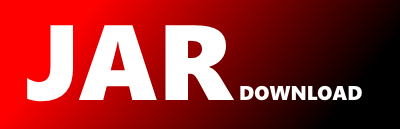
com.api.json.JSONObject Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of API4JSON Show documentation
Show all versions of API4JSON Show documentation
API's to manipulate JSON modeled after IBM's json4j including key-sorted serialization
The newest version!
/**
* (c) Copyright 2018-2023 IBM Corporation
* 1 New Orchard Road,
* Armonk, New York, 10504-1722
* United States
* +1 914 499 1900
* Nathaniel Mills wnm3@us.ibm.com
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*
*/
package com.api.json;
import java.io.IOException;
import java.io.InputStream;
import java.io.OutputStream;
import java.io.Reader;
import java.io.Writer;
import java.nio.charset.StandardCharsets;
import java.util.HashMap;
import java.util.Iterator;
import java.util.Map;
import java.util.TreeSet;
import java.util.function.BiFunction;
public class JSONObject extends HashMap
implements JSONArtifact {
private static final long serialVersionUID = -3778496643896012786L;
/**
* Determines whether the supplied object is a valid JSON value
*
* @param object
* The object to be tested for validity
* @return True if the supplied object is a valid JSON value
*/
public static boolean isValidObject(Object object) {
return JSON.isValidObject(object);
}
/**
* Determines whether the supplied class is a valid JSON type
*
* @param clazz
* The class to be tested for validity
* @return True if the supplied class is a valid JSON type
*/
public static boolean isValidType(Class clazz) {
return JSON.isValidType(clazz);
}
/**
* Parse the supplied input stream to generate a JSONObject
*
* @param is
* InputStream to be parsed
* @return JSONObject parsed from the supplied input stream
* @throws IOException
* if an error occurs reading or parsing the input stream
*/
public static JSONObject parse(InputStream is) throws IOException {
return (JSONObject) JSON.parse(is);
}
/**
* Parse the supplied reader to generate a JSONObject
*
* @param reader
* Reader to be parsed
* @return JSONObject parsed from the supplied reader
* @throws IOException
* if an error occurs reading or parsing the reader
*/
public static JSONObject parse(Reader reader) throws IOException {
return (JSONObject) JSON.parse(reader);
}
/**
* Parse the supplied input to generate a JSONObject
*
* @param input
* InputStream to be parsed
* @return JSONObject parsed from the supplied input
* @throws IOException
* if an error occurs reading or parsing the input
*/
public static JSONObject parse(String input) throws IOException {
return (JSONObject) JSON.parse(input);
}
/**
* Constructor
*/
public JSONObject() {
super();
}
/**
* Provides merger of the supplied key and value according to logic ni the
* supplied remappingFunction
*
* @param key
* The key of the object to be merged
* @param value
* The value of the object to be merged
* @param remappingFunction
* The function to control how merger is accomplished
* @return The resulting object after merger
*/
public Object merge(String key, Object value,
BiFunction