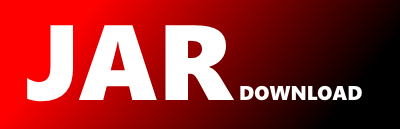
com.github.wshackle.crcl4java.motoman.ui.MotomanCrclServerJInternalFrame Maven / Gradle / Ivy
/*
* This software is public domain software, however it is preferred
* that the following disclaimers be attached.
* Software Copywrite/Warranty Disclaimer
*
* This software was developed at the National Institute of Standards and
* Technology by employees of the Federal Government in the course of their
* official duties. Pursuant to title 17 Section 105 of the United States
* Code this software is not subject to copyright protection and is in the
* public domain.
*
* This software is experimental. NIST assumes no responsibility whatsoever
* for its use by other parties, and makes no guarantees, expressed or
* implied, about its quality, reliability, or any other characteristic.
* We would appreciate acknowledgement if the software is used.
* This software can be redistributed and/or modified freely provided
* that any derivative works bear some notice that they are derived from it,
* and any modified versions bear some notice that they have been modified.
*
* See http://www.copyright.gov/title17/92chap1.html#105
*
*/
package com.github.wshackle.crcl4java.motoman.ui;
import com.github.wshackle.crcl4java.motoman.MotoPlusConnection;
import com.github.wshackle.crcl4java.motoman.MotomanCrclServer;
import crcl.utils.CRCLServerSocket;
import java.io.File;
import java.io.IOException;
import java.net.Socket;
/**
*
* @author shackle
*/
public class MotomanCrclServerJInternalFrame extends javax.swing.JInternalFrame {
/**
* Creates new form MotomanCrclServerJInternalFrame
*/
public MotomanCrclServerJInternalFrame() {
initComponents();
}
/**
* This method is called from within the constructor to initialize the form.
* WARNING: Do NOT modify this code. The content of this method is always
* regenerated by the Form Editor.
*/
@SuppressWarnings("unchecked")
// //GEN-BEGIN:initComponents
private void initComponents() {
motomanCrclServerJPanel1 = new com.github.wshackle.crcl4java.motoman.ui.MotomanCrclServerJPanel();
setIconifiable(true);
setMaximizable(true);
setResizable(true);
setTitle("Motoman CRCL Server");
javax.swing.GroupLayout layout = new javax.swing.GroupLayout(getContentPane());
getContentPane().setLayout(layout);
layout.setHorizontalGroup(
layout.createParallelGroup(javax.swing.GroupLayout.Alignment.LEADING)
.addGroup(layout.createSequentialGroup()
.addContainerGap()
.addComponent(motomanCrclServerJPanel1, javax.swing.GroupLayout.DEFAULT_SIZE, javax.swing.GroupLayout.DEFAULT_SIZE, Short.MAX_VALUE)
.addContainerGap())
);
layout.setVerticalGroup(
layout.createParallelGroup(javax.swing.GroupLayout.Alignment.LEADING)
.addGroup(layout.createSequentialGroup()
.addComponent(motomanCrclServerJPanel1, javax.swing.GroupLayout.DEFAULT_SIZE, javax.swing.GroupLayout.DEFAULT_SIZE, Short.MAX_VALUE)
.addGap(0, 0, 0))
);
pack();
}// //GEN-END:initComponents
public boolean isCrclMotoplusConnected() {
return motomanCrclServerJPanel1.isCrclMotoplusConnected();
}
public void connectCrclMotoplus() throws IOException {
this.motomanCrclServerJPanel1.connectCrclMotoplus();
}
public void disconnectCrclMotoplus() {
this.motomanCrclServerJPanel1.disconnectCrclMotoplus();
}
public File getPropertiesFile() {
return motomanCrclServerJPanel1.getPropertiesFile();
}
/**
* Set the value of propertiesFile
*
* @param propertiesFile new value of propertiesFile
*/
public void setPropertiesFile(File propertiesFile) {
motomanCrclServerJPanel1.setPropertiesFile(propertiesFile);
}
public void saveProperties() throws IOException {
motomanCrclServerJPanel1.saveProperties();
}
public void loadProperties() throws IOException {
motomanCrclServerJPanel1.loadProperties();
}
public void setCrclPort(int crclPort) {
motomanCrclServerJPanel1.setCrclPort(crclPort);
}
public int getCrclPort() {
return motomanCrclServerJPanel1.getCrclPort();
}
// Variables declaration - do not modify//GEN-BEGIN:variables
private com.github.wshackle.crcl4java.motoman.ui.MotomanCrclServerJPanel motomanCrclServerJPanel1;
// End of variables declaration//GEN-END:variables
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy