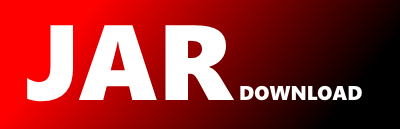
com.github.woostju.ansible.util.JsonUtil Maven / Gradle / Ivy
package com.github.woostju.ansible.util;
import com.fasterxml.jackson.annotation.JsonInclude.Include;
import com.fasterxml.jackson.core.JsonProcessingException;
import com.fasterxml.jackson.databind.DeserializationFeature;
import com.fasterxml.jackson.databind.ObjectMapper;
import com.fasterxml.jackson.databind.SerializationFeature;
import com.fasterxml.jackson.databind.node.ObjectNode;
import java.io.IOException;
import java.text.SimpleDateFormat;
import java.util.ArrayList;
import java.util.HashMap;
import java.util.List;
import java.util.Map;
import java.util.TimeZone;
public class JsonUtil {
private static final ObjectMapper objectMapper;
static {
objectMapper = new ObjectMapper();
objectMapper.configure(SerializationFeature.WRITE_DATES_AS_TIMESTAMPS, false);
objectMapper.setTimeZone(TimeZone.getTimeZone("GMT+8"));
objectMapper.configure(SerializationFeature.WRITE_NULL_MAP_VALUES, false);
objectMapper.setSerializationInclusion(Include.NON_NULL);
objectMapper.getDeserializationConfig().withoutFeatures(DeserializationFeature.FAIL_ON_UNKNOWN_PROPERTIES);
objectMapper.setDateFormat(new SimpleDateFormat("yyyy-MM-dd HH:mm:ss"));
objectMapper.configure(SerializationFeature.FAIL_ON_EMPTY_BEANS, false);
objectMapper.configure(DeserializationFeature.FAIL_ON_UNKNOWN_PROPERTIES, false);
objectMapper.configure(com.fasterxml.jackson.core.JsonParser.Feature.ALLOW_SINGLE_QUOTES, true);
}
public static ObjectMapper getObjectMapper() {
return objectMapper;
}
public static T toObject(Object entity, Class clazz) {
try {
return objectMapper.readValue(objectMapper.writeValueAsString(entity), clazz);
} catch (IOException e) {
throw new RuntimeException(e);
}
}
public static ObjectNode toObjectNode(Object entity) {
return toObject(entity, ObjectNode.class);
}
public static T toObject(String json, Class clazz) {
try {
return objectMapper.readValue(json, clazz);
} catch (IOException e) {
throw new RuntimeException(e);
}
}
public static String toJsonString(T entity) {
try {
return objectMapper.writeValueAsString(entity);
} catch (JsonProcessingException e) {
throw new RuntimeException(e);
}
}
public static List toArray(String json, Class clazz) {
try {
return objectMapper.readValue(json, objectMapper.getTypeFactory().constructCollectionType(ArrayList.class, clazz));
} catch (IOException e) {
throw new RuntimeException(e);
}
}
public static List toArray(Object object, Class clazz) {
try {
return objectMapper.readValue(toJsonString(object), objectMapper.getTypeFactory().constructCollectionType(ArrayList.class, clazz));
} catch (IOException e) {
throw new RuntimeException(e);
}
}
public static Map toHashMap(String json, Class keyClass, Class valueClass) {
try {
return objectMapper.readValue(json, objectMapper.getTypeFactory().constructMapType(HashMap.class, keyClass, valueClass));
} catch (IOException e) {
throw new RuntimeException(e);
}
}
public static Map toHashMap(Object object, Class keyClass, Class valueClass) {
try {
return objectMapper.readValue(toJsonString(object), objectMapper.getTypeFactory().constructMapType(HashMap.class, keyClass, valueClass));
} catch (IOException e) {
throw new RuntimeException(e);
}
}
public static Map, ?> bean2Map(Object bean) {
try {
return (Map, ?>) objectMapper.convertValue(bean, Map.class);
} catch (Exception ex) {
ex.printStackTrace();
}
return null;
}
public static T map2Bean(Map, ?> map, Class clazz) {
return objectMapper.convertValue(map, clazz);
}
public static boolean isJSONValid(String content) {
try {
objectMapper.readTree(content);
return true;
} catch (IOException e) {
return false;
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy