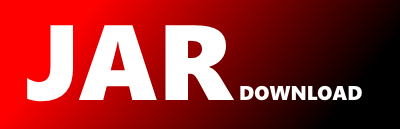
com.github.wshackle.fanuc.robotserver.FRELMCodeConstants Maven / Gradle / Ivy
package com.github.wshackle.fanuc.robotserver ;
import com4j.*;
/**
*
* Constants for LM Codes.
*
*/
public enum FRELMCodeConstants implements ComEnum {
/**
*
* NONE
*
*
* The value of this constant is 0
*
*/
LM_NONE_C(0),
/**
*
* RESERVED DO NOT CHANGE
*
*
* The value of this constant is 0
*
*/
LM_SAMPLE_C(0),
/**
*
* no item
*
*
* The value of this constant is 0
*
*/
LM_NOITM_C(0),
/**
*
* constant
*
*
* The value of this constant is 1
*
*/
LM_CONST_C(1),
/**
*
* index
*
*
* The value of this constant is 2
*
*/
LM_INDEX_C(2),
/**
*
* Register
*
*
* The value of this constant is 3
*
*/
LM_REG_C(3),
/**
*
* Position Register
*
*
* The value of this constant is 4
*
*/
LM_PREG_C(4),
/**
*
* Position Item
*
*
* The value of this constant is 5
*
*/
LM_POSITEM_C(5),
/**
*
* Pallet Register
*
*
* The value of this constant is 6
*
*/
LM_PLREG_C(6),
/**
*
* Pallet Element
*
*
* The value of this constant is 7
*
*/
LM_PLELM_C(7),
/**
*
* SDI
*
*
* The value of this constant is 10
*
*/
LM_SDI_C(10),
/**
*
* RDI
*
*
* The value of this constant is 11
*
*/
LM_RDI_C(11),
/**
*
* AIN
*
*
* The value of this constant is 12
*
*/
LM_AIN_C(12),
/**
*
* WI
*
*
* The value of this constant is 13
*
*/
LM_WI_C(13),
/**
*
* SDO
*
*
* The value of this constant is 15
*
*/
LM_SDO_C(15),
/**
*
* RDO
*
*
* The value of this constant is 16
*
*/
LM_RDO_C(16),
/**
*
* AOUT
*
*
* The value of this constant is 17
*
*/
LM_AOUT_C(17),
/**
*
* WO
*
*
* The value of this constant is 18
*
*/
LM_WO_C(18),
/**
*
* RSR
*
*
* The value of this constant is 20
*
*/
LM_RSR_C(20),
/**
*
* UALM
*
*
* The value of this constant is 21
*
*/
LM_UALM_C(21),
/**
*
* CODE (input)
*
*
* The value of this constant is 22
*
*/
LM_CODEI_C(22),
/**
*
* CODE (output)
*
*
* The value of this constant is 23
*
*/
LM_CODEO_C(23),
/**
*
* OVERRIDE
*
*
* The value of this constant is 24
*
*/
LM_OVRD_C(24),
/**
*
* TIMER
*
*
* The value of this constant is 25
*
*/
LM_TIMER_C(25),
/**
*
* parameter
*
*
* The value of this constant is 26
*
*/
LM_PARAM_C(26),
/**
*
* error program
*
*
* The value of this constant is 27
*
*/
LM_ERPRG_C(27),
/**
*
* resume program
*
*
* The value of this constant is 28
*
*/
LM_RSMPRG_C(28),
/**
*
* semaphore
*
*
* The value of this constant is 29
*
*/
LM_SEMPH_C(29),
/**
*
* comment
*
*
* The value of this constant is 30
*
*/
LM_REM_C(30),
/**
*
* MESSAGE
*
*
* The value of this constant is 31
*
*/
LM_MSG_C(31),
/**
*
* user frame selection
*
*
* The value of this constant is 32
*
*/
LM_UFRNUM_C(32),
/**
*
* user frame
*
*
* The value of this constant is 33
*
*/
LM_UFRAME_C(33),
/**
*
* user tool selection
*
*
* The value of this constant is 34
*
*/
LM_UTLNUM_C(34),
/**
*
* user tool frame
*
*
* The value of this constant is 35
*
*/
LM_UTOOL_C(35),
/**
*
* SI
*
*
* The value of this constant is 36
*
*/
LM_SI_C(36),
/**
*
* UI
*
*
* The value of this constant is 37
*
*/
LM_UI_C(37),
/**
*
* SO
*
*
* The value of this constant is 38
*
*/
LM_SO_C(38),
/**
*
* UO
*
*
* The value of this constant is 39
*
*/
LM_UO_C(39),
/**
*
* Error number
*
*
* The value of this constant is 40
*
*/
LM_ERNUM_C(40),
/**
*
* special constant
*
*
* The value of this constant is 50
*
*/
LM_SPCON_C(50),
/**
*
* pulse constant
*
*
* The value of this constant is 51
*
*/
LM_PULSE_C(51),
/**
*
* lock pos register
*
*
* The value of this constant is 52
*
*/
LM_LKPREG_C(52),
/**
*
* unlock pos register
*
*
* The value of this constant is 53
*
*/
LM_ULPREG_C(53),
/**
*
* Clear resume program
*
*
* The value of this constant is 54
*
*/
LM_CLRSMPRG_C(54),
/**
*
* Return path disable
*
*
* The value of this constant is 55
*
*/
LM_ORGDSBL_C(55),
/**
*
* Pressure
*
*
* The value of this constant is 56
*
*/
LM_PRESSURE_C(56),
/**
*
* Timer overflow
*
*
* The value of this constant is 57
*
*/
LM_TIMOVR_C(57),
/**
*
* Max speed
*
*
* The value of this constant is 58
*
*/
LM_MAXSPD_C(58),
/**
*
* Data monitor
*
*
* The value of this constant is 59
*
*/
LM_DMON_C(59),
/**
*
* Maint. program
*
*
* The value of this constant is 60
*
*/
LM_MNTPRG_C(60),
/**
*
* Call argument
*
*
* The value of this constant is 61
*
*/
LM_ARG_C(61),
/**
*
* Argument: Register
*
*
* The value of this constant is 62
*
*/
LM_ARGREG_C(62),
/**
*
* Argument: String
*
*
* The value of this constant is 63
*
*/
LM_ARGSTR_C(63),
/**
*
* Argument terminator
*
*
* The value of this constant is 64
*
*/
LM_ARGTERM_C(64),
/**
*
* Serrvo gun general
*
*
* The value of this constant is 65
*
*/
LM_SVGGEN_C(65),
/**
*
* TCP Speed Output
*
*
* The value of this constant is 66
*
*/
LM_TCPSPD_C(66),
/**
*
* = (assgin)
*
*
* The value of this constant is 100
*
*/
LM_ASGN_C(100),
/**
*
* +
*
*
* The value of this constant is 101
*
*/
LM_PLUS_C(101),
/**
*
* -
*
*
* The value of this constant is 102
*
*/
LM_MINUS_C(102),
/**
*
* *
*
*
* The value of this constant is 103
*
*/
LM_MULT_C(103),
/**
*
* /
*
*
* The value of this constant is 104
*
*/
LM_DIV_C(104),
/**
*
* DIV
*
*
* The value of this constant is 105
*
*/
LM_DIVI_C(105),
/**
*
* MOD
*
*
* The value of this constant is 106
*
*/
LM_MOD_C(106),
/**
*
* = (compare)
*
*
* The value of this constant is 107
*
*/
LM_EQ_C(107),
/**
*
* <>
*
*
* The value of this constant is 108
*
*/
LM_NE_C(108),
/**
*
* <
*
*
* The value of this constant is 109
*
*/
LM_LT_C(109),
/**
*
* <=
*
*
* The value of this constant is 110
*
*/
LM_LE_C(110),
/**
*
* >
*
*
* The value of this constant is 111
*
*/
LM_GT_C(111),
/**
*
* >=
*
*
* The value of this constant is 112
*
*/
LM_GE_C(112),
/**
*
* AND
*
*
* The value of this constant is 113
*
*/
LM_AND_C(113),
/**
*
* OR
*
*
* The value of this constant is 114
*
*/
LM_OR_C(114),
/**
*
* (
*
*
* The value of this constant is 115
*
*/
LM_L_PAREN_C(115),
/**
*
* )
*
*
* The value of this constant is 116
*
*/
LM_R_PAREN_C(116),
/**
*
* !
*
*
* The value of this constant is 117
*
*/
LM_NOT_C(117),
/**
*
* IF
*
*
* The value of this constant is 120
*
*/
LM_IF_C(120),
/**
*
* SELECT
*
*
* The value of this constant is 121
*
*/
LM_SEL_C(121),
/**
*
* OTHERWISE
*
*
* The value of this constant is 122
*
*/
LM_OTHWS_C(122),
/**
*
* WAIT (delay)
*
*
* The value of this constant is 123
*
*/
LM_DELAY_C(123),
/**
*
* WAIT (condition)
*
*
* The value of this constant is 124
*
*/
LM_WAIT_C(124),
/**
*
* TIMEOUT
*
*
* The value of this constant is 125
*
*/
LM_TMOUT_C(125),
/**
*
* JMP
*
*
* The value of this constant is 126
*
*/
LM_JMP_C(126),
/**
*
* LBL (specifier)
*
*
* The value of this constant is 127
*
*/
LM_JMPDS_C(127),
/**
*
* LBL (definition)
*
*
* The value of this constant is 128
*
*/
LM_LBL_C(128),
/**
*
* CALL
*
*
* The value of this constant is 129
*
*/
LM_CALL_C(129),
/**
*
* program name
*
*
* The value of this constant is 130
*
*/
LM_PGNAM_C(130),
/**
*
* PAUSE
*
*
* The value of this constant is 131
*
*/
LM_PAUSE_C(131),
/**
*
* ABORT
*
*
* The value of this constant is 132
*
*/
LM_ABORT_C(132),
/**
*
* END
*
*
* The value of this constant is 133
*
*/
LM_END_C(133),
/**
*
* RUN
*
*
* The value of this constant is 134
*
*/
LM_RUN_C(134),
/**
*
* MACRO
*
*
* The value of this constant is 135
*
*/
LM_MACRO_C(135),
/**
*
* VEIN ON/OFF
*
*
* The value of this constant is 136
*
*/
LM_VEIN_C(136),
/**
*
* FIGURE CUTTING
*
*
* The value of this constant is 137
*
*/
LM_FC_C(137),
/**
*
* HIGHT SENSOR
*
*
* The value of this constant is 138
*
*/
LM_HTSNS_C(138),
/**
*
* OCP START/END
*
*
* The value of this constant is 139
*
*/
LM_OCP_C(139),
/**
*
* SEND
*
*
* The value of this constant is 140
*
*/
LM_SEND_C(140),
/**
*
* RECEIVE
*
*
* The value of this constant is 141
*
*/
LM_RCV_C(141),
/**
*
* CALMATRIX
*
*
* The value of this constant is 142
*
*/
LM_CALM_C(142),
/**
*
* SENSOR_ON[...]
*
*
* The value of this constant is 143
*
*/
LM_SNSON_C(143),
/**
*
* SENSOR_OFF
*
*
* The value of this constant is 144
*
*/
LM_SNSOF_C(144),
/**
*
* SEARCH_MIGEYE[...]
*
*
* The value of this constant is 145
*
*/
LM_SMIGE_C(145),
/**
*
* TORQUE LIMIT ... %
*
*
* The value of this constant is 146
*
*/
LM_TORQ_C(146),
/**
*
* MONITOR/END MONITOR
*
*
* The value of this constant is 147
*
*/
LM_MONITOR_C(147),
/**
*
* WHEN
*
*
* The value of this constant is 148
*
*/
LM_WHEN_C(148),
/**
*
* PAYLOAD SETTING
*
*
* The value of this constant is 149
*
*/
LM_PLST_C(149),
/**
*
* process logic
*
*
* The value of this constant is 150
*
*/
LM_APPL_C(150),
/**
*
* process logic
*
*
* The value of this constant is 151
*
*/
LM_APPL1_C(151),
/**
*
* process logic
*
*
* The value of this constant is 152
*
*/
LM_APPL2_C(152),
/**
*
* MIG EYE
*
*
* The value of this constant is 160
*
*/
LM_MIG_C(160),
/**
*
* V-400i vision mnemonic
*
*
* The value of this constant is 161
*
*/
LM_VISION_C(161),
/**
*
* IM = x vision mnemonic
*
*
* The value of this constant is 162
*
*/
LM_VIS_IM_C(162),
/**
*
* ST = x vision mnemonic
*
*
* The value of this constant is 163
*
*/
LM_VIS_ST_C(163),
/**
*
* ID = x vision mnemonic
*
*
* The value of this constant is 164
*
*/
LM_VIS_ID_C(164),
/**
*
* FL = x vision mnemonic
*
*
* The value of this constant is 165
*
*/
LM_VIS_FL_C(165),
/**
*
* RS = x vision mnemonic
*
*
* The value of this constant is 166
*
*/
LM_VIS_RS_C(166),
/**
*
* OF = x vision mnemonic
*
*
* The value of this constant is 167
*
*/
LM_VIS_OF_C(167),
/**
*
* QN = x vision mnemonic
*
*
* The value of this constant is 168
*
*/
LM_VIS_QN_C(168),
/**
*
* VIEW[...] vision mnemonic
*
*
* The value of this constant is 169
*
*/
LM_VIS_VW_C(169),
/**
*
* Compliance control
*
*
* The value of this constant is 170
*
*/
LM_CC_C(170),
/**
*
* ASCII INTERFACE
*
*
* The value of this constant is 171
*
*/
LM_IBGNSTRT_C(171),
/**
*
* ASCII INTERFACE
*
*
* The value of this constant is 172
*
*/
LM_IBGNEND_C(172),
/**
*
* ASCII INTERFACE
*
*
* The value of this constant is 173
*
*/
LM_IBGNREC_C(173),
/**
*
* ASCII INTERFACE
*
*
* The value of this constant is 174
*
*/
LM_IBGNRECE_C(174),
/**
*
* Servo gun change
*
*
* The value of this constant is 175
*
*/
LM_SVGC_C(175),
/**
*
* Servo Hand change
*
*
* The value of this constant is 176
*
*/
LM_SVHC_C(176),
/**
*
* Servo Tool change
*
*
* The value of this constant is 176
*
*/
LM_SVTC_C(176),
/**
*
* InterBus-S change
*
*
* The value of this constant is 177
*
*/
LM_IBSC_C(177),
/**
*
* InterBus-S change
*
*
* The value of this constant is 178
*
*/
LM_GRPNAME_C(178),
/**
*
* InterBus PCI segment switching
*
*
* The value of this constant is 179
*
*/
LM_IBPX_C(179),
/**
*
* No application manager
*
*
* The value of this constant is 180
*
*/
LM_NOAMR_APPL_C(180),
/**
*
* 3D vision
*
*
* The value of this constant is 190
*
*/
LM_IV_C(190),
/**
*
* SC = *: 3DV, Schedule Number
*
*
* The value of this constant is 191
*
*/
LM_IV_SC_C(191),
/**
*
* PS = *: 3DV, Schedule Number
*
*
* The value of this constant is 192
*
*/
LM_IV_PS_C(192),
/**
*
* 2D vision
*
*
* The value of this constant is 193
*
*/
LM_PCVIS_C(193),
/**
*
* ST = x, 2D vision status reg num
*
*
* The value of this constant is 194
*
*/
LM_PCVIS_ST_C(194),
/**
*
* OF = x, 2D vision offset reg num
*
*
* The value of this constant is 195
*
*/
LM_PCVIS_OF_C(195),
/**
*
* 2D vision sensor integrated type
*
*
* The value of this constant is 196
*
*/
LM_SIVIS__C(196),
/**
*
* ST = x, 2D vision offset reg num
*
*
* The value of this constant is 197
*
*/
LM_SIVIS_ST_C(197),
/**
*
* OF = x, 2D vision offset reg num
*
*
* The value of this constant is 198
*
*/
LM_SIVIS_OF_C(198),
/**
*
* Wrist joint
*
*
* The value of this constant is 200
*
*/
LM_WJNT_C(200),
/**
*
* Coordinated motion
*
*
* The value of this constant is 201
*
*/
LM_COORD_C(201),
/**
*
* SKIP
*
*
* The value of this constant is 202
*
*/
LM_SKIP_C(202),
/**
*
* SKIP CONDITION
*
*
* The value of this constant is 203
*
*/
LM_SKPCND_C(203),
/**
*
* QUICK SKIP
*
*
* The value of this constant is 204
*
*/
LM_QSKP_C(204),
/**
*
* OFFSET
*
*
* The value of this constant is 205
*
*/
LM_OFST_C(205),
/**
*
* OFFSET for via point
*
*
* The value of this constant is 206
*
*/
LM_VIAOFS_C(206),
/**
*
* OFFSET CONDITION
*
*
* The value of this constant is 207
*
*/
LM_OFSCND_C(207),
/**
*
* SOFT FLOAT
*
*
* The value of this constant is 208
*
*/
LM_SFLT_C(208),
/**
*
* STITCH
*
*
* The value of this constant is 209
*
*/
LM_STITCH_C(209),
/**
*
* Incremental motion
*
*
* The value of this constant is 210
*
*/
LM_INC_C(210),
/**
*
* Acceleation override
*
*
* The value of this constant is 211
*
*/
LM_ACC_C(211),
/**
*
* TOUCH SENSOR
*
*
* The value of this constant is 212
*
*/
LM_TOUCH_C(212),
/**
*
* CORNER_TOL
*
*
* The value of this constant is 213
*
*/
LM_CT_C(213),
/**
*
* Cell Finder
*
*
* The value of this constant is 214
*
*/
LM_CELL_C(214),
/**
*
* WS
*
*
* The value of this constant is 215
*
*/
LM_WS_C(215),
/**
*
* WE (Obsolete)
*
*
* The value of this constant is 216
*
*/
LM_WE_C(216),
/**
*
* TRACK
*
*
* The value of this constant is 216
*
*/
LM_TRACK_C(216),
/**
*
* Motion Cycle Time
*
*
* The value of this constant is 217
*
*/
LM_MCT_C(217),
/**
*
* Line Tracking
*
*
* The value of this constant is 218
*
*/
LM_LNT_C(218),
/**
*
* TOOL OFFSET
*
*
* The value of this constant is 219
*
*/
LM_TOFST_C(219),
/**
*
* TOOL OFFSET for via point
*
*
* The value of this constant is 220
*
*/
LM_TVIAOFS_C(220),
/**
*
* TOOL OFFSET CONDITION
*
*
* The value of this constant is 221
*
*/
LM_TOFSCND_C(221),
/**
*
* Continuous turn
*
*
* The value of this constant is 222
*
*/
LM_CN_C(222),
/**
*
* STICK DETECTION
*
*
* The value of this constant is 223
*
*/
LM_STCK_C(223),
/**
*
* High Sensitive Collision Detection
*
*
* The value of this constant is 224
*
*/
LM_HSCD_C(224),
/**
*
* LOAD CLUTCH
*
*
* The value of this constant is 225
*
*/
LM_LDCL_C(225),
/**
*
* Short Cut Path
*
*
* The value of this constant is 226
*
*/
LM_SCP_C(226),
/**
*
* Simple palletizing
*
*
* The value of this constant is 227
*
*/
LM_PAL2_C(227),
/**
*
* Servo Gun
*
*
* The value of this constant is 228
*
*/
LM_SERVOGUN_C(228),
/**
*
* Servo gun pressure
*
*
* The value of this constant is 229
*
*/
LM_SVGPRES_C(229),
/**
*
* Orientation Fixed
*
*
* The value of this constant is 230
*
*/
LM_OFIX_C(230),
/**
*
* Approach_STOP/WAIT
*
*
* The value of this constant is 231
*
*/
LM_IAINST_C(231),
/**
*
* For frl iwc(dg)
*
*
* The value of this constant is 232
*
*/
LM_IWC_C(232),
/**
*
* Start Linear Distance
*
*
* The value of this constant is 233
*
*/
LM_LD_STRT_C(233),
/**
*
* End Linear Distance
*
*
* The value of this constant is 234
*
*/
LM_LD_END_C(234),
/**
*
* Motion Squeeze
*
*
* The value of this constant is 235
*
*/
LM_MSQZ_C(235),
/**
*
* Path Optimization
*
*
* The value of this constant is 236
*
*/
LM_OPTP_C(236),
/**
*
* PSPD
*
*
* The value of this constant is 237
*
*/
LM_CPPSPD_C(237),
/**
*
* BREAK
*
*
* The value of this constant is 238
*
*/
LM_CPBRK_C(238),
/**
*
* CONCURRENT
*
*
* The value of this constant is 240
*
*/
LM_CONCR_C(240),
/**
*
* ENDCONCUR
*
*
* The value of this constant is 241
*
*/
LM_ENDCN_C(241),
/**
*
* motion group mask
*
*
* The value of this constant is 242
*
*/
LM_MOGRP_C(242),
/**
*
* EV (Extended Axes Speed)
*
*
* The value of this constant is 243
*
*/
LM_AUXF_C(243),
/**
*
* PTH
*
*
* The value of this constant is 244
*
*/
LM_PTH_C(244),
/**
*
* RTCP
*
*
* The value of this constant is 245
*
*/
LM_RTCP_C(245),
/**
*
* TIME BEFORE and TIME AFTER
*
*
* The value of this constant is 246
*
*/
LM_MOPTIME_C(246),
/**
*
* CD (Corner Distance)
*
*
* The value of this constant is 247
*
*/
LM_VC_C(247),
/**
*
* proc_sync feature (for multi-arm)
*
*
* The value of this constant is 248
*
*/
LM_PROCSYNC_C(248),
/**
*
* SING AVOID ON
*
*
* The value of this constant is 248
*
*/
LM_SD_ON_C(248),
/**
*
* SING AVOID OFF
*
*
* The value of this constant is 249
*
*/
LM_SD_OFF_C(249),
/**
*
* FOR FUTURE EXPANSION FOR MOTION OPTIONS
*
*
* The value of this constant is 249
*
*/
LM_MOTN_EXT_C(249),
/**
*
* MROT (Min Rotation)
*
*
* The value of this constant is 250
*
*/
LM_MROT_C(250),
/**
*
* Path Switching
*
*
* The value of this constant is 251
*
*/
LM_PS_C(251),
/**
*
* AccuCal II instruction
*
*
* The value of this constant is 252
*
*/
LM_CALB_C(252),
/**
*
* Independent/Simultaneous GP
*
*
* The value of this constant is 253
*
*/
LM_MLGP_C(253),
/**
*
* motion
*
*
* The value of this constant is 254
*
*/
LM_MOVE_C(254),
/**
*
* terminator
*
*
* The value of this constant is 255
*
*/
LM_TERM_C(255),
/**
*
* integer (1 byte)
*
*
* The value of this constant is 1
*
*/
LM_CNST_BYT_C(1),
/**
*
* longword integer (4 bytes)
*
*
* The value of this constant is 2
*
*/
LM_CNST_LNG_C(2),
/**
*
* Short real (4 bytes)
*
*
* The value of this constant is 3
*
*/
LM_CNST_FLT_C(3),
/**
*
* OFF
*
*
* The value of this constant is 0
*
*/
LM_SPC_OFF_C(0),
/**
*
* ON
*
*
* The value of this constant is 1
*
*/
LM_SPC_ON_C(1),
/**
*
* ENABLE
*
*
* The value of this constant is 2
*
*/
LM_SPC_ENB_C(2),
/**
*
* DISABLE
*
*
* The value of this constant is 3
*
*/
LM_SPC_DIS_C(3),
/**
*
* START
*
*
* The value of this constant is 4
*
*/
LM_SPC_START_C(4),
/**
*
* STOP
*
*
* The value of this constant is 5
*
*/
LM_SPC_STOP_C(5),
/**
*
* RESET
*
*
* The value of this constant is 6
*
*/
LM_SPC_RESET_C(6),
/**
*
* LPOS
*
*
* The value of this constant is 7
*
*/
LM_SPC_LPOS_C(7),
/**
*
* JPOS
*
*
* The value of this constant is 8
*
*/
LM_SPC_JPOS_C(8),
/**
*
* OPEN
*
*
* The value of this constant is 9
*
*/
LM_SPC_OPEN_C(9),
/**
*
* CLOSE
*
*
* The value of this constant is 10
*
*/
LM_SPC_CLOSE_C(10),
/**
*
* ENTER
*
*
* The value of this constant is 11
*
*/
LM_SPC_ENTER_C(11),
/**
*
* EXIT
*
*
* The value of this constant is 12
*
*/
LM_SPC_EXIT_C(12),
/**
*
* OPEN FOR CLAMP
*
*
* The value of this constant is 13
*
*/
LM_SPC_COPEN_C(13),
/**
*
* REPOZITION FOR CLAMP
*
*
* The value of this constant is 14
*
*/
LM_SPC_CREP_C(14),
/**
*
* HIGH FOR SPOT[..,V=H,...]
*
*
* The value of this constant is 17
*
*/
LM_SPC_SVHIGH_C(17),
/**
*
* MID FOR SPOT[..,V=M,...]
*
*
* The value of this constant is 18
*
*/
LM_SPC_SVMID_C(18),
/**
*
* LOW FOR SPOT[..,V=L,...]
*
*
* The value of this constant is 19
*
*/
LM_SPC_SVLOW_C(19),
/**
*
* * of BACKUP[i]=* etc.
*
*
* The value of this constant is 20
*
*/
LM_SPC_NOCHG_C(20),
/**
*
* OFF EDGE
*
*
* The value of this constant is 21
*
*/
LM_SPC_OFFE_C(21),
/**
*
* ON EDGE
*
*
* The value of this constant is 22
*
*/
LM_SPC_ONE_C(22),
/**
*
* TMP_DISABLE
*
*
* The value of this constant is 23
*
*/
LM_SPC_TMPDIS_C(23),
/**
*
* RELEASE
*
*
* The value of this constant is 24
*
*/
LM_SPC_DIS_RELEASE_C(24),
/**
*
* "*" of SPOT[P=*, S=, BU=*]
*
*
* The value of this constant is 255
*
*/
LM_SPC_NOCHG2_C(255),
/**
*
* reserve (untaught)
*
*
* The value of this constant is 0
*
*/
LM_MTN_UNT_C(0),
/**
*
* joint
*
*
* The value of this constant is 1
*
*/
LM_MTN_JNT_C(1),
/**
*
* linear
*
*
* The value of this constant is 2
*
*/
LM_MTN_LIN_C(2),
/**
*
* circular
*
*
* The value of this constant is 3
*
*/
LM_MTN_CIR_C(3),
/**
*
* inclination control
*
*
* The value of this constant is 4
*
*/
LM_MTN_INCL_C(4),
/**
*
* home
*
*
* The value of this constant is 5
*
*/
LM_MTN_HOME_C(5),
/**
*
* circle arc
*
*
* The value of this constant is 6
*
*/
LM_MTN_ARC_C(6),
/**
*
* normal position
*
*
* The value of this constant is 0
*
*/
LM_MTN_NPOS_C(0),
/**
*
* position register
*
*
* The value of this constant is 1
*
*/
LM_MTN_RPOS_C(1),
/**
*
* pallet motion
*
*
* The value of this constant is 2
*
*/
LM_MTN_PALLET_C(2),
/**
*
* Simple palletizing
*
*
* The value of this constant is 3
*
*/
LM_MTN_PAL2_C(3),
/**
*
* normal speed
*
*
* The value of this constant is 0
*
*/
LM_MTN_NSPD_C(0),
/**
*
* Direct register speed
*
*
* The value of this constant is 64
*
*/
LM_MTN_RSPD_C(64),
/**
*
* Indirect register speed
*
*
* The value of this constant is 128
*
*/
LM_MTN_RRSPD_C(128),
/**
*
* Application speed
*
*
* The value of this constant is 192
*
*/
LM_MTN_APPLSPD_C(192),
/**
*
* Mask for speed type field
*
*
* The value of this constant is 192
*
*/
LM_MTN_UNSPD_C(192),
/**
*
* % (joint)
*
*
* The value of this constant is 0
*
*/
LM_MTN_PER_C(0),
/**
*
* mm/sec
*
*
* The value of this constant is 1
*
*/
LM_MTN_MMSEC_C(1),
/**
*
* cm/min
*
*
* The value of this constant is 2
*
*/
LM_MTN_CMMIN_C(2),
/**
*
* inch/min
*
*
* The value of this constant is 3
*
*/
LM_MTN_INCMN_C(3),
/**
*
* deg/sec
*
*
* The value of this constant is 4
*
*/
LM_MTN_DGSEC_C(4),
/**
*
* sec
*
*
* The value of this constant is 5
*
*/
LM_MTN_SEC_C(5),
/**
*
* msec
*
*
* The value of this constant is 6
*
*/
LM_MTN_MSEC_C(6),
/**
*
* max_speed
*
*
* The value of this constant is 7
*
*/
LM_MTN_MSPD_C(7),
/**
*
* Fine
*
*
* The value of this constant is 0
*
*/
LM_MTN_FINE_C(0),
/**
*
* Cnt
*
*
* The value of this constant is 128
*
*/
LM_MTN_CNT_C(128),
/**
*
* CD
*
*
* The value of this constant is 192
*
*/
LM_MTN_CD_C(192),
/**
*
* Cnt (Indeirect)
*
*
* The value of this constant is 64
*
*/
LM_MTN_RCNT_C(64),
/**
*
* System defined AIR
*
*
* The value of this constant is 0
*
*/
LM_SYS_AIR(0),
/**
*
* User defined AIR
*
*
* The value of this constant is 1
*
*/
LM_USER_AIR(1),
/**
*
* Not use AIR
*
*
* The value of this constant is 2
*
*/
LM_NONE_AIR(2),
/**
*
* B=
*
*
* The value of this constant is 71
*
*/
LM_SP1_BB(71),
/**
*
* V=
*
*
* The value of this constant is 72
*
*/
LM_SP1_V(72),
/**
*
* S=
*
*
* The value of this constant is 73
*
*/
LM_SP1_S(73),
/**
*
* B=
*
*
* The value of this constant is 74
*
*/
LM_SP1_BA(74),
/**
*
* B=
*
*
* The value of this constant is 75
*
*/
LM_SP2_BB(75),
/**
*
* V=
*
*
* The value of this constant is 76
*
*/
LM_SP2_V(76),
/**
*
* S=
*
*
* The value of this constant is 77
*
*/
LM_SP2_S(77),
/**
*
* B=
*
*
* The value of this constant is 78
*
*/
LM_SP2_BA(78),
/**
*
* EQ=
*
*
* The value of this constant is 79
*
*/
LM_SP2_EQ(79),
/**
*
* WC=
*
*
* The value of this constant is 80
*
*/
LM_SP2_WC(80),
/**
*
*
*
*
* The value of this constant is 81
*
*/
LM_SP2_CT(81),
/**
*
*
*
*
* The value of this constant is 82
*
*/
LM_SP2_SN(82),
/**
*
*
*
*
* The value of this constant is 83
*
*/
LM_SP2_VL(83),
/**
*
* AOV(,)
*
*
* The value of this constant is 84
*
*/
LM_SP2_AV(84),
/**
*
*
*
*
* The value of this constant is 85
*
*/
LM_STK_FCTR(85),
/**
*
* Tip Stick data (invisible)
*
*
* The value of this constant is 86
*
*/
LM_TIP_STIK(86),
/**
*
*
*
*
* The value of this constant is 87
*
*/
LM_AVEN_C(87),
/**
*
* Equalization pressure EP=
*
*
* The value of this constant is 88
*
*/
LM_SP2_EP(88),
/**
*
* Servogun Zero Master[]
*
*
* The value of this constant is 0
*
*/
LM_SG_ZERO_C(0),
/**
*
* SV_SPOT[P=1, S=1]
*
*
* The value of this constant is 1
*
*/
LM_SVG_SINGLE(1),
/**
*
* SV_SPOT[P=(1,1), S=(1,1)]
*
*
* The value of this constant is 2
*
*/
LM_SVG_DOUBLE(2),
/**
*
* Pressure level Gun1
*
*
* The value of this constant is 3
*
*/
LM_PRES_GUN1_C(3),
/**
*
* Pressure level Gun2
*
*
* The value of this constant is 4
*
*/
LM_PRES_GUN2_C(4),
/**
*
* Press Motion[AP=,P=]
*
*
* The value of this constant is 5
*
*/
LM_SGPM1_DST_C(5),
/**
*
* Press Motion[AP=(,),P=(,)]
*
*
* The value of this constant is 6
*
*/
LM_SGPM2_DST_C(6),
/**
*
* GUN ATTACH [...]
*
*
* The value of this constant is 0
*
*/
LM_SVGC_ATCH_C(0),
/**
*
* GUN DETACH [...]
*
*
* The value of this constant is 1
*
*/
LM_SVGC_DTCH_C(1),
/**
*
* GUN EXCHANGE [...]
*
*
* The value of this constant is 2
*
*/
LM_SVGC_XCHG_C(2),
/**
*
* TOOL ATTACH [...]
*
*
* The value of this constant is 0
*
*/
LM_SVTC_ATCH_C(0),
/**
*
* TOOL DETACH [...]
*
*
* The value of this constant is 1
*
*/
LM_SVTC_DTCH_C(1),
/**
*
* IBS ATTACH GROUP_NAME
*
*
* The value of this constant is 0
*
*/
LM_IBSC_ATCH_C(0),
/**
*
* IBS DETACH GROUP_NAME
*
*
* The value of this constant is 1
*
*/
LM_IBSC_DTCH_C(1),
/**
*
* IBS attach SEGMENT_NUM
*
*
* The value of this constant is 0
*
*/
LM_IBPX_ATCH_C(0),
/**
*
* IBS detach SEGMENT_NUM
*
*
* The value of this constant is 1
*
*/
LM_IBPX_DTCH_C(1),
/**
*
* Soft float [...]
*
*
* The value of this constant is 0
*
*/
LM_SFLTSCD_C(0),
/**
*
* Sfot float END
*
*
* The value of this constant is 3
*
*/
LM_SFLTEND_C(3),
/**
*
* Follow up
*
*
* The value of this constant is 2
*
*/
LM_SFLTFLWUP_C(2),
/**
*
* Array of force command
*
*
* The value of this constant is 0
*
*/
LM_CC_ARRY_C(0),
/**
*
* Force Basic
*
*
* The value of this constant is 1
*
*/
LM_CC_BD_C(1),
/**
*
* Force Basic Array
*
*
* The value of this constant is 2
*
*/
LM_CC_BDA_C(2),
/**
*
* Insertion
*
*
* The value of this constant is 3
*
*/
LM_CC_ID_C(3),
/**
*
* Insertion Array
*
*
* The value of this constant is 4
*
*/
LM_CC_IDA_C(4),
/**
*
* GET Force postion
*
*
* The value of this constant is 5
*
*/
LM_CC_GET_C(5),
/**
*
* DEL Force postion
*
*
* The value of this constant is 6
*
*/
LM_CC_DEL_C(6),
/**
*
* Calibration tool
*
*
* The value of this constant is 7
*
*/
LM_CC_CAL_C(7),
/**
*
* Sensor Diagnosis
*
*
* The value of this constant is 8
*
*/
LM_CC_SNS_C(8),
/**
*
* Get monitor
*
*
* The value of this constant is 9
*
*/
LM_CC_GM_C(9),
/**
*
* Enable monitor
*
*
* The value of this constant is 10
*
*/
LM_CC_EM_C(10),
/**
*
* Disable monitor
*
*
* The value of this constant is 11
*
*/
LM_CC_DM_C(11),
/**
*
* SNAP vision mnemonic
*
*
* The value of this constant is 0
*
*/
LM_SNAP_C(0),
/**
*
* FIND vision mnemonic
*
*
* The value of this constant is 1
*
*/
LM_FIND_C(1),
/**
*
* FIND_NEXT vision mnemonic
*
*
* The value of this constant is 2
*
*/
LM_FNDNXT_C(2),
/**
*
* MEASURE vision mnemonic
*
*
* The value of this constant is 3
*
*/
LM_MEASURE_C(3),
/**
*
* CALC_OFFSET vision mnemonic
*
*
* The value of this constant is 4
*
*/
LM_CALOFST_C(4),
/**
*
* DISPLAY vision mnemonic
*
*
* The value of this constant is 5
*
*/
LM_DISPLAY_C(5),
/**
*
* CLEAR_MONITOR vision mnemonic
*
*
* The value of this constant is 6
*
*/
LM_CLRMON_C(6),
/**
*
* DO_PROCESS vision mnemonic
*
*
* The value of this constant is 7
*
*/
LM_DOPROC_C(7),
/**
*
* SAVE_QIMG vision mnemonic
*
*
* The value of this constant is 8
*
*/
LM_SAVQIMG_C(8),
/**
*
* DISP_QIMG vision mnemonic
*
*
* The value of this constant is 9
*
*/
LM_DSPQIMG_C(9),
/**
*
* CAM_CALIB vision mnemonic
*
*
* The value of this constant is 10
*
*/
LM_CAMCALI_C(10),
/**
*
* VIS SNAP ... VIEW[...]
*
*
* The value of this constant is 11
*
*/
LM_SNAP2_C(11),
/**
*
* VIS FIND ... VIEW[...]
*
*
* The value of this constant is 12
*
*/
LM_FIND2_C(12),
/**
*
* VIS FIND_NEXT ... VIEW[...]
*
*
* The value of this constant is 13
*
*/
LM_FNDNXT2_C(13),
/**
*
* VIS CALC_OFFSET ...
*
*
* The value of this constant is 14
*
*/
LM_CALOFST2_C(14),
/**
*
* VIS DISPLAY ... VIEW[...]
*
*
* The value of this constant is 15
*
*/
LM_DISPVIEW_C(15),
/**
*
* VIS DO_PROCESS ...
*
*
* The value of this constant is 16
*
*/
LM_DOPROC2_C(16),
/**
*
* VIS DO_PROC_NEXT ...
*
*
* The value of this constant is 17
*
*/
LM_DOPRONXT_C(17),
/**
*
* VIS DISPLAY ...
*
*
* The value of this constant is 18
*
*/
LM_DISPLAY2_C(18),
/**
*
* VIS POP_QUEUE
*
*
* The value of this constant is 19
*
*/
LM_POPQU_C(19),
/**
*
* VIS WAIT_PART ...
*
*
* The value of this constant is 20
*
*/
LM_WAIPT_C(20),
/**
*
* STICK DETECT ON
*
*
* The value of this constant is 0
*
*/
LM_STCK_ON_C(0),
/**
*
* STICK DETECT OFF
*
*
* The value of this constant is 1
*
*/
LM_STCK_OF_C(1),
/**
*
* PAYLOAD[...]
*
*
* The value of this constant is 1
*
*/
LM_SGPLST_C(1),
/**
*
* PAYLOAD[GP:...]
*
*
* The value of this constant is 2
*
*/
LM_MLPLST_C(2),
/**
*
* LOW SPEED DATA
*
*
* The value of this constant is 3
*
*/
LM_LOWD_C(3),
/**
*
* LOW SPEED PROC
*
*
* The value of this constant is 4
*
*/
LM_LOWP_C(4),
/**
*
* HIGH SPEED DATA
*
*
* The value of this constant is 5
*
*/
LM_HIGHD_C(5),
/**
*
* ESTIMATION
*
*
* The value of this constant is 6
*
*/
LM_EST_C(6),
/**
*
* ESTIMATION M=...
*
*
* The value of this constant is 7
*
*/
LM_EST_M_C(7),
/**
*
* APPLY EST [...]
*
*
* The value of this constant is 8
*
*/
LM_APLY_C(8),
/**
*
* LOAD CLUTCH START
*
*
* The value of this constant is 0
*
*/
LM_LDCL_STA_C(0),
/**
*
* LOAD CLUTCH END
*
*
* The value of this constant is 1
*
*/
LM_LDCL_END_C(1),
/**
*
* Joint Max Speed
*
*
* The value of this constant is 1
*
*/
LM_MSPD_JNT_C(1),
/**
*
* Linear Max Speed
*
*
* The value of this constant is 2
*
*/
LM_MSPD_LIN_C(2),
/**
*
* 2DVIS DO_PROCESS menmonic
*
*
* The value of this constant is 1
*
*/
LM_PCVIS_DOPRO_C(1),
/**
*
* STITCH[...]
*
*
* The value of this constant is 0
*
*/
LM_STCH_START_C(0),
/**
*
* STITCH END
*
*
* The value of this constant is 1
*
*/
LM_STCH_END_C(1),
/**
*
* Approach_STOP[...]
*
*
* The value of this constant is 0
*
*/
LM_IA_IASTOP_C(0),
/**
*
* Approach_WAIT[...]
*
*
* The value of this constant is 1
*
*/
LM_IA_IAWAIT_C(1),
/**
*
* Approach_RATE[...]
*
*
* The value of this constant is 2
*
*/
LM_IA_IARATE_C(2),
/**
*
* VIS DO_PROCESS menmonic
*
*
* The value of this constant is 1
*
*/
LM_SIVIS_DOPRO_C(1),
/**
*
* LM_IWC_ISOCON_C
*
*
* The value of this constant is 0
*
*/
LM_IWC_ISOCON_C(0),
/**
*
* LM_IWC_RSTSTP_C
*
*
* The value of this constant is 1
*
*/
LM_IWC_RSTSTP_C(1),
/**
*
* LM_IWC_RSTWLD_C
*
*
* The value of this constant is 2
*
*/
LM_IWC_RSTWLD_C(2),
/**
*
* PROC_START[...]
*
*
* The value of this constant is 0
*
*/
LM_PSYN_START_C(0),
/**
*
* PROC_END
*
*
* The value of this constant is 1
*
*/
LM_PSYN_END_C(1),
/**
*
* PROC_SYNC[...]
*
*
* The value of this constant is 2
*
*/
LM_PSYN_SYNC_C(2),
/**
*
* INPOS[..]
*
*
* The value of this constant is 3
*
*/
LM_PSYN_INPOS_C(3),
;
private final int value;
FRELMCodeConstants(int value) { this.value=value; }
public int comEnumValue() { return value; }
}