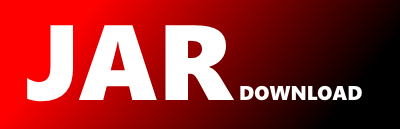
com.github.wshackle.fanuc.robotserver.ISysInfo Maven / Gradle / Ivy
package com.github.wshackle.fanuc.robotserver ;
import com4j.*;
/**
* Provides access to robot controller system information.
*/
@IID("{4553DA61-ACA1-11D3-8783-00C04F81118D}")
public interface ISysInfo extends com.github.wshackle.fanuc.robotserver.IRobotObject {
// Methods:
/**
*
* Returns/sets the date and time on the robot's system clock.
*
*
* Getter method for the COM property "Clock"
*
* @return Returns a value of type java.util.Date
*/
@DISPID(101) //= 0x65. The runtime will prefer the VTID if present
@VTID(8)
java.util.Date clock();
/**
*
* Returns/sets the date and time on the robot's system clock.
*
*
* Setter method for the COM property "Clock"
*
* @param dateTime Mandatory java.util.Date parameter.
*/
@DISPID(101) //= 0x65. The runtime will prefer the VTID if present
@VTID(9)
void clock(
java.util.Date dateTime);
/**
*
* Returns the number of bytes of battery backed CMOS hardware configured on the controller.
*
*
* Getter method for the COM property "CMOS"
*
* @return Returns a value of type int
*/
@DISPID(102) //= 0x66. The runtime will prefer the VTID if present
@VTID(10)
int cmos();
/**
*
* Returns the number of bytes of DRAM hardware configured on the controller.
*
*
* Getter method for the COM property "DRAM"
*
* @return Returns a value of type int
*/
@DISPID(103) //= 0x67. The runtime will prefer the VTID if present
@VTID(11)
int dram();
/**
*
* Returns the number of bytes of Flash ROM (FROM) hardware configured on the controller.
*
*
* Getter method for the COM property "From"
*
* @return Returns a value of type int
*/
@DISPID(104) //= 0x68. The runtime will prefer the VTID if present
@VTID(12)
int from();
/**
*
* Returns the number of bytes allocated to the PERM memory pool.
*
*
* Getter method for the COM property "PermMemTotal"
*
* @return Returns a value of type int
*/
@DISPID(105) //= 0x69. The runtime will prefer the VTID if present
@VTID(13)
int permMemTotal();
/**
*
* Returns the number of bytes available in the PERM memory pool.
*
*
* Getter method for the COM property "PermMemFree"
*
* @return Returns a value of type int
*/
@DISPID(106) //= 0x6a. The runtime will prefer the VTID if present
@VTID(14)
int permMemFree();
/**
*
* Returns the size of the largest free block in the PERM memory pool.
*
*
* Getter method for the COM property "PermMemLargestFreeBlock"
*
* @return Returns a value of type int
*/
@DISPID(107) //= 0x6b. The runtime will prefer the VTID if present
@VTID(15)
int permMemLargestFreeBlock();
/**
*
* Returns the number of bytes used out of the PERM memory pool.
*
*
* Getter method for the COM property "PermMemUsed"
*
* @return Returns a value of type int
*/
@DISPID(108) //= 0x6c. The runtime will prefer the VTID if present
@VTID(16)
int permMemUsed();
/**
*
* Returns the number of bytes allocated to the TEMP memory pool.
*
*
* Getter method for the COM property "TempMemTotal"
*
* @return Returns a value of type int
*/
@DISPID(109) //= 0x6d. The runtime will prefer the VTID if present
@VTID(17)
int tempMemTotal();
/**
*
* Returns the number of bytes available in the TEMP memory pool.
*
*
* Getter method for the COM property "TempMemFree"
*
* @return Returns a value of type int
*/
@DISPID(110) //= 0x6e. The runtime will prefer the VTID if present
@VTID(18)
int tempMemFree();
/**
*
* Returns the size of the largest free block in the TEMP memory pool.
*
*
* Getter method for the COM property "TempMemLargestFreeBlock"
*
* @return Returns a value of type int
*/
@DISPID(111) //= 0x6f. The runtime will prefer the VTID if present
@VTID(19)
int tempMemLargestFreeBlock();
/**
*
* Returns the number of bytes used out of the TEMP memory pool.
*
*
* Getter method for the COM property "TempMemUsed"
*
* @return Returns a value of type int
*/
@DISPID(112) //= 0x70. The runtime will prefer the VTID if present
@VTID(20)
int tempMemUsed();
/**
*
* Returns the number of bytes allocated to the TPP memory pool.
*
*
* Getter method for the COM property "TPPMemTotal"
*
* @return Returns a value of type int
*/
@DISPID(113) //= 0x71. The runtime will prefer the VTID if present
@VTID(21)
int tppMemTotal();
/**
*
* Returns the number of bytes available in the TPP memory pool.
*
*
* Getter method for the COM property "TPPMemFree"
*
* @return Returns a value of type int
*/
@DISPID(114) //= 0x72. The runtime will prefer the VTID if present
@VTID(22)
int tppMemFree();
/**
*
* Returns the size of the largest free block in the TPP memory pool.
*
*
* Getter method for the COM property "TPPMemLargestFreeBlock"
*
* @return Returns a value of type int
*/
@DISPID(115) //= 0x73. The runtime will prefer the VTID if present
@VTID(23)
int tppMemLargestFreeBlock();
/**
*
* Returns the number of bytes used out of the TPP memory pool.
*
*
* Getter method for the COM property "TPPMemUsed"
*
* @return Returns a value of type int
*/
@DISPID(116) //= 0x74. The runtime will prefer the VTID if present
@VTID(24)
int tppMemUsed();
/**
*
* Returns the number of bytes allocated to the SYSTEM memory pool.
*
*
* Getter method for the COM property "SystemMemTotal"
*
* @return Returns a value of type int
*/
@DISPID(117) //= 0x75. The runtime will prefer the VTID if present
@VTID(25)
int systemMemTotal();
/**
*
* Returns the number of bytes available in the SYSTEM memory pool.
*
*
* Getter method for the COM property "SystemMemFree"
*
* @return Returns a value of type int
*/
@DISPID(118) //= 0x76. The runtime will prefer the VTID if present
@VTID(26)
int systemMemFree();
/**
*
* Returns the size of the largest free block in the SYSTEM memory pool.
*
*
* Getter method for the COM property "SystemMemLargestFreeBlock"
*
* @return Returns a value of type int
*/
@DISPID(119) //= 0x77. The runtime will prefer the VTID if present
@VTID(27)
int systemMemLargestFreeBlock();
/**
*
* Returns the number of bytes used out of the SYSTEM memory pool.
*
*
* Getter method for the COM property "SystemMemUsed"
*
* @return Returns a value of type int
*/
@DISPID(120) //= 0x78. The runtime will prefer the VTID if present
@VTID(28)
int systemMemUsed();
/**
*
* Returns a number representing the manufacturer and type of Flash ROM installed on the controller.
*
*
* Getter method for the COM property "FROMType"
*
* @return Returns a value of type int
*/
@DISPID(121) //= 0x79. The runtime will prefer the VTID if present
@VTID(29)
int fromType();
/**
*
* Returns a constant indicating in which start mode the controller is operating.
*
*
* Getter method for the COM property "StartMode"
*
* @return Returns a value of type com.github.wshackle.fanuc.robotserver.FREStartModeConstants
*/
@DISPID(122) //= 0x7a. The runtime will prefer the VTID if present
@VTID(30)
com.github.wshackle.fanuc.robotserver.FREStartModeConstants startMode();
// Properties:
}