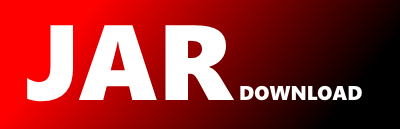
com.github.wglanzer.annosave.api.AnnoSaveGZip Maven / Gradle / Ivy
package com.github.wglanzer.annosave.api;
import org.apache.commons.io.input.CloseShieldInputStream;
import org.apache.commons.io.output.CloseShieldOutputStream;
import org.jetbrains.annotations.NotNull;
import java.io.*;
import java.net.URI;
import java.nio.file.FileSystem;
import java.nio.file.*;
import java.util.*;
import java.util.zip.*;
/**
* Contains API-Methods to compress multiple classes to a .zip-file
*
* @author W.Glanzer, 13.09.2017
*/
@SuppressWarnings({"unused", "UnusedReturnValue"})
public class AnnoSaveGZip
{
/**
* Saves all annotation-descriptions from all classes
* inside a .zip-File which is located at pZipFile
*
* @param pClasses All classes which should be stored
* @param pZipFile Path to zip-file
* @return all created containers
*/
@NotNull
public static IAnnotationContainer[] write(@NotNull Class>[] pClasses, @NotNull File pZipFile)
{
try (ZipOutputStream zipOutputStream = new ZipOutputStream(new FileOutputStream(pZipFile)))
{
IAnnotationContainer[] containers = new IAnnotationContainer[pClasses.length];
for (int i = 0; i < pClasses.length; i++)
{
zipOutputStream.putNextEntry(new ZipEntry(pClasses[i].getName() + ".json"));
IAnnotationContainer container = AnnoSave.write(pClasses[i], new CloseShieldOutputStream(zipOutputStream));
zipOutputStream.closeEntry();
containers[i] = container;
}
return containers;
}
catch (Exception e)
{
throw new RuntimeException(e);
}
}
/**
* Saves all annotation-descriptions
* inside a .zip-File which is located at pZipFile
*
* @param pObjects All objects which should be stored
* @param pConverter Converter to convert T-objects to saveable IAnnotation-Objects
* @param pZipFile Path to zip-file
* @return all created containers
*/
@NotNull
public static IAnnotationContainer[] write(@NotNull T[] pObjects, @NotNull IAnnoSaveConverter pConverter, @NotNull File pZipFile)
{
// Nothing to write -> no zip-file, empty array
if(pObjects.length == 0)
return new IAnnotationContainer[0];
try
{
HashMap env = new HashMap<>();
env.put("create", String.valueOf(!pZipFile.exists()));
env.put("encoding", "UTF-8");
try (FileSystem zipFs = FileSystems.newFileSystem(URI.create("jar:" + pZipFile.toURI()), env))
{
IAnnotationContainer[] containers = new IAnnotationContainer[pObjects.length];
for (int i = 0; i < pObjects.length; i++)
{
try (ByteArrayOutputStream baos = new ByteArrayOutputStream())
{
IAnnotationContainer container = AnnoSave.write(pObjects[i], pConverter, baos);
Path file = zipFs.getPath("/" + container.getName() + ".json");
Files.copy(new ByteArrayInputStream(baos.toByteArray()), file, StandardCopyOption.REPLACE_EXISTING);
containers[i] = container;
}
}
return containers;
}
}
catch(Exception e)
{
throw new RuntimeException(e);
}
}
/**
* Reads all annotation-descriptions from a .zip-File
*
* @param pZipFile File, which should be read
* @return all containers
*/
@NotNull
public static IAnnotationContainer[] read(@NotNull File pZipFile)
{
List containers = new ArrayList<>();
try (ZipFile zipFile = new ZipFile(pZipFile))
{
Enumeration extends ZipEntry> entries = zipFile.entries();
while (entries.hasMoreElements())
{
InputStream inputStream = zipFile.getInputStream(entries.nextElement());
containers.add(AnnoSave.read(inputStream));
}
return containers.toArray(new IAnnotationContainer[containers.size()]);
}
catch (Exception e)
{
throw new RuntimeException(e);
}
}
/**
* Reads all annotation-descriptions from an InputStream
*
* @param pStream Stream, which should be read
* @return all containers
*/
@NotNull
public static IAnnotationContainer[] read(@NotNull InputStream pStream)
{
List containers = new ArrayList<>();
try (ZipInputStream zipFile = new ZipInputStream(pStream))
{
while (zipFile.getNextEntry() != null)
containers.add(AnnoSave.read(new CloseShieldInputStream(zipFile)));
return containers.toArray(new IAnnotationContainer[containers.size()]);
}
catch (Exception e)
{
throw new RuntimeException(e);
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy