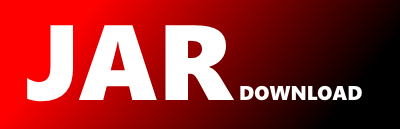
com.github.wshackle.fanuc.robotserver.IRobot2 Maven / Gradle / Ivy
package com.github.wshackle.fanuc.robotserver ;
import com4j.*;
/**
* Top level Robot Object interface used to establish connections and get references objects representing other system areas.
*/
@IID("{A6861F10-5F34-4053-ABE4-55C55F595814}")
public interface IRobot2 extends Com4jObject {
// Methods:
/**
*
* Gets the host name of the robot to which this object is connected.
*
*
* Getter method for the COM property "HostName"
*
* @return Returns a value of type java.lang.String
*/
@DISPID(101) //= 0x65. The runtime will prefer the VTID if present
@VTID(7)
java.lang.String hostName();
/**
*
* Gets the current position of the robot arm.
*
*
* Getter method for the COM property "CurPosition"
*
* @return Returns a value of type com.github.wshackle.fanuc.robotserver.ICurPosition
*/
@DISPID(102) //= 0x66. The runtime will prefer the VTID if present
@VTID(8)
com.github.wshackle.fanuc.robotserver.ICurPosition curPosition();
/**
*
* Gets the devices of the robot.
*
*
* Getter method for the COM property "Devices"
*
* @return Returns a value of type com4j.Com4jObject
*/
@DISPID(103) //= 0x67. The runtime will prefer the VTID if present
@VTID(9)
@ReturnValue(type=NativeType.Dispatch)
com4j.Com4jObject devices();
/**
*
* Gets the list of programs currently loaded on the controller.
*
*
* Getter method for the COM property "Programs"
*
* @return Returns a value of type com.github.wshackle.fanuc.robotserver.IPrograms
*/
@DISPID(104) //= 0x68. The runtime will prefer the VTID if present
@VTID(10)
com.github.wshackle.fanuc.robotserver.IPrograms programs();
/**
*
* Gets the position registers of the robot.
*
*
* Getter method for the COM property "RegPositions"
*
* @return Returns a value of type com.github.wshackle.fanuc.robotserver.ISysPositions
*/
@DISPID(105) //= 0x69. The runtime will prefer the VTID if present
@VTID(11)
com.github.wshackle.fanuc.robotserver.ISysPositions regPositions();
/**
*
* Gets the numeric registers of the robot.
*
*
* Getter method for the COM property "RegNumerics"
*
* @return Returns a value of type com.github.wshackle.fanuc.robotserver.IVars
*/
@DISPID(106) //= 0x6a. The runtime will prefer the VTID if present
@VTID(12)
com.github.wshackle.fanuc.robotserver.IVars regNumerics();
/**
*
* Gets the system variables of the robot.
*
*
* Getter method for the COM property "SysVariables"
*
* @return Returns a value of type com.github.wshackle.fanuc.robotserver.IVars
*/
@DISPID(107) //= 0x6b. The runtime will prefer the VTID if present
@VTID(13)
com.github.wshackle.fanuc.robotserver.IVars sysVariables();
/**
*
* Gets or sets which device is currently the remote master of the motion system.
*
*
* Getter method for the COM property "RemoteMotionMaster"
*
* @return Returns a value of type com.github.wshackle.fanuc.robotserver.FRERemoteMotionMasterConstants
*/
@DISPID(108) //= 0x6c. The runtime will prefer the VTID if present
@VTID(14)
com.github.wshackle.fanuc.robotserver.FRERemoteMotionMasterConstants remoteMotionMaster();
/**
*
* Gets or sets which device is currently the remote master of the motion system.
*
*
* Setter method for the COM property "RemoteMotionMaster"
*
* @param remoteMotionMaster Mandatory com.github.wshackle.fanuc.robotserver.FRERemoteMotionMasterConstants parameter.
*/
@DISPID(108) //= 0x6c. The runtime will prefer the VTID if present
@VTID(15)
void remoteMotionMaster(
com.github.wshackle.fanuc.robotserver.FRERemoteMotionMasterConstants remoteMotionMaster);
/**
*
* Gets the list of active and logged alarms for the robot.
*
*
* Getter method for the COM property "Alarms"
*
* @return Returns a value of type com.github.wshackle.fanuc.robotserver.IAlarms
*/
@DISPID(109) //= 0x6d. The runtime will prefer the VTID if present
@VTID(16)
com.github.wshackle.fanuc.robotserver.IAlarms alarms();
/**
*
* Gets the IO objects of the robot.
*
*
* Getter method for the COM property "IOTypes"
*
* @return Returns a value of type com.github.wshackle.fanuc.robotserver.IIOTypes
*/
@DISPID(110) //= 0x6e. The runtime will prefer the VTID if present
@VTID(17)
com.github.wshackle.fanuc.robotserver.IIOTypes ioTypes();
/**
*
* Gets the Teach Pendant screen interface object.
*
*
* Getter method for the COM property "TPScreen"
*
* @return Returns a value of type com.github.wshackle.fanuc.robotserver.ITPScreen
*/
@DISPID(111) //= 0x6f. The runtime will prefer the VTID if present
@VTID(18)
com.github.wshackle.fanuc.robotserver.ITPScreen tpScreen();
/**
*
* Gets or sets the language of the robot controller.
*
*
* Getter method for the COM property "Language"
*
* @return Returns a value of type com.github.wshackle.fanuc.robotserver.FRELanguageConstants
*/
@DISPID(112) //= 0x70. The runtime will prefer the VTID if present
@VTID(19)
com.github.wshackle.fanuc.robotserver.FRELanguageConstants language();
/**
*
* Gets or sets the language of the robot controller.
*
*
* Setter method for the COM property "Language"
*
* @param language Mandatory com.github.wshackle.fanuc.robotserver.FRELanguageConstants parameter.
*/
@DISPID(112) //= 0x70. The runtime will prefer the VTID if present
@VTID(20)
void language(
com.github.wshackle.fanuc.robotserver.FRELanguageConstants language);
/**
*
* Gets the collection of user defined tool frames for teach pendant programs.
*
*
* Getter method for the COM property "ToolFrames"
*
* @return Returns a value of type com.github.wshackle.fanuc.robotserver.ISysPositions
*/
@DISPID(113) //= 0x71. The runtime will prefer the VTID if present
@VTID(21)
com.github.wshackle.fanuc.robotserver.ISysPositions toolFrames();
/**
*
* Gets the collection of user defined frames for teach pendant programs.
*
*
* Getter method for the COM property "UserFrames"
*
* @return Returns a value of type com.github.wshackle.fanuc.robotserver.ISysPositions
*/
@DISPID(114) //= 0x72. The runtime will prefer the VTID if present
@VTID(22)
com.github.wshackle.fanuc.robotserver.ISysPositions userFrames();
/**
*
* Gets the jog frames of the robot.
*
*
* Getter method for the COM property "JogFrames"
*
* @return Returns a value of type com.github.wshackle.fanuc.robotserver.ISysPositions
*/
@DISPID(115) //= 0x73. The runtime will prefer the VTID if present
@VTID(23)
com.github.wshackle.fanuc.robotserver.ISysPositions jogFrames();
/**
*
* Gets the list of KAREL or TP programs tasks active on the controller.
*
*
* Getter method for the COM property "Tasks"
*
* @return Returns a value of type com.github.wshackle.fanuc.robotserver.ITasks
*/
@DISPID(116) //= 0x74. The runtime will prefer the VTID if present
@VTID(24)
com.github.wshackle.fanuc.robotserver.ITasks tasks();
/**
*
* Gets the list of loaded features for the robot.
*
*
* Getter method for the COM property "SynchData"
*
* @return Returns a value of type com.github.wshackle.fanuc.robotserver.ISynchData
*/
@DISPID(117) //= 0x75. The runtime will prefer the VTID if present
@VTID(25)
com.github.wshackle.fanuc.robotserver.ISynchData synchData();
/**
*
* Gets the Applications collection of the robot.
*
*
* Getter method for the COM property "Applications"
*
* @return Returns a value of type com.github.wshackle.fanuc.robotserver.IApplications
*/
@DISPID(118) //= 0x76. The runtime will prefer the VTID if present
@VTID(26)
com.github.wshackle.fanuc.robotserver.IApplications applications();
/**
*
* Enables/disables the teach pendant as the motion control source.
*
*
* Getter method for the COM property "TPMotionSource"
*
* @return Returns a value of type boolean
*/
@DISPID(119) //= 0x77. The runtime will prefer the VTID if present
@VTID(27)
boolean tpMotionSource();
/**
*
* Enables/disables the teach pendant as the motion control source.
*
*
* Setter method for the COM property "TPMotionSource"
*
* @param tpMotionSource Mandatory boolean parameter.
*/
@DISPID(119) //= 0x77. The runtime will prefer the VTID if present
@VTID(28)
void tpMotionSource(
boolean tpMotionSource);
/**
*
* Returns True if the Robot Server is currently connected offline.
*
*
* Getter method for the COM property "IsOffline"
*
* @return Returns a value of type boolean
*/
@DISPID(120) //= 0x78. The runtime will prefer the VTID if present
@VTID(29)
boolean isOffline();
/**
*
* This property returns a Boolean flag indicating if the object is connected as an event logger.
*
*
* Getter method for the COM property "IsEventLogger"
*
* @return Returns a value of type boolean
*/
@DISPID(121) //= 0x79. The runtime will prefer the VTID if present
@VTID(30)
boolean isEventLogger();
/**
*
* Obsolete. Use ConnectEx method
*
* @param hostName Mandatory java.lang.String parameter.
*/
@DISPID(151) //= 0x97. The runtime will prefer the VTID if present
@VTID(31)
void connect(
java.lang.String hostName);
/**
*
* Loads a file from a controller device into memory.
*
* @param fileName Mandatory java.lang.String parameter.
* @param option Mandatory com.github.wshackle.fanuc.robotserver.FRELoadOptionConstants parameter.
*/
@DISPID(153) //= 0x99. The runtime will prefer the VTID if present
@VTID(33)
void load(
java.lang.String fileName,
com.github.wshackle.fanuc.robotserver.FRELoadOptionConstants option);
/**
*
* Creates a ROS packet event object for receiving events from the robot.
*
* @param subSystemCode Optional parameter. Default value is com4j.Variant.getMissing()
* @param requestCode Optional parameter. Default value is com4j.Variant.getMissing()
* @param packetID Optional parameter. Default value is com4j.Variant.getMissing()
* @return Returns a value of type com.github.wshackle.fanuc.robotserver.IPacketEvent
*/
@DISPID(154) //= 0x9a. The runtime will prefer the VTID if present
@VTID(34)
com.github.wshackle.fanuc.robotserver.IPacketEvent createPacketEvent(
@Optional @MarshalAs(NativeType.VARIANT) java.lang.Object subSystemCode,
@Optional @MarshalAs(NativeType.VARIANT) java.lang.Object requestCode,
@Optional @MarshalAs(NativeType.VARIANT) java.lang.Object packetID);
/**
*
* Connects to an off-line simulated robot controller, specified by a virtual hostname.
*
* @param hostName Optional parameter. Default value is com4j.Variant.getMissing()
* @param mdPath Optional parameter. Default value is com4j.Variant.getMissing()
* @param frsPath Optional parameter. Default value is com4j.Variant.getMissing()
* @param ignoreVersion Optional parameter. Default value is com4j.Variant.getMissing()
*/
@DISPID(155) //= 0x9b. The runtime will prefer the VTID if present
@VTID(35)
void offlineConnect(
@Optional @MarshalAs(NativeType.VARIANT) java.lang.Object hostName,
@Optional @MarshalAs(NativeType.VARIANT) java.lang.Object mdPath,
@Optional @MarshalAs(NativeType.VARIANT) java.lang.Object frsPath,
@Optional @MarshalAs(NativeType.VARIANT) java.lang.Object ignoreVersion);
/**
*
* Returns extended error information for the last error thrown.
*
* @return Returns a value of type com.github.wshackle.fanuc.robotserver.IRobotErrorInfo
*/
@DISPID(156) //= 0x9c. The runtime will prefer the VTID if present
@VTID(36)
com.github.wshackle.fanuc.robotserver.IRobotErrorInfo getErrorInfo();
/**
*
* Connects the Robot object as an event logger service.
*
*/
@DISPID(157) //= 0x9d. The runtime will prefer the VTID if present
@VTID(37)
void eventLoggerConnect();
/**
* @param command Mandatory java.lang.String parameter.
* @param wait Mandatory boolean parameter.
*/
@DISPID(158) //= 0x9e. The runtime will prefer the VTID if present
@VTID(38)
void kcl(
java.lang.String command,
boolean wait);
/**
* @param fileName Mandatory java.lang.String parameter.
*/
@DISPID(159) //= 0x9f. The runtime will prefer the VTID if present
@VTID(39)
void spruncmd(
java.lang.String fileName);
/**
* @param message Mandatory java.lang.String parameter.
*/
@DISPID(160) //= 0xa0. The runtime will prefer the VTID if present
@VTID(40)
void outputDebugMessage(
java.lang.String message);
/**
*
* Attempts connection to the robot controller specified by HostName the number of times specified by NumRetries at a the Period specified. NoWait = TRUE will return immediately.
*
* @param hostName Mandatory java.lang.String parameter.
* @param noWait Mandatory boolean parameter.
* @param numRetries Mandatory int parameter.
* @param period Mandatory int parameter.
*/
@DISPID(161) //= 0xa1. The runtime will prefer the VTID if present
@VTID(41)
void connectEx(
java.lang.String hostName,
boolean noWait,
int numRetries,
int period);
/**
*
* Returns whether the Robot Object is connected to a robot controller.
*
*
* Getter method for the COM property "IsConnected"
*
* @return Returns a value of type boolean
*/
@DISPID(162) //= 0xa2. The runtime will prefer the VTID if present
@VTID(42)
boolean isConnected();
/**
*
* Returns an FRCSysInfo object from which system information can be obtained.
*
*
* Getter method for the COM property "SysInfo"
*
* @return Returns a value of type com.github.wshackle.fanuc.robotserver.ISysInfo
*/
@DISPID(164) //= 0xa4. The runtime will prefer the VTID if present
@VTID(44)
com.github.wshackle.fanuc.robotserver.ISysInfo sysInfo();
/**
*
* Returns the list of pipes currently registered on the controller.
*
*
* Getter method for the COM property "Pipes"
*
* @return Returns a value of type com.github.wshackle.fanuc.robotserver.IPipes
*/
@DISPID(165) //= 0xa5. The runtime will prefer the VTID if present
@VTID(45)
com.github.wshackle.fanuc.robotserver.IPipes pipes();
/**
*
* Creates a new FRCIndPosition class object for your use.
*
* @param groupBitMask Optional parameter. Default value is 0
* @return Returns a value of type com.github.wshackle.fanuc.robotserver.IIndPosition
*/
@DISPID(166) //= 0xa6. The runtime will prefer the VTID if present
@VTID(46)
com.github.wshackle.fanuc.robotserver.IIndPosition createIndependentPosition(
@Optional @DefaultValue("0") com.github.wshackle.fanuc.robotserver.FREGroupBitMaskConstants groupBitMask);
/**
*
* Process ID
*
*
* Getter method for the COM property "ProcessID"
*
* @return Returns a value of type int
*/
@DISPID(167) //= 0xa7. The runtime will prefer the VTID if present
@VTID(47)
int processID();
/**
*
* Gets the string registers of the robot.
*
*
* Getter method for the COM property "RegStrings"
*
* @return Returns a value of type com.github.wshackle.fanuc.robotserver.IVars
*/
@DISPID(168) //= 0xa8. The runtime will prefer the VTID if present
@VTID(48)
com.github.wshackle.fanuc.robotserver.IVars regStrings();
// Properties:
}