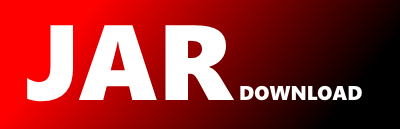
crcl.ui.PropertiesJPanel Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of crcl4java-ui Show documentation
Show all versions of crcl4java-ui Show documentation
User interfaces for CRCL Simulated Robot Server and Client Pendant.
/*
* To change this license header, choose License Headers in Project Properties.
* To change this template file, choose Tools | Templates
* and open the template in the editor.
*/
package crcl.ui;
import java.awt.Frame;
import java.util.Collections;
import java.util.HashMap;
import java.util.Map;
import java.util.Properties;
import java.util.logging.Logger;
import javax.swing.JDialog;
import javax.swing.table.DefaultTableModel;
/**
*
* @author Will Shackleford {@literal }
*/
public class PropertiesJPanel extends javax.swing.JPanel {
/**
* Creates new form PropertiesJPanel
*/
public PropertiesJPanel() {
initComponents();
}
/**
* This method is called from within the constructor to initialize the form.
* WARNING: Do NOT modify this code. The content of this method is always
* regenerated by the Form Editor.
*/
@SuppressWarnings("unchecked")
// //GEN-BEGIN:initComponents
private void initComponents() {
jScrollPane1 = new javax.swing.JScrollPane();
jTable1 = new javax.swing.JTable();
jButtonCancel = new javax.swing.JButton();
jButtonOk = new javax.swing.JButton();
jTable1.setModel(new javax.swing.table.DefaultTableModel(
new Object [][] {
},
new String [] {
"Property Name", "Value"
}
) {
Class[] types = new Class [] {
java.lang.String.class, java.lang.Object.class
};
boolean[] canEdit = new boolean [] {
false, true
};
public Class getColumnClass(int columnIndex) {
return types [columnIndex];
}
public boolean isCellEditable(int rowIndex, int columnIndex) {
return canEdit [columnIndex];
}
});
jScrollPane1.setViewportView(jTable1);
jButtonCancel.setText("Cancel");
jButtonCancel.addActionListener(new java.awt.event.ActionListener() {
public void actionPerformed(java.awt.event.ActionEvent evt) {
jButtonCancelActionPerformed(evt);
}
});
jButtonOk.setText("OK");
jButtonOk.addActionListener(new java.awt.event.ActionListener() {
public void actionPerformed(java.awt.event.ActionEvent evt) {
jButtonOkActionPerformed(evt);
}
});
javax.swing.GroupLayout layout = new javax.swing.GroupLayout(this);
this.setLayout(layout);
layout.setHorizontalGroup(
layout.createParallelGroup(javax.swing.GroupLayout.Alignment.LEADING)
.addGroup(layout.createSequentialGroup()
.addContainerGap()
.addGroup(layout.createParallelGroup(javax.swing.GroupLayout.Alignment.LEADING)
.addComponent(jScrollPane1, javax.swing.GroupLayout.DEFAULT_SIZE, 375, Short.MAX_VALUE)
.addGroup(javax.swing.GroupLayout.Alignment.TRAILING, layout.createSequentialGroup()
.addGap(0, 0, Short.MAX_VALUE)
.addComponent(jButtonOk)
.addPreferredGap(javax.swing.LayoutStyle.ComponentPlacement.RELATED)
.addComponent(jButtonCancel)))
.addContainerGap())
);
layout.setVerticalGroup(
layout.createParallelGroup(javax.swing.GroupLayout.Alignment.LEADING)
.addGroup(layout.createSequentialGroup()
.addContainerGap()
.addComponent(jScrollPane1, javax.swing.GroupLayout.DEFAULT_SIZE, 282, Short.MAX_VALUE)
.addPreferredGap(javax.swing.LayoutStyle.ComponentPlacement.RELATED)
.addGroup(layout.createParallelGroup(javax.swing.GroupLayout.Alignment.BASELINE)
.addComponent(jButtonCancel)
.addComponent(jButtonOk))
.addContainerGap())
);
}// //GEN-END:initComponents
private void jButtonOkActionPerformed(java.awt.event.ActionEvent evt) {//GEN-FIRST:event_jButtonOkActionPerformed
Map newMap = new HashMap<>();
for(int i= 0; i < this.jTable1.getRowCount(); i++) {
newMap.put(this.jTable1.getValueAt(i, 0).toString(),
this.jTable1.getValueAt(i, 1).toString());
}
this.setMap(newMap);
this.cancelled = false;
if(null != dialog && dialog.isVisible()) {
dialog.setVisible(false);
}
if(null != this.getParent() && this.getParent().isVisible()) {
this.getParent().setVisible(false);
}
}//GEN-LAST:event_jButtonOkActionPerformed
private void jButtonCancelActionPerformed(java.awt.event.ActionEvent evt) {//GEN-FIRST:event_jButtonCancelActionPerformed
this.cancelled = true;
if(null != dialog && dialog.isVisible()) {
dialog.setVisible(false);
}
if(null != this.getParent() && this.getParent().isVisible()) {
this.getParent().setVisible(false);
}
}//GEN-LAST:event_jButtonCancelActionPerformed
private boolean cancelled = false;
private Map map;
/**
* Get the value of map
*
* @return the value of map
*/
public Map getMap() {
return Collections.unmodifiableMap(map);
}
/**
* Set the value of map
*
* @param map new value of map
*/
public void setMap(Map map) {
this.map = map;
Object tableData[][] = new Object[map.size()][];
int i = 0;
for(Map.Entry e : map.entrySet()) {
tableData[i] = new Object[]{e.getKey(),e.getValue()};
i++;
}
this.jTable1.setModel(
new DefaultTableModel(tableData,
new String[]{"Property Name", "value"})
);
}
private JDialog dialog = null;
public static Properties confirmProperties(Frame _owner, String _title, boolean _modal, Properties props) {
Map map = new HashMap<>();
for(String name : props.stringPropertyNames()) {
map.put(name, props.getProperty(name));
}
Map newMap = confirmPropertiesMap(_owner, _title, _modal, map);
if(null == newMap) {
return null;
}
Properties newProps = new Properties();
for(Map.Entry e : newMap.entrySet()) {
newProps.put(e.getKey(), e.getValue());
}
return newProps;
}
public static Map confirmPropertiesMap(Frame _owner, String _title,boolean _modal, Map _map) {
JDialog dialog = new JDialog(_owner, _title, _modal);
PropertiesJPanel panel = new PropertiesJPanel();
panel.setMap(_map);
dialog.add(panel);
panel.dialog = dialog;
dialog.pack();
dialog.setVisible(true);
if (panel.cancelled) {
return null;
}
return panel.getMap();
}
// Variables declaration - do not modify//GEN-BEGIN:variables
private javax.swing.JButton jButtonCancel;
private javax.swing.JButton jButtonOk;
private javax.swing.JScrollPane jScrollPane1;
private javax.swing.JTable jTable1;
// End of variables declaration//GEN-END:variables
private static final Logger LOG = Logger.getLogger(PropertiesJPanel.class.getName());
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy