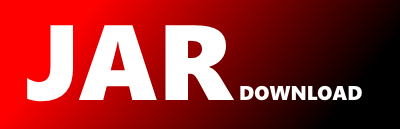
com.github.wshackle.fanuc.robotserver.FREAlarmSeverityConstants Maven / Gradle / Ivy
package com.github.wshackle.fanuc.robotserver ;
import com4j.*;
/**
*
* An enumeration of constants used for Alarms.
*
*/
public enum FREAlarmSeverityConstants implements ComEnum {
/**
*
* Execution mask
*
*
* The value of this constant is 3
*
*/
frSevExMask(3),
/**
*
* Execution: no effect
*
*
* The value of this constant is 0
*
*/
frSevExNone(0),
/**
*
* Execution: debug
*
*
* The value of this constant is 1
*
*/
frSevExDebug(1),
/**
*
* Execution: pause
*
*
* The value of this constant is 2
*
*/
frSevExPause(2),
/**
*
* Execution: abort
*
*
* The value of this constant is 3
*
*/
frSevExAbort(3),
/**
*
* Motion mask
*
*
* The value of this constant is 12
*
*/
frSevMoMask(12),
/**
*
* Motion: no effect
*
*
* The value of this constant is 0
*
*/
frSevMoNone(0),
/**
*
* Motion: stop
*
*
* The value of this constant is 4
*
*/
frSevMoStop(4),
/**
*
* Motion: cancel
*
*
* The value of this constant is 8
*
*/
frSevMoCancel(8),
/**
*
* Severity: none
*
*
* The value of this constant is 128
*
*/
frSevNone(128),
/**
*
* Severity: warning
*
*
* The value of this constant is 0
*
*/
frSevWarn(0),
/**
*
* Severity: pause
*
*
* The value of this constant is 34
*
*/
frSevPause(34),
/**
*
* Severity: local pause
*
*
* The value of this constant is 2
*
*/
frSevPauseL(2),
/**
*
* Severity: stop
*
*
* The value of this constant is 38
*
*/
frSevStop(38),
/**
*
* Severity: local stop
*
*
* The value of this constant is 6
*
*/
frSevStopL(6),
/**
*
* Severity: servo
*
*
* The value of this constant is 54
*
*/
frSevServo(54),
/**
*
* Severity: servo 2
*
*
* The value of this constant is 59
*
*/
frSevServo2(59),
/**
*
* Severity: abort
*
*
* The value of this constant is 43
*
*/
frSevAbort(43),
/**
*
* Severity: local abort
*
*
* The value of this constant is 11
*
*/
frSevAbortL(11),
/**
*
* Severity: system
*
*
* The value of this constant is 123
*
*/
frSevSystem(123),
/**
*
* Error class: normal
*
*
* The value of this constant is 0
*
*/
frErrorNormal(0),
/**
*
* Error class: reset
*
*
* The value of this constant is 1
*
*/
frErrorReset(1),
/**
*
* Error class: clear
*
*
* The value of this constant is 2
*
*/
frErrorClear(2),
/**
*
* Error class: clear all
*
*
* The value of this constant is 3
*
*/
frErrorClearAll(3),
;
private final int value;
FREAlarmSeverityConstants(int value) { this.value=value; }
public int comEnumValue() { return value; }
}