resMsg_ = s;
return s;
}
}
/**
* optional string res_msg = 2;
*/
public com.google.protobuf.ByteString
getResMsgBytes() {
java.lang.Object ref = resMsg_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
resMsg_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
public static final int SERVERS_FIELD_NUMBER = 3;
private java.util.List servers_;
/**
* repeated .raft.Server servers = 3;
*/
public java.util.List getServersList() {
return servers_;
}
/**
* repeated .raft.Server servers = 3;
*/
public java.util.List extends com.github.wenweihu86.raft.proto.RaftMessage.ServerOrBuilder>
getServersOrBuilderList() {
return servers_;
}
/**
* repeated .raft.Server servers = 3;
*/
public int getServersCount() {
return servers_.size();
}
/**
* repeated .raft.Server servers = 3;
*/
public com.github.wenweihu86.raft.proto.RaftMessage.Server getServers(int index) {
return servers_.get(index);
}
/**
* repeated .raft.Server servers = 3;
*/
public com.github.wenweihu86.raft.proto.RaftMessage.ServerOrBuilder getServersOrBuilder(
int index) {
return servers_.get(index);
}
public static final int LEADER_FIELD_NUMBER = 4;
private com.github.wenweihu86.raft.proto.RaftMessage.Server leader_;
/**
* optional .raft.Server leader = 4;
*/
public boolean hasLeader() {
return leader_ != null;
}
/**
* optional .raft.Server leader = 4;
*/
public com.github.wenweihu86.raft.proto.RaftMessage.Server getLeader() {
return leader_ == null ? com.github.wenweihu86.raft.proto.RaftMessage.Server.getDefaultInstance() : leader_;
}
/**
* optional .raft.Server leader = 4;
*/
public com.github.wenweihu86.raft.proto.RaftMessage.ServerOrBuilder getLeaderOrBuilder() {
return getLeader();
}
private byte memoizedIsInitialized = -1;
public final boolean isInitialized() {
byte isInitialized = memoizedIsInitialized;
if (isInitialized == 1) return true;
if (isInitialized == 0) return false;
memoizedIsInitialized = 1;
return true;
}
public void writeTo(com.google.protobuf.CodedOutputStream output)
throws java.io.IOException {
if (resCode_ != com.github.wenweihu86.raft.proto.RaftMessage.ResCode.RES_CODE_SUCCESS.getNumber()) {
output.writeEnum(1, resCode_);
}
if (!getResMsgBytes().isEmpty()) {
com.google.protobuf.GeneratedMessageV3.writeString(output, 2, resMsg_);
}
for (int i = 0; i < servers_.size(); i++) {
output.writeMessage(3, servers_.get(i));
}
if (leader_ != null) {
output.writeMessage(4, getLeader());
}
}
public int getSerializedSize() {
int size = memoizedSize;
if (size != -1) return size;
size = 0;
if (resCode_ != com.github.wenweihu86.raft.proto.RaftMessage.ResCode.RES_CODE_SUCCESS.getNumber()) {
size += com.google.protobuf.CodedOutputStream
.computeEnumSize(1, resCode_);
}
if (!getResMsgBytes().isEmpty()) {
size += com.google.protobuf.GeneratedMessageV3.computeStringSize(2, resMsg_);
}
for (int i = 0; i < servers_.size(); i++) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(3, servers_.get(i));
}
if (leader_ != null) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(4, getLeader());
}
memoizedSize = size;
return size;
}
private static final long serialVersionUID = 0L;
@java.lang.Override
public boolean equals(final java.lang.Object obj) {
if (obj == this) {
return true;
}
if (!(obj instanceof com.github.wenweihu86.raft.proto.RaftMessage.GetConfigurationResponse)) {
return super.equals(obj);
}
com.github.wenweihu86.raft.proto.RaftMessage.GetConfigurationResponse other = (com.github.wenweihu86.raft.proto.RaftMessage.GetConfigurationResponse) obj;
boolean result = true;
result = result && resCode_ == other.resCode_;
result = result && getResMsg()
.equals(other.getResMsg());
result = result && getServersList()
.equals(other.getServersList());
result = result && (hasLeader() == other.hasLeader());
if (hasLeader()) {
result = result && getLeader()
.equals(other.getLeader());
}
return result;
}
@java.lang.Override
public int hashCode() {
if (memoizedHashCode != 0) {
return memoizedHashCode;
}
int hash = 41;
hash = (19 * hash) + getDescriptorForType().hashCode();
hash = (37 * hash) + RES_CODE_FIELD_NUMBER;
hash = (53 * hash) + resCode_;
hash = (37 * hash) + RES_MSG_FIELD_NUMBER;
hash = (53 * hash) + getResMsg().hashCode();
if (getServersCount() > 0) {
hash = (37 * hash) + SERVERS_FIELD_NUMBER;
hash = (53 * hash) + getServersList().hashCode();
}
if (hasLeader()) {
hash = (37 * hash) + LEADER_FIELD_NUMBER;
hash = (53 * hash) + getLeader().hashCode();
}
hash = (29 * hash) + unknownFields.hashCode();
memoizedHashCode = hash;
return hash;
}
public static com.github.wenweihu86.raft.proto.RaftMessage.GetConfigurationResponse parseFrom(
com.google.protobuf.ByteString data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static com.github.wenweihu86.raft.proto.RaftMessage.GetConfigurationResponse parseFrom(
com.google.protobuf.ByteString data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static com.github.wenweihu86.raft.proto.RaftMessage.GetConfigurationResponse parseFrom(byte[] data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static com.github.wenweihu86.raft.proto.RaftMessage.GetConfigurationResponse parseFrom(
byte[] data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static com.github.wenweihu86.raft.proto.RaftMessage.GetConfigurationResponse parseFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static com.github.wenweihu86.raft.proto.RaftMessage.GetConfigurationResponse parseFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
public static com.github.wenweihu86.raft.proto.RaftMessage.GetConfigurationResponse parseDelimitedFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input);
}
public static com.github.wenweihu86.raft.proto.RaftMessage.GetConfigurationResponse parseDelimitedFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input, extensionRegistry);
}
public static com.github.wenweihu86.raft.proto.RaftMessage.GetConfigurationResponse parseFrom(
com.google.protobuf.CodedInputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static com.github.wenweihu86.raft.proto.RaftMessage.GetConfigurationResponse parseFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
public Builder newBuilderForType() { return newBuilder(); }
public static Builder newBuilder() {
return DEFAULT_INSTANCE.toBuilder();
}
public static Builder newBuilder(com.github.wenweihu86.raft.proto.RaftMessage.GetConfigurationResponse prototype) {
return DEFAULT_INSTANCE.toBuilder().mergeFrom(prototype);
}
public Builder toBuilder() {
return this == DEFAULT_INSTANCE
? new Builder() : new Builder().mergeFrom(this);
}
@java.lang.Override
protected Builder newBuilderForType(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
Builder builder = new Builder(parent);
return builder;
}
/**
* Protobuf type {@code raft.GetConfigurationResponse}
*/
public static final class Builder extends
com.google.protobuf.GeneratedMessageV3.Builder implements
// @@protoc_insertion_point(builder_implements:raft.GetConfigurationResponse)
com.github.wenweihu86.raft.proto.RaftMessage.GetConfigurationResponseOrBuilder {
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return com.github.wenweihu86.raft.proto.RaftMessage.internal_static_raft_GetConfigurationResponse_descriptor;
}
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return com.github.wenweihu86.raft.proto.RaftMessage.internal_static_raft_GetConfigurationResponse_fieldAccessorTable
.ensureFieldAccessorsInitialized(
com.github.wenweihu86.raft.proto.RaftMessage.GetConfigurationResponse.class, com.github.wenweihu86.raft.proto.RaftMessage.GetConfigurationResponse.Builder.class);
}
// Construct using com.github.wenweihu86.raft.proto.RaftMessage.GetConfigurationResponse.newBuilder()
private Builder() {
maybeForceBuilderInitialization();
}
private Builder(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
super(parent);
maybeForceBuilderInitialization();
}
private void maybeForceBuilderInitialization() {
if (com.google.protobuf.GeneratedMessageV3
.alwaysUseFieldBuilders) {
getServersFieldBuilder();
}
}
public Builder clear() {
super.clear();
resCode_ = 0;
resMsg_ = "";
if (serversBuilder_ == null) {
servers_ = java.util.Collections.emptyList();
bitField0_ = (bitField0_ & ~0x00000004);
} else {
serversBuilder_.clear();
}
if (leaderBuilder_ == null) {
leader_ = null;
} else {
leader_ = null;
leaderBuilder_ = null;
}
return this;
}
public com.google.protobuf.Descriptors.Descriptor
getDescriptorForType() {
return com.github.wenweihu86.raft.proto.RaftMessage.internal_static_raft_GetConfigurationResponse_descriptor;
}
public com.github.wenweihu86.raft.proto.RaftMessage.GetConfigurationResponse getDefaultInstanceForType() {
return com.github.wenweihu86.raft.proto.RaftMessage.GetConfigurationResponse.getDefaultInstance();
}
public com.github.wenweihu86.raft.proto.RaftMessage.GetConfigurationResponse build() {
com.github.wenweihu86.raft.proto.RaftMessage.GetConfigurationResponse result = buildPartial();
if (!result.isInitialized()) {
throw newUninitializedMessageException(result);
}
return result;
}
public com.github.wenweihu86.raft.proto.RaftMessage.GetConfigurationResponse buildPartial() {
com.github.wenweihu86.raft.proto.RaftMessage.GetConfigurationResponse result = new com.github.wenweihu86.raft.proto.RaftMessage.GetConfigurationResponse(this);
int from_bitField0_ = bitField0_;
int to_bitField0_ = 0;
result.resCode_ = resCode_;
result.resMsg_ = resMsg_;
if (serversBuilder_ == null) {
if (((bitField0_ & 0x00000004) == 0x00000004)) {
servers_ = java.util.Collections.unmodifiableList(servers_);
bitField0_ = (bitField0_ & ~0x00000004);
}
result.servers_ = servers_;
} else {
result.servers_ = serversBuilder_.build();
}
if (leaderBuilder_ == null) {
result.leader_ = leader_;
} else {
result.leader_ = leaderBuilder_.build();
}
result.bitField0_ = to_bitField0_;
onBuilt();
return result;
}
public Builder clone() {
return (Builder) super.clone();
}
public Builder setField(
com.google.protobuf.Descriptors.FieldDescriptor field,
Object value) {
return (Builder) super.setField(field, value);
}
public Builder clearField(
com.google.protobuf.Descriptors.FieldDescriptor field) {
return (Builder) super.clearField(field);
}
public Builder clearOneof(
com.google.protobuf.Descriptors.OneofDescriptor oneof) {
return (Builder) super.clearOneof(oneof);
}
public Builder setRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
int index, Object value) {
return (Builder) super.setRepeatedField(field, index, value);
}
public Builder addRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
Object value) {
return (Builder) super.addRepeatedField(field, value);
}
public Builder mergeFrom(com.google.protobuf.Message other) {
if (other instanceof com.github.wenweihu86.raft.proto.RaftMessage.GetConfigurationResponse) {
return mergeFrom((com.github.wenweihu86.raft.proto.RaftMessage.GetConfigurationResponse)other);
} else {
super.mergeFrom(other);
return this;
}
}
public Builder mergeFrom(com.github.wenweihu86.raft.proto.RaftMessage.GetConfigurationResponse other) {
if (other == com.github.wenweihu86.raft.proto.RaftMessage.GetConfigurationResponse.getDefaultInstance()) return this;
if (other.resCode_ != 0) {
setResCodeValue(other.getResCodeValue());
}
if (!other.getResMsg().isEmpty()) {
resMsg_ = other.resMsg_;
onChanged();
}
if (serversBuilder_ == null) {
if (!other.servers_.isEmpty()) {
if (servers_.isEmpty()) {
servers_ = other.servers_;
bitField0_ = (bitField0_ & ~0x00000004);
} else {
ensureServersIsMutable();
servers_.addAll(other.servers_);
}
onChanged();
}
} else {
if (!other.servers_.isEmpty()) {
if (serversBuilder_.isEmpty()) {
serversBuilder_.dispose();
serversBuilder_ = null;
servers_ = other.servers_;
bitField0_ = (bitField0_ & ~0x00000004);
serversBuilder_ =
com.google.protobuf.GeneratedMessageV3.alwaysUseFieldBuilders ?
getServersFieldBuilder() : null;
} else {
serversBuilder_.addAllMessages(other.servers_);
}
}
}
if (other.hasLeader()) {
mergeLeader(other.getLeader());
}
onChanged();
return this;
}
public final boolean isInitialized() {
return true;
}
public Builder mergeFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
com.github.wenweihu86.raft.proto.RaftMessage.GetConfigurationResponse parsedMessage = null;
try {
parsedMessage = PARSER.parsePartialFrom(input, extensionRegistry);
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
parsedMessage = (com.github.wenweihu86.raft.proto.RaftMessage.GetConfigurationResponse) e.getUnfinishedMessage();
throw e.unwrapIOException();
} finally {
if (parsedMessage != null) {
mergeFrom(parsedMessage);
}
}
return this;
}
private int bitField0_;
private int resCode_ = 0;
/**
* optional .raft.ResCode res_code = 1;
*/
public int getResCodeValue() {
return resCode_;
}
/**
* optional .raft.ResCode res_code = 1;
*/
public Builder setResCodeValue(int value) {
resCode_ = value;
onChanged();
return this;
}
/**
* optional .raft.ResCode res_code = 1;
*/
public com.github.wenweihu86.raft.proto.RaftMessage.ResCode getResCode() {
com.github.wenweihu86.raft.proto.RaftMessage.ResCode result = com.github.wenweihu86.raft.proto.RaftMessage.ResCode.valueOf(resCode_);
return result == null ? com.github.wenweihu86.raft.proto.RaftMessage.ResCode.UNRECOGNIZED : result;
}
/**
* optional .raft.ResCode res_code = 1;
*/
public Builder setResCode(com.github.wenweihu86.raft.proto.RaftMessage.ResCode value) {
if (value == null) {
throw new NullPointerException();
}
resCode_ = value.getNumber();
onChanged();
return this;
}
/**
* optional .raft.ResCode res_code = 1;
*/
public Builder clearResCode() {
resCode_ = 0;
onChanged();
return this;
}
private java.lang.Object resMsg_ = "";
/**
* optional string res_msg = 2;
*/
public java.lang.String getResMsg() {
java.lang.Object ref = resMsg_;
if (!(ref instanceof java.lang.String)) {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
resMsg_ = s;
return s;
} else {
return (java.lang.String) ref;
}
}
/**
* optional string res_msg = 2;
*/
public com.google.protobuf.ByteString
getResMsgBytes() {
java.lang.Object ref = resMsg_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
resMsg_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
* optional string res_msg = 2;
*/
public Builder setResMsg(
java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
resMsg_ = value;
onChanged();
return this;
}
/**
* optional string res_msg = 2;
*/
public Builder clearResMsg() {
resMsg_ = getDefaultInstance().getResMsg();
onChanged();
return this;
}
/**
* optional string res_msg = 2;
*/
public Builder setResMsgBytes(
com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
checkByteStringIsUtf8(value);
resMsg_ = value;
onChanged();
return this;
}
private java.util.List servers_ =
java.util.Collections.emptyList();
private void ensureServersIsMutable() {
if (!((bitField0_ & 0x00000004) == 0x00000004)) {
servers_ = new java.util.ArrayList(servers_);
bitField0_ |= 0x00000004;
}
}
private com.google.protobuf.RepeatedFieldBuilderV3<
com.github.wenweihu86.raft.proto.RaftMessage.Server, com.github.wenweihu86.raft.proto.RaftMessage.Server.Builder, com.github.wenweihu86.raft.proto.RaftMessage.ServerOrBuilder> serversBuilder_;
/**
* repeated .raft.Server servers = 3;
*/
public java.util.List getServersList() {
if (serversBuilder_ == null) {
return java.util.Collections.unmodifiableList(servers_);
} else {
return serversBuilder_.getMessageList();
}
}
/**
* repeated .raft.Server servers = 3;
*/
public int getServersCount() {
if (serversBuilder_ == null) {
return servers_.size();
} else {
return serversBuilder_.getCount();
}
}
/**
* repeated .raft.Server servers = 3;
*/
public com.github.wenweihu86.raft.proto.RaftMessage.Server getServers(int index) {
if (serversBuilder_ == null) {
return servers_.get(index);
} else {
return serversBuilder_.getMessage(index);
}
}
/**
* repeated .raft.Server servers = 3;
*/
public Builder setServers(
int index, com.github.wenweihu86.raft.proto.RaftMessage.Server value) {
if (serversBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
ensureServersIsMutable();
servers_.set(index, value);
onChanged();
} else {
serversBuilder_.setMessage(index, value);
}
return this;
}
/**
* repeated .raft.Server servers = 3;
*/
public Builder setServers(
int index, com.github.wenweihu86.raft.proto.RaftMessage.Server.Builder builderForValue) {
if (serversBuilder_ == null) {
ensureServersIsMutable();
servers_.set(index, builderForValue.build());
onChanged();
} else {
serversBuilder_.setMessage(index, builderForValue.build());
}
return this;
}
/**
* repeated .raft.Server servers = 3;
*/
public Builder addServers(com.github.wenweihu86.raft.proto.RaftMessage.Server value) {
if (serversBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
ensureServersIsMutable();
servers_.add(value);
onChanged();
} else {
serversBuilder_.addMessage(value);
}
return this;
}
/**
* repeated .raft.Server servers = 3;
*/
public Builder addServers(
int index, com.github.wenweihu86.raft.proto.RaftMessage.Server value) {
if (serversBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
ensureServersIsMutable();
servers_.add(index, value);
onChanged();
} else {
serversBuilder_.addMessage(index, value);
}
return this;
}
/**
* repeated .raft.Server servers = 3;
*/
public Builder addServers(
com.github.wenweihu86.raft.proto.RaftMessage.Server.Builder builderForValue) {
if (serversBuilder_ == null) {
ensureServersIsMutable();
servers_.add(builderForValue.build());
onChanged();
} else {
serversBuilder_.addMessage(builderForValue.build());
}
return this;
}
/**
* repeated .raft.Server servers = 3;
*/
public Builder addServers(
int index, com.github.wenweihu86.raft.proto.RaftMessage.Server.Builder builderForValue) {
if (serversBuilder_ == null) {
ensureServersIsMutable();
servers_.add(index, builderForValue.build());
onChanged();
} else {
serversBuilder_.addMessage(index, builderForValue.build());
}
return this;
}
/**
* repeated .raft.Server servers = 3;
*/
public Builder addAllServers(
java.lang.Iterable extends com.github.wenweihu86.raft.proto.RaftMessage.Server> values) {
if (serversBuilder_ == null) {
ensureServersIsMutable();
com.google.protobuf.AbstractMessageLite.Builder.addAll(
values, servers_);
onChanged();
} else {
serversBuilder_.addAllMessages(values);
}
return this;
}
/**
* repeated .raft.Server servers = 3;
*/
public Builder clearServers() {
if (serversBuilder_ == null) {
servers_ = java.util.Collections.emptyList();
bitField0_ = (bitField0_ & ~0x00000004);
onChanged();
} else {
serversBuilder_.clear();
}
return this;
}
/**
* repeated .raft.Server servers = 3;
*/
public Builder removeServers(int index) {
if (serversBuilder_ == null) {
ensureServersIsMutable();
servers_.remove(index);
onChanged();
} else {
serversBuilder_.remove(index);
}
return this;
}
/**
* repeated .raft.Server servers = 3;
*/
public com.github.wenweihu86.raft.proto.RaftMessage.Server.Builder getServersBuilder(
int index) {
return getServersFieldBuilder().getBuilder(index);
}
/**
* repeated .raft.Server servers = 3;
*/
public com.github.wenweihu86.raft.proto.RaftMessage.ServerOrBuilder getServersOrBuilder(
int index) {
if (serversBuilder_ == null) {
return servers_.get(index); } else {
return serversBuilder_.getMessageOrBuilder(index);
}
}
/**
* repeated .raft.Server servers = 3;
*/
public java.util.List extends com.github.wenweihu86.raft.proto.RaftMessage.ServerOrBuilder>
getServersOrBuilderList() {
if (serversBuilder_ != null) {
return serversBuilder_.getMessageOrBuilderList();
} else {
return java.util.Collections.unmodifiableList(servers_);
}
}
/**
* repeated .raft.Server servers = 3;
*/
public com.github.wenweihu86.raft.proto.RaftMessage.Server.Builder addServersBuilder() {
return getServersFieldBuilder().addBuilder(
com.github.wenweihu86.raft.proto.RaftMessage.Server.getDefaultInstance());
}
/**
* repeated .raft.Server servers = 3;
*/
public com.github.wenweihu86.raft.proto.RaftMessage.Server.Builder addServersBuilder(
int index) {
return getServersFieldBuilder().addBuilder(
index, com.github.wenweihu86.raft.proto.RaftMessage.Server.getDefaultInstance());
}
/**
* repeated .raft.Server servers = 3;
*/
public java.util.List
getServersBuilderList() {
return getServersFieldBuilder().getBuilderList();
}
private com.google.protobuf.RepeatedFieldBuilderV3<
com.github.wenweihu86.raft.proto.RaftMessage.Server, com.github.wenweihu86.raft.proto.RaftMessage.Server.Builder, com.github.wenweihu86.raft.proto.RaftMessage.ServerOrBuilder>
getServersFieldBuilder() {
if (serversBuilder_ == null) {
serversBuilder_ = new com.google.protobuf.RepeatedFieldBuilderV3<
com.github.wenweihu86.raft.proto.RaftMessage.Server, com.github.wenweihu86.raft.proto.RaftMessage.Server.Builder, com.github.wenweihu86.raft.proto.RaftMessage.ServerOrBuilder>(
servers_,
((bitField0_ & 0x00000004) == 0x00000004),
getParentForChildren(),
isClean());
servers_ = null;
}
return serversBuilder_;
}
private com.github.wenweihu86.raft.proto.RaftMessage.Server leader_ = null;
private com.google.protobuf.SingleFieldBuilderV3<
com.github.wenweihu86.raft.proto.RaftMessage.Server, com.github.wenweihu86.raft.proto.RaftMessage.Server.Builder, com.github.wenweihu86.raft.proto.RaftMessage.ServerOrBuilder> leaderBuilder_;
/**
* optional .raft.Server leader = 4;
*/
public boolean hasLeader() {
return leaderBuilder_ != null || leader_ != null;
}
/**
* optional .raft.Server leader = 4;
*/
public com.github.wenweihu86.raft.proto.RaftMessage.Server getLeader() {
if (leaderBuilder_ == null) {
return leader_ == null ? com.github.wenweihu86.raft.proto.RaftMessage.Server.getDefaultInstance() : leader_;
} else {
return leaderBuilder_.getMessage();
}
}
/**
* optional .raft.Server leader = 4;
*/
public Builder setLeader(com.github.wenweihu86.raft.proto.RaftMessage.Server value) {
if (leaderBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
leader_ = value;
onChanged();
} else {
leaderBuilder_.setMessage(value);
}
return this;
}
/**
* optional .raft.Server leader = 4;
*/
public Builder setLeader(
com.github.wenweihu86.raft.proto.RaftMessage.Server.Builder builderForValue) {
if (leaderBuilder_ == null) {
leader_ = builderForValue.build();
onChanged();
} else {
leaderBuilder_.setMessage(builderForValue.build());
}
return this;
}
/**
* optional .raft.Server leader = 4;
*/
public Builder mergeLeader(com.github.wenweihu86.raft.proto.RaftMessage.Server value) {
if (leaderBuilder_ == null) {
if (leader_ != null) {
leader_ =
com.github.wenweihu86.raft.proto.RaftMessage.Server.newBuilder(leader_).mergeFrom(value).buildPartial();
} else {
leader_ = value;
}
onChanged();
} else {
leaderBuilder_.mergeFrom(value);
}
return this;
}
/**
* optional .raft.Server leader = 4;
*/
public Builder clearLeader() {
if (leaderBuilder_ == null) {
leader_ = null;
onChanged();
} else {
leader_ = null;
leaderBuilder_ = null;
}
return this;
}
/**
* optional .raft.Server leader = 4;
*/
public com.github.wenweihu86.raft.proto.RaftMessage.Server.Builder getLeaderBuilder() {
onChanged();
return getLeaderFieldBuilder().getBuilder();
}
/**
* optional .raft.Server leader = 4;
*/
public com.github.wenweihu86.raft.proto.RaftMessage.ServerOrBuilder getLeaderOrBuilder() {
if (leaderBuilder_ != null) {
return leaderBuilder_.getMessageOrBuilder();
} else {
return leader_ == null ?
com.github.wenweihu86.raft.proto.RaftMessage.Server.getDefaultInstance() : leader_;
}
}
/**
* optional .raft.Server leader = 4;
*/
private com.google.protobuf.SingleFieldBuilderV3<
com.github.wenweihu86.raft.proto.RaftMessage.Server, com.github.wenweihu86.raft.proto.RaftMessage.Server.Builder, com.github.wenweihu86.raft.proto.RaftMessage.ServerOrBuilder>
getLeaderFieldBuilder() {
if (leaderBuilder_ == null) {
leaderBuilder_ = new com.google.protobuf.SingleFieldBuilderV3<
com.github.wenweihu86.raft.proto.RaftMessage.Server, com.github.wenweihu86.raft.proto.RaftMessage.Server.Builder, com.github.wenweihu86.raft.proto.RaftMessage.ServerOrBuilder>(
getLeader(),
getParentForChildren(),
isClean());
leader_ = null;
}
return leaderBuilder_;
}
public final Builder setUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return this;
}
public final Builder mergeUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return this;
}
// @@protoc_insertion_point(builder_scope:raft.GetConfigurationResponse)
}
// @@protoc_insertion_point(class_scope:raft.GetConfigurationResponse)
private static final com.github.wenweihu86.raft.proto.RaftMessage.GetConfigurationResponse DEFAULT_INSTANCE;
static {
DEFAULT_INSTANCE = new com.github.wenweihu86.raft.proto.RaftMessage.GetConfigurationResponse();
}
public static com.github.wenweihu86.raft.proto.RaftMessage.GetConfigurationResponse getDefaultInstance() {
return DEFAULT_INSTANCE;
}
private static final com.google.protobuf.Parser
PARSER = new com.google.protobuf.AbstractParser() {
public GetConfigurationResponse parsePartialFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return new GetConfigurationResponse(input, extensionRegistry);
}
};
public static com.google.protobuf.Parser parser() {
return PARSER;
}
@java.lang.Override
public com.google.protobuf.Parser getParserForType() {
return PARSER;
}
public com.github.wenweihu86.raft.proto.RaftMessage.GetConfigurationResponse getDefaultInstanceForType() {
return DEFAULT_INSTANCE;
}
}
private static final com.google.protobuf.Descriptors.Descriptor
internal_static_raft_EndPoint_descriptor;
private static final
com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internal_static_raft_EndPoint_fieldAccessorTable;
private static final com.google.protobuf.Descriptors.Descriptor
internal_static_raft_Server_descriptor;
private static final
com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internal_static_raft_Server_fieldAccessorTable;
private static final com.google.protobuf.Descriptors.Descriptor
internal_static_raft_Configuration_descriptor;
private static final
com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internal_static_raft_Configuration_fieldAccessorTable;
private static final com.google.protobuf.Descriptors.Descriptor
internal_static_raft_LogMetaData_descriptor;
private static final
com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internal_static_raft_LogMetaData_fieldAccessorTable;
private static final com.google.protobuf.Descriptors.Descriptor
internal_static_raft_SnapshotMetaData_descriptor;
private static final
com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internal_static_raft_SnapshotMetaData_fieldAccessorTable;
private static final com.google.protobuf.Descriptors.Descriptor
internal_static_raft_LogEntry_descriptor;
private static final
com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internal_static_raft_LogEntry_fieldAccessorTable;
private static final com.google.protobuf.Descriptors.Descriptor
internal_static_raft_VoteRequest_descriptor;
private static final
com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internal_static_raft_VoteRequest_fieldAccessorTable;
private static final com.google.protobuf.Descriptors.Descriptor
internal_static_raft_VoteResponse_descriptor;
private static final
com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internal_static_raft_VoteResponse_fieldAccessorTable;
private static final com.google.protobuf.Descriptors.Descriptor
internal_static_raft_AppendEntriesRequest_descriptor;
private static final
com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internal_static_raft_AppendEntriesRequest_fieldAccessorTable;
private static final com.google.protobuf.Descriptors.Descriptor
internal_static_raft_AppendEntriesResponse_descriptor;
private static final
com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internal_static_raft_AppendEntriesResponse_fieldAccessorTable;
private static final com.google.protobuf.Descriptors.Descriptor
internal_static_raft_InstallSnapshotRequest_descriptor;
private static final
com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internal_static_raft_InstallSnapshotRequest_fieldAccessorTable;
private static final com.google.protobuf.Descriptors.Descriptor
internal_static_raft_InstallSnapshotResponse_descriptor;
private static final
com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internal_static_raft_InstallSnapshotResponse_fieldAccessorTable;
private static final com.google.protobuf.Descriptors.Descriptor
internal_static_raft_GetLeaderRequest_descriptor;
private static final
com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internal_static_raft_GetLeaderRequest_fieldAccessorTable;
private static final com.google.protobuf.Descriptors.Descriptor
internal_static_raft_GetLeaderResponse_descriptor;
private static final
com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internal_static_raft_GetLeaderResponse_fieldAccessorTable;
private static final com.google.protobuf.Descriptors.Descriptor
internal_static_raft_AddPeersRequest_descriptor;
private static final
com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internal_static_raft_AddPeersRequest_fieldAccessorTable;
private static final com.google.protobuf.Descriptors.Descriptor
internal_static_raft_AddPeersResponse_descriptor;
private static final
com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internal_static_raft_AddPeersResponse_fieldAccessorTable;
private static final com.google.protobuf.Descriptors.Descriptor
internal_static_raft_RemovePeersRequest_descriptor;
private static final
com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internal_static_raft_RemovePeersRequest_fieldAccessorTable;
private static final com.google.protobuf.Descriptors.Descriptor
internal_static_raft_RemovePeersResponse_descriptor;
private static final
com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internal_static_raft_RemovePeersResponse_fieldAccessorTable;
private static final com.google.protobuf.Descriptors.Descriptor
internal_static_raft_GetConfigurationRequest_descriptor;
private static final
com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internal_static_raft_GetConfigurationRequest_fieldAccessorTable;
private static final com.google.protobuf.Descriptors.Descriptor
internal_static_raft_GetConfigurationResponse_descriptor;
private static final
com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internal_static_raft_GetConfigurationResponse_fieldAccessorTable;
public static com.google.protobuf.Descriptors.FileDescriptor
getDescriptor() {
return descriptor;
}
private static com.google.protobuf.Descriptors.FileDescriptor
descriptor;
static {
java.lang.String[] descriptorData = {
"\n\nraft.proto\022\004raft\"&\n\010EndPoint\022\014\n\004host\030\001" +
" \001(\t\022\014\n\004port\030\002 \001(\r\">\n\006Server\022\021\n\tserver_i" +
"d\030\001 \001(\r\022!\n\tend_point\030\002 \001(\0132\016.raft.EndPoi" +
"nt\".\n\rConfiguration\022\035\n\007servers\030\001 \003(\0132\014.r" +
"aft.Server\"O\n\013LogMetaData\022\024\n\014current_ter" +
"m\030\001 \001(\004\022\021\n\tvoted_for\030\002 \001(\r\022\027\n\017first_log_" +
"index\030\003 \001(\004\"w\n\020SnapshotMetaData\022\033\n\023last_" +
"included_index\030\001 \001(\004\022\032\n\022last_included_te" +
"rm\030\002 \001(\004\022*\n\rconfiguration\030\003 \001(\0132\023.raft.C" +
"onfiguration\"T\n\010LogEntry\022\014\n\004term\030\001 \001(\004\022\r",
"\n\005index\030\002 \001(\004\022\035\n\004type\030\003 \001(\0162\017.raft.Entry" +
"Type\022\014\n\004data\030\004 \001(\014\"]\n\013VoteRequest\022\021\n\tser" +
"ver_id\030\001 \001(\r\022\014\n\004term\030\002 \001(\004\022\025\n\rlast_log_t" +
"erm\030\003 \001(\004\022\026\n\016last_log_index\030\004 \001(\004\"-\n\014Vot" +
"eResponse\022\014\n\004term\030\001 \001(\004\022\017\n\007granted\030\002 \001(\010" +
"\"\235\001\n\024AppendEntriesRequest\022\021\n\tserver_id\030\001" +
" \001(\r\022\014\n\004term\030\002 \001(\004\022\026\n\016prev_log_index\030\003 \001" +
"(\004\022\025\n\rprev_log_term\030\004 \001(\004\022\037\n\007entries\030\005 \003" +
"(\0132\016.raft.LogEntry\022\024\n\014commit_index\030\006 \001(\004" +
"\"^\n\025AppendEntriesResponse\022\037\n\010res_code\030\001 ",
"\001(\0162\r.raft.ResCode\022\014\n\004term\030\002 \001(\004\022\026\n\016last" +
"_log_index\030\003 \001(\004\"\301\001\n\026InstallSnapshotRequ" +
"est\022\021\n\tserver_id\030\001 \001(\r\022\014\n\004term\030\002 \001(\004\0222\n\022" +
"snapshot_meta_data\030\003 \001(\0132\026.raft.Snapshot" +
"MetaData\022\021\n\tfile_name\030\004 \001(\t\022\016\n\006offset\030\005 " +
"\001(\004\022\014\n\004data\030\006 \001(\014\022\020\n\010is_first\030\007 \001(\010\022\017\n\007i" +
"s_last\030\010 \001(\010\"H\n\027InstallSnapshotResponse\022" +
"\037\n\010res_code\030\001 \001(\0162\r.raft.ResCode\022\014\n\004term" +
"\030\002 \001(\004\"\022\n\020GetLeaderRequest\"e\n\021GetLeaderR" +
"esponse\022\037\n\010res_code\030\001 \001(\0162\r.raft.ResCode",
"\022\017\n\007res_msg\030\002 \001(\t\022\036\n\006leader\030\003 \001(\0132\016.raft" +
".EndPoint\"0\n\017AddPeersRequest\022\035\n\007servers\030" +
"\001 \003(\0132\014.raft.Server\"D\n\020AddPeersResponse\022" +
"\037\n\010res_code\030\001 \001(\0162\r.raft.ResCode\022\017\n\007res_" +
"msg\030\002 \001(\t\"3\n\022RemovePeersRequest\022\035\n\007serve" +
"rs\030\001 \003(\0132\014.raft.Server\"G\n\023RemovePeersRes" +
"ponse\022\037\n\010res_code\030\001 \001(\0162\r.raft.ResCode\022\017" +
"\n\007res_msg\030\002 \001(\t\"\031\n\027GetConfigurationReque" +
"st\"\211\001\n\030GetConfigurationResponse\022\037\n\010res_c" +
"ode\030\001 \001(\0162\r.raft.ResCode\022\017\n\007res_msg\030\002 \001(",
"\t\022\035\n\007servers\030\003 \003(\0132\014.raft.Server\022\034\n\006lead" +
"er\030\004 \001(\0132\014.raft.Server*K\n\007ResCode\022\024\n\020RES" +
"_CODE_SUCCESS\020\000\022\021\n\rRES_CODE_FAIL\020\001\022\027\n\023RE" +
"S_CODE_NOT_LEADER\020\002*>\n\tEntryType\022\023\n\017ENTR" +
"Y_TYPE_DATA\020\000\022\034\n\030ENTRY_TYPE_CONFIGURATIO" +
"N\020\001B/\n com.github.wenweihu86.raft.protoB" +
"\013RaftMessageb\006proto3"
};
com.google.protobuf.Descriptors.FileDescriptor.InternalDescriptorAssigner assigner =
new com.google.protobuf.Descriptors.FileDescriptor. InternalDescriptorAssigner() {
public com.google.protobuf.ExtensionRegistry assignDescriptors(
com.google.protobuf.Descriptors.FileDescriptor root) {
descriptor = root;
return null;
}
};
com.google.protobuf.Descriptors.FileDescriptor
.internalBuildGeneratedFileFrom(descriptorData,
new com.google.protobuf.Descriptors.FileDescriptor[] {
}, assigner);
internal_static_raft_EndPoint_descriptor =
getDescriptor().getMessageTypes().get(0);
internal_static_raft_EndPoint_fieldAccessorTable = new
com.google.protobuf.GeneratedMessageV3.FieldAccessorTable(
internal_static_raft_EndPoint_descriptor,
new java.lang.String[] { "Host", "Port", });
internal_static_raft_Server_descriptor =
getDescriptor().getMessageTypes().get(1);
internal_static_raft_Server_fieldAccessorTable = new
com.google.protobuf.GeneratedMessageV3.FieldAccessorTable(
internal_static_raft_Server_descriptor,
new java.lang.String[] { "ServerId", "EndPoint", });
internal_static_raft_Configuration_descriptor =
getDescriptor().getMessageTypes().get(2);
internal_static_raft_Configuration_fieldAccessorTable = new
com.google.protobuf.GeneratedMessageV3.FieldAccessorTable(
internal_static_raft_Configuration_descriptor,
new java.lang.String[] { "Servers", });
internal_static_raft_LogMetaData_descriptor =
getDescriptor().getMessageTypes().get(3);
internal_static_raft_LogMetaData_fieldAccessorTable = new
com.google.protobuf.GeneratedMessageV3.FieldAccessorTable(
internal_static_raft_LogMetaData_descriptor,
new java.lang.String[] { "CurrentTerm", "VotedFor", "FirstLogIndex", });
internal_static_raft_SnapshotMetaData_descriptor =
getDescriptor().getMessageTypes().get(4);
internal_static_raft_SnapshotMetaData_fieldAccessorTable = new
com.google.protobuf.GeneratedMessageV3.FieldAccessorTable(
internal_static_raft_SnapshotMetaData_descriptor,
new java.lang.String[] { "LastIncludedIndex", "LastIncludedTerm", "Configuration", });
internal_static_raft_LogEntry_descriptor =
getDescriptor().getMessageTypes().get(5);
internal_static_raft_LogEntry_fieldAccessorTable = new
com.google.protobuf.GeneratedMessageV3.FieldAccessorTable(
internal_static_raft_LogEntry_descriptor,
new java.lang.String[] { "Term", "Index", "Type", "Data", });
internal_static_raft_VoteRequest_descriptor =
getDescriptor().getMessageTypes().get(6);
internal_static_raft_VoteRequest_fieldAccessorTable = new
com.google.protobuf.GeneratedMessageV3.FieldAccessorTable(
internal_static_raft_VoteRequest_descriptor,
new java.lang.String[] { "ServerId", "Term", "LastLogTerm", "LastLogIndex", });
internal_static_raft_VoteResponse_descriptor =
getDescriptor().getMessageTypes().get(7);
internal_static_raft_VoteResponse_fieldAccessorTable = new
com.google.protobuf.GeneratedMessageV3.FieldAccessorTable(
internal_static_raft_VoteResponse_descriptor,
new java.lang.String[] { "Term", "Granted", });
internal_static_raft_AppendEntriesRequest_descriptor =
getDescriptor().getMessageTypes().get(8);
internal_static_raft_AppendEntriesRequest_fieldAccessorTable = new
com.google.protobuf.GeneratedMessageV3.FieldAccessorTable(
internal_static_raft_AppendEntriesRequest_descriptor,
new java.lang.String[] { "ServerId", "Term", "PrevLogIndex", "PrevLogTerm", "Entries", "CommitIndex", });
internal_static_raft_AppendEntriesResponse_descriptor =
getDescriptor().getMessageTypes().get(9);
internal_static_raft_AppendEntriesResponse_fieldAccessorTable = new
com.google.protobuf.GeneratedMessageV3.FieldAccessorTable(
internal_static_raft_AppendEntriesResponse_descriptor,
new java.lang.String[] { "ResCode", "Term", "LastLogIndex", });
internal_static_raft_InstallSnapshotRequest_descriptor =
getDescriptor().getMessageTypes().get(10);
internal_static_raft_InstallSnapshotRequest_fieldAccessorTable = new
com.google.protobuf.GeneratedMessageV3.FieldAccessorTable(
internal_static_raft_InstallSnapshotRequest_descriptor,
new java.lang.String[] { "ServerId", "Term", "SnapshotMetaData", "FileName", "Offset", "Data", "IsFirst", "IsLast", });
internal_static_raft_InstallSnapshotResponse_descriptor =
getDescriptor().getMessageTypes().get(11);
internal_static_raft_InstallSnapshotResponse_fieldAccessorTable = new
com.google.protobuf.GeneratedMessageV3.FieldAccessorTable(
internal_static_raft_InstallSnapshotResponse_descriptor,
new java.lang.String[] { "ResCode", "Term", });
internal_static_raft_GetLeaderRequest_descriptor =
getDescriptor().getMessageTypes().get(12);
internal_static_raft_GetLeaderRequest_fieldAccessorTable = new
com.google.protobuf.GeneratedMessageV3.FieldAccessorTable(
internal_static_raft_GetLeaderRequest_descriptor,
new java.lang.String[] { });
internal_static_raft_GetLeaderResponse_descriptor =
getDescriptor().getMessageTypes().get(13);
internal_static_raft_GetLeaderResponse_fieldAccessorTable = new
com.google.protobuf.GeneratedMessageV3.FieldAccessorTable(
internal_static_raft_GetLeaderResponse_descriptor,
new java.lang.String[] { "ResCode", "ResMsg", "Leader", });
internal_static_raft_AddPeersRequest_descriptor =
getDescriptor().getMessageTypes().get(14);
internal_static_raft_AddPeersRequest_fieldAccessorTable = new
com.google.protobuf.GeneratedMessageV3.FieldAccessorTable(
internal_static_raft_AddPeersRequest_descriptor,
new java.lang.String[] { "Servers", });
internal_static_raft_AddPeersResponse_descriptor =
getDescriptor().getMessageTypes().get(15);
internal_static_raft_AddPeersResponse_fieldAccessorTable = new
com.google.protobuf.GeneratedMessageV3.FieldAccessorTable(
internal_static_raft_AddPeersResponse_descriptor,
new java.lang.String[] { "ResCode", "ResMsg", });
internal_static_raft_RemovePeersRequest_descriptor =
getDescriptor().getMessageTypes().get(16);
internal_static_raft_RemovePeersRequest_fieldAccessorTable = new
com.google.protobuf.GeneratedMessageV3.FieldAccessorTable(
internal_static_raft_RemovePeersRequest_descriptor,
new java.lang.String[] { "Servers", });
internal_static_raft_RemovePeersResponse_descriptor =
getDescriptor().getMessageTypes().get(17);
internal_static_raft_RemovePeersResponse_fieldAccessorTable = new
com.google.protobuf.GeneratedMessageV3.FieldAccessorTable(
internal_static_raft_RemovePeersResponse_descriptor,
new java.lang.String[] { "ResCode", "ResMsg", });
internal_static_raft_GetConfigurationRequest_descriptor =
getDescriptor().getMessageTypes().get(18);
internal_static_raft_GetConfigurationRequest_fieldAccessorTable = new
com.google.protobuf.GeneratedMessageV3.FieldAccessorTable(
internal_static_raft_GetConfigurationRequest_descriptor,
new java.lang.String[] { });
internal_static_raft_GetConfigurationResponse_descriptor =
getDescriptor().getMessageTypes().get(19);
internal_static_raft_GetConfigurationResponse_fieldAccessorTable = new
com.google.protobuf.GeneratedMessageV3.FieldAccessorTable(
internal_static_raft_GetConfigurationResponse_descriptor,
new java.lang.String[] { "ResCode", "ResMsg", "Servers", "Leader", });
}
// @@protoc_insertion_point(outer_class_scope)
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy