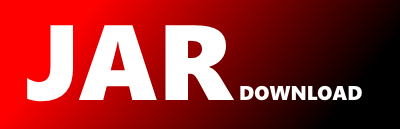
com.github.xdcrafts.flower.tools.MapApi Maven / Gradle / Ivy
/*
* Copyright (c) 2017 Vadim Dubs https://github.com/xdcrafts
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing,
* software distributed under the License is distributed on an
* "AS IS" BASIS, WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND,
* either express or implied. See the License for the specific
* language governing permissions and limitations under the License.
*/
package com.github.xdcrafts.flower.tools;
import java.util.Arrays;
import java.util.List;
import java.util.HashSet;
import java.util.Map;
import java.util.Optional;
import java.util.Set;
import java.util.stream.Collectors;
/**
* Utility methods for Map.
*/
@SuppressWarnings("unchecked")
public final class MapApi {
private static final String PATH_MUST_BE_SPECIFIED = "Path must be specified!";
/**
* Private constructor.
*/
private MapApi() {
// Nothing
}
/**
* Walks by map's nodes and extracts optional value of type T.
* @param value type
* @param clazz type of value
* @param map subject
* @param path nodes to walk in map
* @return optional value of type T
*/
public static Optional get(final Map map, final Class clazz, final Object... path) {
if (path == null || path.length == 0) {
throw new IllegalArgumentException(PATH_MUST_BE_SPECIFIED);
}
if (path.length == 1) {
return Optional.ofNullable((T) map.get(path[0]));
}
final Object[] pathToLastNode = Arrays.copyOfRange(path, 0, path.length - 1);
final Object lastKey = path[path.length - 1];
Map
© 2015 - 2025 Weber Informatics LLC | Privacy Policy