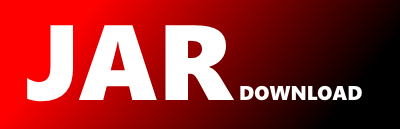
com.github.wz2cool.dynamic.UpdateQuery Maven / Gradle / Ivy
package com.github.wz2cool.dynamic;
import com.github.wz2cool.dynamic.exception.InternalRuntimeException;
import com.github.wz2cool.dynamic.helper.CommonsHelper;
import com.github.wz2cool.dynamic.lambda.*;
import com.github.wz2cool.dynamic.model.SelectPropertyConfig;
import com.github.wz2cool.dynamic.mybatis.ColumnInfo;
import com.github.wz2cool.dynamic.mybatis.ParamExpression;
import com.github.wz2cool.dynamic.mybatis.QueryHelper;
import com.github.wz2cool.dynamic.mybatis.mapper.constant.MapperConstants;
import java.math.BigDecimal;
import java.util.*;
import java.util.function.UnaryOperator;
/**
* @author Frank
**/
public class UpdateQuery extends BaseFilterGroup> {
private static final QueryHelper QUERY_HELPER = new QueryHelper();
private final Map setColumnValueMap = new HashMap<>();
private Class entityClass;
public UpdateQuery() {
// for json
}
public UpdateQuery(Class entityClass) {
this.entityClass = entityClass;
}
public static UpdateQuery createQuery(Class entityClass) {
return new UpdateQuery<>(entityClass);
}
public Class getEntityClass() {
return entityClass;
}
public UpdateQuery set(GetBigDecimalPropertyFunction getPropertyFunc, BigDecimal value) {
return set(true, getPropertyFunc, value);
}
public UpdateQuery set(GetBytePropertyFunction getPropertyFunc, Byte value) {
return set(true, getPropertyFunc, value);
}
public UpdateQuery set(GetDatePropertyFunction getPropertyFunc, Date value) {
return set(true, getPropertyFunc, value);
}
public UpdateQuery set(GetDoublePropertyFunction getPropertyFunc, Double value) {
return set(true, getPropertyFunc, value);
}
public UpdateQuery set(GetFloatPropertyFunction getPropertyFunc, Float value) {
return set(true, getPropertyFunc, value);
}
public UpdateQuery set(GetIntegerPropertyFunction getPropertyFunc, Integer value) {
return set(true, getPropertyFunc, value);
}
public UpdateQuery set(GetLongPropertyFunction getPropertyFunc, Long value) {
return set(true, getPropertyFunc, value);
}
public UpdateQuery set(GetShortPropertyFunction getPropertyFunc, Short value) {
return set(true, getPropertyFunc, value);
}
public UpdateQuery set(GetStringPropertyFunction getPropertyFunc, String value) {
return set(true, getPropertyFunc, value);
}
public UpdateQuery set(T record) {
return set(true, record);
}
public UpdateQuery set(boolean enable, T record) {
return set(enable, record, null);
}
public UpdateQuery set(T record, UnaryOperator> getConfigFunc) {
return set(true, record, getConfigFunc);
}
public UpdateQuery set(boolean enable, T record, UnaryOperator> getConfigFunc) {
if (enable) {
SelectPropertyConfig config = null;
if (Objects.nonNull(getConfigFunc)) {
config = getConfigFunc.apply(new SelectPropertyConfig<>());
}
Map propertyColumnInfoMap = QUERY_HELPER.getPropertyColumnInfoMap(record.getClass());
final Set needUpdatePropertyNames = getNeedUpdatePropertyNames(propertyColumnInfoMap.keySet(), config);
for (Map.Entry propertyColumnInfoEntry : propertyColumnInfoMap.entrySet()) {
String propertyName = propertyColumnInfoEntry.getKey();
ColumnInfo columnInfo = propertyColumnInfoEntry.getValue();
try {
if (needUpdatePropertyNames.contains(propertyName)) {
Object value = columnInfo.getField().get(record);
setColumnValueMap.put(propertyName, value);
}
} catch (IllegalAccessException e) {
throw new InternalRuntimeException(e);
}
}
}
return this;
}
public UpdateQuery set(boolean enable, GetBigDecimalPropertyFunction getPropertyFunc, BigDecimal value) {
if (enable) {
final String propertyName = CommonsHelper.getPropertyName(getPropertyFunc);
setColumnValueMap.put(propertyName, value);
}
return this;
}
public UpdateQuery set(boolean enable, GetBytePropertyFunction getPropertyFunc, Byte value) {
if (enable) {
final String propertyName = CommonsHelper.getPropertyName(getPropertyFunc);
setColumnValueMap.put(propertyName, value);
}
return this;
}
public UpdateQuery set(boolean enable, GetDatePropertyFunction getPropertyFunc, Date value) {
if (enable) {
final String propertyName = CommonsHelper.getPropertyName(getPropertyFunc);
setColumnValueMap.put(propertyName, value);
}
return this;
}
public UpdateQuery set(boolean enable, GetDoublePropertyFunction getPropertyFunc, Double value) {
if (enable) {
final String propertyName = CommonsHelper.getPropertyName(getPropertyFunc);
setColumnValueMap.put(propertyName, value);
}
return this;
}
public UpdateQuery set(boolean enable, GetFloatPropertyFunction getPropertyFunc, Float value) {
if (enable) {
final String propertyName = CommonsHelper.getPropertyName(getPropertyFunc);
setColumnValueMap.put(propertyName, value);
}
return this;
}
public UpdateQuery set(boolean enable, GetIntegerPropertyFunction getPropertyFunc, Integer value) {
if (enable) {
final String propertyName = CommonsHelper.getPropertyName(getPropertyFunc);
setColumnValueMap.put(propertyName, value);
}
return this;
}
public UpdateQuery set(boolean enable, GetLongPropertyFunction getPropertyFunc, Long value) {
if (enable) {
final String propertyName = CommonsHelper.getPropertyName(getPropertyFunc);
setColumnValueMap.put(propertyName, value);
}
return this;
}
public UpdateQuery set(boolean enable, GetShortPropertyFunction getPropertyFunc, Short value) {
if (enable) {
final String propertyName = CommonsHelper.getPropertyName(getPropertyFunc);
setColumnValueMap.put(propertyName, value);
}
return this;
}
public UpdateQuery set(boolean enable, GetStringPropertyFunction getPropertyFunc, String value) {
if (enable) {
final String propertyName = CommonsHelper.getPropertyName(getPropertyFunc);
setColumnValueMap.put(propertyName, value);
}
return this;
}
public Map toQueryParamMap(boolean isMapUnderscoreToCamelCase) {
BaseFilterDescriptor[] filters = this.getFilters();
ParamExpression whereParamExpression = QUERY_HELPER.toWhereExpression(entityClass, filters);
String whereExpression = whereParamExpression.getExpression();
Map paramMap = whereParamExpression.getParamMap();
for (Map.Entry param : paramMap.entrySet()) {
String key = param.getKey();
String newKey = String.format("%s.%s", MapperConstants.DYNAMIC_QUERY_PARAMS, key);
whereExpression = whereExpression.replace(key, newKey);
}
paramMap.put(MapperConstants.WHERE_EXPRESSION, whereExpression);
final Map updateParamMap = toUpdateParamMap(isMapUnderscoreToCamelCase, setColumnValueMap);
paramMap.putAll(updateParamMap);
return paramMap;
}
private Map toUpdateParamMap(boolean isMapUnderscoreToCamelCase, Map setColumnValueMap) {
Map result = new HashMap<>();
List setExpressionItems = new ArrayList<>();
for (Map.Entry columnValueEntry : setColumnValueMap.entrySet()) {
String propertyName = columnValueEntry.getKey();
String valuePlaceHolder = String.format("param_%s", columnValueEntry.getKey());
final String columnName = QUERY_HELPER.getQueryColumnByProperty(this.getEntityClass(), propertyName);
final String setExpressionItem = String.format("%s=#{%s.%s}",
columnName, MapperConstants.DYNAMIC_QUERY_PARAMS, valuePlaceHolder);
setExpressionItems.add(setExpressionItem);
result.put(valuePlaceHolder, columnValueEntry.getValue());
}
String setExpression = String.join(",", setExpressionItems);
result.put(MapperConstants.SET_EXPRESSION, setExpression);
return result;
}
private Set getNeedUpdatePropertyNames(Set allPropertyNames, SelectPropertyConfig config) {
if (Objects.isNull(config)) {
return allPropertyNames;
}
if (!config.getSelectPropertyNames().isEmpty()) {
return config.getSelectPropertyNames();
}
Set result = new HashSet<>();
for (String propertyName : allPropertyNames) {
if (config.getIgnorePropertyNames().contains(propertyName)) {
continue;
}
result.add(propertyName);
}
return result;
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy