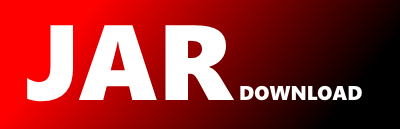
com.github.wshackle.fanuc.robotserver.IPosition2 Maven / Gradle / Ivy
package com.github.wshackle.fanuc.robotserver ;
import com4j.*;
/**
* Provides access to the positional data.
*/
@IID("{E494B8E1-A59A-11D2-8724-00C04F918427}")
public interface IPosition2 extends com.github.wshackle.fanuc.robotserver.IRobotObject {
// Methods:
/**
*
* Returns the owning program object.
*
*
* Getter method for the COM property "Program"
*
* @return Returns a value of type com.github.wshackle.fanuc.robotserver.IProgram
*/
@DISPID(101) //= 0x65. The runtime will prefer the VTID if present
@VTID(8)
com.github.wshackle.fanuc.robotserver.IProgram program();
/**
*
* Getter method for the COM property "AutomaticUpdate"
*
* @return Returns a value of type boolean
*/
@DISPID(201) //= 0xc9. The runtime will prefer the VTID if present
@VTID(9)
boolean automaticUpdate();
/**
*
* Setter method for the COM property "AutomaticUpdate"
*
* @param automaticUpdate Mandatory boolean parameter.
*/
@DISPID(201) //= 0xc9. The runtime will prefer the VTID if present
@VTID(10)
void automaticUpdate(
boolean automaticUpdate);
/**
*
* Returns/sets the format the position is stored in.
*
*
* Getter method for the COM property "Type"
*
* @return Returns a value of type com.github.wshackle.fanuc.robotserver.FRETypeCodeConstants
*/
@DISPID(202) //= 0xca. The runtime will prefer the VTID if present
@VTID(11)
com.github.wshackle.fanuc.robotserver.FRETypeCodeConstants type();
/**
*
* Returns/sets the format the position is stored in.
*
*
* Setter method for the COM property "Type"
*
* @param type Mandatory com.github.wshackle.fanuc.robotserver.FRETypeCodeConstants parameter.
*/
@DISPID(202) //= 0xca. The runtime will prefer the VTID if present
@VTID(12)
void type(
com.github.wshackle.fanuc.robotserver.FRETypeCodeConstants type);
/**
*
* Returns positional data in the specified format.
*
*
* Getter method for the COM property "Formats"
*
* @param type Mandatory com.github.wshackle.fanuc.robotserver.FRETypeCodeConstants parameter.
* @return Returns a value of type com4j.Com4jObject
*/
@DISPID(203) //= 0xcb. The runtime will prefer the VTID if present
@VTID(13)
@ReturnValue(type=NativeType.Dispatch)
com4j.Com4jObject formats(
com.github.wshackle.fanuc.robotserver.FRETypeCodeConstants type);
/**
*
* Returns the group number for the position.
*
*
* Getter method for the COM property "GroupNum"
*
* @return Returns a value of type short
*/
@DISPID(204) //= 0xcc. The runtime will prefer the VTID if present
@VTID(14)
short groupNum();
/**
*
* Returns the position identification number for the position.
*
*
* Getter method for the COM property "Id"
*
* @return Returns a value of type int
*/
@DISPID(205) //= 0xcd. The runtime will prefer the VTID if present
@VTID(15)
int id();
/**
*
* Returns whether the robot is currently at the position.
*
*
* Getter method for the COM property "IsAtCurPosition"
*
* @return Returns a value of type boolean
*/
@DISPID(206) //= 0xce. The runtime will prefer the VTID if present
@VTID(16)
boolean isAtCurPosition();
/**
*
* Returns whether the position is initialized.
*
*
* Getter method for the COM property "IsInitialized"
*
* @return Returns a value of type boolean
*/
@DISPID(207) //= 0xcf. The runtime will prefer the VTID if present
@VTID(17)
boolean isInitialized();
/**
*
* Returns/sets the User Frame to which the position is referenced.
*
*
* Getter method for the COM property "UserFrame"
*
* @return Returns a value of type int
*/
@DISPID(208) //= 0xd0. The runtime will prefer the VTID if present
@VTID(18)
int userFrame();
/**
*
* Returns/sets the User Frame to which the position is referenced.
*
*
* Setter method for the COM property "UserFrame"
*
* @param userFrame Mandatory int parameter.
*/
@DISPID(208) //= 0xd0. The runtime will prefer the VTID if present
@VTID(19)
void userFrame(
int userFrame);
/**
*
* Returns/sets the User Tool to which the position is referenced.
*
*
* Getter method for the COM property "UserTool"
*
* @return Returns a value of type int
*/
@DISPID(209) //= 0xd1. The runtime will prefer the VTID if present
@VTID(20)
int userTool();
/**
*
* Returns/sets the User Tool to which the position is referenced.
*
*
* Setter method for the COM property "UserTool"
*
* @param userTool Mandatory int parameter.
*/
@DISPID(209) //= 0xd1. The runtime will prefer the VTID if present
@VTID(21)
void userTool(
int userTool);
/**
*
* Returns whether the position is currently being monitored for changes.
*
*
* Getter method for the COM property "IsMonitoring"
*
* @return Returns a value of type boolean
*/
@DISPID(210) //= 0xd2. The runtime will prefer the VTID if present
@VTID(22)
boolean isMonitoring();
/**
*
* Updates any changes to the position back to the controller.
*
*/
@DISPID(251) //= 0xfb. The runtime will prefer the VTID if present
@VTID(23)
void update();
/**
*
* Clears all changes to the position since the last update of the position.
*
*/
@DISPID(252) //= 0xfc. The runtime will prefer the VTID if present
@VTID(24)
void refresh();
/**
*
* Moves the robot to this position.
*
*/
@DISPID(253) //= 0xfd. The runtime will prefer the VTID if present
@VTID(25)
void moveto();
/**
*
* Records the current position of the robot to this position.
*
*/
@DISPID(254) //= 0xfe. The runtime will prefer the VTID if present
@VTID(26)
void record();
/**
*
* Enables the Change event, with specified latency.
*
* @param latency Mandatory int parameter.
*/
@DISPID(255) //= 0xff. The runtime will prefer the VTID if present
@VTID(27)
void startMonitor(
int latency);
/**
*
* Stops the Change event from occurring.
*
*/
@DISPID(256) //= 0x100. The runtime will prefer the VTID if present
@VTID(28)
void stopMonitor();
/**
*
* Uninitializes the position.
*
*/
@DISPID(257) //= 0x101. The runtime will prefer the VTID if present
@VTID(29)
void uninitialize();
/**
*
* Multiply two input positions? transformation matrices and set the results to this position.
*
* @param leftPos Mandatory com4j.Com4jObject parameter.
* @param rightPos Mandatory com4j.Com4jObject parameter.
*/
@DISPID(258) //= 0x102. The runtime will prefer the VTID if present
@VTID(30)
void matMul(
@MarshalAs(NativeType.Dispatch) com4j.Com4jObject leftPos,
@MarshalAs(NativeType.Dispatch) com4j.Com4jObject rightPos);
/**
*
* Invert the input position transformation matrix and set the results to this position.
*
* @param inputPos Mandatory com4j.Com4jObject parameter.
*/
@DISPID(259) //= 0x103. The runtime will prefer the VTID if present
@VTID(31)
void matInv(
@MarshalAs(NativeType.Dispatch) com4j.Com4jObject inputPos);
/**
*
* Returns a boolean value that indicates if the positional data contained in the current object is 'almost equal to' the positional data of another object.
*
*
* Getter method for the COM property "IsEqualTo"
*
* @param targetPos Mandatory com4j.Com4jObject parameter.
* @return Returns a value of type boolean
*/
@DISPID(260) //= 0x104. The runtime will prefer the VTID if present
@VTID(32)
boolean isEqualTo(
@MarshalAs(NativeType.Dispatch) com4j.Com4jObject targetPos);
/**
*
* Returns a Boolean indicating if the robot can reach the position or not.
*
*
* Getter method for the COM property "IsReachable"
*
* @param from Optional parameter. Default value is com4j.Variant.getMissing()
* @param moType Optional parameter. Default value is 6
* @param orientType Optional parameter. Default value is 2
* @param destination Optional parameter. Default value is com4j.Variant.getMissing()
* @param motionErrorInfo Optional parameter. Default value is 0
* @return Returns a value of type boolean
*/
@DISPID(261) //= 0x105. The runtime will prefer the VTID if present
@VTID(33)
boolean isReachable(
@Optional @MarshalAs(NativeType.VARIANT) java.lang.Object from,
@Optional @DefaultValue("6") com.github.wshackle.fanuc.robotserver.FREMotionTypeConstants moType,
@Optional @DefaultValue("2") com.github.wshackle.fanuc.robotserver.FREOrientTypeConstants orientType,
@Optional @MarshalAs(NativeType.VARIANT) java.lang.Object destination,
@Optional @DefaultValue("0") Holder motionErrorInfo);
/**
*
* Copies the positional data from another object into this one.
*
* @param position Mandatory com4j.Com4jObject parameter.
*/
@DISPID(262) //= 0x106. The runtime will prefer the VTID if present
@VTID(34)
void copy(
@MarshalAs(NativeType.Dispatch) com4j.Com4jObject position);
/**
*
* Returns a Boolean indicating if the robot can reach the position or not.
*
* @param from Optional parameter. Default value is com4j.Variant.getMissing()
* @param moType Optional parameter. Default value is 6
* @param orientType Optional parameter. Default value is 2
* @param destination Optional parameter. Default value is com4j.Variant.getMissing()
* @param motionErrorInfo Optional parameter. Default value is 0
* @return Returns a value of type boolean
*/
@DISPID(263) //= 0x107. The runtime will prefer the VTID if present
@VTID(35)
boolean checkReach(
@Optional @MarshalAs(NativeType.VARIANT) java.lang.Object from,
@Optional @DefaultValue("6") com.github.wshackle.fanuc.robotserver.FREMotionTypeConstants moType,
@Optional @DefaultValue("2") com.github.wshackle.fanuc.robotserver.FREOrientTypeConstants orientType,
@Optional @MarshalAs(NativeType.VARIANT) java.lang.Object destination,
@Optional @DefaultValue("0") Holder motionErrorInfo);
// Properties:
}