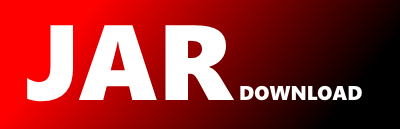
com.github.wshackle.fanuc.robotserver.FRETPKeyConstants Maven / Gradle / Ivy
package com.github.wshackle.fanuc.robotserver ;
import com4j.*;
/**
*
* Constants for Teach Pendant keys.
*
*/
public enum FRETPKeyConstants implements ComEnum {
/**
*
* Up arrow.
*
*
* The value of this constant is 212
*
*/
frTPKeyUpArw(212),
/**
*
* Down arrow.
*
*
* The value of this constant is 213
*
*/
frTPKeyDnArw(213),
/**
*
* Right arrow.
*
*
* The value of this constant is 208
*
*/
frTPKeyRtArw(208),
/**
*
* Left arrow.
*
*
* The value of this constant is 209
*
*/
frTPKeyLfArw(209),
/**
*
* Shift up arrow.
*
*
* The value of this constant is 204
*
*/
frTPKeyShfUpArw(204),
/**
*
* Shift down arrow.
*
*
* The value of this constant is 205
*
*/
frTPKeyShfDnArw(205),
/**
*
* Shift right arrow.
*
*
* The value of this constant is 206
*
*/
frTPKeyShfRtArw(206),
/**
*
* Shift left arrow.
*
*
* The value of this constant is 207
*
*/
frTPKeyShfLfArw(207),
/**
*
* PREV key.
*
*
* The value of this constant is 128
*
*/
frTPKeyPrev(128),
/**
*
* F1 key.
*
*
* The value of this constant is 129
*
*/
frTPKeyF1(129),
/**
*
* F2 key.
*
*
* The value of this constant is 131
*
*/
frTPKeyF2(131),
/**
*
* F3 key.
*
*
* The value of this constant is 132
*
*/
frTPKeyF3(132),
/**
*
* F4 key.
*
*
* The value of this constant is 133
*
*/
frTPKeyF4(133),
/**
*
* F5 key.
*
*
* The value of this constant is 134
*
*/
frTPKeyF5(134),
/**
*
* NEXT key.
*
*
* The value of this constant is 135
*
*/
frTPKeyNext(135),
/**
*
* Shift PREV key.
*
*
* The value of this constant is 136
*
*/
frTPKeyShfPrev(136),
/**
*
* Shift F1 key.
*
*
* The value of this constant is 137
*
*/
frTPKeyShfF1(137),
/**
*
* Shift F2 key.
*
*
* The value of this constant is 138
*
*/
frTPKeyShfF2(138),
/**
*
* Shift F3 key.
*
*
* The value of this constant is 139
*
*/
frTPKeyShfF3(139),
/**
*
* Shift F4 key.
*
*
* The value of this constant is 140
*
*/
frTPKeyShfF4(140),
/**
*
* Shift F5 key.
*
*
* The value of this constant is 141
*
*/
frTPKeyShfF5(141),
/**
*
* Shift NEXT key.
*
*
* The value of this constant is 142
*
*/
frTPKeyShfNext(142),
/**
*
* User 1 key.
*
*
* The value of this constant is 173
*
*/
frTPKeyUsr1(173),
/**
*
* User 2 key.
*
*
* The value of this constant is 174
*
*/
frTPKeyUsr2(174),
/**
*
* User 3 key.
*
*
* The value of this constant is 175
*
*/
frTPKeyUsr3(175),
/**
*
* User 4 key.
*
*
* The value of this constant is 176
*
*/
frTPKeyUsr4(176),
/**
*
* User 5 key.
*
*
* The value of this constant is 177
*
*/
frTPKeyUsr5(177),
/**
*
* User 6 key.
*
*
* The value of this constant is 178
*
*/
frTPKeyUsr6(178),
/**
*
* User 7 key.
*
*
* The value of this constant is 210
*
*/
frTPKeyUsr7(210),
/**
*
* Shift User 1 key.
*
*
* The value of this constant is 179
*
*/
frTPKeyShfUsr1(179),
/**
*
* Shift User 2 key.
*
*
* The value of this constant is 180
*
*/
frTPKeyShfUsr2(180),
/**
*
* Shift User 3 key.
*
*
* The value of this constant is 181
*
*/
frTPKeyShfUsr3(181),
/**
*
* Shift User 4 key.
*
*
* The value of this constant is 182
*
*/
frTPKeyShfUsr4(182),
/**
*
* Shift User 5 key.
*
*
* The value of this constant is 183
*
*/
frTPKeyShfUsr5(183),
/**
*
* Shift User 6 key.
*
*
* The value of this constant is 184
*
*/
frTPKeyShfUsr6(184),
/**
*
* Shift User 7 key.
*
*
* The value of this constant is 211
*
*/
frTPKeyShfUsr7(211),
/**
*
* ITEM key.
*
*
* The value of this constant is 148
*
*/
frTPKeyItem(148),
/**
*
* STEP key.
*
*
* The value of this constant is 152
*
*/
frTPKeyStep(152),
/**
*
* Shift ITEM key.
*
*
* The value of this constant is 154
*
*/
frTPKeyShfItem(154),
/**
*
* Shift STEP key.
*
*
* The value of this constant is 157
*
*/
frTPKeyShfStep(157),
/**
*
* Enter.
*
*
* The value of this constant is 13
*
*/
frTPKeyEnter(13),
/**
*
* Backspace.
*
*
* The value of this constant is 8
*
*/
frTPKeyBackspace(8),
;
private final int value;
FRETPKeyConstants(int value) { this.value=value; }
public int comEnumValue() { return value; }
}