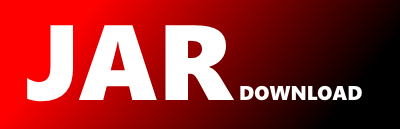
com.github.wshackle.fanuc.robotneighborhood.IRNRobot Maven / Gradle / Ivy
package com.github.wshackle.fanuc.robotneighborhood ;
import com4j.*;
/**
* Provides access to a specific robot in the Robot Neighborhood
*/
@IID("{AD89CAA8-F912-4638-954A-3EDDE19DC8E5}")
public interface IRNRobot extends Com4jObject {
// Methods:
/**
*
* Returns/Sets the name of this robot.
*
*
* Getter method for the COM property "Name"
*
* @return Returns a value of type java.lang.String
*/
@DISPID(1) //= 0x1. The runtime will prefer the VTID if present
@VTID(7)
java.lang.String name();
/**
*
* Returns/Sets the name of this robot.
*
*
* Setter method for the COM property "Name"
*
* @param name Mandatory java.lang.String parameter.
*/
@DISPID(1) //= 0x1. The runtime will prefer the VTID if present
@VTID(8)
void name(
java.lang.String name);
/**
*
* Returns the type of the robot ? Real or Virtual.
*
*
* Getter method for the COM property "Type"
*
* @return Returns a value of type com.github.wshackle.fanuc.robotneighborhood.FRERNRobotTypeConstants
*/
@DISPID(2) //= 0x2. The runtime will prefer the VTID if present
@VTID(9)
com.github.wshackle.fanuc.robotneighborhood.FRERNRobotTypeConstants type();
/**
*
* Returns the FRCRNRobots object that holds this FRCRNRobot object.
*
*
* Getter method for the COM property "Parent"
*
* @return Returns a value of type com.github.wshackle.fanuc.robotneighborhood.IRNRobots
*/
@DISPID(3) //= 0x3. The runtime will prefer the VTID if present
@VTID(10)
com.github.wshackle.fanuc.robotneighborhood.IRNRobots parent();
/**
*
* Returns the fully decorated name of this robot.
*
*
* Getter method for the COM property "PathName"
*
* @return Returns a value of type java.lang.String
*/
@DISPID(4) //= 0x4. The runtime will prefer the VTID if present
@VTID(11)
java.lang.String pathName();
/**
*
* Returns/sets the enable status of the AutoReconnect feature.
*
*
* Getter method for the COM property "AutoReconnectEnable"
*
* @return Returns a value of type boolean
*/
@DISPID(5) //= 0x5. The runtime will prefer the VTID if present
@VTID(12)
boolean autoReconnectEnable();
/**
*
* Returns/sets the enable status of the AutoReconnect feature.
*
*
* Setter method for the COM property "AutoReconnectEnable"
*
* @param autoReconnectEnable Mandatory boolean parameter.
*/
@DISPID(5) //= 0x5. The runtime will prefer the VTID if present
@VTID(13)
void autoReconnectEnable(
boolean autoReconnectEnable);
/**
*
* Returns/sets the number of reconnection attempts that the AutoReconnect feature will try when activated.
*
*
* Getter method for the COM property "AutoReconnectNumRetries"
*
* @return Returns a value of type int
*/
@DISPID(6) //= 0x6. The runtime will prefer the VTID if present
@VTID(14)
int autoReconnectNumRetries();
/**
*
* Returns/sets the number of reconnection attempts that the AutoReconnect feature will try when activated.
*
*
* Setter method for the COM property "AutoReconnectNumRetries"
*
* @param autoReconnectNumRetries Mandatory int parameter.
*/
@DISPID(6) //= 0x6. The runtime will prefer the VTID if present
@VTID(15)
void autoReconnectNumRetries(
int autoReconnectNumRetries);
/**
*
* Returns/sets the frequency at which the AutoReconnect feature will attempt to reconnect.
*
*
* Getter method for the COM property "AutoReconnectPeriod"
*
* @return Returns a value of type int
*/
@DISPID(7) //= 0x7. The runtime will prefer the VTID if present
@VTID(16)
int autoReconnectPeriod();
/**
*
* Returns/sets the frequency at which the AutoReconnect feature will attempt to reconnect.
*
*
* Setter method for the COM property "AutoReconnectPeriod"
*
* @param autoReconnectPeriod Mandatory int parameter.
*/
@DISPID(7) //= 0x7. The runtime will prefer the VTID if present
@VTID(17)
void autoReconnectPeriod(
int autoReconnectPeriod);
/**
*
* Returns the last know status of the connection to the robot.
*
*
* Getter method for the COM property "ConnectionStatus"
*
* @return Returns a value of type com.github.wshackle.fanuc.robotneighborhood.FRERNConnectionStatusConstants
*/
@DISPID(8) //= 0x8. The runtime will prefer the VTID if present
@VTID(18)
com.github.wshackle.fanuc.robotneighborhood.FRERNConnectionStatusConstants connectionStatus();
/**
*
* Returns/sets the enable status of the Heartbeat feature.
*
*
* Getter method for the COM property "HeartbeatEnable"
*
* @return Returns a value of type boolean
*/
@DISPID(9) //= 0x9. The runtime will prefer the VTID if present
@VTID(19)
boolean heartbeatEnable();
/**
*
* Returns/sets the enable status of the Heartbeat feature.
*
*
* Setter method for the COM property "HeartbeatEnable"
*
* @param heartbeatEnable Mandatory boolean parameter.
*/
@DISPID(9) //= 0x9. The runtime will prefer the VTID if present
@VTID(20)
void heartbeatEnable(
boolean heartbeatEnable);
/**
*
* Returns/sets the frequency for which the monitoring of the physical connection to a robot is executed.
*
*
* Getter method for the COM property "HeartbeatPeriod"
*
* @return Returns a value of type int
*/
@DISPID(10) //= 0xa. The runtime will prefer the VTID if present
@VTID(21)
int heartbeatPeriod();
/**
*
* Returns/sets the frequency for which the monitoring of the physical connection to a robot is executed.
*
*
* Setter method for the COM property "HeartbeatPeriod"
*
* @param heartbeatPeriod Mandatory int parameter.
*/
@DISPID(10) //= 0xa. The runtime will prefer the VTID if present
@VTID(22)
void heartbeatPeriod(
int heartbeatPeriod);
/**
*
* Returns/sets the enable status of the KeepAlive feature.
*
*
* Getter method for the COM property "KeepAliveEnable"
*
* @return Returns a value of type boolean
*/
@DISPID(11) //= 0xb. The runtime will prefer the VTID if present
@VTID(23)
boolean keepAliveEnable();
/**
*
* Returns/sets the enable status of the KeepAlive feature.
*
*
* Setter method for the COM property "KeepAliveEnable"
*
* @param keepAliveEnable Mandatory boolean parameter.
*/
@DISPID(11) //= 0xb. The runtime will prefer the VTID if present
@VTID(24)
void keepAliveEnable(
boolean keepAliveEnable);
/**
*
* Returns/sets the amount of time that the Robot Neighborhood will keep the Robot Object active after all connections are released.
*
*
* Getter method for the COM property "KeepAliveDuration"
*
* @return Returns a value of type int
*/
@DISPID(12) //= 0xc. The runtime will prefer the VTID if present
@VTID(25)
int keepAliveDuration();
/**
*
* Returns/sets the amount of time that the Robot Neighborhood will keep the Robot Object active after all connections are released.
*
*
* Setter method for the COM property "KeepAliveDuration"
*
* @param keepAliveDuration Mandatory int parameter.
*/
@DISPID(12) //= 0xc. The runtime will prefer the VTID if present
@VTID(26)
void keepAliveDuration(
int keepAliveDuration);
/**
*
* Returns IP Address of the RJ-3 controller that the connection represents.
*
*
* Getter method for the COM property "IPAddress"
*
* @return Returns a value of type java.lang.String
*/
@DISPID(13) //= 0xd. The runtime will prefer the VTID if present
@VTID(27)
java.lang.String ipAddress();
/**
*
* This property will return a collection of communication services that the robot supports.
*
*
* Getter method for the COM property "Services"
*
* @return Returns a value of type com.github.wshackle.fanuc.robotneighborhood.IRNServices
*/
@DISPID(14) //= 0xe. The runtime will prefer the VTID if present
@VTID(28)
com.github.wshackle.fanuc.robotneighborhood.IRNServices services();
/**
*
* Returns a Robot Server object.
*
*
* Getter method for the COM property "RobotServer"
*
* @return Returns a value of type com4j.Com4jObject
*/
@DISPID(15) //= 0xf. The runtime will prefer the VTID if present
@VTID(29)
@ReturnValue(type=NativeType.Dispatch)
com4j.Com4jObject robotServer();
/**
*
* Copies the description of this robot to the Windows clipboard.
*
*/
@DISPID(17) //= 0x11. The runtime will prefer the VTID if present
@VTID(30)
void copy();
// Properties:
}