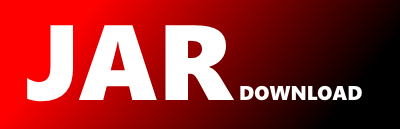
com.github.jy2.introspection.IntrospectionClient Maven / Gradle / Ivy
package com.github.jy2.introspection;
import java.net.InetAddress;
import java.net.URI;
import java.net.URISyntaxException;
import java.net.UnknownHostException;
import java.util.ArrayList;
import java.util.Collection;
import java.util.HashMap;
import java.util.HashSet;
import java.util.Map.Entry;
import java.util.function.Consumer;
import java.util.function.Predicate;
import com.github.jy2.Subscriber;
import com.github.jy2.di.JyroscopeDi;
import com.github.jy2.di.LogSeldom;
import com.github.jy2.di.annotations.Repeat;
import com.github.jy2.di.annotations.Subscribe;
import com.github.jy2.log.Jy2DiLog;
import com.github.jy2.mapper.RosTypeConverters;
import com.jyroscope.types.ConversionException;
import go.jyroscope.ros.introspection_msgs.Member;
import go.jyroscope.ros.introspection_msgs.Node;
public class IntrospectionClient {
private static final LogSeldom LOG = new Jy2DiLog(IntrospectionClient.class);
private JyroscopeDi jy2;
private HashMap members = new HashMap<>();
private Object mutex = new Object();
private static final long TIMEOUT = 2000;
private ArrayList tmp = new ArrayList<>();
public IntrospectionClient(JyroscopeDi hzDi) {
this.jy2 = hzDi;
}
@Subscribe("/introspection")
public void handleIntrospection(Member member) {
member.time = System.currentTimeMillis();
synchronized (mutex) {
members.put(member.name, member);
}
}
@Repeat(interval = 1000)
public void repeat() {
long now = System.currentTimeMillis();
synchronized (mutex) {
tmp.clear();
for (Entry m : members.entrySet()) {
if (now - m.getValue().time > TIMEOUT) {
tmp.add(m.getKey());
}
}
for (String s : tmp) {
members.remove(s);
}
}
}
public ArrayList getTopicList() {
ArrayList result = new ArrayList<>();
ArrayList> rosTopics = jy2.getMasterClient().getTopicTypes();
for (ArrayList topic : rosTopics) {
final String topicName = topic.get(0);
// final String typeName = topic.get(1);
result.add(topicName);
}
return result;
}
public Class> getTopicType(String topicName) {
ArrayList> rosTopics = jy2.getMasterClient().getTopicTypes();
for (ArrayList topic : rosTopics) {
if (topicName.equals(topic.get(0))) {
final String typeName = topic.get(1);
try {
RosTypeConverters.precompileByRosName(typeName);
} catch (ConversionException e) {
LOG.error("Cannot find java class for type: " + typeName, e);
return null;
}
Class> clazz = RosTypeConverters.getRosType(typeName);
return clazz;
}
}
return null;
}
public TopicInfo getTopicInfo(String topicName) {
Subscriber
© 2015 - 2025 Weber Informatics LLC | Privacy Policy