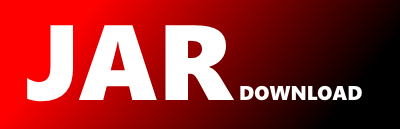
com.github.wshackle.fanuc.robotserver.ITask Maven / Gradle / Ivy
package com.github.wshackle.fanuc.robotserver ;
import com4j.*;
/**
* The task object represents a running thread of execution on the controller. A running task may be running different programs or program routines at various points in time.
*/
@IID("{7745C8FE-4626-11D1-B745-00C04FBBE42A}")
public interface ITask extends com.github.wshackle.fanuc.robotserver.IRobotObject {
// Methods:
/**
*
* Returns/sets the state of the busy lamp behavior.
*
*
* Getter method for the COM property "BusyLampOff"
*
* @return Returns a value of type boolean
*/
@DISPID(201) //= 0xc9. The runtime will prefer the VTID if present
@VTID(8)
boolean busyLampOff();
/**
*
* Returns/sets the state of the busy lamp behavior.
*
*
* Setter method for the COM property "BusyLampOff"
*
* @param busyLampOff Mandatory boolean parameter.
*/
@DISPID(201) //= 0xc9. The runtime will prefer the VTID if present
@VTID(9)
void busyLampOff(
boolean busyLampOff);
/**
*
* Returns the current line of execution in the current program.
*
*
* Getter method for the COM property "CurLine"
*
* @return Returns a value of type int
*/
@DISPID(202) //= 0xca. The runtime will prefer the VTID if present
@VTID(10)
int curLine();
/**
*
* Returns the current program that is being executed.
*
*
* Getter method for the COM property "CurProgram"
*
* @return Returns a value of type com.github.wshackle.fanuc.robotserver.IProgram
*/
@DISPID(203) //= 0xcb. The runtime will prefer the VTID if present
@VTID(11)
com.github.wshackle.fanuc.robotserver.IProgram curProgram();
/**
*
* Returns the name of the currently executing KAREL routine.
*
*
* Getter method for the COM property "CurRoutine"
*
* @return Returns a value of type java.lang.String
*/
@DISPID(204) //= 0xcc. The runtime will prefer the VTID if present
@VTID(12)
java.lang.String curRoutine();
/**
*
* Returns the state of the various hold flags.
*
*
* Getter method for the COM property "HoldCondition"
*
* @param holdCondition Mandatory com.github.wshackle.fanuc.robotserver.FREHoldConditionConstants parameter.
* @return Returns a value of type boolean
*/
@DISPID(205) //= 0xcd. The runtime will prefer the VTID if present
@VTID(13)
boolean holdCondition(
com.github.wshackle.fanuc.robotserver.FREHoldConditionConstants holdCondition);
/**
*
* Returns/sets the ignore abort flags for the task.
*
*
* Getter method for the COM property "IgnoreAbort"
*
* @param ignoreConstant Mandatory com.github.wshackle.fanuc.robotserver.FRETaskIgnoreConstants parameter.
* @return Returns a value of type boolean
*/
@DISPID(206) //= 0xce. The runtime will prefer the VTID if present
@VTID(14)
boolean ignoreAbort(
com.github.wshackle.fanuc.robotserver.FRETaskIgnoreConstants ignoreConstant);
/**
*
* Returns/sets the ignore abort flags for the task.
*
*
* Setter method for the COM property "IgnoreAbort"
*
* @param ignoreConstant Mandatory com.github.wshackle.fanuc.robotserver.FRETaskIgnoreConstants parameter.
* @param ignore Mandatory boolean parameter.
*/
@DISPID(206) //= 0xce. The runtime will prefer the VTID if present
@VTID(15)
void ignoreAbort(
com.github.wshackle.fanuc.robotserver.FRETaskIgnoreConstants ignoreConstant,
boolean ignore);
/**
*
* Returns/sets the ignore pause flags for the task.
*
*
* Getter method for the COM property "IgnorePause"
*
* @param ignoreConstant Mandatory com.github.wshackle.fanuc.robotserver.FRETaskIgnoreConstants parameter.
* @return Returns a value of type boolean
*/
@DISPID(207) //= 0xcf. The runtime will prefer the VTID if present
@VTID(16)
boolean ignorePause(
com.github.wshackle.fanuc.robotserver.FRETaskIgnoreConstants ignoreConstant);
/**
*
* Returns/sets the ignore pause flags for the task.
*
*
* Setter method for the COM property "IgnorePause"
*
* @param ignoreConstant Mandatory com.github.wshackle.fanuc.robotserver.FRETaskIgnoreConstants parameter.
* @param ignore Mandatory boolean parameter.
*/
@DISPID(207) //= 0xcf. The runtime will prefer the VTID if present
@VTID(17)
void ignorePause(
com.github.wshackle.fanuc.robotserver.FRETaskIgnoreConstants ignoreConstant,
boolean ignore);
/**
*
* Returns whether the task shows in the task list.
*
*
* Getter method for the COM property "Invisible"
*
* @return Returns a value of type boolean
*/
@DISPID(208) //= 0xd0. The runtime will prefer the VTID if present
@VTID(18)
boolean invisible();
/**
*
* Returns whether the task shows in the task list.
*
*
* Setter method for the COM property "Invisible"
*
* @param invisible Mandatory boolean parameter.
*/
@DISPID(208) //= 0xd0. The runtime will prefer the VTID if present
@VTID(19)
void invisible(
boolean invisible);
/**
*
* Returns/sets whether the task pauses on Shift release.
*
*
* Getter method for the COM property "PauseOnShift"
*
* @return Returns a value of type boolean
*/
@DISPID(209) //= 0xd1. The runtime will prefer the VTID if present
@VTID(20)
boolean pauseOnShift();
/**
*
* Returns/sets whether the task pauses on Shift release.
*
*
* Setter method for the COM property "PauseOnShift"
*
* @param pauseOnShift Mandatory boolean parameter.
*/
@DISPID(209) //= 0xd1. The runtime will prefer the VTID if present
@VTID(21)
void pauseOnShift(
boolean pauseOnShift);
/**
*
* Returns whether the task is a system task.
*
*
* Getter method for the COM property "SystemTask"
*
* @return Returns a value of type boolean
*/
@DISPID(210) //= 0xd2. The runtime will prefer the VTID if present
@VTID(22)
boolean systemTask();
/**
*
* Returns whether the task is a system task.
*
*
* Setter method for the COM property "SystemTask"
*
* @param systemTask Mandatory boolean parameter.
*/
@DISPID(210) //= 0xd2. The runtime will prefer the VTID if present
@VTID(23)
void systemTask(
boolean systemTask);
/**
*
* Returns/sets whether the task has motion when the TP is enabled.
*
*
* Getter method for the COM property "TPMotion"
*
* @return Returns a value of type boolean
*/
@DISPID(211) //= 0xd3. The runtime will prefer the VTID if present
@VTID(24)
boolean tpMotion();
/**
*
* Returns/sets whether the task has motion when the TP is enabled.
*
*
* Setter method for the COM property "TPMotion"
*
* @param tpMotion Mandatory boolean parameter.
*/
@DISPID(211) //= 0xd3. The runtime will prefer the VTID if present
@VTID(25)
void tpMotion(
boolean tpMotion);
/**
*
* Returns/sets whether the task has tracing enabled.
*
*
* Getter method for the COM property "TraceEnable"
*
* @return Returns a value of type boolean
*/
@DISPID(212) //= 0xd4. The runtime will prefer the VTID if present
@VTID(26)
boolean traceEnable();
/**
*
* Returns/sets whether the task has tracing enabled.
*
*
* Setter method for the COM property "TraceEnable"
*
* @param traceEnable Mandatory boolean parameter.
*/
@DISPID(212) //= 0xd4. The runtime will prefer the VTID if present
@VTID(27)
void traceEnable(
boolean traceEnable);
/**
*
* Returns/sets whether the operator can write program variables even if the task is running.
*
*
* Getter method for the COM property "VarWriteEnable"
*
* @return Returns a value of type boolean
*/
@DISPID(213) //= 0xd5. The runtime will prefer the VTID if present
@VTID(28)
boolean varWriteEnable();
/**
*
* Returns/sets whether the operator can write program variables even if the task is running.
*
*
* Setter method for the COM property "VarWriteEnable"
*
* @param varWriteEnable Mandatory boolean parameter.
*/
@DISPID(213) //= 0xd5. The runtime will prefer the VTID if present
@VTID(29)
void varWriteEnable(
boolean varWriteEnable);
/**
*
* Returns whether the task has any motion groups locked.
*
*
* Getter method for the COM property "LockedArm"
*
* @param groupNum Mandatory short parameter.
* @return Returns a value of type boolean
*/
@DISPID(214) //= 0xd6. The runtime will prefer the VTID if present
@VTID(30)
boolean lockedArm(
short groupNum);
/**
*
* Returns whether the task has motion control of a group.
*
*
* Getter method for the COM property "MotionControl"
*
* @param groupNum Mandatory short parameter.
* @return Returns a value of type boolean
*/
@DISPID(215) //= 0xd7. The runtime will prefer the VTID if present
@VTID(31)
boolean motionControl(
short groupNum);
/**
*
* Returns/sets the name associated with the task.
*
*
* Getter method for the COM property "Name"
*
* @return Returns a value of type java.lang.String
*/
@DISPID(216) //= 0xd8. The runtime will prefer the VTID if present
@VTID(32)
java.lang.String name();
/**
*
* Returns/sets the name associated with the task.
*
*
* Setter method for the COM property "Name"
*
* @param name Mandatory java.lang.String parameter.
*/
@DISPID(216) //= 0xd8. The runtime will prefer the VTID if present
@VTID(33)
void name(
java.lang.String name);
/**
*
* Returns whether the task performs circular motion.
*
*
* Getter method for the COM property "NotCircularMotion"
*
* @return Returns a value of type boolean
*/
@DISPID(217) //= 0xd9. The runtime will prefer the VTID if present
@VTID(34)
boolean notCircularMotion();
/**
*
* Returns whether the task performs circular motion.
*
*
* Setter method for the COM property "NotCircularMotion"
*
* @param notCircularMotion Mandatory boolean parameter.
*/
@DISPID(217) //= 0xd9. The runtime will prefer the VTID if present
@VTID(35)
void notCircularMotion(
boolean notCircularMotion);
/**
*
* Returns the number of child tasks created by this task.
*
*
* Getter method for the COM property "NumChild"
*
* @return Returns a value of type short
*/
@DISPID(218) //= 0xda. The runtime will prefer the VTID if present
@VTID(36)
short numChild();
/**
*
* Returns the number of MMRs associated with the task.
*
*
* Getter method for the COM property "NumMMR"
*
* @return Returns a value of type short
*/
@DISPID(219) //= 0xdb. The runtime will prefer the VTID if present
@VTID(37)
short numMMR();
/**
*
* Returns/sets the current priority of the task.
*
*
* Getter method for the COM property "Priority"
*
* @return Returns a value of type short
*/
@DISPID(220) //= 0xdc. The runtime will prefer the VTID if present
@VTID(38)
short priority();
/**
*
* Returns/sets the current priority of the task.
*
*
* Setter method for the COM property "Priority"
*
* @param priority Mandatory short parameter.
*/
@DISPID(220) //= 0xdc. The runtime will prefer the VTID if present
@VTID(39)
void priority(
short priority);
/**
*
* Returns the type of program the task represents
*
*
* Getter method for the COM property "ProgramType"
*
* @return Returns a value of type com.github.wshackle.fanuc.robotserver.FREProgramTypeConstants
*/
@DISPID(221) //= 0xdd. The runtime will prefer the VTID if present
@VTID(40)
com.github.wshackle.fanuc.robotserver.FREProgramTypeConstants programType();
/**
*
* Returns the stack size for the task.
*
*
* Getter method for the COM property "StackSize"
*
* @return Returns a value of type int
*/
@DISPID(222) //= 0xde. The runtime will prefer the VTID if present
@VTID(41)
int stackSize();
/**
*
* Returns the current status of the task.
*
*
* Getter method for the COM property "Status"
*
* @return Returns a value of type com.github.wshackle.fanuc.robotserver.FRETaskStatusConstants
*/
@DISPID(223) //= 0xdf. The runtime will prefer the VTID if present
@VTID(42)
com.github.wshackle.fanuc.robotserver.FRETaskStatusConstants status();
/**
*
* Returns the task statement stepping type.
*
*
* Getter method for the COM property "StepType"
*
* @return Returns a value of type com.github.wshackle.fanuc.robotserver.FREStepTypeConstants
*/
@DISPID(224) //= 0xe0. The runtime will prefer the VTID if present
@VTID(43)
com.github.wshackle.fanuc.robotserver.FREStepTypeConstants stepType();
/**
*
* Returns the task statement stepping type.
*
*
* Setter method for the COM property "StepType"
*
* @param stepType Mandatory com.github.wshackle.fanuc.robotserver.FREStepTypeConstants parameter.
*/
@DISPID(224) //= 0xe0. The runtime will prefer the VTID if present
@VTID(44)
void stepType(
com.github.wshackle.fanuc.robotserver.FREStepTypeConstants stepType);
/**
*
* Returns/sets whether the task has supervisory motion control of any group.
*
*
* Getter method for the COM property "SuperMotion"
*
* @param groupNum Mandatory short parameter.
* @return Returns a value of type boolean
*/
@DISPID(225) //= 0xe1. The runtime will prefer the VTID if present
@VTID(45)
boolean superMotion(
short groupNum);
/**
*
* Returns/sets whether the task has supervisory motion control of any group.
*
*
* Setter method for the COM property "SuperMotion"
*
* @param groupNum Mandatory short parameter.
* @param superMotion Mandatory boolean parameter.
*/
@DISPID(225) //= 0xe1. The runtime will prefer the VTID if present
@VTID(46)
void superMotion(
short groupNum,
boolean superMotion);
/**
*
* Returns the number of the task.
*
*
* Getter method for the COM property "TaskNum"
*
* @return Returns a value of type short
*/
@DISPID(226) //= 0xe2. The runtime will prefer the VTID if present
@VTID(47)
short taskNum();
/**
*
* Returns the current status of the task TCDs.
*
*
* Getter method for the COM property "TCDStatus"
*
* @return Returns a value of type int
*/
@DISPID(227) //= 0xe3. The runtime will prefer the VTID if present
@VTID(48)
int tcdStatus();
/**
*
* Returns the allotted time slice for the task.
*
*
* Getter method for the COM property "TimeSlice"
*
* @return Returns a value of type int
*/
@DISPID(228) //= 0xe4. The runtime will prefer the VTID if present
@VTID(49)
int timeSlice();
/**
*
* Returns/sets the length of the trace buffer for this task.
*
*
* Getter method for the COM property "TraceLength"
*
* @return Returns a value of type int
*/
@DISPID(229) //= 0xe5. The runtime will prefer the VTID if present
@VTID(50)
int traceLength();
/**
*
* Returns/sets the length of the trace buffer for this task.
*
*
* Setter method for the COM property "TraceLength"
*
* @param traceLength Mandatory int parameter.
*/
@DISPID(229) //= 0xe5. The runtime will prefer the VTID if present
@VTID(51)
void traceLength(
int traceLength);
/**
*
* Returns the top level program object.
*
*
* Getter method for the COM property "TopProgram"
*
* @return Returns a value of type com.github.wshackle.fanuc.robotserver.IProgram
*/
@DISPID(230) //= 0xe6. The runtime will prefer the VTID if present
@VTID(52)
com.github.wshackle.fanuc.robotserver.IProgram topProgram();
/**
*
* Aborts the current execution of the task.
*
* @param force Optional parameter. Default value is com4j.Variant.getMissing()
* @param cancel Optional parameter. Default value is com4j.Variant.getMissing()
*/
@DISPID(250) //= 0xfa. The runtime will prefer the VTID if present
@VTID(53)
void abort(
@Optional @MarshalAs(NativeType.VARIANT) java.lang.Object force,
@Optional @MarshalAs(NativeType.VARIANT) java.lang.Object cancel);
/**
*
* Pauses the task at its current line.
*
* @param force Optional parameter. Default value is com4j.Variant.getMissing()
* @param cancel Optional parameter. Default value is com4j.Variant.getMissing()
*/
@DISPID(251) //= 0xfb. The runtime will prefer the VTID if present
@VTID(54)
void pause(
@Optional @MarshalAs(NativeType.VARIANT) java.lang.Object force,
@Optional @MarshalAs(NativeType.VARIANT) java.lang.Object cancel);
/**
*
* Executes a task after it has been stopped by the Pause method.
*
* @param lineNum Optional parameter. Default value is com4j.Variant.getMissing()
* @param direction Optional parameter. Default value is com4j.Variant.getMissing()
*/
@DISPID(252) //= 0xfc. The runtime will prefer the VTID if present
@VTID(55)
void _continue(
@Optional @MarshalAs(NativeType.VARIANT) java.lang.Object lineNum,
@Optional @MarshalAs(NativeType.VARIANT) java.lang.Object direction);
/**
*
* Causes one or more statements to be skipped. The next statement to be executed is determined by the current value of the StepType property.
*
* @param number Optional parameter. Default value is com4j.Variant.getMissing()
*/
@DISPID(253) //= 0xfd. The runtime will prefer the VTID if present
@VTID(56)
void skip(
@Optional @MarshalAs(NativeType.VARIANT) java.lang.Object number);
// Properties:
}