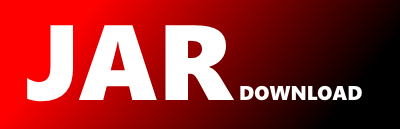
com.github.wshackle.fanuc.robotserver.IIOSignal Maven / Gradle / Ivy
package com.github.wshackle.fanuc.robotserver ;
import com4j.*;
/**
* Represents an I/O signal.
*/
@IID("{59DC16FB-AF91-11D0-A072-00A02436CF7E}")
public interface IIOSignal extends com.github.wshackle.fanuc.robotserver.IRobotObject {
// Methods:
/**
*
* Returns the logical signal number.
*
*
* Getter method for the COM property "LogicalNum"
*
* @return Returns a value of type int
*/
@DISPID(101) //= 0x65. The runtime will prefer the VTID if present
@VTID(8)
int logicalNum();
/**
*
* Returns/sets the comment string of a signal.
*
*
* Getter method for the COM property "Comment"
*
* @return Returns a value of type java.lang.String
*/
@DISPID(102) //= 0x66. The runtime will prefer the VTID if present
@VTID(9)
java.lang.String comment();
/**
*
* Returns/sets the comment string of a signal.
*
*
* Setter method for the COM property "Comment"
*
* @param comment Mandatory java.lang.String parameter.
*/
@DISPID(102) //= 0x66. The runtime will prefer the VTID if present
@VTID(10)
void comment(
java.lang.String comment);
/**
*
* Returns True if the signal is assigned, returns False otherwise.
*
*
* Getter method for the COM property "IsAssigned"
*
* @return Returns a value of type boolean
*/
@DISPID(103) //= 0x67. The runtime will prefer the VTID if present
@VTID(11)
boolean isAssigned();
/**
*
* Returns True if signal is offline, returns False otherwise.
*
*
* Getter method for the COM property "IsOffline"
*
* @return Returns a value of type boolean
*/
@DISPID(104) //= 0x68. The runtime will prefer the VTID if present
@VTID(12)
boolean isOffline();
/**
*
* Returns True if monitoring is enabled, False if not.
*
*
* Getter method for the COM property "IsMonitoring"
*
* @return Returns a value of type boolean
*/
@DISPID(105) //= 0x69. The runtime will prefer the VTID if present
@VTID(13)
boolean isMonitoring();
/**
*
* Starts the monitoring of the I/O signal for changes.
*
* @param latency Mandatory int parameter.
*/
@DISPID(150) //= 0x96. The runtime will prefer the VTID if present
@VTID(14)
void startMonitor(
int latency);
/**
*
* Stops the monitoring of the I/O signal for changes.
*
*/
@DISPID(151) //= 0x97. The runtime will prefer the VTID if present
@VTID(15)
void stopMonitor();
// Properties:
}