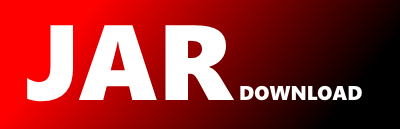
com.github.wshackle.fanuc.robotserver.IIOConfig Maven / Gradle / Ivy
package com.github.wshackle.fanuc.robotserver ;
import com4j.*;
/**
* This object represents an input or output configuration.
*/
@IID("{59DC1702-AF91-11D0-A072-00A02436CF7E}")
public interface IIOConfig extends com.github.wshackle.fanuc.robotserver.IRobotObject {
// Methods:
/**
*
* Returns the first logical signal number of a configuration.
*
*
* Getter method for the COM property "FirstLogicalNum"
*
* @return Returns a value of type int
*/
@DISPID(101) //= 0x65. The runtime will prefer the VTID if present
@VTID(8)
int firstLogicalNum();
/**
*
* Returns/sets the number of logical signals configured for the I/O type; returns/sets the number of physical ports assigned for a group type.
*
*
* Getter method for the COM property "NumSignals"
*
* @return Returns a value of type int
*/
@DISPID(102) //= 0x66. The runtime will prefer the VTID if present
@VTID(9)
int numSignals();
/**
*
* Returns/sets the number of logical signals configured for the I/O type; returns/sets the number of physical ports assigned for a group type.
*
*
* Setter method for the COM property "NumSignals"
*
* @param numSignals Mandatory int parameter.
*/
@DISPID(102) //= 0x66. The runtime will prefer the VTID if present
@VTID(10)
void numSignals(
int numSignals);
/**
*
* Returns/sets the rack containing the physical port module.
*
*
* Getter method for the COM property "Rack"
*
* @return Returns a value of type short
*/
@DISPID(103) //= 0x67. The runtime will prefer the VTID if present
@VTID(11)
short rack();
/**
*
* Returns/sets the rack containing the physical port module.
*
*
* Setter method for the COM property "Rack"
*
* @param rack Mandatory short parameter.
*/
@DISPID(103) //= 0x67. The runtime will prefer the VTID if present
@VTID(12)
void rack(
short rack);
/**
*
* Returns/sets the slot in the rack containing the physical port module.
*
*
* Getter method for the COM property "Slot"
*
* @return Returns a value of type short
*/
@DISPID(104) //= 0x68. The runtime will prefer the VTID if present
@VTID(13)
short slot();
/**
*
* Returns/sets the slot in the rack containing the physical port module.
*
*
* Setter method for the COM property "Slot"
*
* @param slot Mandatory short parameter.
*/
@DISPID(104) //= 0x68. The runtime will prefer the VTID if present
@VTID(14)
void slot(
short slot);
/**
*
* Returns/sets the physical port type to be assigned to.
*
*
* Getter method for the COM property "PhysicalType"
*
* @return Returns a value of type int
*/
@DISPID(105) //= 0x69. The runtime will prefer the VTID if present
@VTID(15)
int physicalType();
/**
*
* Returns/sets the physical port type to be assigned to.
*
*
* Setter method for the COM property "PhysicalType"
*
* @param physicalType Mandatory int parameter.
*/
@DISPID(105) //= 0x69. The runtime will prefer the VTID if present
@VTID(16)
void physicalType(
int physicalType);
/**
*
* Returns/sets the number of the physical port to be assigned to; returns/sets the least-significant bit for group I/O type.
*
*
* Getter method for the COM property "FirstPhysicalNum"
*
* @return Returns a value of type int
*/
@DISPID(106) //= 0x6a. The runtime will prefer the VTID if present
@VTID(17)
int firstPhysicalNum();
/**
*
* Returns/sets the number of the physical port to be assigned to; returns/sets the least-significant bit for group I/O type.
*
*
* Setter method for the COM property "FirstPhysicalNum"
*
* @param firstPhysicalNum Mandatory int parameter.
*/
@DISPID(106) //= 0x6a. The runtime will prefer the VTID if present
@VTID(18)
void firstPhysicalNum(
int firstPhysicalNum);
/**
*
* Returns True if the configuration is valid for the connected robot controller, returns False otherwise.
*
*
* Getter method for the COM property "IsValid"
*
* @return Returns a value of type boolean
*/
@DISPID(107) //= 0x6b. The runtime will prefer the VTID if present
@VTID(19)
boolean isValid();
/**
*
* Returns the owning configurations collection
*
*
* Getter method for the COM property "Parent"
*
* @return Returns a value of type com.github.wshackle.fanuc.robotserver.IIOConfigs
*/
@DISPID(108) //= 0x6c. The runtime will prefer the VTID if present
@VTID(20)
com.github.wshackle.fanuc.robotserver.IIOConfigs parent();
// Properties:
}