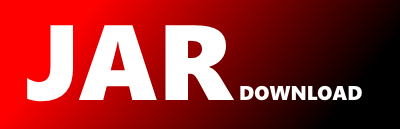
com.github.wshackle.fanuc.robotserver.FRETPScreenConstants Maven / Gradle / Ivy
package com.github.wshackle.fanuc.robotserver ;
import com4j.*;
/**
*
* Constants for TPScreen control characters.
*
*/
public enum FRETPScreenConstants implements ComEnum {
/**
*
* ASCII Backspace.
*
*
* The value of this constant is 8
*
*/
frTPScrnBackspace(8),
/**
*
* ASCII Tab.
*
*
* The value of this constant is 9
*
*/
frTPScrnTab(9),
/**
*
* ASCII Linefeed.
*
*
* The value of this constant is 10
*
*/
frTPScrnLinefeed(10),
/**
*
* ASCII Vertical Tab.
*
*
* The value of this constant is 11
*
*/
frTPScrnVertTab(11),
/**
*
* ASCII Formfeed.
*
*
* The value of this constant is 12
*
*/
frTPScrnFormfeed(12),
/**
*
* ASCII Carriage Return.
*
*
* The value of this constant is 13
*
*/
frTPScrnCR(13),
/**
*
* Clear Window.
*
*
* The value of this constant is 128
*
*/
frTPScrnClear(128),
/**
*
* Clear to End of Line.
*
*
* The value of this constant is 129
*
*/
frTPScrnClearEOL(129),
/**
*
* Clear to End of Window.
*
*
* The value of this constant is 130
*
*/
frTPScrnClearEOW(130),
/**
*
* Cursor Position.
*
*
* The value of this constant is 131
*
*/
frTPScrnCUP(131),
/**
*
* Cursor Return.
*
*
* The value of this constant is 132
*
*/
frTPScrnReturn(132),
/**
*
* Cursor Down.
*
*
* The value of this constant is 133
*
*/
frTPScrnCursorDown(133),
/**
*
* Cursor Up.
*
*
* The value of this constant is 134
*
*/
frTPScrnCursorUp(134),
/**
*
* New Line.
*
*
* The value of this constant is 135
*
*/
frTPScrnNewLine(135),
/**
*
* Cursor Back.
*
*
* The value of this constant is 136
*
*/
frTPScrnCursorBack(136),
/**
*
* Cursor Home.
*
*
* The value of this constant is 137
*
*/
frTPScrnCursorHome(137),
/**
*
* Blink.
*
*
* The value of this constant is 138
*
*/
frTPScrnBlink(138),
/**
*
* Reverse Video.
*
*
* The value of this constant is 139
*
*/
frTPScrnReverseVid(139),
/**
*
* Bold.
*
*
* The value of this constant is 140
*
*/
frTPScrnBold(140),
/**
*
* Underscore.
*
*
* The value of this constant is 141
*
*/
frTPScrnUnderscore(141),
/**
*
* Double Wide.
*
*
* The value of this constant is 142
*
*/
frTPScrnWideSize(142),
/**
*
* Normal Attributes.
*
*
* The value of this constant is 143
*
*/
frTPScrnNormal(143),
/**
*
* Blink Off.
*
*
* The value of this constant is 144
*
*/
frTPScrnNoBlink(144),
/**
*
* Reverse Video Off.
*
*
* The value of this constant is 145
*
*/
frTPScrnNoReverseVid(145),
/**
*
* Graphics Character Set.
*
*
* The value of this constant is 146
*
*/
frTPScrnGraphics(146),
/**
*
* ASCII Character Set.
*
*
* The value of this constant is 147
*
*/
frTPScrnASCII(147),
/**
*
* Double High.
*
*
* The value of this constant is 148
*
*/
frTPScrnHighSize(148),
/**
*
* Normal Size.
*
*
* The value of this constant is 153
*
*/
frTPScrnNormalSize(153),
/**
*
* Cursor Forward.
*
*
* The value of this constant is 156
*
*/
frTPScrnCursorForward(156),
/**
*
* Scroll Region.
*
*
* The value of this constant is 157
*
*/
frTPScrnScroll(157),
/**
*
* European Character Set.
*
*
* The value of this constant is 158
*
*/
frTPScrnEuropean(158),
/**
*
* Katakana Character Set.
*
*
* The value of this constant is 159
*
*/
frTPScrnKatakana(159),
;
private final int value;
FRETPScreenConstants(int value) { this.value=value; }
public int comEnumValue() { return value; }
}